Common Pitfalls in Running JUnit Tests for Android Projects
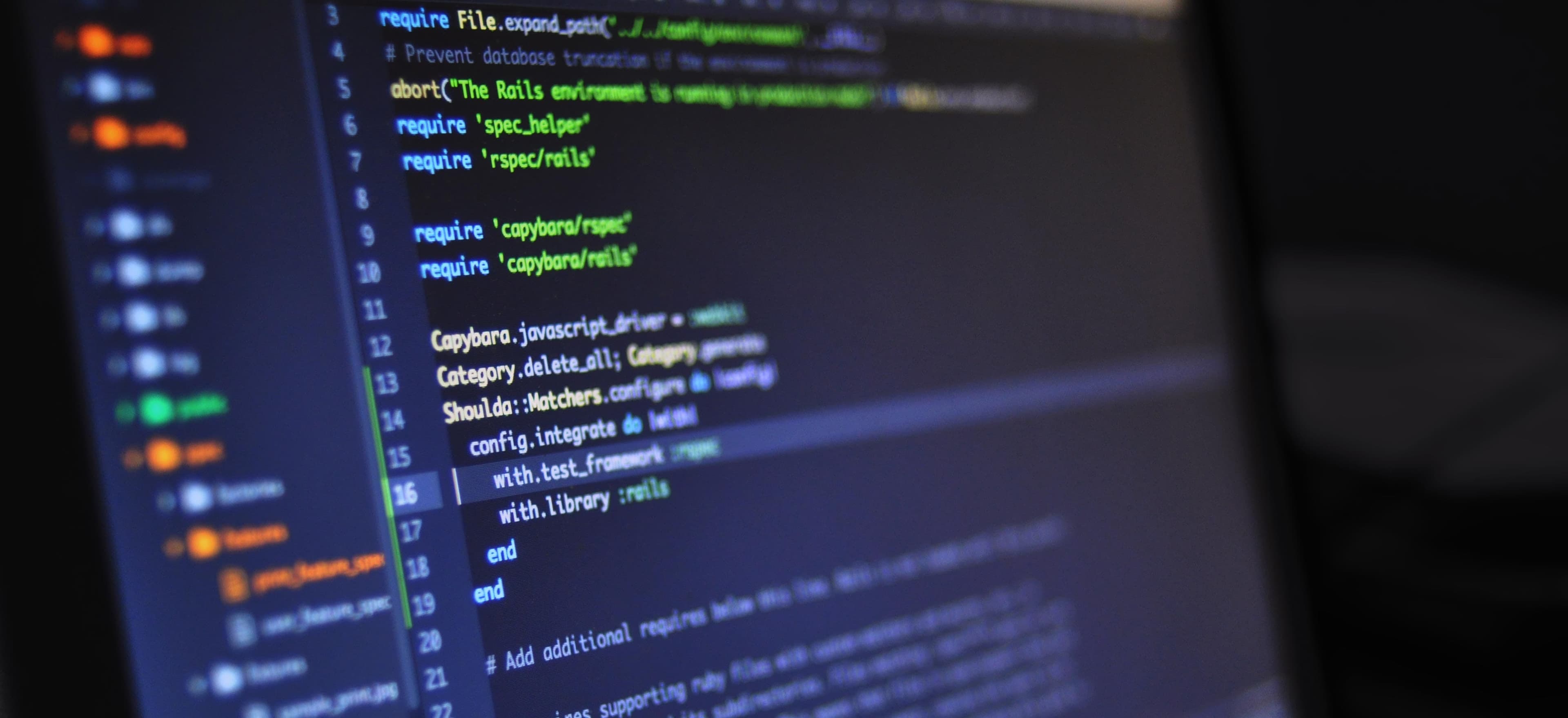
- Published on
Common Pitfalls in Running JUnit Tests for Android Projects
When developing Android applications, employing testing frameworks is essential to ensure that your code behaves as expected. JUnit is a popular framework for unit testing in Java, including Android projects. However, while using JUnit, developers often encounter common pitfalls that can hinder their testing efficiency. In this blog post, we'll explore these pitfalls, how to avoid them, and provide relevant code snippets that emphasize best practices.
Understanding JUnit in Android
JUnit provides a straightforward framework to create and run tests in Java. In Android, JUnit tests are commonly used for unit testing, while instrumented tests (which run on an Android device) use the AndroidJUnitRunner. This dual testing approach allows you to test both your business logic and UI components effectively.
