5 Key Principles for Writing Maintainable Code
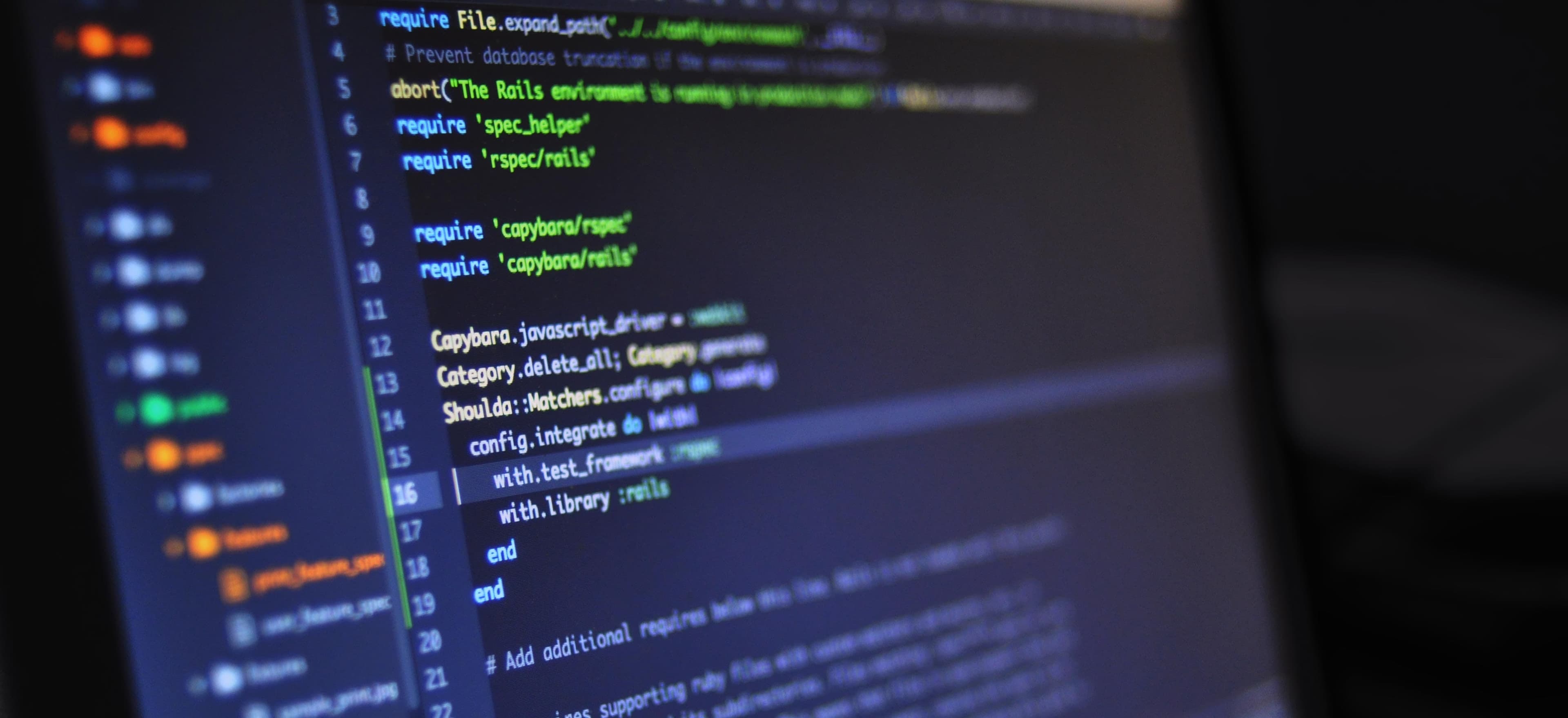
- Published on
5 Key Principles for Writing Maintainable Code
When working with Java, as with any programming language, writing maintainable code is essential for the longevity and functionality of projects. This blog post will explore five key principles for writing maintainable code. These principles will not only enhance the readability of your code but also improve its organization and flexibility for future modifications.
1. Follow Naming Conventions
Clarity starts with naming. Following standard naming conventions makes your code easier to understand. Proper naming helps developers grasp the purpose of variables, methods, and classes at a glance.
