Integrating Vue UI Libraries with Java Backends Simplified
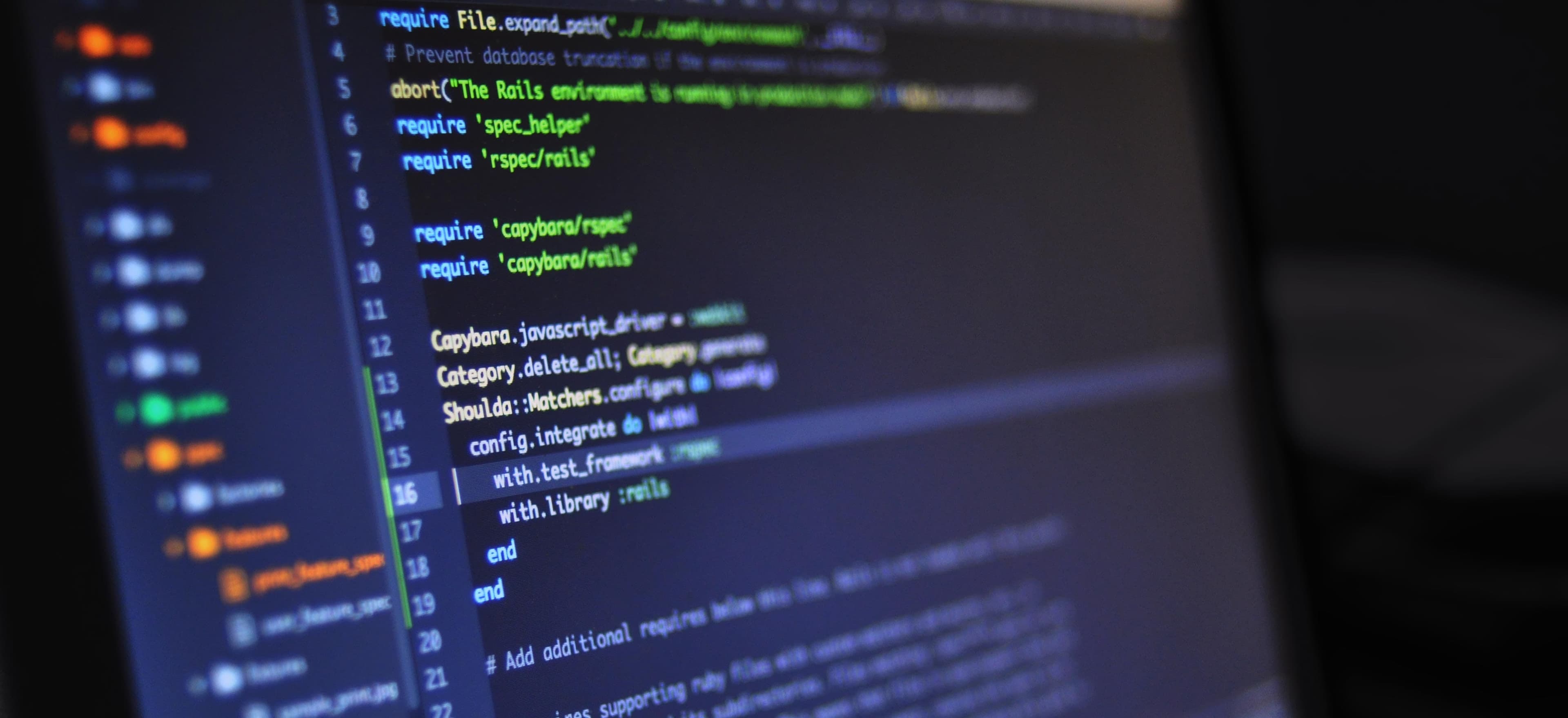
- Published on
Integrating Vue UI Libraries with Java Backends Simplified
When you're diving into modern web development, the combination of a powerful front-end framework like Vue.js with a robust backend solution such as Java can yield exceptional results. This article aims to guide you through integrating Vue UI libraries with Java backends, breaking down complex ideas into digestible segments while providing actionable code snippets.
The Approach to Vue UI Libraries
Vue.js has gained popularity due to its ease of use and flexibility. However, Vue alone cannot create robust user experiences. This is where Vue UI libraries come into play. Libraries like Vuetify, Element UI, and BootstrapVue provide pre-designed components that simplify the user interface development process.
For in-depth insights into choosing the right Vue UI libraries, check out "Struggling to Choose? Top Vue UI Libraries Simplified!" here.
