Common Pitfalls in Spring 3 RESTful Web Services
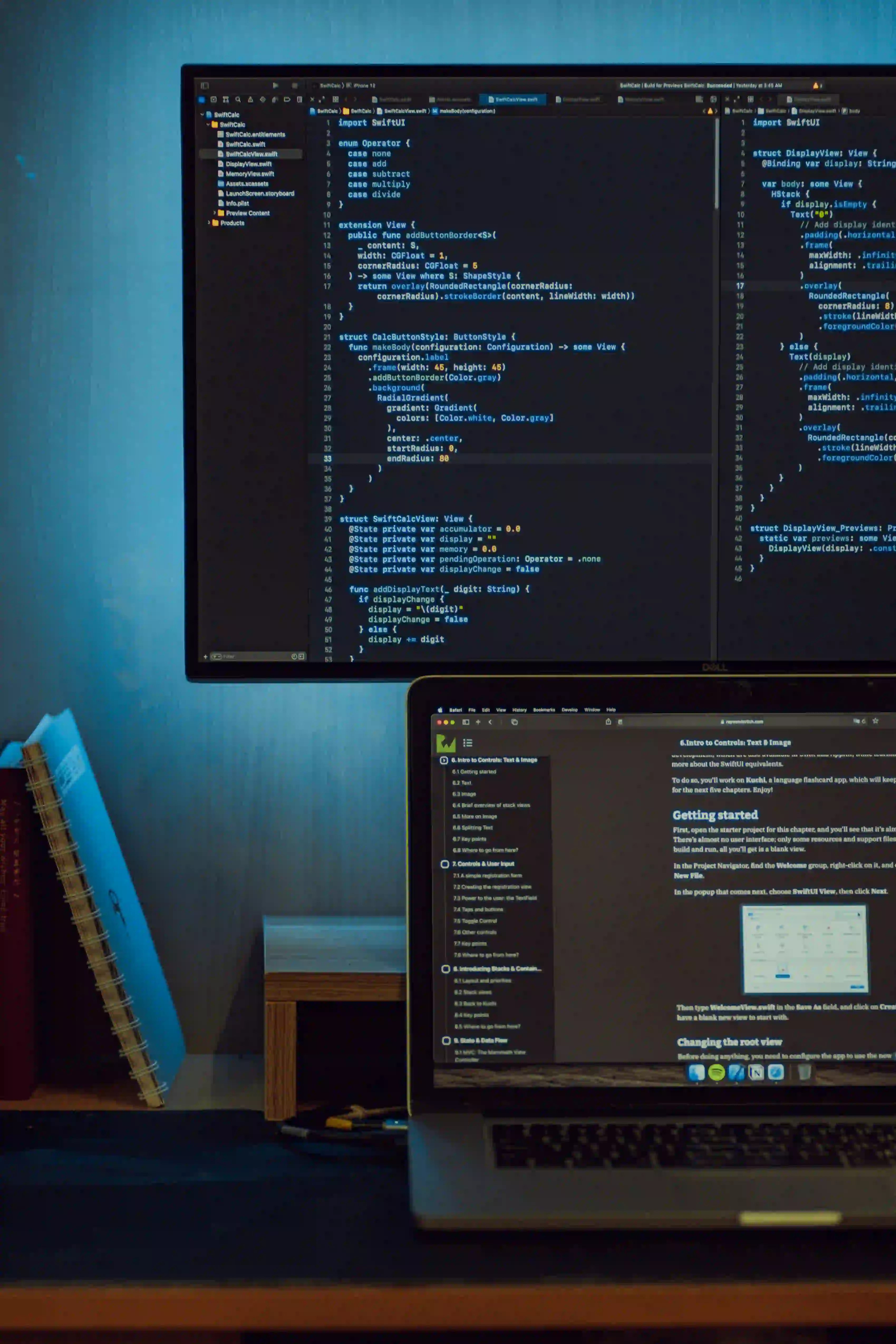
Common Pitfalls in Spring 3 RESTful Web Services
Building RESTful web services using Spring 3, although rewarding, is fraught with common pitfalls that can hinder the performance, maintainability, and usability of your applications. In this post, we will explore these pitfalls, along with best practices to avoid them, ensuring a smooth development experience.
Understanding REST
Before diving into the pitfalls, it’s essential to have a solid understanding of REST (Representational State Transfer). RESTful services provide interoperability between computer systems on the internet. They communicate via defined standards such as HTTP, and utilize standard HTTP methods (GET, POST, PUT, DELETE) to perform operations.
Pitfall 1: Ignoring HTTP Status Codes
One of the core principles of a RESTful service is proper utilization of HTTP status codes. By ignoring these status codes, we fail to communicate the result of an operation effectively.
Why It Matters
HTTP status codes inform the client of the outcome of their request. For example, a 404 indicates that the resource was not found, while a 201 suggests that a new resource has been created successfully.