Setting Up Your Android Project for JUnit Testing
Before diving into common pitfalls, it’s essential to understand how to set up JUnit in your Android project. Below is a simple setup for adding JUnit to your Android project:
-
Add Dependencies: Open your
build.gradle
file for your module and ensure you have the following dependencies:dependencies { testImplementation 'junit:junit:4.13.2' androidTestImplementation 'androidx.test.ext:junit:1.1.3' androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0' }
-
Create a Test Class: Create a new test class under the
src/test/java
directory.import org.junit.Test; import static org.junit.Assert.*; public class ExampleUnitTest { @Test public void addition_isCorrect() { assertEquals(4, 2 + 2); } }
This example demonstrates a simple addition test. From this point, let's explore the common pitfalls.
Common Pitfalls and Solutions
1. Not Isolating Unit Tests from Android Frameworks
The Pitfall
One common mistake is assuming that unit tests can directly interact with Android components such as Activity
or Context
. This can lead to flaky tests since many Android components require an actual device or emulator to run.
The Solution
Ensure that your unit tests are true unit tests and do not depend on the Android framework. Use mocking frameworks like Mockito to substitute Android dependencies. Here’s an example:
import static org.mockito.Mockito.*;
public class UserRepositoryTest {
@Test
public void testGetUser() {
// Create a mock UserService
UserService mockedService = mock(UserService.class);
// Stub the service method
when(mockedService.getUser(1)).thenReturn(new User(1, "John"));
// Create the repository and inject the mock
UserRepository userRepository = new UserRepository(mockedService);
// Call the method under test
User user = userRepository.getUser(1);
// Verify the result
assertEquals("John", user.getName());
}
}
In this example, the UserService
is mocked, isolating the test from the Android framework.
2. Ignoring Test Coverage
The Pitfall
As features get added to applications, developers often overlook the importance of maintaining adequate test coverage. As a result, critical code paths may remain untested, leading to more bugs in production.
The Solution
Leverage coverage tools like Jacoco to ensure comprehensive test coverage. Add the following to your build.gradle
file:
apply plugin: 'jacoco'
jacoco {
toolVersion = "0.8.7"
}
android {
buildTypes {
release {
// Enable code coverage data collection
testCoverageEnabled = true
}
}
}
Running your tests will generate a coverage report, allowing you to identify and add tests to under-covered areas of your code.
3. Lack of Proper Exception Handling
The Pitfall
Assuming that all tests will pass without properly handling exceptions can lead to misleading results. This practice can also obscure what went wrong in the event of a test failure.
The Solution
Assert exceptions to ensure that the code behaves correctly when unexpected inputs are encountered. For example:
@Test(expected = IllegalArgumentException.class)
public void testNullInputThrowsException() {
// This should throw an IllegalArgumentException
myService.processInput(null);
}
Using @Test(expected = Exception.class)
guarantees that the test will pass only if the specified exception is thrown.
4. Not Using AndroidX Test Libraries
The Pitfall
With the introduction of AndroidX libraries, some developers still use legacy Android testing libraries. This can cause compatibility issues and limit functionality.
The Solution
Always use the latest AndroidX testing libraries for unit and instrumented testing. Here's an example of an instrumented test:
import androidx.test.ext.junit.runners.AndroidJUnit4;
import androidx.test.platform.app.InstrumentationRegistry;
import org.junit.Test;
import org.junit.runner.RunWith;
import static org.junit.Assert.assertEquals;
@RunWith(AndroidJUnit4.class)
public class ExampleInstrumentedTest {
@Test
public void useAppContext() {
// Context of the app under test
Context appContext = InstrumentationRegistry.getInstrumentation().getTargetContext();
assertEquals("com.example.myapp", appContext.getPackageName());
}
}
Utilizing AndroidX ensures that your tests are up-to-date and work seamlessly with the latest Android features.
5. Not Running Tests in CI/CD Environments
The Pitfall
Another significant oversight is not incorporating tests into your CI/CD (Continuous Integration/Continuous Deployment) pipeline. This lapse can result in shipping untested code to production.
The Solution
Integrate your tests into CI/CD workflows. For instance, if you are using GitHub Actions, your workflow configuration could look like this:
name: Android CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build and run tests
run: ./gradlew build test
This configuration ensures that tests run automatically whenever code is pushed or a pull request is submitted, catching issues early.
In Conclusion, Here is What Matters
Testing is a critical part of Android app development, and JUnit offers a robust framework for writing unit tests. However, being aware of common pitfalls can significantly enhance both the quality of your tests and the reliability of your application.
Remember to isolate unit tests from Android dependencies, maintain adequate test coverage, properly handle exceptions, utilize AndroidX libraries, and integrate tests into your CI/CD pipeline.
By implementing these best practices, you’ll create a more resilient application that delivers a better experience for users.
For more information about JUnit, visit the JUnit Official Website. Happy testing!
Checkout our other articles