Boosting Java Lambda Performance for Low Latency Systems
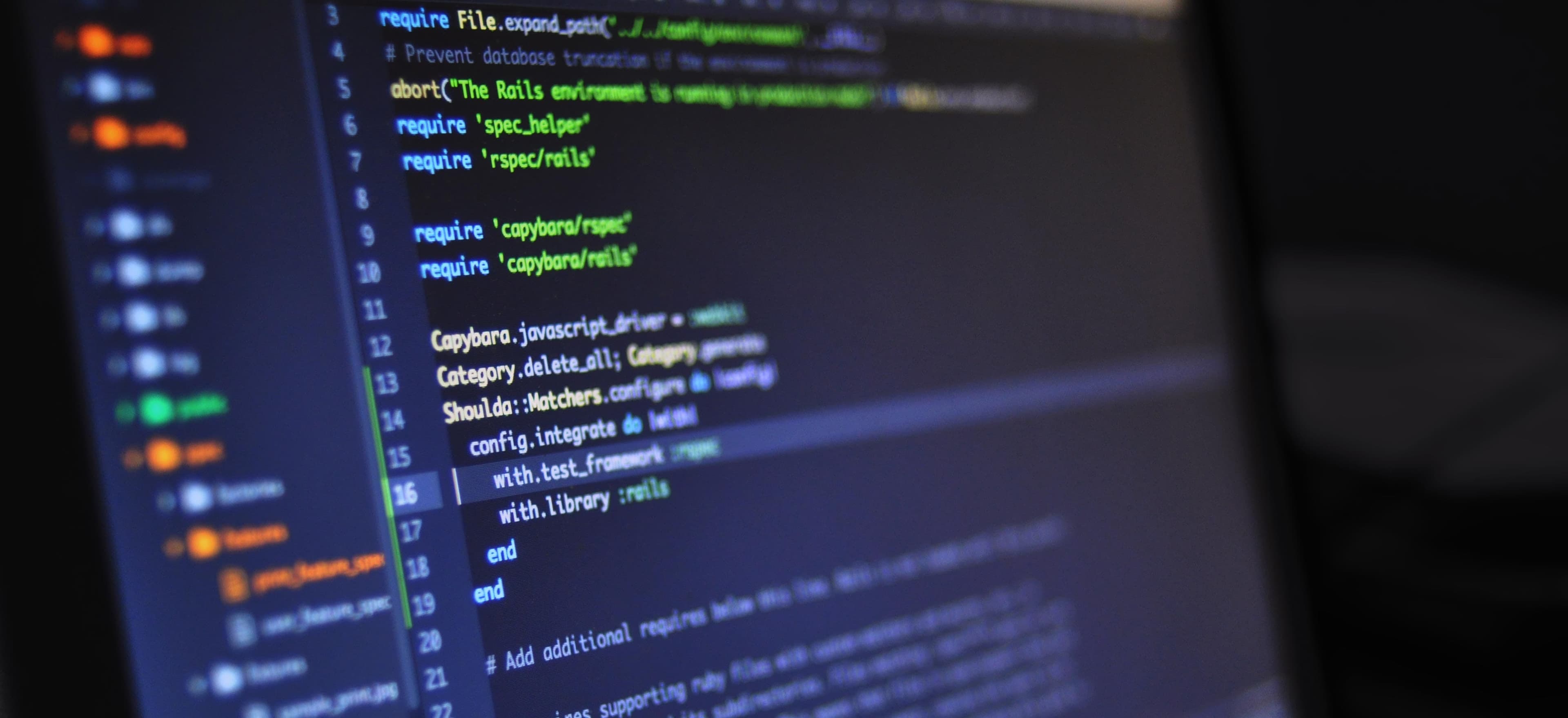
- Published on
Boosting Java Lambda Performance for Low Latency Systems
In today's fast-paced digital world, building applications that deliver optimal performance and low latency is not just a luxury but a necessity. Java, a versatile programming language, has evolved significantly over the years. With the introduction of lambda expressions in Java 8, developers can write cleaner and more efficient code. However, while lambda expressions simplify coding, they can present performance challenges, particularly in low latency systems.
In this blog post, we'll explore strategies to optimize Java lambda performance, ensuring your application runs smoothly even in high-demand scenarios.
