Solving Common Push Notification Challenges in Java Apps
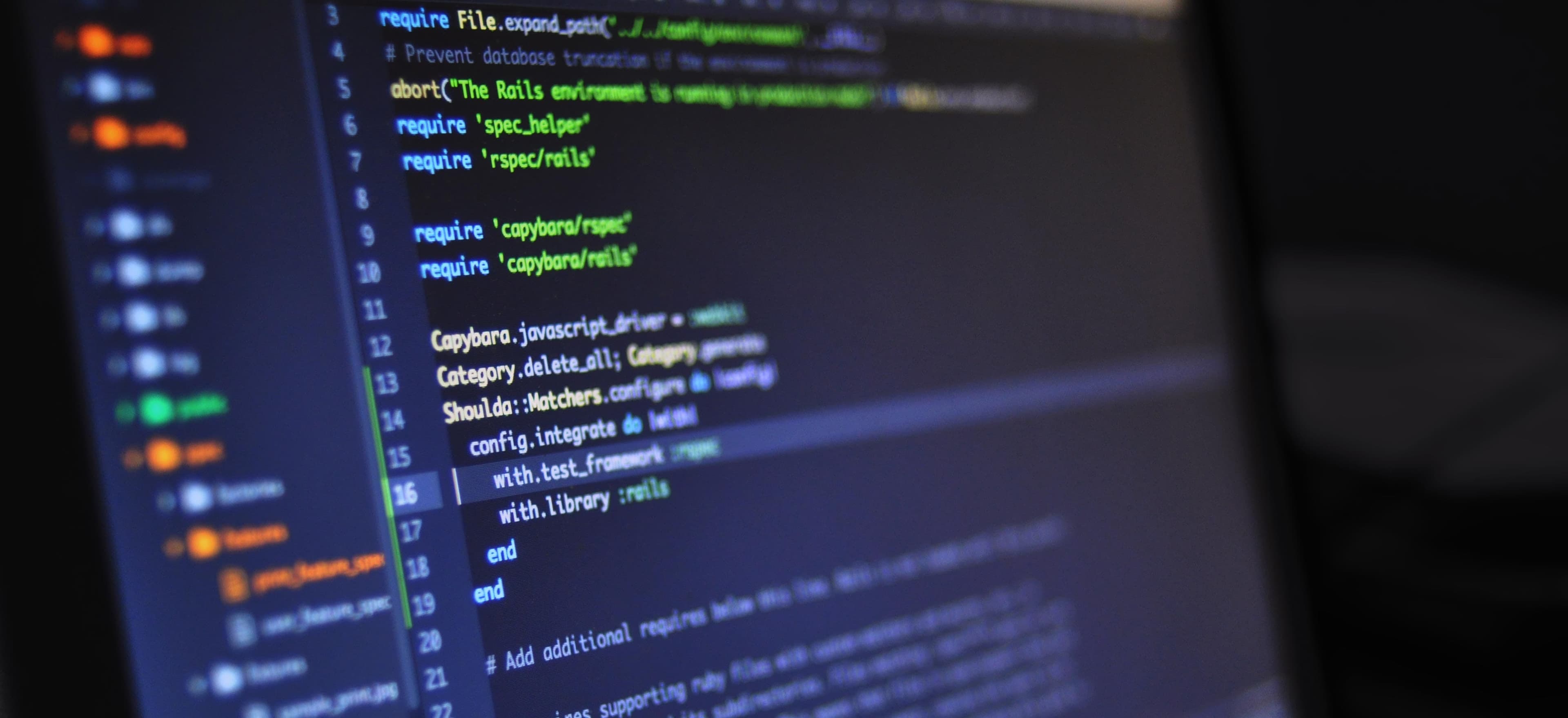
- Published on
Solving Common Push Notification Challenges in Java Apps
Push notifications are an essential component of modern app development, allowing applications to stay connected to their users. When implemented correctly, they enhance user engagement, drive user behavior, and influence overall app success. Yet, the implementation of push notifications, especially using Java, can sometimes lead to challenges. This article aims to discuss common challenges faced while setting up push notifications in Java applications and how to troubleshoot them effectively.
What Are Push Notifications?
Push notifications are messages sent by an application to engage users. They can appear in the notification center on a device, even if the app isn’t open. This functionality is crucial for sending real-time updates, alerts, and reminders to users. Implementing push notifications in a Java application typically involves integrating with services like Firebase Cloud Messaging (FCM) or Amazon SNS.
Understanding the Architecture of Push Notifications
Before diving into the challenges and solutions, it is crucial to understand the architecture of push notifications:
-
Client Application: This is where the user receives the notification. It includes the necessary SDK to handle incoming notifications.
