Common Pitfalls When Starting with Apache Lucene
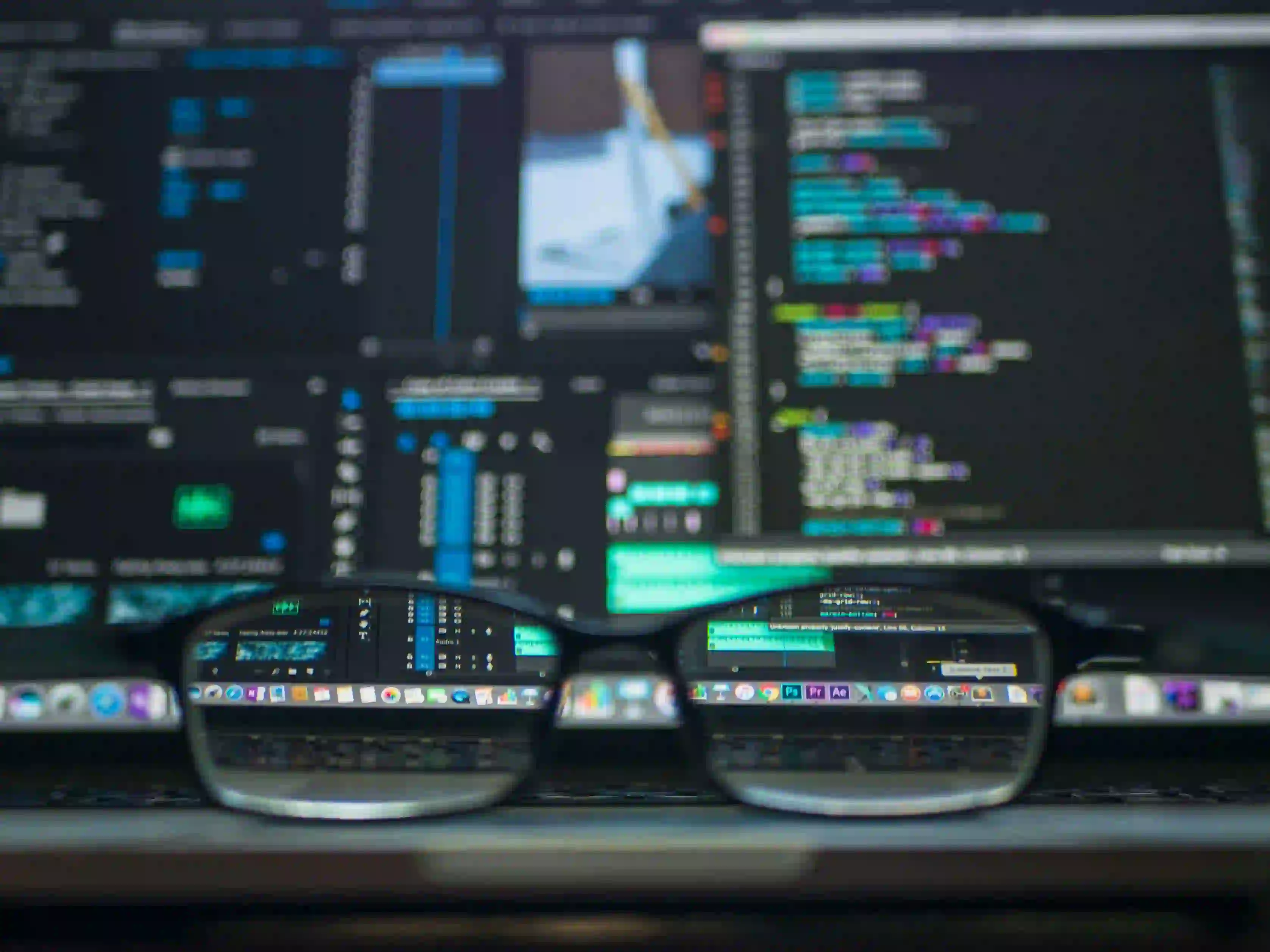
Common Pitfalls When Starting with Apache Lucene
Apache Lucene is a robust and powerful search library used for indexing and searching text data. It is the backbone of many search engines, including Elasticsearch and Apache Solr. While its capabilities are extensive, beginners often encounter common pitfalls when starting with Lucene. This post will highlight these pitfalls, provide some best practices, and offer code snippets to enhance your understanding.
1. Ignoring the Importance of Analyzers
What is an Analyzer?
Analyzers are crucial components in Lucene responsible for breaking down text into meaningful tokens. They do this through tokenization, which assesses the content, determines relevant terms, and removes noise (like stop words).
