Tackling Java Network Programming: Common Pitfalls to Avoid
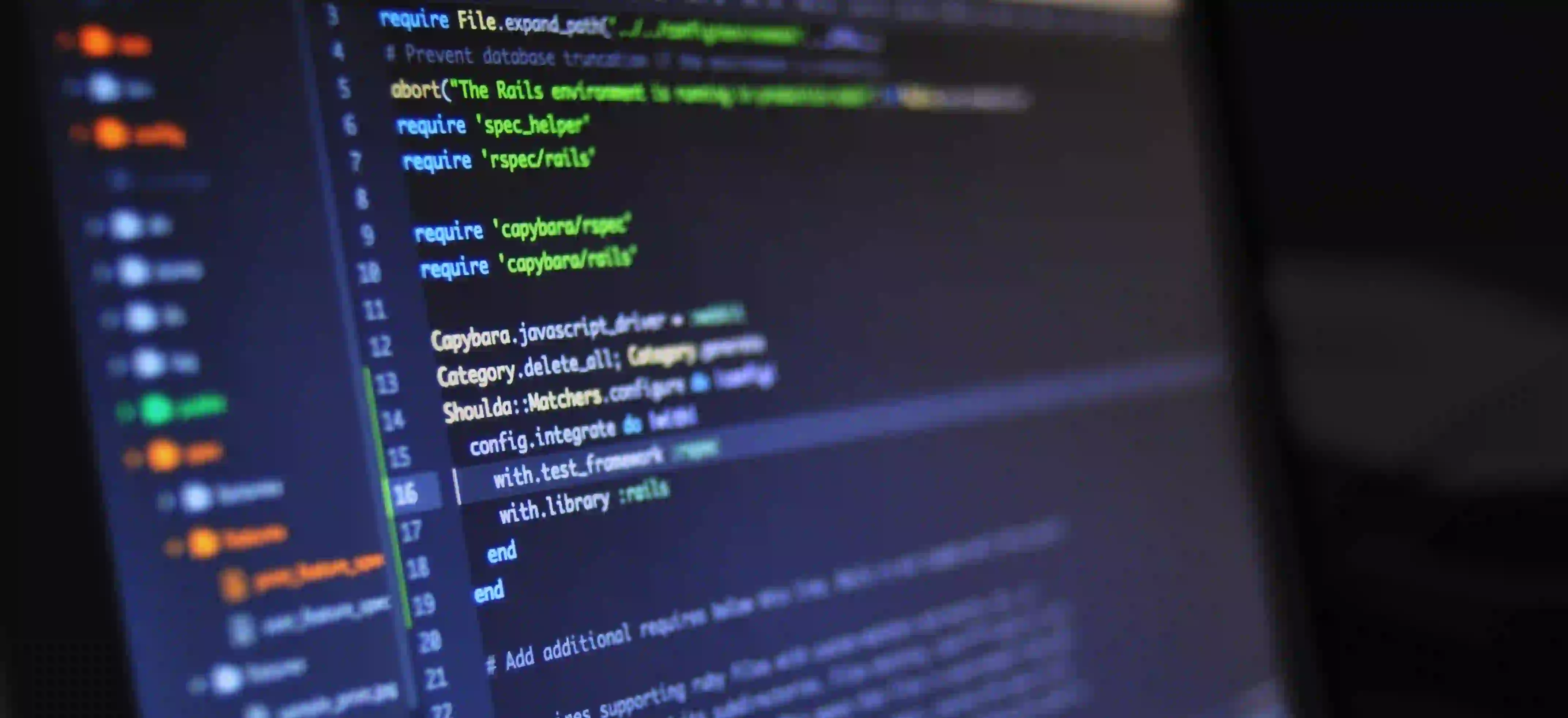
Tackling Java Network Programming: Common Pitfalls to Avoid
Java is a robust programming language that offers a simplified way to handle networking tasks, thanks to its rich set of APIs. However, just like any powerful tool, Java's networking features can be complex and daunting for newcomers. In this post, we’ll explore some common pitfalls that both beginners and experienced Java developers encounter in network programming and how to avoid them effectively.
Understanding Java Network Programming
Java's network programming capabilities are primarily facilitated through the java.net
package. This package provides classes for implementing networking applications, including server and client side sockets, and high-level abstractions like URLs and connections.
Let's dive into some essential concepts and common mistakes.
Common Pitfalls in Java Network Programming
1. Ignoring Exceptions
Networking can often lead to unpredictable issues, such as unreachable servers, timeouts, and connection refusals. A common mistake is failing to handle exceptions properly, which can lead to unresponsive or crashed applications.
