Common Thymeleaf Errors in Spring Applications and How to Fix Them
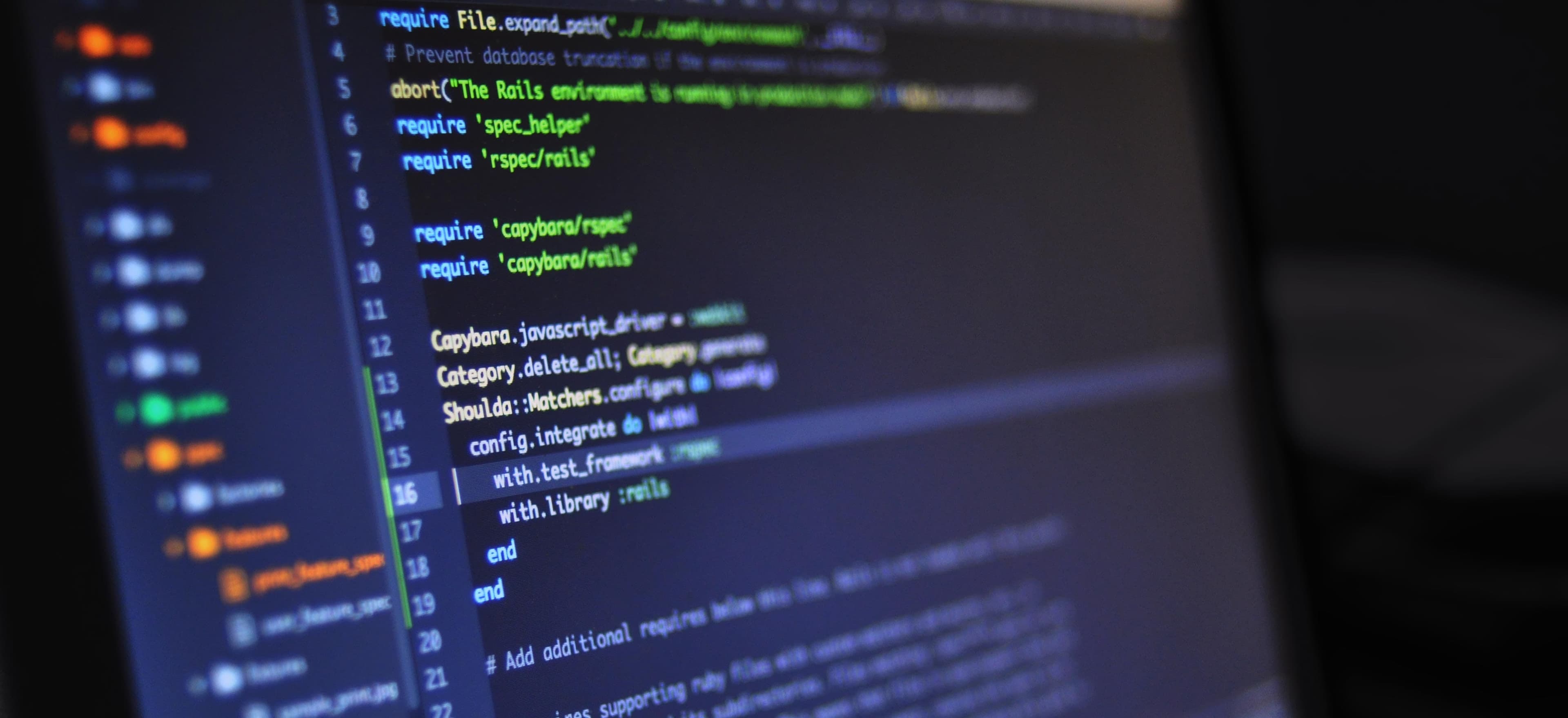
- Published on
Common Thymeleaf Errors in Spring Applications and How to Fix Them
Thymeleaf is a powerful templating engine for Java applications, particularly within Spring MVC. It allows developers to create rich web applications however, debugging Thymeleaf can sometimes be a painful experience. In this blog post, we will explore some common Thymeleaf errors encountered in Spring applications, their causes, and how to fix them effectively.
