Why You Shouldn't Defer Object Validation in Your Code
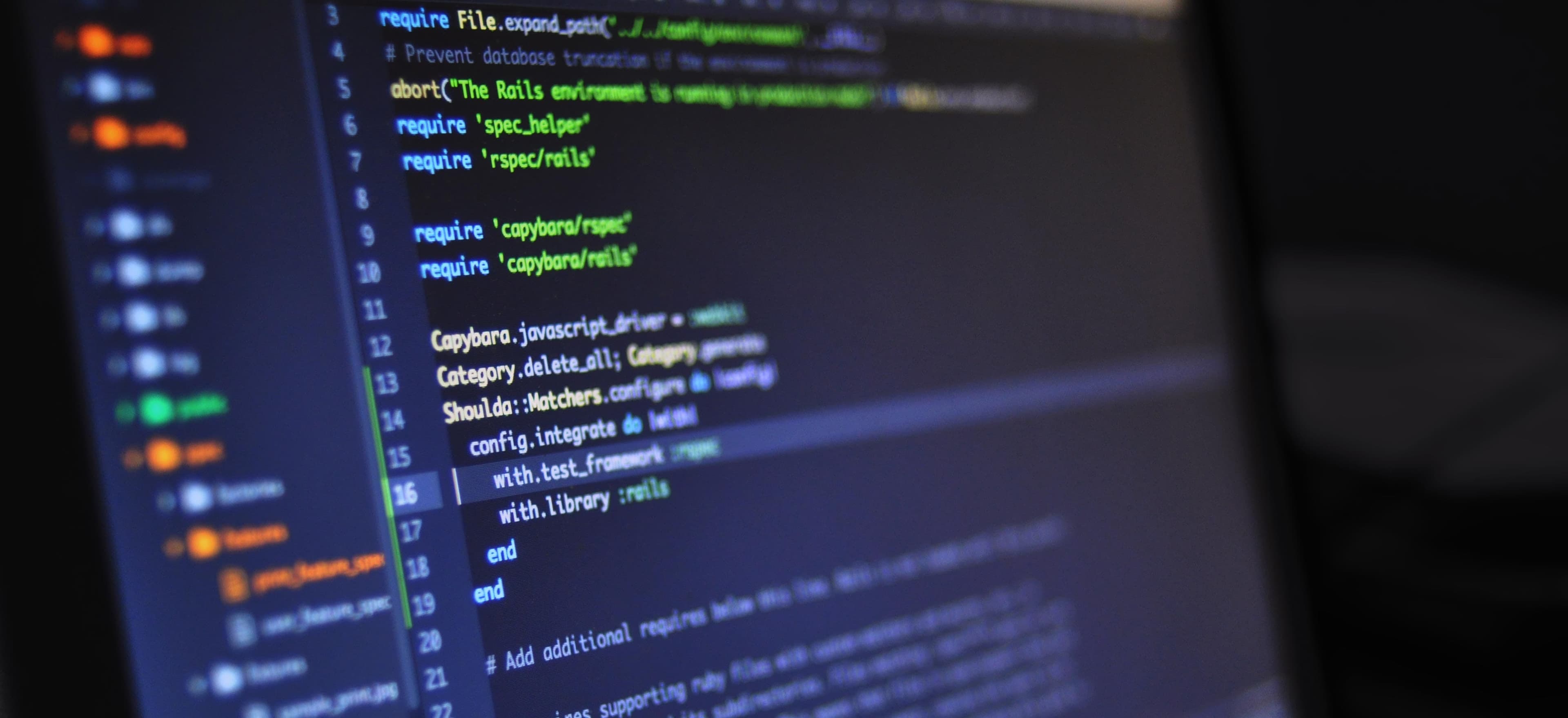
- Published on
Why You Shouldn't Defer Object Validation in Your Code
In the world of software development, particularly when working with Java, the importance of validation cannot be overstated. Validation ensures that the data your application processes meets certain standards and formats. In this blog post, we will explore the concept of object validation, why it should be done early and consistently, and how to implement it effectively in your Java applications.
