Streamline Your Java App: Manage ConfigMaps Effectively
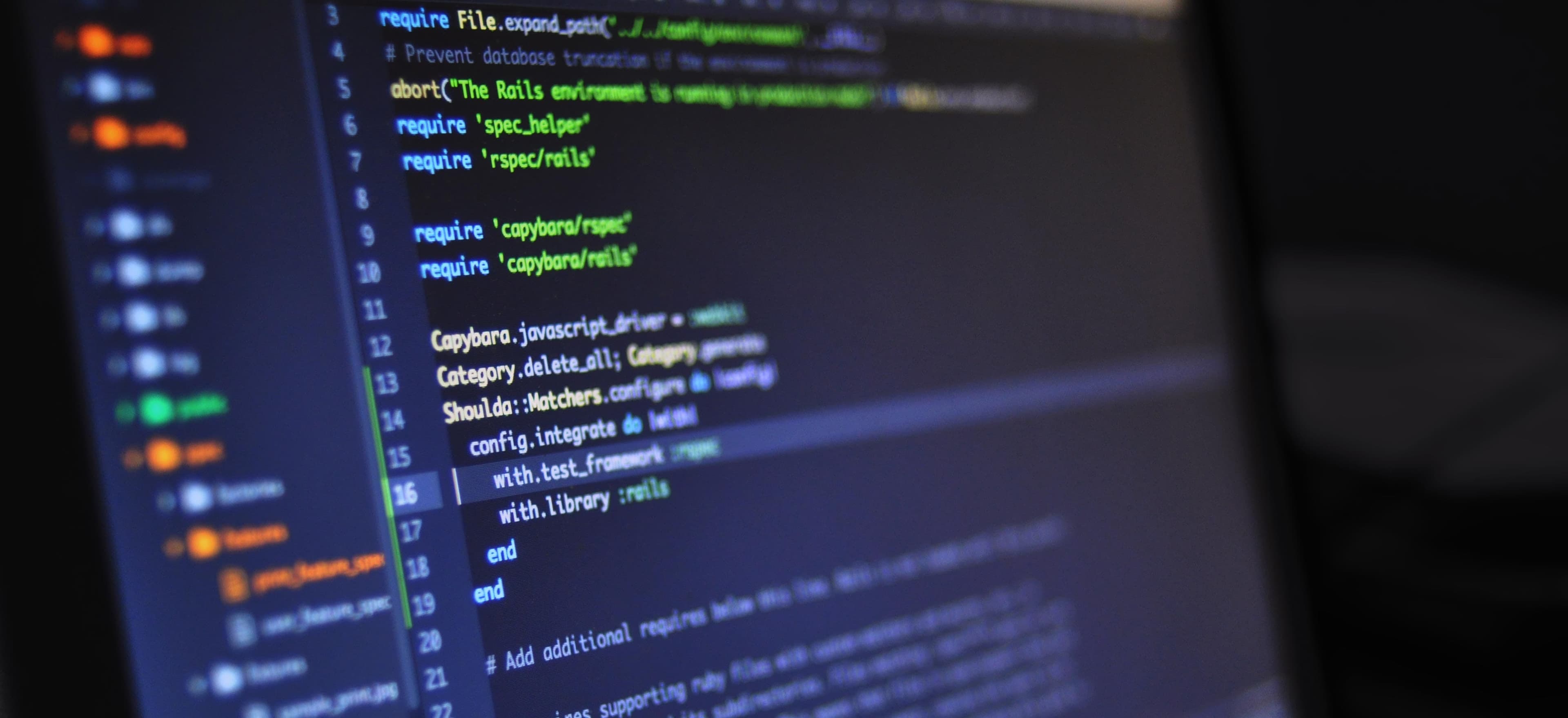
- Published on
Streamline Your Java App: Manage ConfigMaps Effectively
ConfigMaps are vital in the Kubernetes ecosystem. They provide a way to inject configuration data into your applications seamlessly. For Java applications, managing these ConfigMaps effectively can lead to enhanced performance, easier updates, and a more streamlined deployment process. In this post, we will explore how to manage ConfigMaps in your Java applications effectively and why it’s essential to eliminate orphaned ConfigMaps, referencing the existing post titled Eliminate Orphaned ConfigMaps: A Step-by-Step Guide.
Understanding ConfigMaps
ConfigMaps allow you to decouple configuration artifacts from image content. This feature enables your applications to be more portable. Imagine having an application that requires different configurations for development, testing, and production environments. Managing these configurations using ConfigMaps allows for convenient updates without the need to rebuild or redeploy your application.
