Fixing Java Application Connectivity with MySQL on EasyPHP
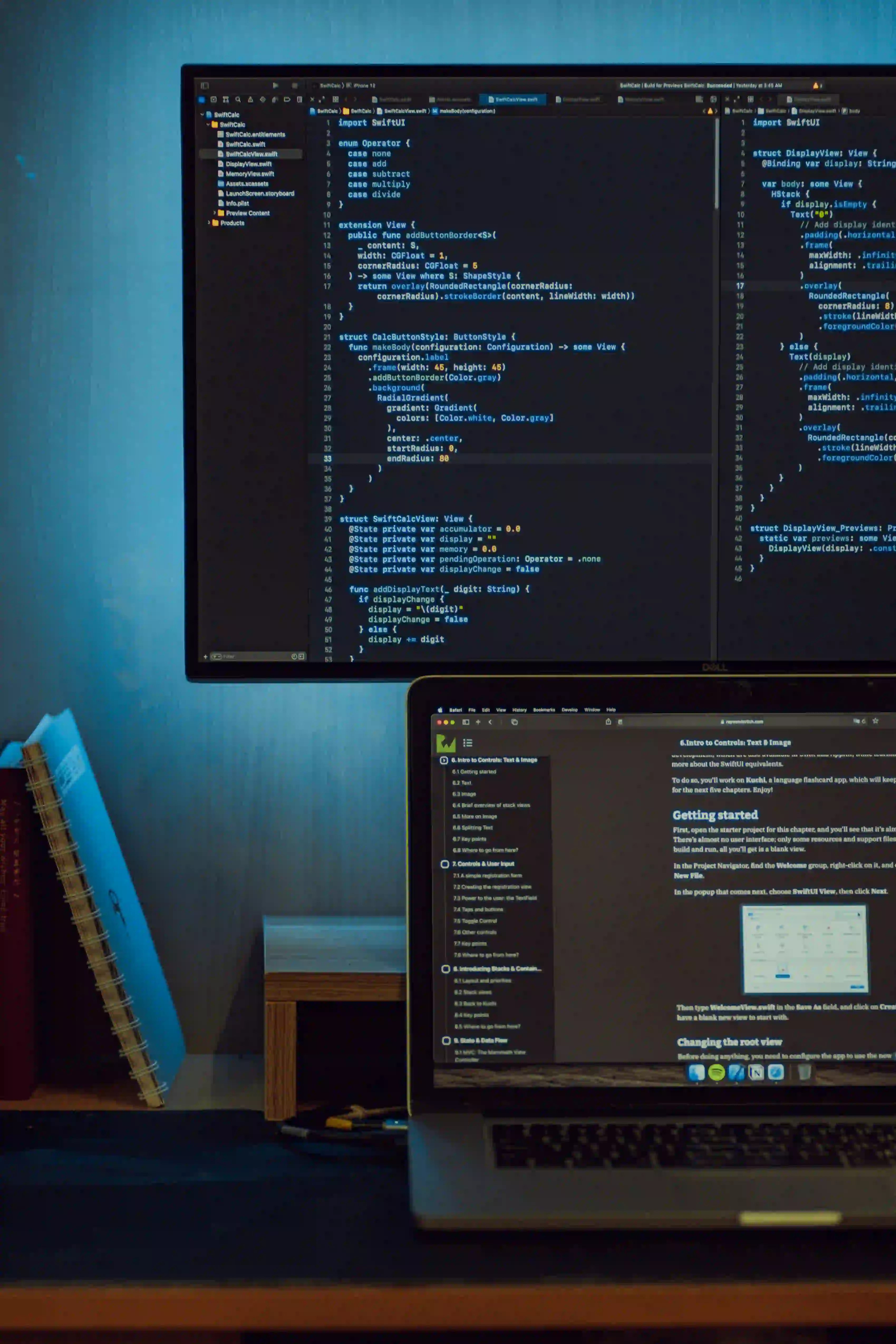
Fixing Java Application Connectivity with MySQL on EasyPHP
Integrating Java applications with MySQL databases can elevate the functionality of both back-end services and applications. However, establishing a robust connection isn't always straightforward, especially when using the EasyPHP stack. In this post, we will explore various strategies to troubleshoot and fix Java application connectivity with MySQL using EasyPHP, ensuring that your project runs smoothly.
Understanding MySQL and EasyPHP
Before diving into the details of how to establish connectivity, let’s clarify what EasyPHP and MySQL are.
What is EasyPHP?
EasyPHP is a Windows-based Web development suite, widely used for developing PHP applications. It bundles PHP, Apache server, and MySQL, providing an out-of-the-box solution for developers.
