Preventing Validation Errors in Java-Based Web Applications
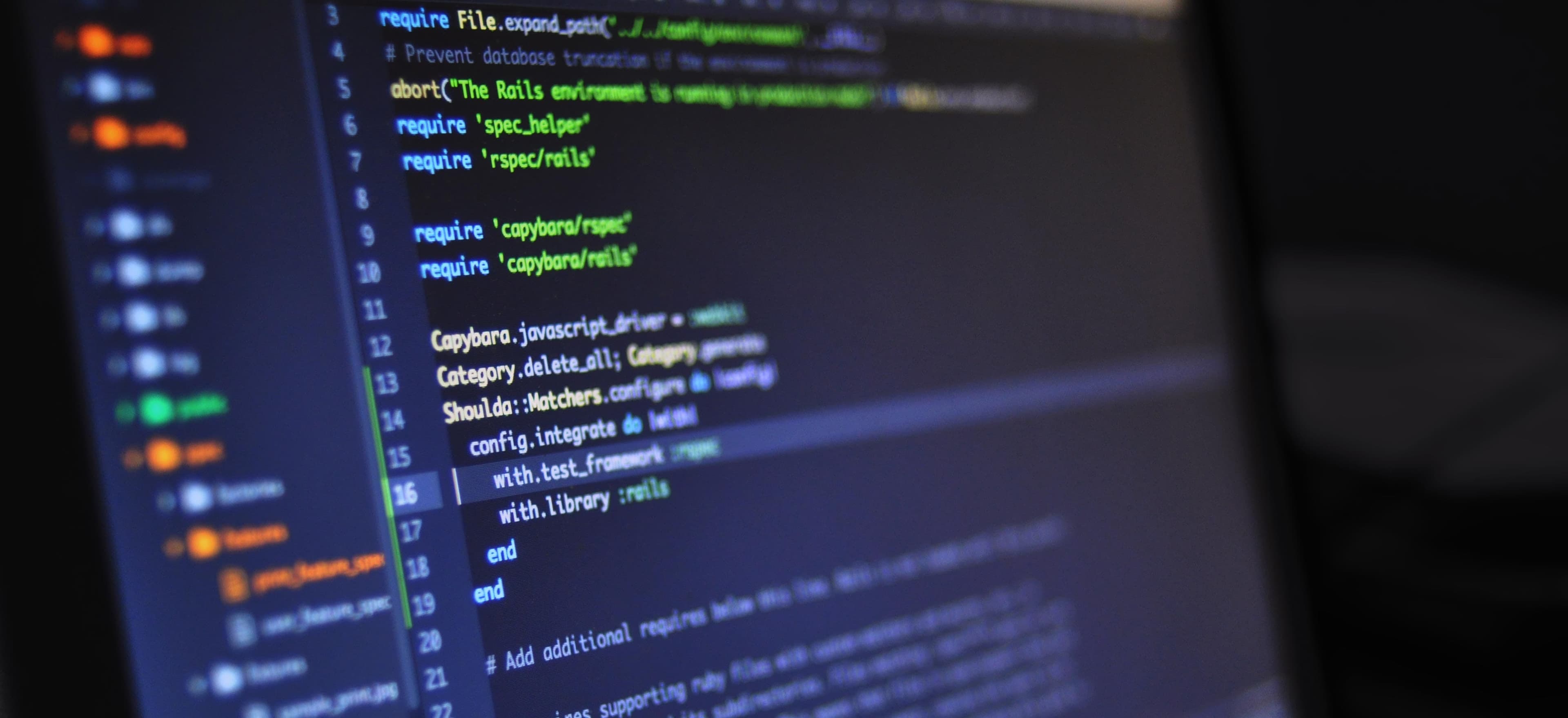
- Published on
Preventing Validation Errors in Java-Based Web Applications
Web applications have become an integral part of our daily lives, from simple portfolio sites to complex enterprise applications. Yet, one critical aspect that continues to challenge developers is the validation of user inputs. Incorrect validation can lead not only to poor user experience but also to severe security vulnerabilities and data corruption. In this blog post, we’ll explore how to effectively prevent validation errors in Java-based web applications.
Understanding Validation
Validation is the process of ensuring that the input provided by users meets the criteria set by the application. This includes checking for:
- Correct Format: Is the email correctly formatted?
- Data Type: If the input is a number, is it actually numeric?
- Range Checks: Does the age provided fall within the acceptable range?
- Mandatory Fields: Are required fields filled?