-
Push Notification Service: This third-party service, such as FCM, sends notifications to client apps. Users need to register their devices to receive notifications.
-
Backend Server: This is usually written in Java (or another language) and communicates with the notification service to send push messages based on specific events or triggers within your application.
Understanding this architecture clarifies where problems are likely to occur, enabling targeted troubleshooting.
Common Challenges in Push Notification Setup
1. Registration Errors
Challenge: Clients sometimes fail to register with the push notification service, which means they will not receive any notifications.
Solution: Make sure the client app correctly initializes the push notification service with proper parameters. Here’s an example code snippet for registering an Android device using FCM.
FirebaseMessaging.getInstance().getToken()
.addOnCompleteListener(task -> {
if (!task.isSuccessful()) {
Log.w(TAG, "Fetching FCM registration token failed", task.getException());
return;
}
// Get new FCM registration token
String token = task.getResult();
Log.d(TAG, "Token: " + token);
// Send token to backend server
sendTokenToServer(token);
});
Why: This snippet captures the FCM token and handles registration errors. Logging the token is vital for troubleshooting token generation and can indicate network issues affecting registration.
2. Notification Delivery Failure
Challenge: Notifications don't get delivered to the intended recipient.
Solution: This can be due to a range of issues including expired tokens, device unavailability, or misconfigured notification payloads. The following code shows how to send a notification using HTTP POST.
HttpUrl url = HttpUrl.parse("https://fcm.googleapis.com/fcm/send");
RequestBody body = new FormBody.Builder()
.add("to", registrationToken) // device token
.add("title", "Hello")
.add("body", "Notification via Java code")
.build();
Request request = new Request.Builder()
.url(url)
.post(body)
.addHeader("Authorization", "key=" + SERVER_KEY)
.build();
try (Response response = client.newCall(request).execute()) {
Log.d(TAG, "Notification response: " + response.body().string());
}
Why: This straightforward HTTP POST request example shows how to correctly structure notification data. Proper headers, especially authorization keys, are vital as they prevent rejection from the push notification service.
3. Handling Different Platforms
Challenge: A single solution may not work across different platforms (iOS, Android, Web).
Solution: Utilizing platform-specific SDKs is crucial. You must also handle device-specific configuration. For example, Java-based backend applications can manage tokens and send requests to different services depending on the user's device type.
Here’s a generic controller in Java you might use for handling webhooks:
@PostMapping("/sendNotification")
public ResponseEntity<Void> sendNotification(@RequestBody NotificationRequest request) {
// Determine the target platform
if (request.getPlatform() == Platform.ANDROID) {
// Call method to send notification to Android
} else if (request.getPlatform() == Platform.IOS) {
// Call method to send notification to iOS
}
return ResponseEntity.ok().build();
}
Why: This piece of code showcases how to manage notifications by checking the target platform. This is vital to ensure you're not sending incompatible payloads to different devices.
Troubleshooting Techniques
-
Log Everything: Always log request and response payloads. This logging can greatly assist in pinpointing issues with user registration or message delivery.
-
Payload Validation: Ensure that the payload follows the necessary structure as outlined in the documentation for the service you are using.
-
Status Codes: Pay close attention to status codes returned when sending notifications. Codes like 404 may indicate an invalid registration token, while 400 could point to a bad request.
-
Testing Setup: Use tools like Postman to test your notification endpoint. This isolates issues by allowing you to determine if the problem lies within the client or server.
For a deeper dive into troubleshooting push notifications specifically in React Native, check out Troubleshooting Push Notification Setup in React Native.
Best Practices for Push Notifications
- User Consent: Always get user permission before sending push notifications.
- Personalization: Tailor notifications to enhance user engagement. Users are more likely to respond positively to personalized notifications relevant to their interests.
- Timeliness: Send notifications at times when users are most likely to engage. This improves the efficacy of your push notifications.
To Wrap Things Up
Push notifications can seem daunting, but understanding common challenges and how to troubleshoot them significantly simplifies the process. A solid implementation in Java not only enhances user engagement but also ensures reliable communication with your app’s audience. By adhering to coding best practices, leveraging log data, and focusing on responsive design across platforms, you can successfully navigate the stormy seas of push notifications.
Remember, testing and iterating your implementation is key to maximizing effectiveness. Push notifications are more than just a feature; they are an avenue for growth and user retention. Ready to implement? Let your push notifications take flight!
Did you find this article helpful? For practical guidance on push notification setups, consider diving into the Troubleshooting Push Notification Setup in React Native for additional insights!
Checkout our other articles