What is MySQL?
MySQL is a powerful relational database management system (RDBMS) that is popular for storing, retrieving, and managing data in applications. It uses SQL (Structured Query Language) for querying data.
The Importance of Connectivity
A Java application that cannot connect to MySQL is essentially impotent, unable to retrieve or store data. Therefore, ensuring a smooth connection is crucial. Multiple factors could lead to connection issues. Let’s explore some common problems and solutions.
Common Issues in Connectivity
-
Wrong JDBC URL: The JDBC (Java Database Connectivity) URL specifies the database server's address and other details necessary for connecting. A common pitfall is an incorrect or malformed URL.
-
Firewall Restrictions: Often, a firewall can block the connection. This can be on the client’s machine or the server's side.
-
Driver Misconfiguration: The right JDBC driver must be included in the application’s classpath. Failing to do so can result in connection failures.
-
User Credentials: Incorrect username or password can prevent access. It's vital to verify that the credentials used are valid.
-
MySQL Server Not Running: The MySQL service itself must be up and running. It might have crashed or been stopped inadvertently.
Establishing a Connection: Step-by-Step Approach
Step 1: Configure the JDBC URL
The JDBC URL is critical for the connection. Below is an example of how to set it up and why each part matters.
String url = "jdbc:mysql://localhost:3306/myDatabase?useSSL=false";
String user = "root";
String password = "mypassword";
Connection connection = null;
try {
connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection established successfully.");
} catch (SQLException e) {
System.out.println("Connection failed: " + e.getMessage());
}
In this snippet:
jdbc:mysql://
indicates that we are using MySQL.localhost:3306
refers to the local server on port 3306 (the default for MySQL).myDatabase
is the name of the database we are trying to connect to.useSSL=false
disables SSL for connection, often necessary if SSL is not configured.
Step 2: Add the JDBC Driver
Without the MySQL Connector/J JDBC driver in the project’s classpath, no connection can be made. This dependency can be added through Maven as follows:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
This ensures that your application can interact seamlessly with the MySQL database.
Step 3: Verify User Credentials
To connect to MySQL, you need appropriate privileges. Here's an example of how to verify user credentials programmatically, with a focus on best practices.
String username = "correctUser";
String password = "correctPassword";
try (Connection con = DriverManager.getConnection(url, username, password)) {
if (con != null) {
System.out.println("Valid credentials!");
}
} catch (SQLException ex) {
if (ex.getErrorCode() == 1045) {
System.out.println("Invalid user credentials, please check your username and password.");
} else {
System.out.println("Connection failure: " + ex.getMessage());
}
}
By checking for specific error codes, you can tailor user feedback, enhancing the debugging process.
Step 4: Confirm MySQL is Running
Before attempting to connect, ensure that MySQL is running. You can do this by accessing the EasyPHP dashboard and checking the MySQL service. You might also want to connect through a MySQL client to validate connectivity outside of your Java application.
Step 5: Firewall Configuration
Make sure that no firewall is blocking the connection. Sometimes, local firewalls may obstruct access to MySQL. You can test this by temporarily disabling the firewall to see if the connection succeeds.
Step 6: Review MySQL Settings
MySQL connections can be influenced by various settings such as:
- The maximum number of connections.
- Permissions granted to specific users.
Review the MySQL configuration file (my.cnf
or my.ini
) to confirm that your configurations are correctly set.
Step 7: Handling Exceptions
Properly handling exceptions can lead to cleaner, more maintainable code. Instead of relying on generic messages, provide detailed context around what went wrong.
try {
// connection code...
} catch (SQLException ex) {
switch (ex.getErrorCode()) {
case 1045:
System.out.println("Access denied for user.");
break;
case 1049:
System.out.println("Unknown database.");
break;
default:
System.out.println("SQL Error: " + ex.getMessage());
break;
}
}
Additional Resources
For more insights on database connectivity issues, you might want to check out "Troubleshooting MySQL Connection Issues in EasyPHP" at tech-snags.com/articles/troubleshooting-mysql-connection-issues-easyphp. This article dives deeper into debugging connectivity problems specific to EasyPHP setups.
Key Takeaways
Establishing a connection between your Java application and MySQL using EasyPHP may come with its challenges. By systematically checking the JDBC URL, verifying credentials, ensuring that the required drivers are in place, and configuring your firewall settings appropriately, you can enhance your chances of a successful connection.
Don't forget to handle exceptions gracefully for better debugging. With the right approach, you can achieve a reliable connection and harness the full power of MySQL within your Java applications.