Best Practice
Always return relevant HTTP status codes based on the response. Here’s an example of how you can implement that in Spring 3:
@RestController
public class UserController {
@RequestMapping(value = "/users/{id}", method = RequestMethod.GET)
public ResponseEntity<User> getUser(@PathVariable("id") Long id) {
User user = userService.findUserById(id);
if (user == null) {
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
return new ResponseEntity<>(user, HttpStatus.OK);
}
}
In this snippet, we return appropriate status codes based on whether the user exists.
Pitfall 2: Overly Complex URL Structures
Complex URLs can confuse clients and make it difficult for developers to understand the API.
Why It Matters
Simplicity in URLs enhances usability and readability. RESTful services should leverage nouns to represent resources and keep the endpoint structure intuitive.
Best Practice
Design your API endpoints consistently. Here’s an example:
@RequestMapping(value = "/api/users", method = RequestMethod.GET)
public List<User> listUsers() {
return userService.findAllUsers();
}
In this case, /api/users
is a straightforward and easy-to-understand endpoint that lists all users.
Pitfall 3: Lack of Versioning
Not including a version number in your API can lead to problems as your application evolves.
Why It Matters
Versioning ensures that clients can still use an older version of your API even after you introduce breaking changes. This will facilitate a smooth transition for your users.
Best Practice
Incorporate versioning in your API URLs. The following example demonstrates this:
@RequestMapping(value = "/api/v1/users", method = RequestMethod.GET)
public List<User> getUsersV1() {
return userService.findAllUsers();
}
Here, the API version is clearly indicated in the URL, allowing for future expansion without disrupting existing clients.
Pitfall 4: Failing to Handle Exceptions Properly
Unhandled exceptions can result in server errors that return little information to the client.
Why It Matters
Proper exception handling improves the robustness of your service and facilitates debugging for clients.
Best Practice
Utilize Spring's @ControllerAdvice
to handle exceptions globally. Here’s an example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<Object> handleUserNotFound(UserNotFoundException ex) {
return new ResponseEntity<>("User not found", HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleGlobalException(Exception ex) {
return new ResponseEntity<>("An error occurred", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this case, specific exceptions trigger meaningful responses, improving the client's experience.
Pitfall 5: Inefficient Data Serialization
Inefficient JSON serialization can impact the performance of your web service, leading to slower response times.
Why It Matters
Serialization transforms your Java objects into JSON. However, inefficient object-to-JSON conversions increase the response time.
Best Practice
Utilize Jackson, which comes bundled with Spring, for efficient serialization. Configure your data models appropriately, for example:
@JsonIgnoreProperties({ "hibernateLazyInitializer", "handler" })
public class User {
private Long id;
private String name;
// Getters and Setters
}
Using @JsonIgnoreProperties
alleviates unnecessary data being sent, improving performance.
Pitfall 6: Not Utilizing HATEOAS
HATEOAS (Hypermedia as the Engine of Application State) is a key principle of REST that allows clients to navigate the API dynamically.
Why It Matters
Not employing HATEOAS can make your API less discoverable and harder to navigate for developers.
Best Practice
Include hyperlinks in your responses to guide the client. For example:
public class UserResource {
private User user;
private Link selfLink;
public UserResource(User user) {
this.user = user;
this.selfLink = new Link("/api/users/" + user.getId(), "self");
}
public User getUser() {
return user;
}
public Link getSelfLink() {
return selfLink;
}
}
By including links within your resources, you provide a richer API experience.
Pitfall 7: Insufficient Documentation
Poorly documented APIs can frustrate developers, making them less likely to engage with your service.
Why It Matters
Documentation is vital for adoption. A well-documented API provides clarity and fosters use among developers.
Best Practice
Use tools like Swagger (now known as OpenAPI Specification) to generate comprehensive documentation automatically. By annotating your controllers:
@Api(value = "User Management", description = "Operations pertaining to user management")
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
@ApiOperation(value = "Get all users")
@RequestMapping(method = RequestMethod.GET)
public List<User> listUsers() {
return userService.findAllUsers();
}
}
This annotation approach enhances documentation and enables easier interaction with tools like Swagger UI.
To Wrap Things Up
In conclusion, building RESTful services in Spring 3 presents some unique challenges. By being aware of these common pitfalls and implementing the best practices described, you can significantly enhance the quality and usability of your web services. Proper HTTP status codes, simple URL structures, effective exception handling, efficient serialization, HATEOAS support, and comprehensive documentation are essential for creating a robust RESTful API.
For further reading on REST best practices, you can explore resources such as RESTful Web Services or Spring Framework Documentation.
With these guidelines in place, start developing your Spring RESTful web services with confidence, and avoid the common pitfalls that often trap developers. Happy coding!