Solving Dependency Hell in OSGi with Maven Equinox
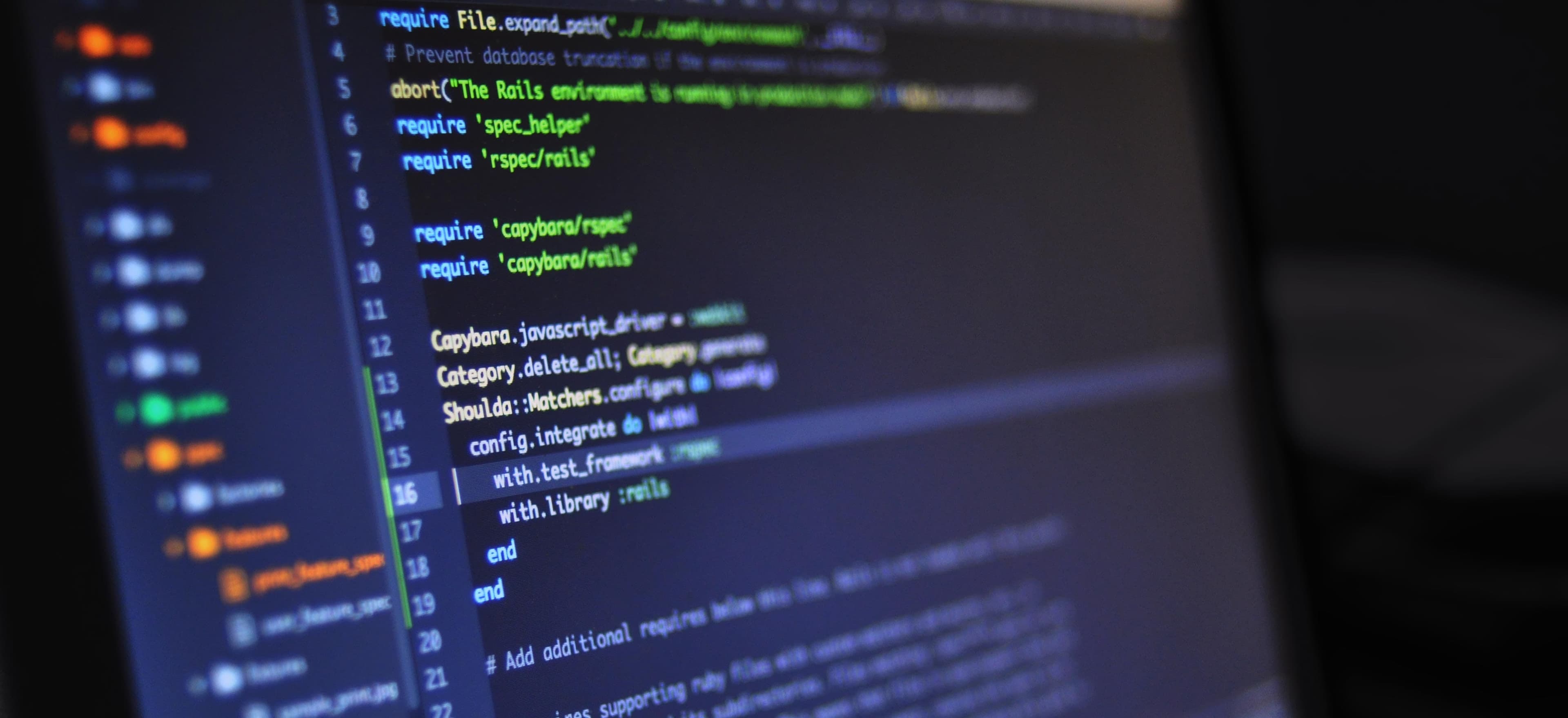
- Published on
Solving Dependency Hell in OSGi with Maven Equinox
Dependency management in software development can often resemble an intricate dance, particularly when leveraging frameworks like OSGi (Open Service Gateway initiative). OSGi provides a robust platform for modular development in Java, but it also invites its own set of challenges — most notably, what's commonly referred to as "Dependency Hell". In this blog post, we will delve into the nuances of dependency management in OSGi and how Maven Equinox provides a resolution.
Understanding OSGi and Dependency Hell
What is OSGi?
OSGi is a set of specifications that enable the development of modular applications. Its primary advantage lies in its ability to dynamically load and unload components, known as bundles.
However, OSGi's dynamic modularity can lead to complex dependency issues, especially when:
- Different bundles require different versions of the same library.
- Circular dependencies are present.
- Bundles fail to resolve dependencies during runtime.
