Tackling Java: Overcoming Zoom SDK Integration Issues
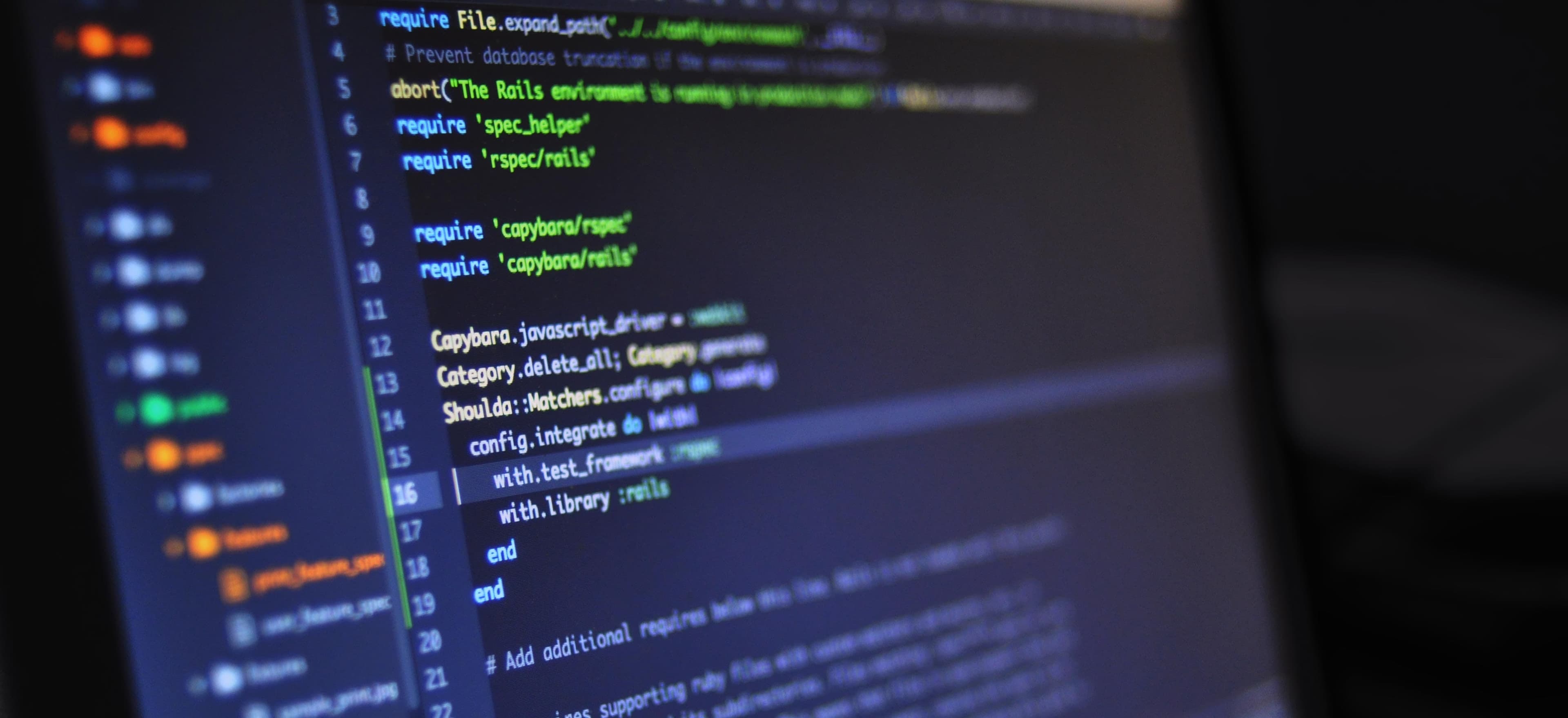
- Published on
Tackling Java: Overcoming Zoom SDK Integration Issues
Integrating external services into a Java application can be challenging, especially when working with tools like the Zoom SDK. While the Zoom SDK offers powerful features for video conferencing, developers often encounter various hurdles during integration. However, with the right approach and knowledge, these challenges can be effectively navigated.
In this blog post, we will discuss various strategies for overcoming common issues associated with integrating the Zoom SDK in Java applications. Additionally, we will cover some essential coding practices to simplify your integration process and enhance the stability of your application.
Understanding the Zoom SDK
The Zoom SDK provides developers with the capability to embed Zoom’s video conferencing features into their applications. This SDK allows for functionalities such as video calls, instant messaging, and screen sharing, creating an enriched user experience. You can find more about Zoom SDK features here.
Before diving into solutions, it’s important to familiarize yourself with the basic setup required for integrating the Zoom SDK in Java.
Basic Configuration
To get started with the Zoom SDK, make sure to have the following prerequisites:
- Java JDK: Ensure you have Java Development Kit installed on your machine.
