Reducing High CPU Use from JVM C1/C2 Compiler Threads
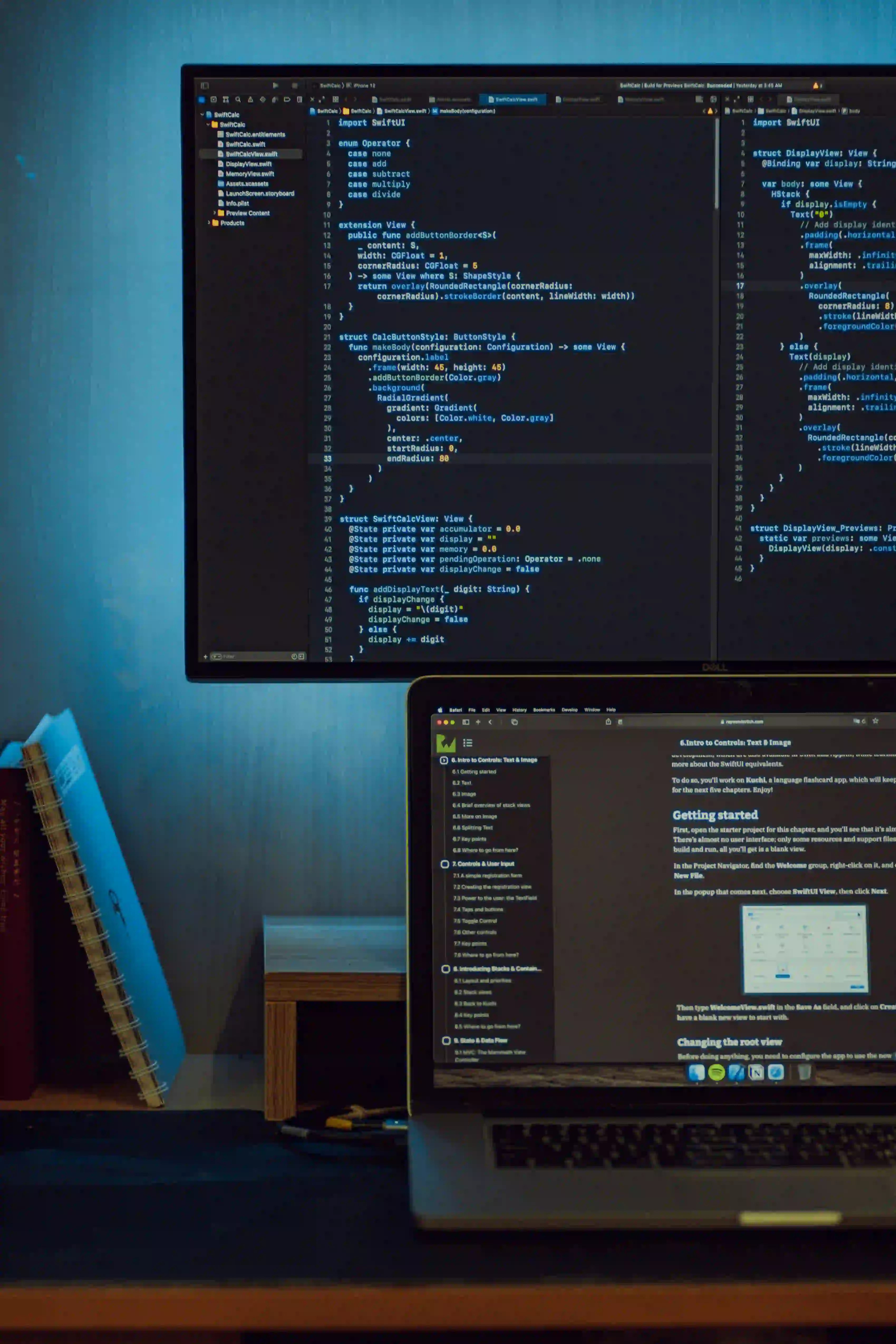
Reducing High CPU Use from JVM C1/C2 Compiler Threads
Java applications can scale remarkably well. However, high CPU usage from JVM compiler threads, particularly C1 (client) and C2 (server) compiler threads, can become a bottleneck. This post dives into understanding what causes high CPU usage in these threads and solutions to mitigate this issue.
Understanding JVM Compiler Threads
The Java Virtual Machine (JVM) includes two primary compilation modes:
- C1 (Client Compiler): Optimizes code for quick startup and is tailored for applications where low latency is critical.
- C2 (Server Compiler): Provides more extensive optimizations, resulting in faster overall execution for long-running applications.
