Handling Validation in Java Frameworks: Common Pitfalls
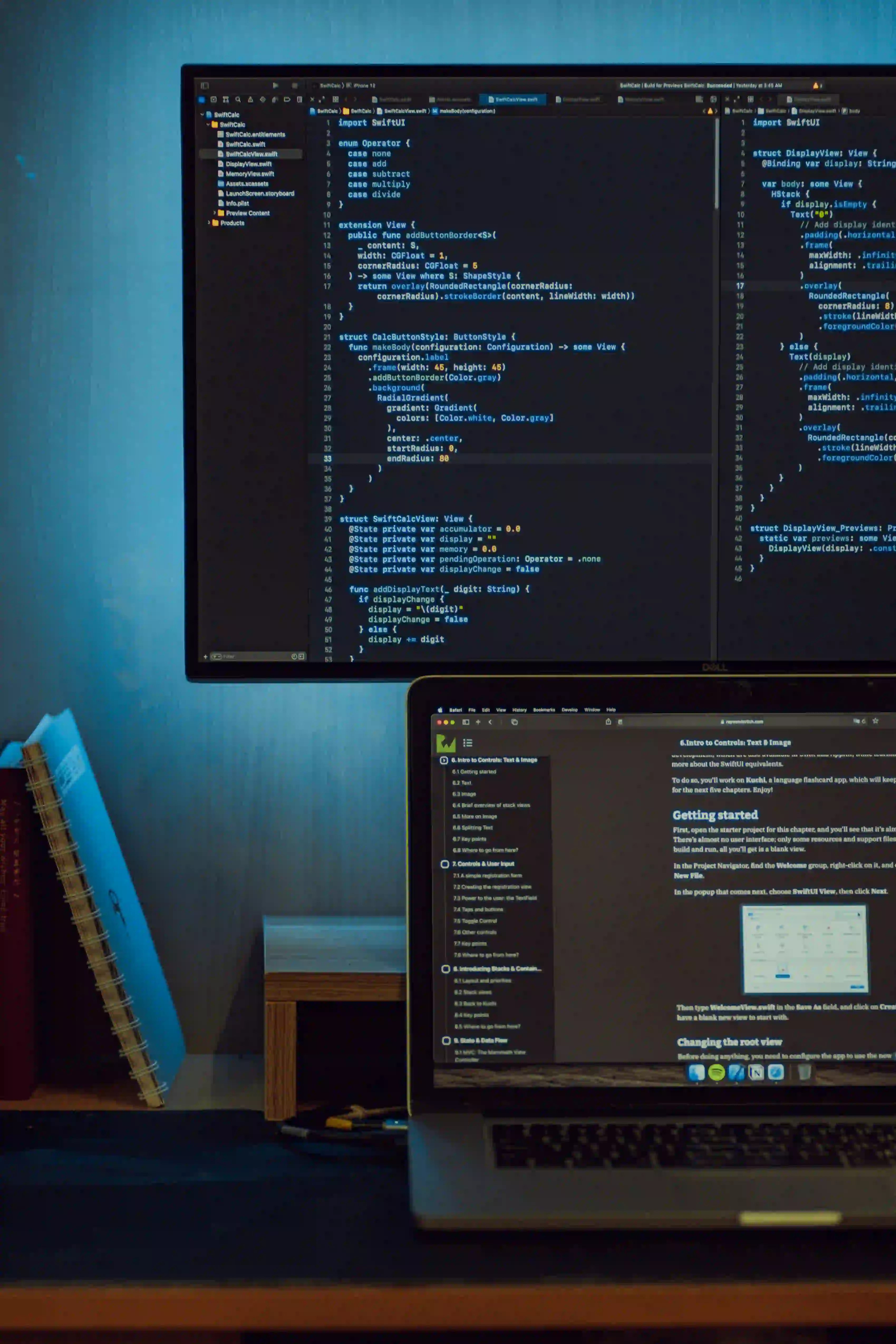
Handling Validation in Java Frameworks: Common Pitfalls
Validation is an essential aspect of building robust applications. It ensures that the data processed by your application meets expected standards and formats. While various Java frameworks facilitate validation, there are common pitfalls developers often encounter. This blog post will explore these pitfalls and provide insights on how to effectively handle validation in Java frameworks.
Understanding Validation in Java
Before diving into the common pitfalls, let's discuss what validation means in the Java ecosystem. At its core, validation is about enforcing rules on data inputs to ensure quality and integrity before performing operations such as storing in a database or processing further. Whether working in Spring, JSF, or any other Java framework, validation remains a critical concern.
Why is Validation Important?
- Data Integrity: Protects against corrupt or malicious data.
- User Experience: Provides immediate feedback to users on their inputs.
- Security: Prevents vulnerabilities such as SQL Injection and Cross-Site Scripting (XSS).
