How TDD Impacts Software Quality and Development Costs
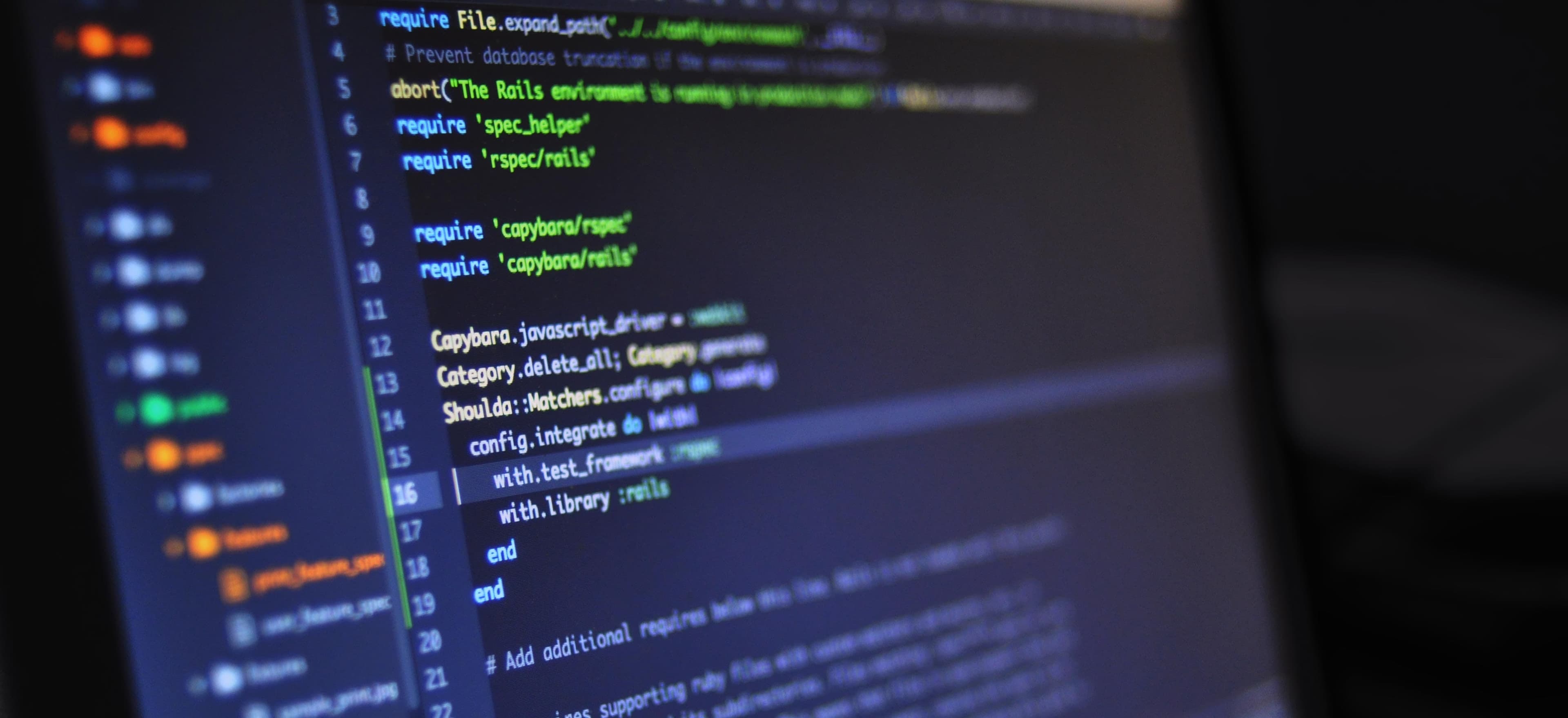
- Published on
How TDD Impacts Software Quality and Development Costs
Test-Driven Development (TDD) is a software development practice that emphasizes writing tests before writing the corresponding code. This methodology has gained traction in the industry due to its remarkable influence on both software quality and overall development costs. In this blog post, we will explore the essence of TDD, its impact on software quality, and how it can help manage and reduce development costs.
What is Test-Driven Development (TDD)?
