Resolving Data Validation Issues in Java Frameworks
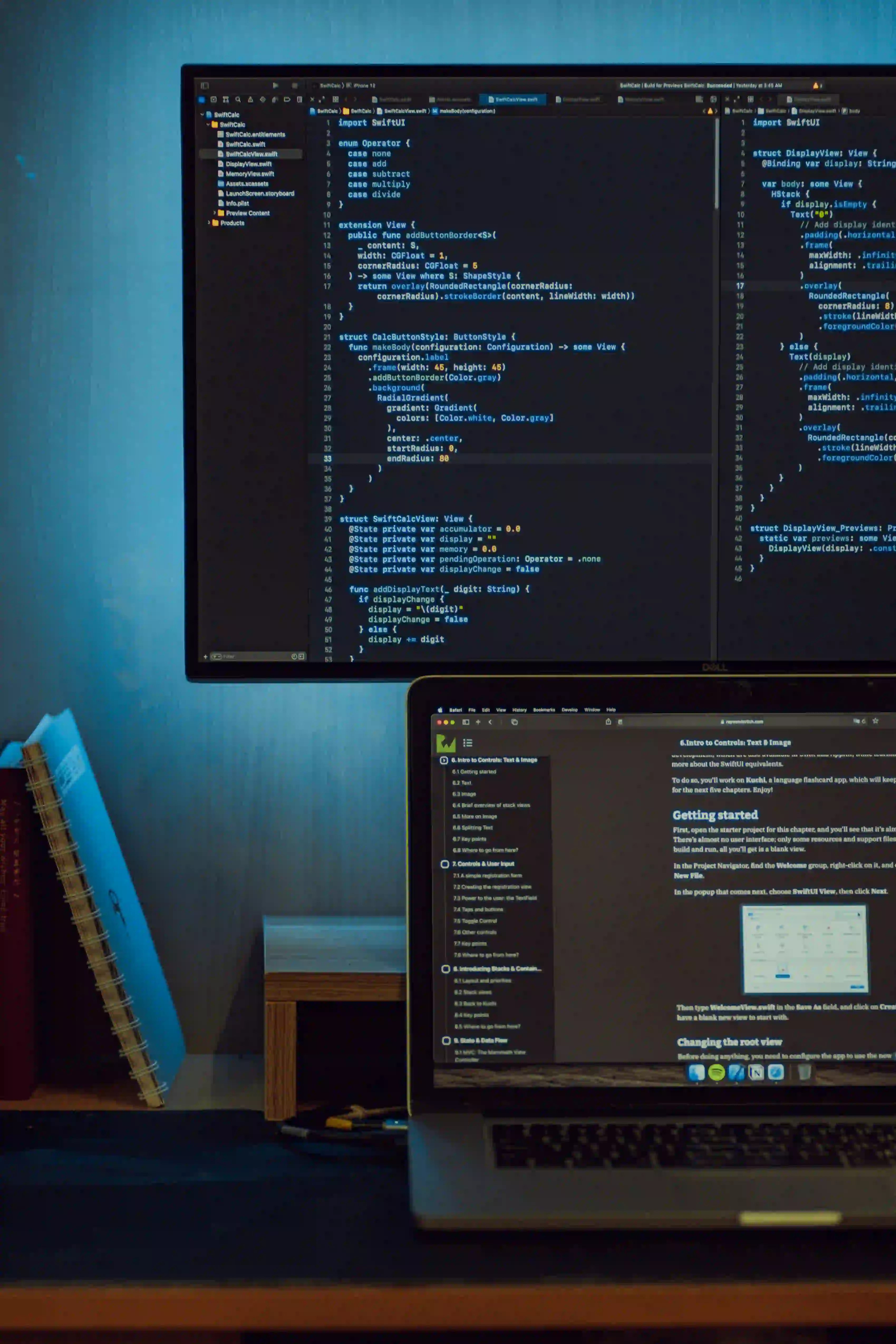
Resolving Data Validation Issues in Java Frameworks
Data validation is a critical aspect of software development that ensures data integrity and security. In Java applications, particularly when using frameworks like Spring or Java EE, it’s essential to employ robust validation mechanisms. This article will explore common data validation issues in Java frameworks, offer solutions, and provide code examples to illustrate effective practices. Additionally, we will draw parallels to challenges found in other environments, such as WordPress development, referencing the article “Common Validation Issues in WordPress Plugins and Fixes” for comparative insights.