By employing proper validation techniques and frameworks, developers can ensure that their applications remain secure and user-friendly.
Common Validation Issues
Validation errors often stem from various sources, which can include:
- Client-Side Validation: Relying solely on client-side scripts for input validation.
- Lack of Server-Side Validation: Failing to validate inputs on the server-side, leading to potential security threats.
- Insufficient Error Messaging: Not providing users with clear, actionable feedback when validation fails.
For a deeper insight into similar themes, especially in plugin development, I highly recommend checking out the article “Common Validation Issues in WordPress Plugins and Fixes” at tech-snags.com/articles/common-validation-issues-wordpress-plugins-fixes.
Using Java for Validation
Java provides various tools that can help manage input validation effectively. Let's discuss some common strategies and techniques.
1. Bean Validation with Hibernate Validator
One of the most robust methods for validating user input in Java applications is to use Bean Validation. The most popular implementation of this standard is Hibernate Validator. By using annotations, you can add validation constraints directly to your data model.
Here is a simple example:
import javax.validation.constraints.*;
public class User {
@NotNull
@Size(min = 2, max = 30)
private String name;
@Email
private String email;
@Min(18)
@Max(99)
private int age;
// Getters and Setters
}
Commentary on the Example
- @NotNull: Ensures the field cannot be null.
- @Size: Validates the length of the name field to be between 2 and 30 characters.
- @Email: Uses a built-in constraint to validate the email format.
- @Min and @Max: These constraints ensure the age provided is within a defined range.
This kind of declarative validation improves code readability and maintainability.
2. Custom Validator Annotations
Sometimes, predefined annotations won’t fully meet your requirements. In such cases, you can create custom validation annotations. This involves three key steps:
- Creating the Annotation: Define your own annotation annotation.
- Implementing the Validator: Write a class that implements the
ConstraintValidator
interface to define the validation logic. - Applying the Annotation: Add your custom annotation to the model class fields.
Here’s how you can implement a custom validator:
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.*;
@Documented
@Constraint(validatedBy = AgeValidator.class)
@Target({ElementType.METHOD, ElementType.FIELD, ElementType.ANNOTATION_TYPE})
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidAge {
String message() default "Age must be between 18 and 99";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
Validator Implementation:
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
public class AgeValidator implements ConstraintValidator<ValidAge, Integer> {
@Override
public void initialize(ValidAge constraintAnnotation) {}
@Override
public boolean isValid(Integer age, ConstraintValidatorContext context) {
return age != null && age >= 18 && age <= 99;
}
}
Commentary on Custom Validator
- The
@Constraint
annotation links our custom annotation to the validator class. - The
isValid
method contains the validation logic which checks if the age falls within the defined range.
3. Validating Inputs in Servlets
While frameworks provide cleaner approaches, sometimes you still need to manage validation manually, particularly in servlet-based applications, where requests and responses must be explicitly handled.
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class UserServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
String email = request.getParameter("email");
String ageStr = request.getParameter("age");
if (name == null || name.length() < 2) {
request.setAttribute("errorMessage", "Name must be at least 2 characters");
} else if (!email.matches("^[\\w-.]+@([\\w-]+\\.)+[\\w-]{2,4}$")) {
request.setAttribute("errorMessage", "Invalid email format");
} else {
int age = Integer.parseInt(ageStr);
if (age < 18 || age > 99) {
request.setAttribute("errorMessage", "Age must be between 18 and 99");
} else {
// Process data, save to database, etc.
}
}
// Forward back to input form with error messages
request.getRequestDispatcher("/form.jsp").forward(request, response);
}
}
Commentary on Servlet Validation
- Manual input checks can lead to long if-else constructions. However, they are sometimes necessary.
- Using regex for email validation may clutter the code, but it’s a quick way to catch format issues.
Bringing It All Together
Validation is an essential part of web development, especially when dealing with user inputs. By leveraging Java’s built-in validation frameworks like Hibernate Validator, crafting custom validators according to project needs, and properly managing manual validations in servlets, developers can ensure their applications are robust and secure.
Continue learning about validation issues in software as you refine your skills to avoid pitfalls encountered in other focusing areas, such as in WordPress plugin development, referenced in “Common Validation Issues in WordPress Plugins and Fixes” at tech-snags.com/articles/common-validation-issues-wordpress-plugins-fixes.
By incorporating strong validation practices into your Java-based applications, you will significantly enhance user experience and safeguard against data integrity issues. Happy coding!
Checkout our other articles