How to Handle Auto-Generated URLs in Java Applications
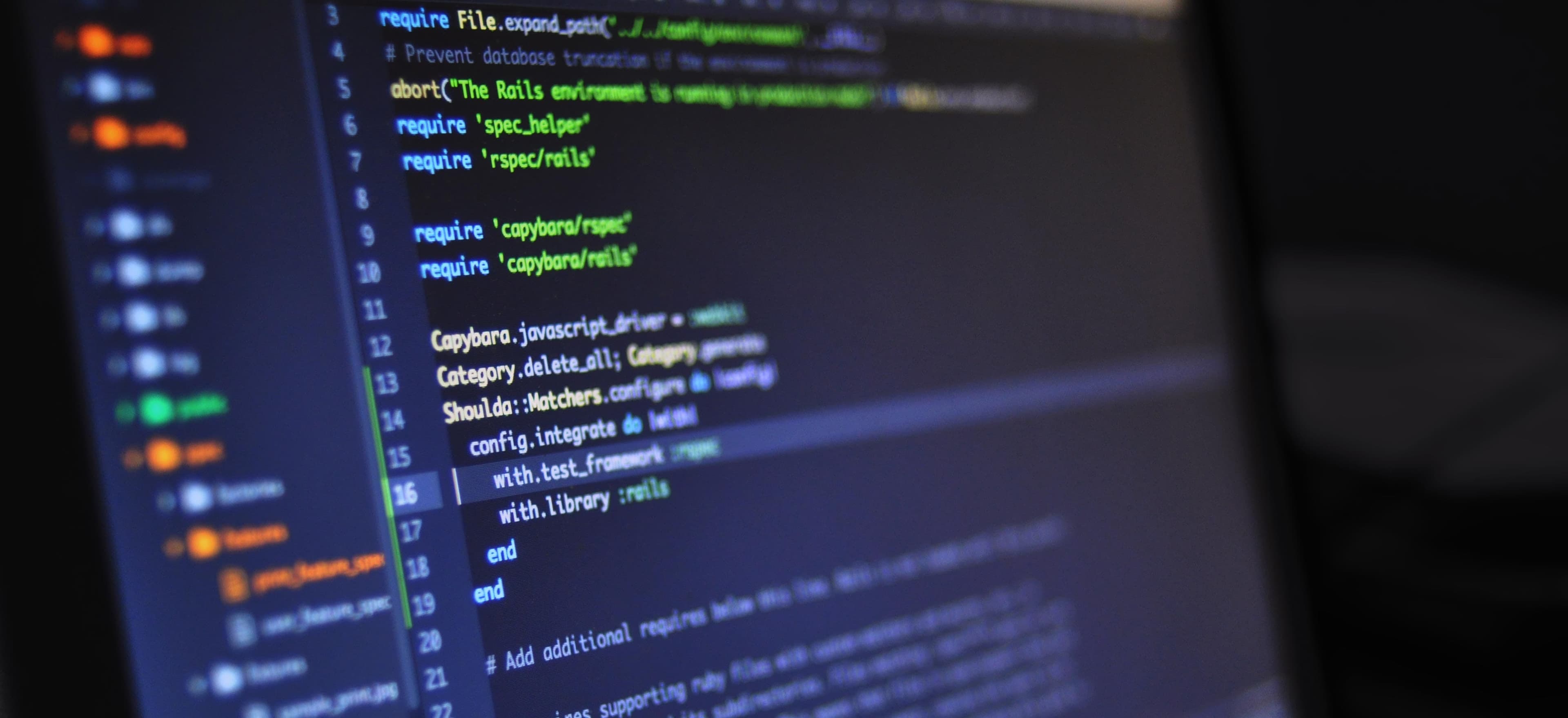
- Published on
How to Handle Auto-Generated URLs in Java Applications
In modern web development, auto-generated URLs are an essential feature, especially for preview environments and dynamic content. However, they can present challenges, particularly when it comes to maintaining structure, search engine optimization (SEO), and usability. This article will explore how to handle auto-generated URLs in Java applications, ensuring that your web app remains both user-friendly and easy for search engines to index.
Understanding Auto-Generated URLs
Auto-generated URLs are links that are created programmatically rather than manually specified. This is common in various scenarios, such as:
- Content Management Systems (CMS) that generate URLs based on user-generated content
- E-commerce applications with product categories and filtering
- RESTful APIs that expose resources dynamically
While auto-generation can simplify link creation, it often leads to URLs that are long, complex, and riddled with parameters, which may confuse users and search engines alike.