Examples of Good Naming Conventions
- Class names should be nouns, formatted in UpperCamelCase. For instance,
CustomerManager
. - Method names should be verbs, formatted in lowerCamelCase, such as
calculateTotalPrice()
. - Variable names should be descriptive and in lowerCamelCase like
totalPrice
.
Code Snippet
public class CustomerManager {
private double totalPrice;
public double calculateTotalPrice(List<Item> items) {
// logic to calculate total price
}
}
Why it Matters
A well-named class or method provides context. It minimizes the cognitive load on a developer, allowing for faster onboarding and easier code reviews.
2. Keep It DRY
DRY stands for "Don't Repeat Yourself." Repetition in code can lead to maintenance headaches. The objective is to ensure that every piece of knowledge or logic exists in a single place.
Application of DRY:
Instead of duplicating the logic of calculating prices in multiple methods, create a single method that can be reused.
Code Snippet
public class Item {
private String name;
private double price;
public double getPrice() {
return price;
}
}
public class CustomerManager {
private List<Item> items;
public double calculateTotalPrice() {
return items.stream()
.mapToDouble(Item::getPrice)
.sum();
}
}
Why it Matters
By adhering to the DRY principle, changes are easier to implement. If the logic needs to change, you only need to update it in one location. This ultimately saves time and reduces bugs.
3. Use Comments Wisely
Comments can clarify complex sections of code, but relying on comments to explain poorly written code is a mistake. It’s important to strike a balance. Use comments to explain why something is done, not what is done—this should be clear from the code itself.
Example of Effective Comments
public class DiscountManager {
/**
* This method applies a discount to the total price.
* Discount is applied based on membership level.
*/
public double applyDiscount(double totalPrice, MembershipLevel level) {
if (level == MembershipLevel.GOLD) {
return totalPrice * 0.9; // 10% off for GOLD members
}
return totalPrice; // No discount
}
}
Why it Matters
Well-placed comments enhance understanding. They can elucidate complex algorithms, document decisions, and inform future maintainers of the code's intent. However, be cautious to avoid over-commenting, as excessive comments can clutter the code.
4. Favor Composition over Inheritance
Inheritance can be a powerful tool but can also lead to tightly coupled code. It's essential to favor composition whenever possible. Using composition allows for more flexible, modular code that is easier to maintain and extend.
Code Snippet
public class PaymentProcessor {
private PaymentStrategy paymentStrategy;
public PaymentProcessor(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void processPayment(double amount) {
paymentStrategy.pay(amount);
}
}
interface PaymentStrategy {
void pay(double amount);
}
class CreditCardPayment implements PaymentStrategy {
public void pay(double amount) {
// Logic for credit card payment
}
}
class PayPalPayment implements PaymentStrategy {
public void pay(double amount) {
// Logic for PayPal payment
}
}
Why it Matters
This approach allows for easy switching or adding of strategies. By keeping classes focused on single responsibilities, you reduce complexity. Over time, as your application grows, code becomes easier to manage.
5. Write Unit Tests
Unit tests are not just an afterthought; they're a crucial part of writing maintainable code. Properly implemented tests can catch regressions, clarify expectations, and serve as documentation for how your code is expected to behave.
Code Snippet
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class DiscountManagerTest {
@Test
public void testApplyDiscountForGoldMember() {
DiscountManager discountManager = new DiscountManager();
double result = discountManager.applyDiscount(100.0, MembershipLevel.GOLD);
assertEquals(90.0, result); // Expect 10% discount
}
}
Why it Matters
Unit tests ensure that your code behaves as expected. Whenever a modification is made, you can re-run your tests to confirm that existing functionality hasn’t been broken. Well-tested code is more maintainable because it provides confidence during refactoring or adding new features.
The Closing Argument
Writing maintainable code is an essential practice in software development, particularly in Java. By adhering to naming conventions, following the DRY principle, using comments wisely, favoring composition over inheritance, and writing unit tests, you can create a codebase that is not only easy to read but also robust and flexible.
These principles may seem simple, but they hold the key to a successful development experience. As you adopt these practices in your Java programming, your code will become more maintainable, helping you and your team save time and effort in the long run.
For an in-depth read on writing clean code, consider exploring Clean Code by Robert C. Martin, a classic in the arena of software engineering. You can also look into Effective Java by Joshua Bloch, which focuses on best practices specific to Java programming.
By committing to these five principles, you will enhance not only the quality of your code but also the overall effectiveness of your teamwork. Happy coding!