Why Use ConfigMaps?
- Separation of Concerns: Keeps configuration data separate from the application code.
- Dynamic Changes: Allows you to update configuration without redeploying your app.
- Environment-Specific Configurations: Easily manage multiple configurations based on environment variables.
Managing ConfigMaps in Your Java Application
Step 1: Creating a ConfigMap
You can create a ConfigMap using a YAML file or directly from the command line. Here’s a simple example using YAML:
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
database-url: jdbc:mysql://localhost:3306/mydb
api-key: my-secret-api-key
To create this ConfigMap, save the above content in a file named configmap.yaml
, and use the following command:
kubectl apply -f configmap.yaml
Step 2: Mounting the ConfigMap in Your Java Application
Once your ConfigMap is created, you need to make this configuration accessible to your Java application. You can do this by referencing it in your deployment YAML file.
apiVersion: apps/v1
kind: Deployment
metadata:
name: java-app
spec:
replicas: 1
template:
metadata:
labels:
app: java-app
spec:
containers:
- name: java-app
image: your-java-app-image:latest
env:
- name: DATABASE_URL
valueFrom:
configMapKeyRef:
name: app-config
key: database-url
- name: API_KEY
valueFrom:
configMapKeyRef:
name: app-config
key: api-key
Commentary on the Code
In this snippet, we are referencing keys from the ConfigMap app-config
in the environment variables of our Java application. The valueFrom
field allows our application to access database-url
and api-key
as environment variables DATABASE_URL
and API_KEY
. This enhances the maintainability of the application by decoupling configuration settings from code.
Step 3: Accessing ConfigMap Values in Java
Now that we have mounted our ConfigMap in the Pod, we can access these environment variables in our Java code. Here’s how we can retrieve those values:
public class Configuration {
private String databaseUrl;
private String apiKey;
public Configuration() {
this.databaseUrl = System.getenv("DATABASE_URL");
this.apiKey = System.getenv("API_KEY");
}
public void printConfig() {
System.out.println("Database URL: " + databaseUrl);
System.out.println("API Key: " + apiKey);
}
}
Commentary on the Code
Using System.getenv()
allows us to read environment variables set in the Pod. This method is effective in a Kubernetes environment since the variables are injected at runtime. This practice ensures that sensitive information is not hard-coded into your application, thus enhancing security.
Cleaning Up Orphaned ConfigMaps
Once you've implemented and effectively managed your ConfigMaps, you might encounter orphaned ConfigMaps that no longer serve any purpose, potentially cluttering your Kubernetes namespace. Orphaned ConfigMaps can lead to confusion and may even contain outdated information that could cause problems if accidentally used.
What Are Orphaned ConfigMaps?
Orphaned ConfigMaps are configurations that are no longer referenced by any Pods or deployments. They take up space in your cluster and can lead to unnecessary complexity in deployment and management.
To mitigate this, refer to the comprehensive guide titled Eliminate Orphaned ConfigMaps: A Step-by-Step Guide, which covers steps to identify and delete such ConfigMaps efficiently. Regular maintenance helps maintain a clean and efficient Kubernetes environment, ensuring your Java applications run optimally.
Updating ConfigMaps
Whenever a change in configuration is needed, updating a ConfigMap is straightforward. Here's how you can update your existing ConfigMap:
kubectl edit configmap app-config
Alternatively, you can apply changes through a modified YAML file again:
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
database-url: jdbc:mysql://localhost:3306/mydb
api-key: new-secret-api-key # updated key
Once edited, apply the file:
kubectl apply -f configmap.yaml
Handling Updates in Your Java Application
When the ConfigMap is updated, the changes are reflected in the environment variables only after the Pod restarts. An important aspect to consider is how the application handles such restart events. Make sure your application is designed to gracefully restart or reload configurations without causing downtime.
In Conclusion, Here is What Matters
Managing ConfigMaps effectively is crucial for Java applications deployed in Kubernetes. By leveraging ConfigMaps, developers can handle environment-specific configurations seamlessly, ensuring builds remain consistent across different stages. Additionally, maintaining a clean environment by removing orphaned ConfigMaps is an essential best practice for resource management.
Continuing to explore tools and strategies to manage your Kubernetes environment can lead to increased efficiency and productivity in your development lifecycle. For more insights on maintaining clean and efficient configurations, consult the Eliminate Orphaned ConfigMaps: A Step-by-Step Guide. Happy coding!
Checkout our other articles