While these compilers are essential for improving Java application performance, they can also lead to unexpectedly high CPU usage, particularly during Just-In-Time (JIT) compilation.
The JIT compilation process may feel resource-heavy due to factors such as code complexity, garbage collection (GC) activities, and the frequency of method invocations.
Symptoms of High CPU Usage
If your application experiences the following symptoms, you might be dealing with high CPU usage related to the JVM C1/C2 compilers:
- Increased response time or latency during application startup.
- High CPU percentage observed in monitoring tools dedicated to tracking health metrics.
- Outliers in performance logs indicating spikes or inconsistencies in execution times.
Diagnosing the Problem
Before applying any fixes, it is essential to diagnose the problem accurately. Here are steps you can take:
-
Enable Verbose Compilation Logging: Add the
-XX:+PrintCompilation
flag when starting your JVM instance. This will log every method that gets compiled by the JIT:🔧snippet.shjava -XX:+PrintCompilation -jar YourApplication.jar
-
Monitor CPU Usage: Utilize monitoring tools like JVisualVM or JConsole. They provide a UI that shows CPU usage, memory consumption, and thread activity, which can give insight into whether your application is spending too much time in JIT compilation.
-
Thread Dumps: Take thread dumps when you notice high CPU usage. This can be done using:
🔧snippet.shkill -3 <PID>
Analyze the dumped stack to see if many threads are in
Compiler
state.
Solutions for Reducing CPU Usage
Once you've diagnosed CPU usage issues related to the C1/C2 compiler threads, you can work on minimizing them. Below are common strategies to consider:
1. Increase Application Warm-up Time
When the JVM starts, it often spends a significant amount of CPU time compiling methods that may not be executed in the future. To minimize this impact, increase the warm-up time of your application. You can achieve this by:
- Reducing the initial work done during startup.
- Introducing a warm-up phase before running performance-critical tasks.
By allowing the application to warm up, you can reduce the burden placed on the compiler over time.
2. Use Compiler Flags
Adjusting compiler flags is a more involved way to tackle high CPU usage:
-
Disable Inline: This will limit optimizations made by the compiler at the expense of performance.
🔧snippet.shjava -Xint -jar YourApplication.jar
Note: Using
-Xint
runs the Java program in interpreted mode. While this alleviates CPU usage, it can lead to significant performance degradation. -
Longer Compilation Timeouts: If you adjust the JIT compiler to allow longer times for method compilation, you might see reduced CPU spikes:
🔧snippet.shjava -XX:CompileThreshold=5000 -jar YourApplication.jar
This increases the count of method invocations needed before it’s compiled, allowing the application to stabilize before JIT compiles kick in.
3. Optimize HotSpot Configuration
The HotSpot JVM, the default JVM for Java, offers configurations to optimize compilation. Adjusting the following options can help:
-
Adjust Tiered Compilation: Tiered compilation (enabled by default) allows both C1 and C2 compilations to take place. However, if your application has a predictable and stable execution pattern, you might want to disable tiered compilation:
🔧snippet.shjava -XX:-TieredCompilation -jar YourApplication.jar
-
Use JVM Flags for Specific Functionality: Depending on your application, certain flags, such as
-XX:MaxInlineLevel
or-XX:MaxInlineSize
, can prove beneficial. These control the extent to which inlining occurs.
4. Profile the Application Code
Poorly structured code can lead to excessive CPU use during compilation. Here are points to consider:
- Regular profiling of your Java application can help identify bottlenecks and hot methods that require optimization. Libraries such as Java Flight Recorder can be particularly useful for tracking down performance issues.
- Optimize hot methods by reducing their complexity, which can lead to fewer resource demands from the compiler.
5. Upgrade Your Java Version
Every Java release optimizes performance and resource management. If your application is on an older Java version, consider upgrading:
- Newer versions might include improvements that reduce CPU usage during JIT compilation, incorporating better algorithms for the C1/C2 compilers.
The Closing Argument
High CPU usage regarding C1 and C2 compiler threads can significantly impact the performance of Java applications. Proper diagnosis, strategic use of compiler flags, code optimizations, and possibly a JVM upgrade are effective strategies to manage and mitigate this problem.
Remember, optimizing CPU usage can lead not just to a smoother application but also more efficient resource utilization, cheaper cloud costs, or better user experiences.
For additional information, you can refer to the official Java Performance Tuning guide provided by Oracle.
High CPU usage is an ever-present challenge, but with proper tools and techniques, you can effectively manage it. As always, test each change thoroughly to assess performance impacts before deploying to production. Happy coding!