Cracking the Code: Analyzing Java Deadlocks Easily
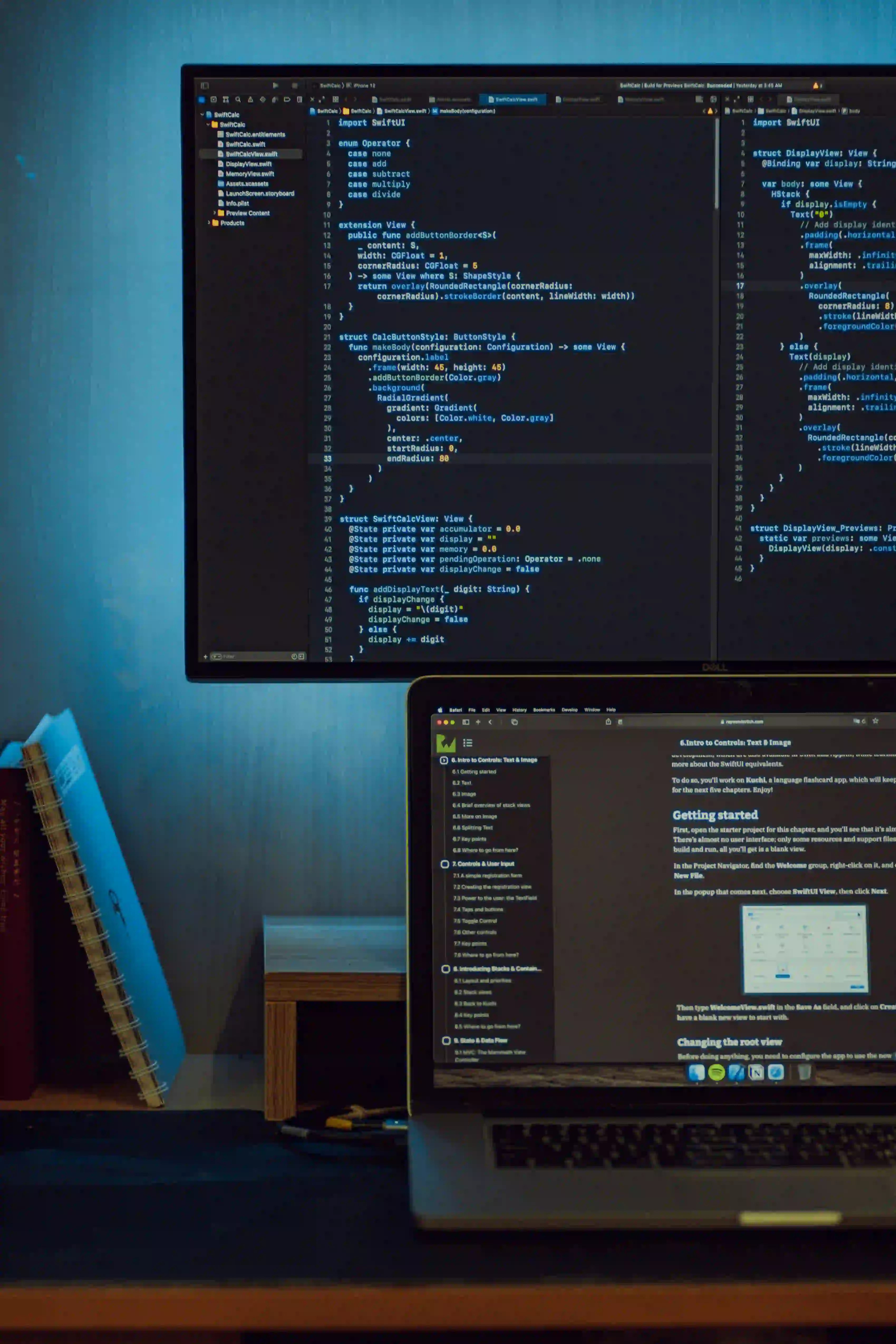
Cracking the Code: Analyzing Java Deadlocks Easily
In the realm of concurrent programming, Java provides powerful tools to handle multiple threads running simultaneously. However, with great power comes great responsibility - specifically, the risk of encountering deadlocks. This blog post aims to demystify Java deadlocks, explaining how they occur, their impacts, and providing methods to effectively analyze and prevent them.
Table of Contents
- What is a Deadlock?
- Identifying Deadlocks
- Example of a Deadlock in Java
- Analyzing Deadlocks
- Strategies to Prevent Deadlocks
- Conclusion
What is a Deadlock?
A deadlock occurs when two or more threads are blocked forever, each waiting for the other to release a resource. In simpler terms, it’s like a traffic jam involving multiple vehicles, where no vehicle can move forward because they're all holding onto resources that others need.
