Preventing Security Flaws in Java Web Applications
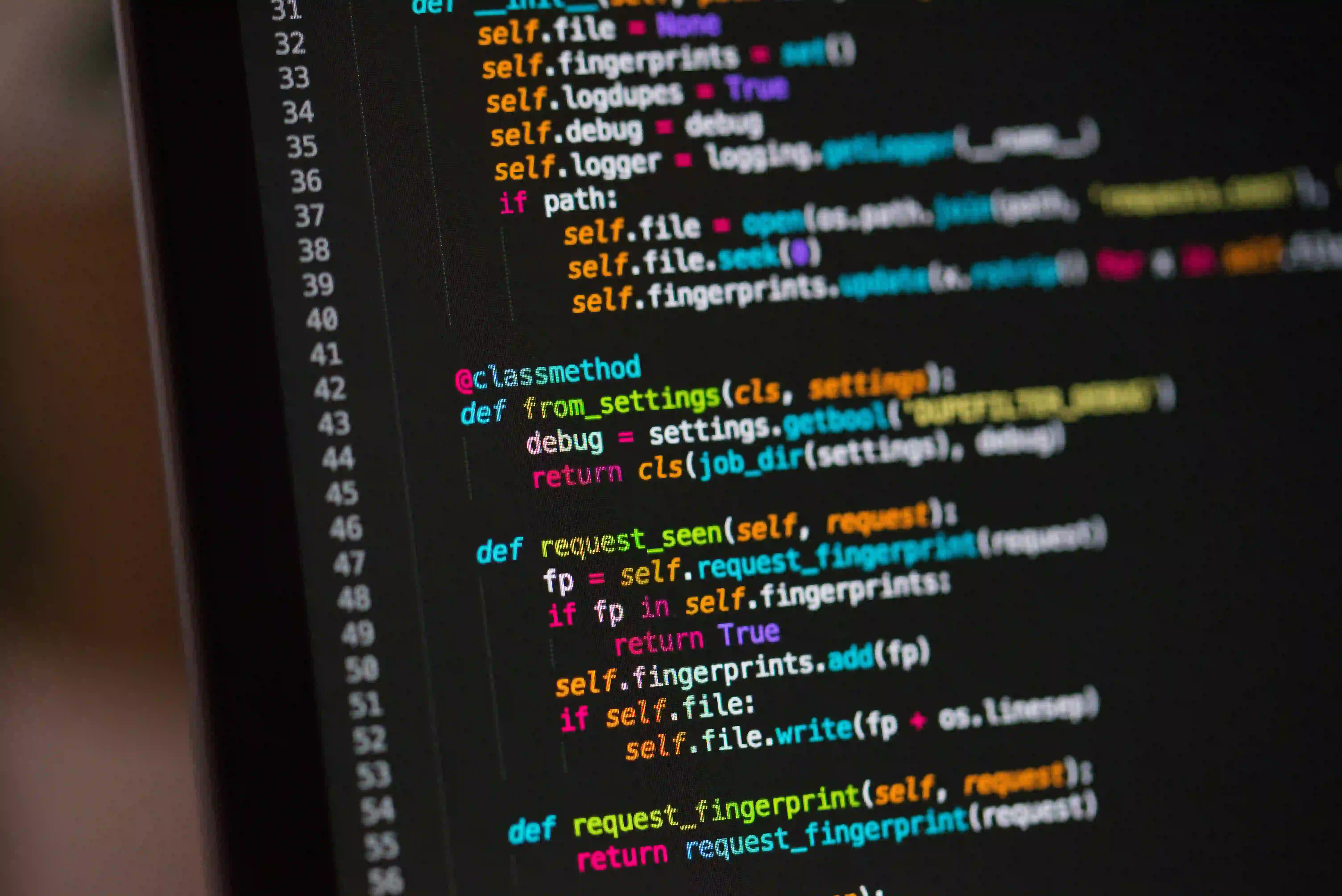
Preventing Security Flaws in Java Web Applications
Web applications have become an integral part of our daily lives. Whether we're handling sensitive information, banking online, or simply communicating through social channels, security should always be a priority in web development. Java, a popular programming language used for building robust web applications, offers numerous tools and libraries for security. However, developers must remain vigilant about potential security flaws. This post delves into best practices for preventing security flaws in Java web applications, inspired by the common vulnerabilities discussed in the article Top 5 Overlooked Security Flaws in Web Apps 2021.