- Zoom SDK: Download the Zoom SDK from the Zoom Developer Portal.
- IDE: Use an Integrated Development Environment like IntelliJ IDEA or Eclipse for efficient coding.
Initial Steps for Integration
To begin with, follow these steps to set up the Zoom SDK in your Java application:
- Create a Maven Project: Use Maven to manage project dependencies. Here is how to create a basic
pom.xml
:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>zoom-sdk-integration</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- Add Zoom SDK Dependency Here -->
<dependency>
<groupId>us.zoom</groupId>
<artifactId>zoom-sdk</artifactId>
<version>5.9.0</version> <!-- Replace with the latest version -->
</dependency>
</dependencies>
</project>
The above configuration sets up the project with the necessary dependencies. Be sure to replace the SDK version with the latest one available.
Common Hurdles
While integrating the Zoom SDK, developers often face several common issues. Below we will discuss some of these hurdles and strategies to overcome them.
1. Authentication Problems
One of the primary issues developers face is related to authentication. The Zoom SDK requires a JWT (JSON Web Token) for authenticating API requests. If your authentication process is not correctly configured, you'll face challenges in interacting with the Zoom service.
Solution
Make sure you generate the JWT token correctly. Here’s a basic structure to help you create a JWT authenticator class:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
public class JwtUtil {
private static final String SECRET_KEY = "your_secret_key"; // Use a strong key
public static String generateToken(String userId) {
return Jwts.builder()
.setSubject(userId)
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + 1000 * 60 * 60 * 10)) // 10 hours expiry
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
}
2. SDK Initialization Errors
Another common hurdle is the proper initialization of the Zoom SDK. Failing to initialize the SDK correctly can prevent features from working as intended.
Solution
Ensure that you initialize the SDK in your main application class as shown below:
import us.zoom.sdk.ZoomSDK;
import us.zoom.sdk.ZoomSDKInitParams;
public class ZoomApp {
public static void main(String[] args) {
ZoomSDK zoomSDK = ZoomSDK.getInstance();
ZoomSDKInitParams params = new ZoomSDKInitParams();
params.appKey = "your_app_key";
params.appSecret = "your_app_secret";
params.domain = "zoom.us"; // Domain for the SDK; usually zoom.us for US
if (!zoomSDK.initialize(params)) {
System.out.println("SDK initialization failed");
return;
}
System.out.println("SDK initialized successfully");
}
}
3. Meeting Join Issues
Developers sometimes report problems when trying to join meetings due to various factors such as incorrect meeting IDs or not handling the instantiation of the meeting object correctly.
Solution
Ensure you validate the meeting details before trying to join. Here’s an efficient way to handle the meeting join process:
import us.zoom.sdk.JoinMeetingOptions;
import us.zoom.sdk.MeetingService;
public void joinMeeting(String meetingId, String password) {
MeetingService meetingService = ZoomSDK.getInstance().getMeetingService();
JoinMeetingOptions options = new JoinMeetingOptions();
options.no_dragon_board = true; // Customize options as needed
int result = meetingService.joinMeeting(meetingId, password, options);
if (result != 0) {
System.out.println("Failed to join meeting, error code: " + result);
} else {
System.out.println("Joining meeting...");
}
}
Best Practices for Successful Integration
Integrating with the Zoom SDK is more than just writing code. It’s crucial to adopt best practices that enhance maintainability and efficiency.
1. Monitor SDK Updates
Zoom frequently updates its SDK with new features and performance improvements. Keep your SDK version up-to-date to leverage these advancements.
2. Error Logging
Implement robust error-handling and logging practices. By catching exceptions and logging errors, you can more easily diagnose issues as they arise.
3. Follow the Documentation
The Zoom SDK Documentation is an invaluable resource that provides guidelines, API references, and sample code. Consulting the documentation can save you hours of debugging.
4. Engage with Community Resources
If you encounter issues, don’t hesitate to reach out to community resources. Websites like Stack Overflow or the Zoom Developer Forum can provide insights from other developers who have faced similar challenges.
5. Refer to Existing Articles
For more comprehensive insights on overcoming Zoom SDK hurdles, check out the article titled "Zoom SDK Integration: Navigating Common Hurdles". It offers additional strategies and technical details that can complement the solutions discussed in this post.
A Final Look
Integrating the Zoom SDK into Java applications can present a series of challenges, but with strategic planning and thoughtful implementation, these hurdles can be effectively managed. By ensuring proper authentication, initialization, and maintaining a focus on best practices, developers can create seamless video conferencing experiences.
Be sure to leverage community resources, documentation, and existing articles to aid in your integration journey. By keeping up-to-date with SDK changes and learning from others' experiences, you can navigate the integration landscape successfully.
Happy coding!
Checkout our other articles