Code Snippet: Exception Handling in Networking
import java.io.*;
import java.net.*;
public class NetworkExample {
public static void main(String[] args) {
try {
Socket socket = new Socket("example.com", 80);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
out.println("GET / HTTP/1.1");
out.println("Host: example.com");
out.println();
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
} catch (UnknownHostException e) {
System.err.println("Unknown host: example.com");
} catch (IOException e) {
System.err.println("Error in communication");
} finally {
System.out.println("Connection closed.");
}
}
}
Why Handling Exceptions is Important: Utilizing try-catch blocks ensures that your application remains stable even when outside factors cause disruptions. Not handling exceptions can lead to a poor user experience.
2. Not Closing Connections
Another frequently overlooked aspect is the importance of closing connections. Neglecting to close sockets, files, or input/output streams can lead to resource leaks and eventually exhaust system resources.
Code Snippet: Closing Resources
import java.io.*;
import java.net.*;
public class SafeNetworkExample {
public static void main(String[] args) {
Socket socket = null;
PrintWriter out = null;
BufferedReader in = null;
try {
socket = new Socket("example.com", 80);
out = new PrintWriter(socket.getOutputStream(), true);
in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out.println("GET / HTTP/1.1");
out.println("Host: example.com");
out.println();
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
} catch (IOException e) {
System.err.println("Network error " + e.getMessage());
} finally {
try {
if (in != null) in.close();
if (out != null) out.close();
if (socket != null) socket.close();
} catch (IOException ex) {
ex.printStackTrace();
}
System.out.println("Resources released.");
}
}
}
Why You Should Always Close Resources: Closing resources promptly helps free up system resources. It ensures that sockets and streams are not left open indefinitely, which could lead to memory leaks.
3. Hardcoding Values
Hardcoding IP addresses, ports, and other configuration settings can lead to inflexible and hard-to-maintain code. Changes to these values often require editing the codebase, which can introduce errors or lead to downtime.
Strategy: Use Config Files
Instead, consider using configuration files to store adjustable parameters. Java's Properties
class can help manage these configurations.
import java.io.*;
import java.net.*;
import java.util.Properties;
public class ConfigurableNetworkExample {
public static void main(String[] args) {
Properties properties = new Properties();
try (FileInputStream input = new FileInputStream("config.properties")) {
properties.load(input);
String host = properties.getProperty("host");
int port = Integer.parseInt(properties.getProperty("port"));
try (Socket socket = new Socket(host, port);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()))) {
out.println("GET / HTTP/1.1");
out.println("Host: " + host);
out.println();
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Avoid Hardcoding: Making your application configurable allows for quick adjustments without the need to alter the codebase. This practice is crucial for production-grade applications.
4. Not Setting Timeouts
Network latency can be a significant hurdle in applications. Failing to set timeouts for your socket can result in your application hanging indefinitely if the remote server becomes unresponsive.
Code Snippet: Setting Timeouts
import java.io.*;
import java.net.*;
public class TimeoutExample {
public static void main(String[] args) {
Socket socket = null;
try {
socket = new Socket();
socket.connect(new InetSocketAddress("example.com", 80), 5000); // 5 seconds timeout
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
out.println("GET / HTTP/1.1");
out.println("Host: example.com");
out.println();
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
} catch (SocketTimeoutException e) {
System.err.println("Connection timed out");
} catch (IOException e) {
System.err.println("Error in communication");
} finally {
if (socket != null && !socket.isClosed()) {
try {
socket.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
System.out.println("Socket closed.");
}
}
}
Why Set Timeouts: Implementing timeouts prevents your application from indefinitely waiting for a response, enhancing reliability.
5. Skipping Security Considerations
In today's world, securing network applications is more critical than ever. Failing to implement proper security measures, such as encryption, can expose sensitive data to potential threats.
Use Secure Connections
Whenever possible, use secure protocols (like HTTPS). Here’s a simple way to create an SSL socket.
import javax.net.ssl.*;
import java.io.*;
public class SecureSocketExample {
public static void main(String[] args) {
try {
SSLSocketFactory factory = (SSLSocketFactory) SSLSocketFactory.getDefault();
SSLSocket socket = (SSLSocket) factory.createSocket("example.com", 443);
socket.startHandshake();
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out.println("GET / HTTP/1.1");
out.println("Host: example.com");
out.println();
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Secure Your Connections: The consequences of disregarding security can be severe, including data breaches and loss of customer trust. Secure sockets provide essential encryption that can help safeguard sensitive information.
In Conclusion, Here is What Matters
Java network programming can unlock incredible potential for your applications, but it does come with its set of challenges. By addressing common pitfalls, such as exception handling, resource management, configuration, timeout settings, and security, you set a solid foundation for your network applications.
To further enhance your network programming skills, consider reading the article "5 Common Network Issues Beginners Face and Fix," available at configzen.com/blog/common-network-issues-beginners-fix.
With the right practices in place, you'll be well on your way to developing resilient and reliable networked applications in Java. Happy coding!