Understanding Thymeleaf
Before diving into the common errors, it is essential to understand what makes Thymeleaf such a popular and powerful tool. Thymeleaf allows developers to create dynamic web pages using simple HTML templates—making it not only intuitive but also easy to integrate with various frameworks, specifically Spring.
With support for both server-side rendering and client-side features, Thymeleaf can also operate in a Spring Boot environment, enhancing web development capabilities considerably.
Common Thymeleaf Errors
1. Template Not Found
Error Message:
404 error: Template [templateName.html] not found
Cause:
This error usually occurs when Thymeleaf is unable to locate the template you referenced in your controller. The default path where Thymeleaf looks for templates is /src/main/resources/templates
.
Fix: Ensure that your HTML templates are in the correct directory. Check the spelling and casing of the template name. The path in the controller must match exactly.
@RequestMapping("/example")
public String getExamplePage(Model model) {
// Make sure the template name matches the file under src/main/resources/templates
return "exampleTemplate"; // Will look for exampleTemplate.html
}
2. Unable to Resolve Expression
Error Message:
Cannot evaluate expression "${expression}"
Cause: This typically happens when there is an issue with the variable names in your model or if the context does not have a reference to the expected object.
Fix: Check that the variables you are trying to reference in your template are correctly added to your model.
@RequestMapping("/details")
public String getDetails(Model model) {
model.addAttribute("item", itemService.getItem());
return "detailsTemplate";
}
Then in your detailsTemplate.html
, ensure you are using the variable correctly:
<p th:text="${item.name}">Item Name</p>
If item
is not present or null, the expression will not evaluate, resulting in an error.
3. Template Engine Configuration
Error Message:
Could not resolve view with name 'viewName'
Cause: This issue can occur due to improper configuration of the Thymeleaf template engine in your Spring application. The required dependencies may be missing, or your Spring configuration may not be properly set up.
Fix:
Ensure you have the correct dependencies in your pom.xml
or build.gradle
.
Maven Example:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
Gradle Example:
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
Also, confirm the entry point for Thymeleaf is properly defined in your configuration class or application.properties.
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.suffix=.html
This will ensure Thymeleaf looks for templates in the appropriate directory.
4. Thymeleaf Fragment Not Rendered
Error Message:
Fragment 'fragmentName' not found
Cause: This error usually occurs when attempting to include a fragment that does not exist or is not properly defined in the HTML template.
Fix: Ensure the fragment is correctly defined and the syntax is accurate.
Define your fragment:
<!-- In commonFragment.html -->
<div th:fragment="header">Header Content</div>
Include your fragment in another template:
<!-- In anotherTemplate.html -->
<div th:replace="fragments/commonFragment :: header"></div>
Check that both files are in the templates directory and paths are correct.
5. Attribute Not Found
Error Message:
Could not find attribute 'attributeName'.
Cause: When Thymeleaf templates use an attribute that hasn't been set or is incorrectly spelled, this error is thrown.
Fix: Verify both the spelling of the attribute and that it has been added to the model.
@RequestMapping("/user")
public String getUser(Model model) {
// Simulating adding user
User user = userService.findUserById(1);
model.addAttribute("user", user);
return "userTemplate";
}
Also, in the userTemplate.html
:
<p th:text="${user.name}">Name</p>
Be sure name
exactly exists within the user
object.
6. Mismatched Data Types
Error Message:
Expression '${value}' cannot be resolved to a type.
Cause: When Thymeleaf tries to perform operations on data with mismatched types, this error arises.
Fix: Ensure the data types being used in expressions or mathematical operations are compatible. For example, attempting to perform arithmetic on a string would cause an issue.
Example Fix:
<!-- Correctly formatting a number -->
<p th:text="${#numbers.formatDecimal(value, 2, 'POINT')}"></p>
My Closing Thoughts on the Matter
Thymeleaf is a robust tool for rendering dynamic HTML in Java applications, but like any framework, it comes with its own set of challenges. Understanding and fixing common errors can greatly enhance your development experience and elevate the quality of your applications.
For further reading, check out the official Thymeleaf documentation and the Spring Framework documentation for comprehensive guides on using Thymeleaf within Spring applications.
By honing your skills to effectively debug errors, you'll be able to leverage Thymeleaf's full potential and create seamless web experiences for your users. Happy coding!