Exploring Java's Role in Visualizing Aura Photography Data
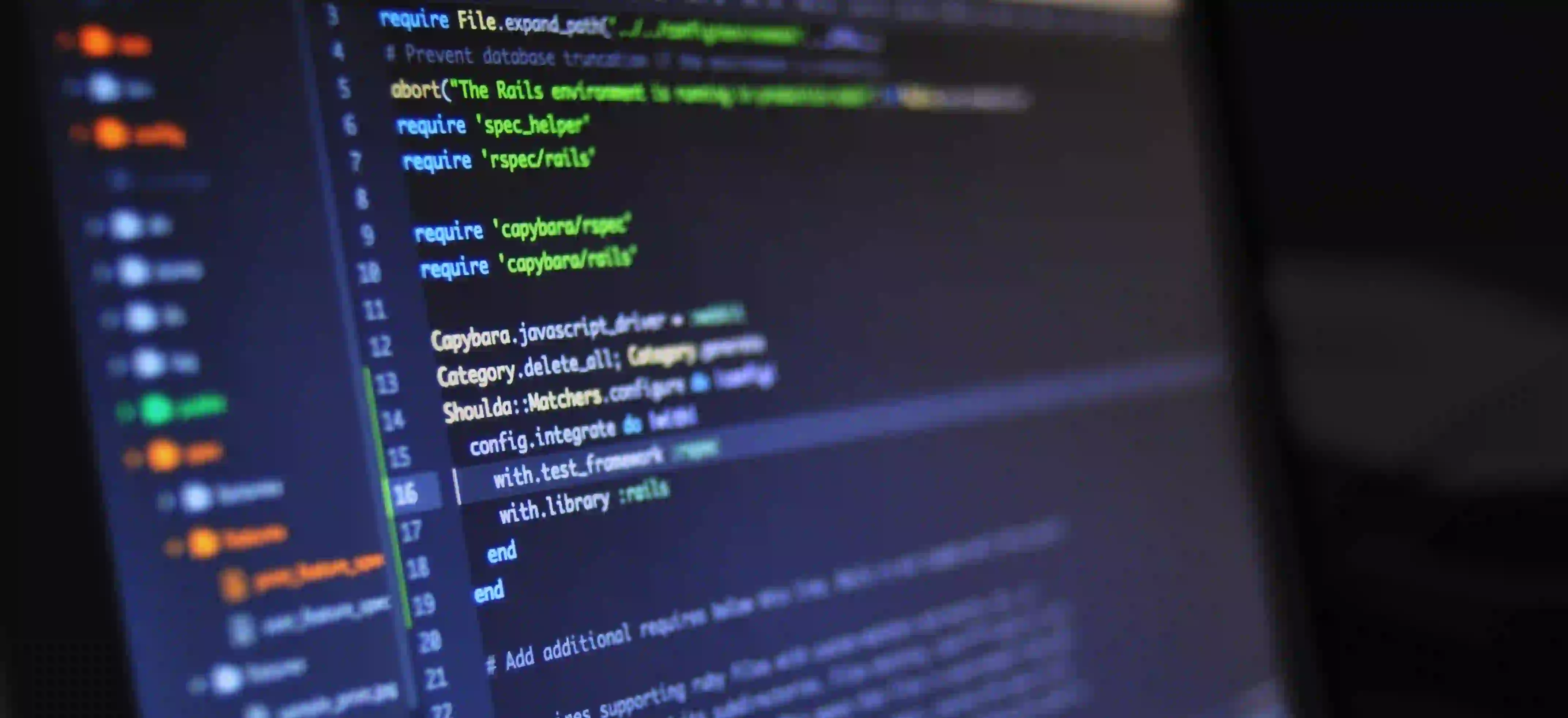
Exploring Java's Role in Visualizing Aura Photography Data
In the digital era, visualizing complex data is critical for both artists and scientists. Aura photography, a fascinating blend of metaphysics and technology, generates visual representations of human energy fields. If you're intrigued by the connection between programming and aura photography, you're in the right place. This blog post explores how Java can be harnessed to visualize aura photography data, creating meaningful representations of human emotional and spiritual states.