Understanding Object Validation
Object validation is a process that checks if an object meets specific criteria before being processed. This typically includes verifying that the object's data is not only correct but also reliable. Problems arise when developers defer validation, leading to issues down the line that can be hard to trace and rectify.
The Dangers of Deferred Validation
Deferring validation can lead to:
-
Increased Debugging Time: If validation is performed later in the code, you may end up with complex error messages that require more effort to debug.
-
Security Vulnerabilities: Unvalidated data, especially from user inputs, can expose your application to security risks such as SQL injection or cross-site scripting.
-
Poor Performance: Validating at the last possible moment might seem like a good idea for performance, but in reality, catching such issues early saves heaps of time in performance tuning later.
-
Compounded Errors: Validating at different points can lead to inconsistent application behavior and multiple sources for the same validation logic.
Real-World Example of Deferred Validation
To better illustrate the issues with deferred validation, consider the following Java class designed to process user data.
public class User {
private String username;
private String email;
public User(String username, String email) {
this.username = username;
this.email = email;
}
// Getters and Setters
}
In this instance, there are no validation checks applied to the username or email. The programmer intends to validate these fields later. However, passing an invalid email address or an empty username could lead to issues when user data is processed.
Immediate Validation: A Better Approach
Instead of deferring validation, let's implement it directly in the constructor or through setter methods. Early validation gives you the chance to handle user errors right away—preventing further complications.
Consider how we can modify the User
class to include validation:
public class User {
private String username;
private String email;
public User(String username, String email) {
setUsername(username);
setEmail(email);
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
if (username == null || username.trim().isEmpty()) {
throw new IllegalArgumentException("Username cannot be null or empty.");
}
this.username = username;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
if (email == null || !email.matches("^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$")) {
throw new IllegalArgumentException("Email is not valid.");
}
this.email = email;
}
}
Why Immediate Validation Works
-
Clear Fault Reporting: By throwing exceptions in the setter methods, any issues with the input are clearly communicated, aiding in debugging.
-
Consistent State: The object can never be in an invalid state—ensuring that your application logic works on robust inputs.
-
Improved Security: Valid inputs lessen the chances of vulnerabilities and make your application more resilient against attacks.
Best Practices for Object Validation
1. Centralized Validation Logic
To avoid duplication, centralize your validation rules wherever possible. This can be achieved by using utility classes or frameworks like Hibernate Validator.
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Email;
public class User {
@NotNull
private String username;
@Email
private String email;
// Other members and methods
}
In this example, annotations provide a cleaner way to enforce validation without mixing it up within the business logic.
2. Use of Design Patterns
Applying design patterns such as the Decorator Pattern can help extend and enhance validation rules without modifying existing code.
3. Testing Your Validations
Automated tests are crucial. A set of unit tests covering various scenarios (valid and invalid inputs) ensures that the validations work as intended and remain robust against future changes.
import static org.junit.Assert.*;
import org.junit.Test;
public class UserTest {
@Test(expected = IllegalArgumentException.class)
public void testInvalidUsername() {
User user = new User("", "test@example.com");
}
@Test(expected = IllegalArgumentException.class)
public void testInvalidEmail() {
User user = new User("testUser", "invalid-email");
}
}
The Bottom Line
Deferring object validation can lead to a myriad of issues, from security risks to increased debugging complexity. By validating your objects early, you can ensure data integrity and application reliability, making your code cleaner and more maintainable.
Incorporating sound validation strategies not only aids in software quality but also enhances user experience by promptly addressing invalid inputs. Adopting a mindset of early validation will serve you well throughout your development career and will pay dividends in terms of reduced bug counts and cleaner, more secure code.
Additional Resources
- Java Bean Validation Specification (JSR 380)
- Effective Java by Joshua Bloch
- Introduction to Design Patterns in Java
Feel free to explore these resources for more in-depth understanding and practices of object validation in Java. Remember, a little extra effort up front can save a lot of time and trouble down the line!
Checkout our other articles