Common Pitfall
One common mistake is failing to choose the right analyzer for your use case. Using a generic analyzer can lead to suboptimal search results.
Best Practice
Evaluate your data and select an analyzer that fits your needs. For example, if you are dealing with English text, you might consider using the StandardAnalyzer
.
import org.apache.lucene.analysis.standard.StandardAnalyzer;
StandardAnalyzer analyzer = new StandardAnalyzer();
Why: The StandardAnalyzer
is designed to work effectively with English text, providing appropriate tokenization and normalization (like lowercasing).
Additional Resources
For an in-depth understanding of analyzers, refer to the Lucene Analysis documentation.
2. Misunderstanding Indexing
What is Indexing?
Indexing is the process of adding documents to the Lucene index, which allows for efficient searching later on.
Common Pitfall
Many beginners think that indexing text is straightforward and simply add documents without understanding the structure of a Lucene document.
Best Practice
Always define your document schema clearly. Each document should contain fields, and each field should be given an appropriate type.
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.StringField;
import org.apache.lucene.document.TextField;
Document doc = new Document();
doc.add(new StringField("id", "1", Field.Store.YES));
doc.add(new TextField("content", "This is a sample document.", Field.Store.YES));
Why: The StringField
is used for indexed but not analyzed data, while the TextField
is for indexed and analyzed data. This distinction is key for optimal performance in searching.
3. Underestimating the Importance of Query Parsing
What is Query Parsing?
Query parsing is the process by which user input is transformed into a format that can be understood by Lucene, facilitating the search process.
Common Pitfall
Many beginners think they can simply search using raw strings without parsing correctly, which often leads to unexpected results.
Best Practice
Use QueryParser
to transform user input into a query. It automatically handles operators and ensures the query is formatted correctly.
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.Query;
String queryString = "sample";
QueryParser parser = new QueryParser("content", analyzer);
Query query = parser.parse(queryString);
Why: Using QueryParser
allows complex inputs from users to be parsed, incorporating features like phrase searching and boolean operators, enhancing search capabilities.
4. Not Handling Relevance Ranking
What is Relevance Ranking?
Relevance ranking is a method used to order the results of a search query based on how similar they are to the search term.
Common Pitfall
It's easy to overlook relevance ranking when beginning with Lucene. Simplistic searches might yield excessive results without any prioritization.
Best Practice
Utilize Lucene’s built-in scoring capabilities, customizing the scoring by using Boosting
.
import org.apache.lucene.search.BooleanClause;
import org.apache.lucene.search.BooleanQuery;
// Boosting content field
BooleanQuery.Builder builder = new BooleanQuery.Builder();
builder.add(new BoostQuery(new TermQuery(new Term("content", "sample")), 2.0f), BooleanClause.Occur.SHOULD);
Query boostedQuery = builder.build();
Why: Boosting allows specific fields or terms to weigh more heavily in the search results, improving the relevance of the output.
5. Neglecting Caching Strategies
What is Caching?
Caching in Lucene involves storing frequently accessed search results for quicker retrieval upon subsequent queries.
Common Pitfall
New users often neglect the potential for caching, resulting in unnecessarily slow performance, especially with repeat searches.
Best Practice
Implement caching mechanisms, particularly for repetitive queries or expensive operations.
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.cache.CacheKey;
import org.apache.lucene.search.cache.Cacheable;
IndexSearcher searcher = new IndexSearcher(reader);
searcher.setQueryCache(null); // Consider setting up a proper cache implementation
Why: Utilizing caching ensures that repeated searches execute faster, improving overall system performance and user satisfaction.
6. Failing to Optimize Indexes
What is Index Optimization?
Index optimization is the process of improving the search performance by reducing the size of the index and improving the access speed.
Common Pitfall
Many developers skip index optimization, which grows larger over time, impacting search performance significantly.
Best Practice
Regularly optimize your Lucene index to enhance efficiency. This includes merging segments and erasing deleted documents.
import org.apache.lucene.index.IndexWriter;
indexWriter.forceMerge(1); // merges all segments into one
Why: Regularly optimizing indexes can drastically improve search performance and reduce resource usage.
To Wrap Things Up
Starting with Apache Lucene can be challenging, but understanding and avoiding these common pitfalls can significantly enhance your experience. By carefully selecting analyzers, structuring your documents properly, using the right query parsing strategies, considering relevance ranking, implementing caching, and optimizing your indexes, you can harness the full power of Lucene for efficient search functionalities.
For further information and detailed tutorials, visit Apache Lucene's official documentation and explore the many features that Lucene has to offer. Happy coding!