Why Data Validation Matters
Data validation is more than just an afterthought; it acts as the first line of defense against data integrity violations and security vulnerabilities. Factors such as user input, API requests, and data consistency demand rigorous validation checks.
Key Benefits of Data Validation
- Data Integrity: Ensures that only correctly formatted and reasonable data is accepted.
- User Security: Protects against attacks such as SQL injection and cross-site scripting (XSS).
- Reduced Bugs: Prevents unexpected behaviors in the application by catching errors early.
Common Data Validation Issues
In Java applications, several types of validation issues commonly arise:
- Missing Validation Logic
- Improper Error Handling
- Inconsistent Validation Rules
- Overly Complex Validation Logic
Let us delve deeper into each of these issues and discuss exemplary code snippets.
1. Missing Validation Logic
Failing to implement validation logic can lead to the entry of corrupted or harmful data into the application.
Solution: Use annotations provided by Java validation frameworks.
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Email;
public class User {
@NotBlank(message = "Username is mandatory")
private String username;
@Email(message = "Email should be valid")
private String email;
// Getters and setters
}
Why: The @NotBlank
and @Email
annotations serve to ensure that user attributes are validated against specific rules, making the code more readable and maintainable.
2. Improper Error Handling
When validation errors occur, it is crucial to report them accurately to the user. Poor error messaging can lead to confusion.
Solution: Implement a standard method for handling validation exceptions.
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import java.util.HashMap;
import java.util.Map;
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<Map<String, String>> handleValidationExceptions(MethodArgumentNotValidException ex) {
Map<String, String> errors = new HashMap<>();
ex.getBindingResult().getFieldErrors().forEach(error ->
errors.put(error.getField(), error.getDefaultMessage()));
return new ResponseEntity<>(errors, HttpStatus.BAD_REQUEST);
}
}
Why: This centralized exception handling ensures that all validation errors are processed similarly, providing clear messages to users while maintaining a clean code architecture.
3. Inconsistent Validation Rules
When validation logic is spread across multiple layers, it can lead to inconsistencies, resulting in confusion and potential vulnerabilities.
Solution: Centralize validation logic using DTOs (Data Transfer Objects).
import javax.validation.Valid;
public class UserController {
@PostMapping("/users")
public ResponseEntity<User> createUser(@Valid @RequestBody User user) {
// User service logic
return ResponseEntity.ok(userService.save(user));
}
}
Why: By using DTOs and the @Valid
annotation, we ensure that all validation is handled in a single layer, minimizing the chances of inconsistencies.
4. Overly Complex Validation Logic
Validation logic can become overly complex if not designed effectively, leading to maintainability issues.
Solution: Use validation groups to segregate different validation rules.
import javax.validation.GroupSequence;
import javax.validation.Valid;
@GroupSequence({BasicChecks.class, AdvancedChecks.class})
public interface ValidationGroups {
interface BasicChecks {}
interface AdvancedChecks {}
}
public class User {
@NotBlank(groups = ValidationGroups.BasicChecks.class)
private String username;
// Other fields with various validations depending on group
}
Why: By utilizing validation groups, you simplify the validation process and uphold clarity in functionality.
The Closing Argument
Data validation in Java frameworks is indispensable for creating robust, secure applications. By addressing common issues through disciplined coding practices, you can significantly enhance user experience and application integrity.
For those interested in further expanding their understanding of validation issues across platforms, consider checking out the article “Common Validation Issues in WordPress Plugins and Fixes” at https://tech-snags.com/articles/common-validation-issues-wordpress-plugins-fixes.
References
- Java Validation Framework
- Spring Boot Validation Documentation
- Effective Java by Joshua Bloch
By implementing these best practices and considerations, Java developers will be better equipped to tackle data validation issues effectively, ensuring a smoother development process and a cleaner codebase. Happy coding!