Java Error Handling: When to Bug-In or Bug-Out
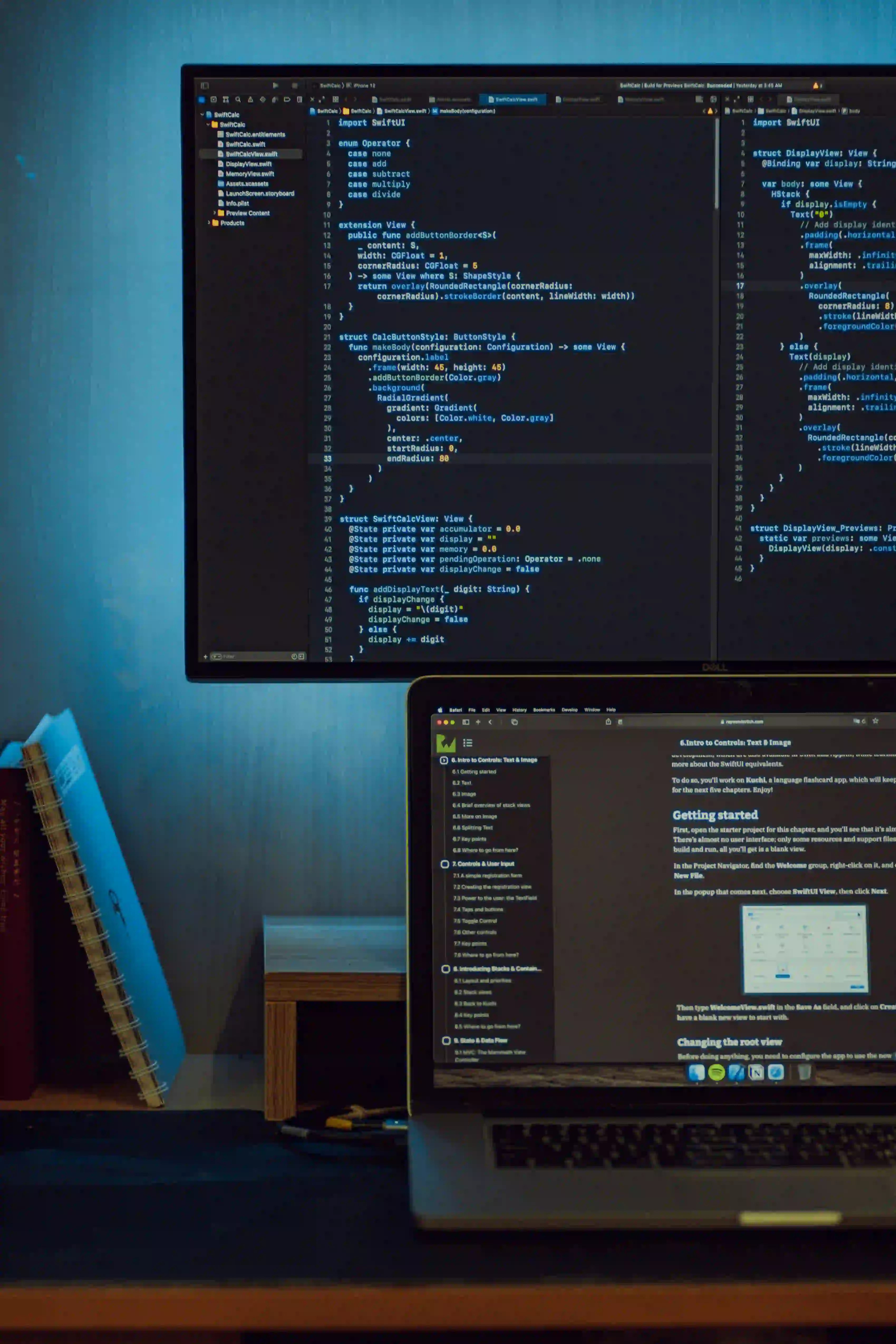
Java Error Handling: When to Bug-In or Bug-Out
In the realm of programming, particularly in Java development, error handling can sometimes feel like a survival scenario. Just as you might choose to “bug-in” for safety or “bug-out” for a quick escape—like in outdoor survival situations outlined in "Bug-In vs. Bug-Out: Making the Right Survival Choice" (youvswild.com/blog/bug-in-vs-bug-out-survival-choice)—error handling in Java requires you to assess when to manage errors internally versus when to let them propagate outside of your current context.
This blog post will explore error handling strategies in Java, providing guidance on when to "bug-in" by managing errors and when to "bug-out" by letting them escape the method context.