Why Use Java for the Backend?
Java is renowned for its reliability, scalability, and strong community support. It offers numerous frameworks like Spring Boot, which make it easier to create RESTful APIs. This is crucial when you're looking to connect a Vue frontend with a Java backend efficiently.
Key Benefits of Using Java
- Robust Performance: Java applications are known for their speed and efficiency, especially when using multi-threading.
- Community Support: A large community means constant updates, best practices, and resources for troubleshooting.
- Integration Capabilities: Java can easily integrate with various databases and third-party services.
Setting Up Your Development Environment
Before diving into code, make sure you have a suitable development environment. You will need:
- Java JDK: Ensure you have the latest version of the Java Development Kit installed.
- Node.js and NPM: These are essential for managing your Vue.js dependencies.
- IDE: Use an IDE like IntelliJ IDEA for Java and Visual Studio Code or Vue CLI for your Vue development.
Basic Project Structure
Your project structure would generally look like this:
/my-project
├── /backend
│ └── src
│ ├── main
│ │ └── java
│ │ └── com
│ │ └── example
│ │ └── demo
│ └── resources
│ └── application.properties
└── /frontend
├── package.json
├── src
│ └── main.js
└── public
Building the Backend with Spring Boot
Let’s create a simple REST API in Java using Spring Boot. This API will serve as the backend for our Vue front end.
Maven Dependency Setup
First, ensure your pom.xml
includes the necessary Spring Boot dependencies:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
Creating a Simple Rest Controller
Now, let's create a REST endpoint that our Vue app can call.
@RestController
@RequestMapping("/api/items")
public class ItemController {
@GetMapping
public List<Item> getAllItems() {
// This would typically fetch items from the database
return Arrays.asList(new Item(1, "Item 1"), new Item(2, "Item 2"));
}
}
Why This Code Works
- @RestController: This annotation marks the class as a REST controller, allowing it to handle HTTP requests.
- @GetMapping: Maps HTTP GET requests to the
getAllItems
method, which sends back a list of items. - Data Handling: Here, we're simply returning a static list for demonstration, but in a real-world scenario, this would interact with the database.
Setting Up the Vue Frontend
Let’s now set up the Vue side of things, which will consume the REST API we just created.
Creating a Basic Vue App
-
Use Vue CLI to create a new project:
vue create frontend
-
Install Axios for making HTTP requests:
cd frontend npm install axios
Fetching Data from the Java Backend
We’ll create a simple Vue component that fetches the items from our Java backend.
<template>
<div>
<h1>Items List</h1>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
items: []
};
},
created() {
this.fetchItems();
},
methods: {
fetchItems() {
axios.get('http://localhost:8080/api/items')
.then(res => this.items = res.data)
.catch(error => console.error(error));
}
}
}
</script>
Understanding the Vue Component
- Template: The template section contains the HTML that displays the items.
- Data Method: Initializes the
items
array, which will be populated once the data is fetched. - Axios HTTP Request: The
fetchItems
method uses Axios to retrieve data from the Java backend.
Running the Application
Now that both the front end and back end are set up, ensure your Java application is running on port 8080, as specified in the Axios get call. Start your Vue app using:
npm run serve
Navigate to http://localhost:8080
to see your items rendered on the page.
Testing and Debugging
Testing and debugging are essential parts of the development process. Here are some tools you can leverage:
- Postman: This is great for testing your Java REST API endpoints manually.
- Vue Devtools: A Chrome extension that allows you to inspect Vue components and their states.
Best Practices for Integration
-
CORS Configuration: Ensure you configure Cross-Origin Resource Sharing (CORS) in your Java backend. This will allow your Vue front end to make requests to the server.
@Configuration public class WebConfig implements WebMvcConfigurer { @Override public void addCorsMappings(CorsRegistry registry) { registry.addMapping("/api/**").allowedOrigins("http://localhost:8080"); } }
-
Error Handling: Implement robust error handling on both the frontend and backend to ensure a smooth user experience.
-
Environment Variables: Use environment variables to store sensitive data such as API URLs and database credentials.
My Closing Thoughts on the Matter
Integrating Vue UI libraries with a Java backend allows for crafting rich web applications that are fast, reliable, and user-friendly. By following this guide, you are equipped with the knowledge to set up a basic application structure, make HTTP requests, and manage data between your front end and back end.
For further information on selecting the right UI libraries for your Vue application, refer to the article "Struggling to Choose? Top Vue UI Libraries Simplified!" here.
Happy coding!
Checkout our other articles