Our understanding of aura photography is beautifully encapsulated in an article titled "Aurafotografie: Fenster zur Seele oder Science-Fiction?" (auraglyphs.com/post/aurafotografie-fenster-zur-seele-oder-science-fiction). The juxtaposition of science and art in this context sets the stage for examining Java's role in transforming abstract aura data into visual stories.
Why Visualize Aura Data?
Before diving into Java specifics, it’s essential to understand why we need to visualize aura photography data. Visualizations help in:
-
Understanding: Complex phenomena become more accessible through visual representation.
-
Analysis: Patterns and correlations can be identified more easily.
-
Communication: Data becomes shareable and persuasive when visualized.
The Basics of Java for Visualization
Java is an excellent choice for creating data visualizations due to its portability, ease of deployment, and vast libraries. Let's start by discussing some key libraries and frameworks used for graphic rendering:
- JavaFX: A modern framework for building rich client applications.
- Processing: A flexible software sketchbook and language for learning how to code within the context of the visual arts.
- JFreeChart: Specifically designed for creating various types of charts.
Each of these libraries can play a significant role in aura data visualization. For our purposes, we will explore how to use JavaFX, as it provides rich capabilities for creating stunning visual interactions.
Setting Up Your JavaFX Environment
Before we start coding, make sure you have JavaFX set up in your development environment. You may need to download the JavaFX SDK and link it to your IDE (like IntelliJ IDEA or Eclipse). Here’s how to do it:
- Download the JavaFX SDK.
- Configure your IDE to include the JavaFX libraries. In IntelliJ, for instance, go to File > Project Structure > Libraries and add the JavaFX SDK.
Coding an Aura Visualization Tool
Now that our environment is ready, let’s create a simple aura visualization application. For the sake of this demonstration, we will simulate aura data using random values.
Step 1: Creating the JavaFX Application
Let's create a JavaFX application that renders aura data as colorful circles. Each circle will represent an individual's aura's intensity and hue.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import java.util.Random;
public class AuraVisualizer extends Application {
@Override
public void start(Stage primaryStage) {
Pane pane = new Pane();
Random random = new Random();
for (int i = 0; i < 10; i++) {
// Create a circle with random position and aura intensity
Circle auraCircle = new Circle();
auraCircle.setCenterX(random.nextInt(800));
auraCircle.setCenterY(random.nextInt(600));
auraCircle.setRadius(random.nextInt(50) + 10);
// Random color
auraCircle.setFill(Color.hsb(random.nextInt(360), 1.0, 0.8));
pane.getChildren().add(auraCircle);
}
Scene scene = new Scene(pane, 800, 600);
primaryStage.setTitle("Aura Visualization");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
- Random Circle Generation: We utilize the Random class to generate random positions and sizes, simulating diverse aura aspects.
- Color Mapping: The use of Color.hsb() allows us to translate the aura's emotional spectrum into visible hues. The hue value can correspond to different emotions or states of being.
Step 2: Enhancing the Visualization
While the basic visualization is engaging, let's enhance it by adding interaction—allowing users to click on the circles to see more information about the aura data. For this, we will implement an event listener.
import javafx.scene.input.MouseEvent;
…
auraCircle.addEventHandler(MouseEvent.MOUSE_CLICKED, event -> {
System.out.println("Aura Intensity: " + auraCircle.getRadius());
System.out.println("Aura Color: " + auraCircle.getFill());
});
Commentary on Enhancements
- Event Handling: Adding mouse event handlers enriches the user experience, allowing the audience to interact with the data and derive personal insights.
- Data Representation: By outputting the aura's radius and color upon clicking, we allow users to interpret the data dynamically.
Closing the Chapter
Java is a powerful tool for visualizing aura photography data. By using JavaFX, you can create compelling visual representations that not only enlighten but also engage users. The blending of code and art opens a world of possibilities, allowing individuals to explore their inner wavelengths through the colorful lenses of visualizations.
In summary, whether you're a developer or an enthusiast of aura photography, Java provides the necessary capabilities to transform abstract concepts into intuitive graphics. So, start coding and see how data visualization can make the invisible visible!
For more on aura photography, be sure to check the article on Aurafotografie for thought-provoking insights (auraglyphs.com/post/aurafotografie-fenster-zur-seele-oder-science-fiction). By merging technology and metaphysical concepts, the potential for understanding human energy fields is limitless.
Happy coding!