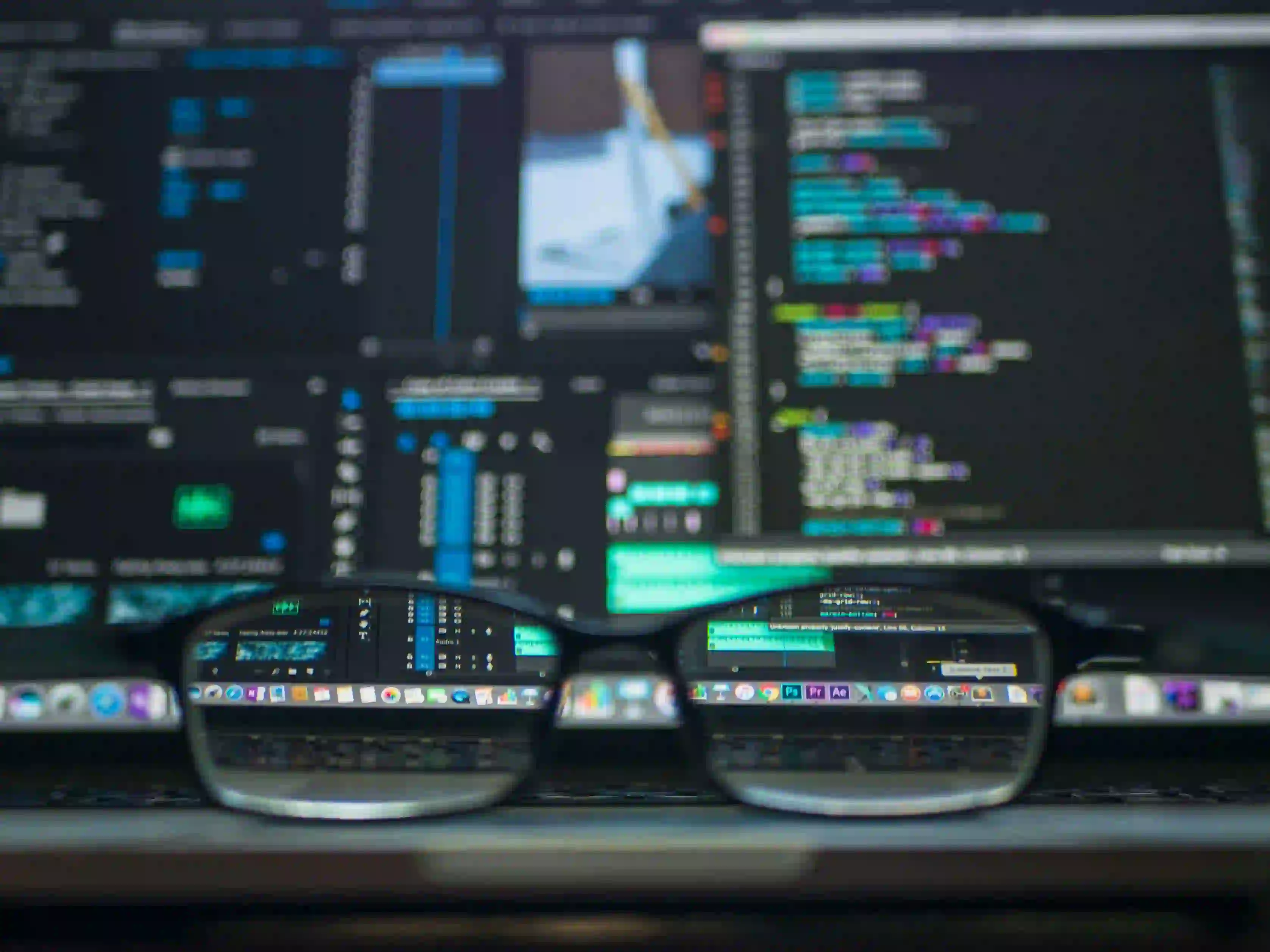
Enhancing Java App Layouts with Feng Shui Principles
In the world of software development, aesthetics play a crucial role in user experience. Just as practitioners of Feng Shui design spaces to promote harmony, developers can enhance application layouts to create more user-friendly interfaces. This blog post explores how integrating Feng Shui principles into Java application layouts can greatly improve usability and engagement. Let's dive into this engaging approach while featuring some valuable Java coding practices.
Understanding Feng Shui
Feng Shui is an ancient Chinese practice focused on the arrangement of space to foster positive energy flow. The principles of Feng Shui may seem far removed from coding, but they emphasize balance, organization, and a mindful approach to space usage. Applying these concepts to Java application layouts can vastly improve how users interact with your app.