- Maintains Business Logic: Ensures that business rules are implemented consistently throughout the application.
Common Validation Pitfalls
1. Ignoring Framework Features
Many Java frameworks come equipped with built-in validation features that are simple yet powerful. For instance, if you are using Spring, you can leverage the @Valid
annotation alongside JSR-303 (Bean Validation).
Example Code
import javax.validation.constraints.NotBlank;
public class User {
@NotBlank(message = "Name must not be empty")
private String name;
// Constructors, getters, and setters
}
Why This Matters: By using annotations, you can reduce boilerplate code and focus on core functionalities. This way, you ensure that validation is consistently applied without additional logic scattered around your application.
2. Over-Validation
While it might seem beneficial to validate every possible input, over-validation can clutter your code and lead to poor maintainability. Excessive checks may also hinder user experience with unnecessary errors.
Example Scenario
Imagine a scenario where you are validating every character input in a username field. While it's crucial to check for certain criteria, validating against all characters individually adds complexity without improving security or data integrity.
Recommendation: Set reasonable rules for input validation and ensure they align with business requirements. Focus on key patterns rather than every edge case.
3. Not Handling Validation Errors Gracefully
Failing to provide users with clear, actionable feedback on input errors can frustrate them. When validation fails, the response should be informative and user-friendly.
Example Code
Here's an example using Spring MVC to handle validation errors:
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.ui.Model;
@Controller
public class UserController {
@PostMapping("/register")
public String registerUser(@ModelAttribute @Valid User user, BindingResult bindingResult, Model model) {
if(bindingResult.hasErrors()) {
model.addAttribute("errors", bindingResult.getAllErrors());
return "registration"; // Show the registration page again with errors
}
// Process the user registration
return "success";
}
}
Why This Matters: Providing clear feedback allows users to easily rectify their mistakes. It also improves overall user satisfaction and trust in your application.
4. Failing to Sanitize Input
Validation goes hand-in-hand with sanitization. Even after validating inputs, developers might forget to sanitize them properly, exposing applications to security vulnerabilities.
What to Remember:
- Always validate inputs.
- Sanitize before processing any user data.
5. Inconsistent Validation Logic
In large applications, different developers might implement validation in varied manners, leading to inconsistencies. This can result in a poor user experience and potential data integrity issues.
Recommendation: A centralized validation strategy can be useful. You can create a validation service or utility classes that all components can utilize.
6. Neglecting Client-Side Validation
While server-side validation is crucial, neglecting client-side validation can lead to performance issues. Users have to wait for a round trip to the server to identify simple mistakes, which can disrupt the experience.
Example Code: Client-Side Validation with JavaScript
<input type="text" id="username" name="username" required>
<span id="error-message"></span>
<script>
document.getElementById('username').addEventListener('input', function() {
const input = this.value;
if (input.length < 3) {
document.getElementById('error-message').textContent = "Username must be at least 3 characters.";
} else {
document.getElementById('error-message').textContent = "";
}
});
</script>
Why is This Important?: Implementing client-side validation helps catch errors before data is sent to the server, enhancing performance and user experience.
7. Bypassing Validation in Development
In a fast-paced development environment, it's tempting to bypass validation during local testing. This practice can lead to larger issues when the application goes live.
What to Do: Always adhere to validation rules during development. Consider enforcing validations in different environments, ensuring consistent application behavior.
8. Lack of Testing
Lastly, a major pitfall is failing to include validation in unit and integration tests. If validation logic is not checked through tests, it opens the door for bugs and unforeseen issues.
Example of a Test Case with JUnit
import org.junit.jupiter.api.Test;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import static org.junit.jupiter.api.Assertions.*;
public class UserValidationTest {
private final Validator validator;
public UserValidationTest() {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
this.validator = factory.getValidator();
}
@Test
public void testUserNameNotEmpty() {
User user = new User();
user.setName(""); // This will violate the @NotBlank constraint
assertFalse(validator.validate(user).isEmpty());
}
}
Why Testing is Crucial: Regular testing helps ensure that validation work is not only effective but also maintains over time. It gives you confidence that your application behaves as expected during various conditions.
Wrapping Up
Java frameworks offer a rich set of features for validating input data. However, it is easy to stumble into common pitfalls if one’s not careful. From ignoring framework features to ensuring proper testing of validation logic, being aware of these challenges can lead to more robust applications.
For a broader perspective on validation, especially in the context of plugins and WordPress, you might find the article on Common Validation Issues in WordPress Plugins and Fixes useful. You can read it at tech-snags.com/articles/common-validation-issues-wordpress-plugins-fixes.
In summary, always prioritize validation within your Java applications; not only does it safeguard against errors and vulnerabilities, but it also contributes significantly to an excellent user experience.