Test-Driven Development is a software development practice that involves three main steps, often summarized as the "Red-Green-Refactor" cycle:
- Red: Write a failing test for a new feature.
- Green: Write the minimum amount of code necessary to make the test pass.
- Refactor: Optimize and improve the code while ensuring all tests still pass.
This cycle continues as developers continually add more features and functionality to their codebase.
Code Sample: A Simple TDD Example in Java
Let's dive into a very simple example. We will write a function that checks if a number is even.
Step 1: Write a Failing Test
Before we implement any code, let's define our test.
import org.junit.Assert;
import org.junit.Test;
public class NumberUtilsTest {
@Test
public void testIsEven() {
Assert.assertTrue(NumberUtils.isEven(2));
Assert.assertFalse(NumberUtils.isEven(3));
}
}
In this code snippet, we anticipate a method called isEven
that returns true if a number is even and false otherwise. Notice how our test is defined well before any actual functionality.
Step 2: Write Code to Make the Test Pass
Now, we create the isEven
method to satisfy the test.
public class NumberUtils {
public static boolean isEven(int number) {
return number % 2 == 0;
}
}
With this implementation, our test will pass, enabling the next stage: refactoring.
Step 3: Refactoring
In this example, our implementation is already quite efficient. However, during more complex scenarios, refactoring is essential. Suppose we had some redundant code we want to clean up or optimize.
// Example of refactoring, if necessary, would happen here
The fun of TDD lies in its cyclical nature. By consistently looping through these steps, you not only solidify your code with tests but also foster an environment of thoughtful coding.
The Impact of TDD on Software Quality
1. Increased Code Coverage
TDD promotes higher code coverage as developers write tests for nearly every new feature from the outset. High code coverage leads to a lower chance of bugs slipping through into production, enhancing software quality.
2. Improved Test Quality
When tests are written first, developers are encouraged to think critically about edge cases and potential failures. This results in high-quality tests that meticulously verify the implementation.
3. Documentation of Code
Tests serve as a form of documentation. They illustrate how the code is intended to function, which can be extremely valuable for new team members or when revisiting old code.
4. Reduced Regression Bugs
As new features are developed, TDD ensures existing functionality is covered by tests. This acts as a safety net, which is vital for avoiding regression bugs.
5. Encourages Simplicity and Clarity
In striving to ensure that tests pass with the minimal code, developers are further encouraged to avoid over-engineering. This usually leads to simpler, more focused codebases.
TDD and Development Costs
1. Fewer Defects Mean Lower Costs
One of the most significant benefits of TDD is its potential to reduce the number of bugs in production. The cost of fixing defects increases exponentially the later they are found in the development cycle. By identifying defects early through unit tests, developers save money and time in the long run.
2. Reduced Debugging Time
As the number of defects reduces, developers spend less time debugging and maintaining code. Time saved can be allocated to new feature development or improving existing functionality.
3. Enhanced Collaboration and Efficiency
Teams who adopt TDD often find that it fosters collaboration. As tests provide clear expectations, team members can work on different parts of a project without extensive documentation or micromanagement.
4. Higher Developer Satisfaction
When developers can deliver high-quality, bug-free software, their job satisfaction usually improves. Happier developers tend to be more productive, which further decreases costs associated with development.
Challenges of Implementing TDD
While TDD offers myriad benefits, implementing it isn't always straightforward:
- Learning Curve: Developers may find the TDD mindset challenging to adopt initially.
- Time Investment: The upfront time required for writing tests can seem excessive, especially for teams inexperienced with TDD.
- Requires Discipline: TDD demands a disciplined approach to development, which may strain teams in fast-paced environments.
The Closing Argument
Test-Driven Development is a powerful methodology that significantly impacts software quality and development costs. By promoting high-quality tests and documentation, reducing the likelihood of defects, and fostering collaboration among developers, TDD allows teams to deliver software that meets both user needs and business goals. While it might present initial challenges, the long-term benefits of TDD often outweigh the short-term investments.
For those looking to delve deeper into TDD, consider exploring the following resources:
Embracing TDD can indeed transform your development process, leading to more robust software and streamlined costs over time.