Understanding Java Exceptions
To navigate the landscape of error handling in Java, it's imperative to first understand the types of exceptions. Java categorizes exceptions into two main types:
-
Checked Exceptions: These are exceptions that need to be declared in a method's
throws
clause or handled within the method. They are checked at compile-time. Examples includeIOException
,SQLException
, andClassNotFoundException
. -
Unchecked Exceptions: Also known as runtime exceptions, these exceptions don’t need to be declared or caught. They usually arise from programming errors, such as
NullPointerException
,ArrayIndexOutOfBoundsException
, andArithmeticException
.
This classification is similar to the choice of whether to bug-in or bug-out. Sometimes, you need to take the time to address an issue thoroughly (checked) while other times, the error simply indicated a broader problem (unchecked).
The Art of 'Bugging-In'
In programming, "bugging-in" translates to certain strategies where you choose to handle exceptions as they arise, ensuring your program remains stable and user-friendly. Below are some common scenarios to "bug-in".
1. Logging Errors
Instead of bombarding users with error messages, log the error for debugging purposes.
import java.util.logging.Logger;
public class ErrorLogger {
private static final Logger logger = Logger.getLogger(ErrorLogger.class.getName());
public void processFile(String filePath) {
try {
// Simulate file processing
throw new IOException("File not found");
} catch (IOException e) {
logger.severe("Error occurred: " + e.getMessage());
// Provide user-friendly message or handling
}
}
}
Why This Matters: Logging allows developers to identify and debug issues without user interference. It’s a way of "bugging-in" to keep everything running smoothly while keeping a record of unexpected behavior.
2. Retrying Operations
Sometimes, temporary situations cause errors. In such cases, it might be wise to "bug-in" and retry the operation.
public class RetryOperation {
public void fetchData() {
int attempts = 0;
while (attempts < 3) {
try {
// Perform network operation
attempts++;
throw new IOException("Network issue");
} catch (IOException e) {
if (attempts < 3) {
System.out.println("Retrying... Attempt " + attempts);
} else {
System.out.println("Failed to fetch data after 3 attempts.");
}
}
}
}
}
Why This Matters: Automatic retries can enhance user experience, ensuring that transient errors do not impact overall functionality. By managing the issue internally, the application maintains its zen-like state.
3. Validating Inputs
Another critical aspect is input validation. You can catch errors before they become exceptions.
public class InputValidator {
public void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
// Set age logic
}
}
Why This Matters: Validating inputs helps catch errors early, avoiding costly runtime exceptions. It is an essential step in ensuring code integrity and stability.
The Necessity of 'Bugging-Out'
However, there are moments where you should allow errors to propagate. By "bugging-out," you acknowledge that certain errors are beyond your control, often due to architectural decisions or the nature of the program.
1. Propagating Checked Exceptions
Sometimes, you need to let your method declaration propagate checked exceptions to inform callers of your method.
public class FileHandler {
public String readFile(String filePath) throws IOException {
// Attempt to read file, propagate IOExceptions
Files.readAllLines(Paths.get(filePath));
return "File read successfully";
}
}
Why This Matters: By throwing the IOException
, you're forcing the caller of readFile()
to handle or acknowledge the potential issue. This creates a contract, increasing the robustness of your codebase.
2. Relying on Default Error Handling
In some cases, it’s appropriate to let a higher-level framework handle errors.
public class Application {
public void run() {
try {
// Your application logic here
} catch (Exception e) {
// Allow the server or framework to handle the exception
throw e;
}
}
}
Why This Matters: Allowing higher-level components to deal with exceptions can simplify your application logic and offload error handling responsibilities, particularly for broader contexts.
3. Using Custom Exception Classes
When you need to convey specific information regarding the nature of an error, custom exceptions can be beneficial.
public class DataNotFoundException extends Exception {
public DataNotFoundException(String message) {
super(message);
}
}
// Usage
public class DataService {
public String fetchData(int id) throws DataNotFoundException {
// Logic to fetch data, if not found
throw new DataNotFoundException("Data with id " + id + " not found");
}
}
Why This Matters: Custom exceptions provide clarity for consumers of your method while allowing the caller to decide how to handle specific scenarios.
Key Takeaways: Finding Balance
Mastering Java error handling is integral to the success of any stable application. Like a skilled survivalist, knowing when to "bug-in" by managing errors internally or "bug-out" by allowing exceptions to propagate can mean the difference between a failed application and a resilient one.
By employing effective strategies, such as logging, validating, or propagating exceptions, Java developers can create reliable and user-friendly applications. Remember, whether you choose to bug-in or bug-out, the ultimate goal is to cultivate an environment where errors are manageable, paving the way for a robust user experience.
For more on making informed choices in uncertain circumstances, check out "Bug-In vs. Bug-Out: Making the Right Survival Choice" (youvswild.com/blog/bug-in-vs-bug-out-survival-choice).
By setting the right strategies in place, Java developers can form a strong foundation. It's all about being prepared, making the right calls, and ensuring that your applications thrive in unpredictable environments.