What are Lambda Expressions?
Lambda expressions are a feature of Java that enable the creation of anonymous functions. They allow developers to pass behavior as a parameter, making code more concise and easier to read. For example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
In this snippet, the lambda expression name -> System.out.println(name)
replaces the necessity of writing an entire class just to define the operation.
Why Optimize Lambda Performance?
While lambda expressions are efficient, they do introduce certain overheads that can impact performance, especially in low-latency environments. The use of lambda may lead to increased memory allocation and garbage collection pressure as they often capture variables from their surrounding context. This behavior can lead to latency due to frequent garbage collection cycles.
Memory Allocation Concerns
In Java, when you create a lambda expression, the Java Virtual Machine (JVM) may allocate an instance of a functional interface for that lambda. This allocation, while usually not significant in small applications, can become problematic when the application scales and lambda expressions are used extensively in tight loops.
Strategies for Optimizing Performance
1. Avoid Unnecessary Object Creation
One of the simplest yet most effective optimization techniques is to minimize unnecessary object creation. The Java compiler generates a synthetic class for each lambda expression, which means increased memory overhead. By using method references, you can often avoid creating new instances, leading to improved performance.
Example:
Instead of using a lambda expression:
names.forEach(name -> processName(name));
Use a method reference:
names.forEach(this::processName);
Method references tell the JVM to directly call the processName
method without the overhead of creating a lambda object.
2. Cache Results
If your lambda expressions are performing calculations or accessing resources, consider caching the results. This is particularly useful for repetitive calculations.
Example:
Map<String, Integer> cache = new HashMap<>();
names.forEach(name -> {
if (!cache.containsKey(name)) {
cache.put(name, calculateLength(name)); // Expensive operation
}
System.out.println(cache.get(name));
});
In this example, the expensive calculateLength()
operation is only executed once for each unique name, reducing computational overhead.
3. Use Fork/Join Framework
For computations that can run asynchronously, consider using the Fork/Join framework, which is part of the java.util.concurrent
package. This framework allows you to split tasks into smaller, independent subtasks, which can be processed concurrently.
Example:
ForkJoinPool forkJoinPool = new ForkJoinPool();
forkJoinPool.invoke(new RecursiveTask<Integer>() {
@Override
protected Integer compute() {
// Split task or delegate to subtasks here
return total;
}
});
By leveraging parallelism, you can speed up processing, thus reducing latency.
4. Use Primitive Types Instead of Objects
When working with primitive types, try to avoid boxing and unboxing, which can introduce performance overhead. Use streams with explicit primitive data types.
Example:
Instead of:
List<Integer> integers = Arrays.asList(1, 2, 3, 4);
int sum = integers.stream().mapToInt(i -> i).sum();
Try this:
int sum = IntStream.of(1, 2, 3, 4).sum();
Using IntStream
avoids the overhead of boxing and improves performance.
5. Implement HotSpot Optimizations
Make use of the JVM’s HotSpot capabilities. After a certain period, the JVM optimizes the execution of frequently-used code paths. You can help this process by isolating hot code paths and ensuring they are invoked frequently enough.
For example, ensure that your lambda expressions and their calls are located in performance-critical paths.
6. Profile and Monitor Resource Usage
To fully grasp where lambda expressions are causing bottlenecks, use profiling tools like Java Mission Control or VisualVM. Monitoring resource usage will guide your optimization efforts, allowing you to target specific areas for improvement.
In Conclusion, Here is What Matters
Optimizing Java lambda expressions for low-latency systems requires a careful balance between the advantages that lambda expressions offer and the performance ramifications they might carry. By incorporating these strategies, you can enhance your application's responsiveness and overall performance.
Low-latency systems demand consistent performance. Understanding how to manage memory, utilizing caches, using the right data types, and leveraging the capabilities of the JVM can lead to a notable improvement in your application's performance.
Further Reading
- Java Lambda Expressions: A Quick Guide
- Java Performance Tuning
- Understanding Java Streams
By implementing these strategies, you’ll not only improve your Java application's performance but also deliver a better experience for your users. Always remember to test optimizations in your specific environment since the impact can vary based on the context in which your application runs.
Happy coding!