Understanding Common Security Flaws
Before we dive into prevention strategies, it's essential to recognize common security flaws that can affect Java web applications:
- Injection Attacks: Such as SQL injection, where an attacker manipulates a query to gain unauthorized access.
- Cross-Site Scripting (XSS): Where malicious scripts are injected into trusted websites.
- Insecure Deserialization: When untrusted data is used to create Java objects, potentially leading to remote code execution.
- Security Misconfiguration: Default settings that are insecure or unpatched components.
- Sensitive Data Exposure: Failures to protect sensitive information like passwords and credit card details.
By understanding these flaws, we can harness preventive measures to safeguard our applications.
Best Practices for Preventing Security Flaws
1. Use Prepared Statements
SQL injections are one of the most prevalent security risks. Using prepared statements is a fundamental way to prevent these types of attacks.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class SecureDatabaseAccess {
public User getUserById(int userId) {
String query = "SELECT * FROM users WHERE id = ?";
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
PreparedStatement preparedStatement = connection.prepareStatement(query)) {
// Set the parameter to prevent SQL injection
preparedStatement.setInt(1, userId);
ResultSet resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
return new User(resultSet.getInt("id"), resultSet.getString("name"), resultSet.getString("email"));
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
Why it works: Prepared statements segregate SQL code from user input, ensuring that any input is treated simply as data rather than executable code.
2. Implement Input Validation
Always validate user inputs to mitigate XSS and other injection vulnerabilities. This can be done through sanitization.
import org.apache.commons.text.StringEscapeUtils;
public class InputValidator {
public String sanitizeInput(String input) {
// Escape HTML to prevent XSS
return StringEscapeUtils.escapeHtml4(input);
}
}
Why it works: By escaping HTML, we prevent an attacker from injecting executable scripts that can be executed in the user's browser context.
3. Use Secure Coding Practices
Secure coding practices are vital for avoiding deserialization vulnerabilities and ensuring sound security measures.
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.Serializable;
public class SecureDeserializer {
public static Object deserializeObject(byte[] data) throws IOException, ClassNotFoundException {
try (ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(data))) {
// Custom method to restrict deserialization
Object obj = ois.readObject();
if (!(obj instanceof TrustedClass)) {
throw new IllegalArgumentException("Unauthorized deserialization attempt");
}
return obj;
}
}
}
Why it works: By checking the type of the deserialized object, we can prevent malicious code from executing through improperly handled data structures.
4. Configure Security Headers
Security headers add extra layers of security to web applications by mitigating broad types of attacks.
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class SecurityHeadersFilter implements Filter {
@Override
public void doFilter(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException {
response.setHeader("X-Content-Type-Options", "nosniff");
response.setHeader("X-XSS-Protection", "1; mode=block");
response.setHeader("Strict-Transport-Security", "max-age=31536000; includeSubDomains");
chain.doFilter(request, response);
}
}
Why it works: These headers provide instructions that help protect against content-type sniffing and cross-site scripting attacks, ultimately enhancing the security of your application.
5. Encrypt Sensitive Data
To protect sensitive data, implementing strong encryption is essential. Libraries like JCE (Java Cryptography Extension) can be an asset.
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class EncryptionUtil {
private static final String ALGORITHM = "AES";
public static byte[] encrypt(String data, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data.getBytes());
}
public static String decrypt(byte[] encryptedData, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
return new String(cipher.doFinal(encryptedData));
}
}
Why it works: By encrypting sensitive data before storing or transmitting it, we make it nearly impossible for unauthorized individuals to access the original information.
My Closing Thoughts on the Matter
Building secure Java web applications requires a proactive approach to identifying and mitigating potential vulnerabilities. Familiarizing yourself with common security flaws and adhering to best coding practices is vital. By using prepared statements, validating user input, enforcing strong coding practices, configuring security headers, and encrypting sensitive data, you can significantly reduce the risk of security breaches.
For additional insights on overlooked security flaws, remember to check out the article Top 5 Overlooked Security Flaws in Web Apps 2021. Strengthen your Java applications today and ensure that user data remains secure and private.
Implementing the tips outlined here can make all the difference. Stay informed, stay secure!