These scenarios contribute to the frictions we refer to as Dependency Hell.
What is Maven Equinox?
Maven Equinox is an OSGi implementation that seamlessly integrates with Maven, a popular build automation tool in the Java ecosystem. It allows for simplified management of dependencies while still adhering to OSGi's principles. Utilizing Maven with Equinox serves as a foundation for addressing the complications associated with dependency management.
Why Use Maven for OSGi?
Maven brings a plethora of benefits that helps clarity and reduces complexity:
- Convention over Configuration: Maven enforces a standard project layout, which is beneficial in maintaining modular OSGi projects.
- Dependency Management: Through a centralized management system, dependencies can be specified easily in the
pom.xml
file, which Maven handles during the build phase. - Flexibility: Maven plugins, such as the maven-bundle-plugin, create OSGi bundles from standard Maven projects effortlessly.
Introducing the maven-bundle-plugin
The maven-bundle-plugin is essential for working with OSGi specifications within Maven. It converts your Java project's JAR files into OSGi bundles, making dependency resolution much more manageable. Below is an example of how to configure the plugin.
<plugin>
<groupId>org.apache.felix</groupId>
<artifactId>maven-bundle-plugin</artifactId>
<version>4.2.1</version>
<extensions>true</extensions>
<configuration>
<instructions>
<Bundle-SymbolicName>${project.artifactId}</Bundle-SymbolicName>
<Bundle-Version>${project.version}</Bundle-Version>
<Import-Package>
org.osgi.framework,
*;version="[1.0,2.0)" // Import dependencies
</Import-Package>
</instructions>
</configuration>
</plugin>
Commentary on the Configuration
- Bundle-SymbolicName: A unique identifier for the bundle. It is crucial for managing the bundle's lifecycle.
- Bundle-Version: Necessary to define the version of your bundle; OSGi relies heavily on versioning.
- Import-Package: Specifies the packages that your bundle will use from other bundles, and the version range indicates acceptable package versions.
Understanding these components is foundational as they guide the behavior of your OSGi bundle and its dependencies.
Typical Scenario of Dependency Hell
Let’s consider a practical example to illustrate the concept.
Scenario
You have two bundles:
- Bundle A requires version 1.0 of library X.
- Bundle B requires version 2.0 of the same library X.
Unsure of how to resolve this conflict? This is where Dependency Hell manifests. The OSGi runtime can only load one version of the library at a time, leading to potential failures if one bundle encounters functionality incompatible with the other version.
Resolving the Conflict with Maven Equinox
- Define the dependencies in the
pom.xml
:
<dependencies>
<dependency>
<groupId>com.example.library</groupId>
<artifactId>library-x</artifactId>
<version>1.0</version>
</dependency>
<dependency>
<groupId>com.example.library</groupId>
<artifactId>library-x</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
-
Utilize the
resolution strategy
provided by OSGi.You can instruct Maven to allow multiple versions by manipulating the import settings in the
pom.xml
. By using ranges or importing the all versions, you can resolve dependency conflicts.
<Import-Package>
com.example.library;version="[1.0,2.0]", *
</Import-Package>
Explanation on the Import Strategy
Defining version ranges allows your bundle to accommodate both versions of the library. By specifying "[1.0,2.0]"
, we inform OSGi that 1.0
to 2.0
inclusively is acceptable, effectively resolving the Dependency Hell conflict.
This method of version resolution ensures that your application remains modular and flexible while reducing the chances of runtime failures.
Best Practices for Managing OSGi Dependencies
To further alleviate Dependency Hell, consider implementing these best practices:
-
Use Versioning Wisely: Always specify exact versions for your dependencies. Use version ranges cautiously and only when necessary.
-
Keep Bundles Small and Focused: This minimizes potential conflicts and simplifies dependencies.
-
Document Dependencies: Ensure that all developers understand and can easily find the dependencies required within the project.
-
Leverage OSGi Services: Instead of tightly coupling bundles, make use of OSGi services to decouple modules.
-
Automate with CI Systems: Incorporating Continuous Integration (CI) allows seamless detection of breaks due to dependency changes.
A Final Look
By integrating Maven Equinox into the OSGi development process, you can effectively tackle Dependency Hell and streamline the building of modular applications. The use of the maven-bundle-plugin combined with thoughtful dependency management aids in resolving various issues related to conflicting library versions.
For more in-depth reading, check out these resources:
By adopting OSGi principles alongside powerful tools like Maven Equinox, you can create a resilient, maintainable architecture that not only minimizes dependency conflicts but sets your projects on the path to long-term success.