The Problem with Auto-Generated URLs
One relevant discussion is the challenges associated with auto-generated URLs in preview environments. The article titled The Problem with Auto-Generated URLs for Preview Environments highlights these issues, including poor user experience and potential SEO pitfalls.
Understanding these problems is vital so that Java developers can implement best practices to generate effective URLs.
Best Practices for Generating Clean URLs
Here are some essential best practices to adhere to while generating URLs in your Java applications:
1. URL Structure: Keep It Simple and Descriptive
URLs should be clear, concise, and descriptive. A good URL provides context about the content contained within. For example:
String username = "john.doe";
String postTitle = "how-to-handle-URL-generation";
String generatedUrl = String.format("/users/%s/posts/%s", username, postTitle);
System.out.println(generatedUrl); // Output: /users/john.doe/posts/how-to-handle-URL-generation
This URL is user-friendly and conveys what the user can expect from it. Adjusting the URL to remove special characters, replacing spaces with hyphens, and ensuring lower-case letters will also improve readability.
2. Use a URL Shortener for Long URLs
For very long-generated URLs, consider using a URL shortener service or implementing an in-house solution. A short link can redirect to a longer one while maintaining user-friendly characteristics.
String longUrl = "https://example.com/products/category?id=12345&ref=homepage";
String shortUrl = shortenUrl(longUrl); // Implement this method using a URL-shortening API
System.out.println(shortUrl); // Output: https://exmpl.com/abc123
3. Implement URL Mapping with Java Frameworks
If you’re using Java frameworks like Spring or Java EE, take advantage of URL mapping annotations to structure your URLs better. Here's how you can use Spring MVC:
@Controller
@RequestMapping("/posts")
public class PostController {
@GetMapping("/{username}/{postTitle}")
public String viewPost(@PathVariable String username, @PathVariable String postTitle, Model model) {
// Logic to retrieve and display the post details
return "postView";
}
}
In this example, the URL structure coincides beautifully with the controller’s annotations. Using these frameworks provides a layer of abstraction and makes working with URLs straightforward.
4. Normalize URLs
To prevent duplicate content and potential SEO issues, normalize URLs by redirecting all variants of a URL to a single canonical form. You can achieve this using Java filters or application configurations.
Example using a servlet filter to redirect:
@WebFilter(filterName = "UrlNormalizationFilter", urlPatterns = {"/*"})
public class UrlNormalizationFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) request;
String uri = httpRequest.getRequestURI();
// Redirecting to canonical URL
if (uri.contains("//")) {
String normalizedUrl = uri.replace("//", "/");
((HttpServletResponse) response).sendRedirect(normalizedUrl);
return;
}
chain.doFilter(request, response);
}
}
This filter checks for double slashes and performs a redirect to normalize the URL.
5. Leverage Query Parameters Wisely
When injecting parameters into URLs, use them sparingly and only when necessary. Too many query parameters can make a URL unwieldy. Instead of:
https://example.com/products?id=12345&category=electronics&sort=price&filter=latest
You might consider using cleaner routes:
https://example.com/products/electronics/latest
In Java, your controller can handle the mapping simply:
@GetMapping("/products/{category}/{filter}")
public String listProducts(@PathVariable String category, @PathVariable String filter) {
// Logic to retrieve products based on the category and filter
return "productList";
}
6. Logging and Analytics for Monitoring
Regularly monitor and analyze your auto-generated URLs' performance. Use logging in your application to capture how users engage with auto-generated links.
logger.info("Generated URL accessed: {}", generatedUrl);
Integrating tools like Google Analytics can enhance your understanding of user interactions with these URLs.
7. A/B Testing Your URLs
Consider conducting A/B tests to assess how users respond to different URL structures. This can be instrumental in optimizing for both conversion rates and SEO.
String variantA = "/products/item123";
String variantB = "/products/item-123";
Monitor engagement metrics for the two variants to determine which performs better in terms of user click-through rates.
Lessons Learned
Effectively managing auto-generated URLs in Java applications is a cornerstone of creating a user-friendly web environment. By following best practices around simplicity, navigation, SEO, and analytics, you can ensure that your application provides a better experience for both users and search engines.
Remember, dealing with URLs is not just about technical implementation. It’s about creating a structure that users can trust and understand. For more insights on related challenges, you may refer to the article The Problem with Auto-Generated URLs for Preview Environments.
Engage with your URLs and their structure, and let your Java application shine in both usability and performance!