Integrating Feng Shui into Java App Layouts
When designing your Java application's user interface (UI), consider these core Feng Shui principles:
- Balance and Harmony
- Flow of Energy (Chi)
- Clutter-Free Environment
- Appropriate Use of Colors
Now let's explore how to implement these principles through practical Java coding techniques.
Step 1: Balance and Harmony in Layouts
In Java, the Swing and JavaFX libraries allow developers to create a variety of layouts. A balanced layout maintains visual interest while ensuring that elements are not overcrowded.
Here is an example of a balanced layout using GridBagLayout in Swing:
import javax.swing.*;
import java.awt.*;
public class BalancedLayout {
public static void main(String[] args) {
JFrame frame = new JFrame("Balanced Layout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints constraints = new GridBagConstraints();
// Add first component
constraints.gridx = 0;
constraints.gridy = 0;
constraints.insets = new Insets(10, 10, 10, 10);
panel.add(new JButton("Button 1"), constraints);
// Add second component
constraints.gridx = 1;
constraints.gridy = 0;
panel.add(new JButton("Button 2"), constraints);
// Add third component
constraints.gridx = 0;
constraints.gridy = 1;
constraints.gridwidth = 2; // Span both columns
panel.add(new JLabel("Balanced Label"), constraints);
frame.add(panel);
frame.setVisible(true);
}
}
Commentary
In this code:
- GridBagLayout is chosen for its flexibility.
- Components are added with GridBagConstraints to achieve balance—ensuring that elements do not overlap while also showcasing each part efficiently.
Using this layout method promotes visual appearance and effectiveness, channeling the principle of balance in Feng Shui.
Step 2: Promote the Flow of Energy (Chi)
In Feng Shui, the flow of energy is paramount. Similarly, the navigation and interaction within an application need to be seamless. Leveraging layout managers effectively can facilitate this flow.
Consider using BoxLayout in this example:
import javax.swing.*;
import java.awt.*;
public class FlowLayoutDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Flow Layout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS)); // Vertical flow
panel.add(new JButton("Start"));
panel.add(new JButton("Options"));
panel.add(new JButton("Exit"));
frame.add(panel);
frame.setVisible(true);
}
}
Commentary
Here, BoxLayout is employed to arrange buttons in a vertical stack. This layout encourages users to navigate effortlessly from one option to the next. Properly structuring user interactions keeps the 'Chi' of the application flowing, enhancing user satisfaction.
Step 3: Maintain a Clutter-Free Environment
Just as clutter can disrupt positive energy flow in Feng Shui, unnecessary elements in your app can lead to a confusing user experience. Use JTabbedPane to group similar functionalities within your application.
import javax.swing.*;
public class ClutterFreeDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Clutter-Free Layout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
JTabbedPane tabbedPane = new JTabbedPane();
tabbedPane.addTab("Home", new JLabel("Welcome to Home"));
tabbedPane.addTab("Settings", new JLabel("Adjust your preferences"));
tabbedPane.addTab("Help", new JLabel("Help and support options"));
frame.add(tabbedPane);
frame.setVisible(true);
}
}
Commentary
By using JTabbedPane, we distribute content logically across tabs, minimizing cognitive load. This aligns with Feng Shui's vision of creating accessible and open environments. Keeping UI elements well-organized fosters better user engagement.
Step 4: Engage with Appropriate Color Usage
Color can be a crucial element in design and Feng Shui. Each hue affects mood differently. In Java applications, you can alter component colors to create visual interest and evoke certain feelings.
Here's how to adjust button colors based on the thematic presence you want to project:
import javax.swing.*;
import java.awt.*;
public class ColorDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Color Usage Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
JButton sunnyButton = new JButton("Sunny Yellow");
sunnyButton.setBackground(Color.YELLOW);
JButton calmButton = new JButton("Calm Blue");
calmButton.setBackground(Color.BLUE);
JPanel panel = new JPanel();
panel.add(sunnyButton);
panel.add(calmButton);
frame.add(panel);
frame.setVisible(true);
}
}
Commentary
In the above code:
- The setBackground() method is used to apply colors to buttons.
- The use of yellow promotes energy and positivity, while blue induces calmness.
By mindfully choosing colors, you guide the emotional experience users have interacting with your application.
Lessons Learned
Integrating Feng Shui principles into Java app layouts is an innovative way to craft user-friendly environments that enhance engagement. You can promote balance, ensure the flow of energy, maintain organization, and choose colors wisely while coding.
Remember, as you develop your application, think of the user experience as an ecosystem where clarity and positive interaction are paramount. For further insights into the harmony of space and functionality, consider reading our article titled Feng Shui im Studio: Harmonie im kleinen Raum.
By bringing these principles into your Java applications, you’re not just creating software – you’re building experiences that resonate positively with users. Happy coding!