In Java, a deadlock often arises from the improper use of synchronized blocks or methods when multiple threads compete for the same locks. Since these threads wait indefinitely, deadlocks can severely impact an application’s performance.
Identifying Deadlocks
Identifying deadlocks is crucial since they can lead to unresponsive applications. Here are a few tell-tale signs:
- Threads are blocked at a certain point in the application.
- Some threads are not completing, while others may be working perfectly.
Java provides a built-in mechanism to detect deadlocks. The ThreadMXBean
allows developers to manage thread activities, including detecting deadlocks. We’ll explore this further in our analysis section.
Example of a Deadlock in Java
Let’s take a look at a simple example to illustrate a deadlock situation in Java.
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
System.out.println("Holding lock 1...");
// Simulate work
try { Thread.sleep(100); } catch (InterruptedException e) {}
synchronized (lock2) {
System.out.println("Acquired lock 2!");
}
}
}
public void method2() {
synchronized (lock2) {
System.out.println("Holding lock 2...");
// Simulate work
try { Thread.sleep(100); } catch (InterruptedException e) {}
synchronized (lock1) {
System.out.println("Acquired lock 1!");
}
}
}
public static void main(String[] args) {
DeadlockExample example = new DeadlockExample();
Thread thread1 = new Thread(example::method1);
Thread thread2 = new Thread(example::method2);
thread1.start();
thread2.start();
}
}
Commentary on the Code
In this code:
- Two Locks: We have two objects,
lock1
andlock2
, which act as mutual exclusions. - Two Methods:
method1
tries to acquirelock1
first and thenlock2
, whilemethod2
tries to acquire them in reverse order. - Deadlock Scenario: If
thread1
holdslock1
andthread2
holdslock2
, they will wait indefinitely for each other.
Analyzing Deadlocks
To analyze deadlocks, you'll need to employ a few strategic tools available in Java.
1. Java Management Extensions (JMX)
JMX provides monitoring capabilities for Java applications. The ThreadMXBean
can help you detect deadlocks programmatically. Here's how you can implement it:
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadInfo;
import java.lang.management.ThreadMXBean;
public class DeadlockDetector {
public static void main(String[] args) {
ThreadMXBean threadMXBean = ManagementFactory.getThreadMXBean();
long[] deadlockedThreads = threadMXBean.findDeadlockedThreads();
if (deadlockedThreads != null) {
for (long threadId : deadlockedThreads) {
ThreadInfo threadInfo = threadMXBean.getThreadInfo(threadId);
System.out.println("Deadlocked Thread: " + threadInfo.getThreadName());
}
} else {
System.out.println("No deadlocks detected!");
}
}
}
Commentary on the Detector
This code snippet:
- Initializes JMX: It accesses the
ThreadMXBean
class to manage threads. - Finds Deadlocks: The
findDeadlockedThreads()
method retrieves all threads that are deadlocked. - Reports Outcomes: It prints out the names of deadlocked threads if found, otherwise, it states that no deadlocks exist.
Strategies to Prevent Deadlocks
Preventing deadlocks requires a thoughtful design of your multithreading environment. Here are several strategies:
1. Lock Ordering
One effective strategy is to enforce a global order of locks. By ensuring that all threads acquire locks in the same order, you can avoid circular waiting - a necessary condition for a deadlock.
For example:
void method1() {
synchronized (lock1) {
synchronized (lock2) {
// Critical section
}
}
}
void method2() {
synchronized (lock1) {
synchronized (lock2) {
// Critical section
}
}
}
2. Timeout Mechanisms
Implementing timeouts can also be beneficial. Instead of waiting indefinitely for a lock, a thread can attempt to acquire a lock for a specified duration, and if it fails, it can back off and retry later.
3. Using Higher-Level Concurrency Utilities
Java provides higher-level abstractions in the java.util.concurrent
package. Using classes such as ReentrantLock
with timeouts, or Semaphore
, can greatly reduce the risk of deadlocks.
For example:
import java.util.concurrent.locks.ReentrantLock;
public class AdvancedLock {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void method1() {
try {
if (lock1.tryLock() && lock2.tryLock()) {
// Critical section
}
} finally {
if (lock1.isHeldByCurrentThread()) lock1.unlock();
if (lock2.isHeldByCurrentThread()) lock2.unlock();
}
}
}
In Conclusion, Here is What Matters
Understanding and analyzing deadlocks in Java is crucial for building robust applications. By identifying how deadlocks occur, using tools like JMX for detection, and employing prevention strategies, you can minimize the impact of deadlocks in your multithreaded applications.
For further reading, explore more on Java Threading Basics and delve into Effective Java to learn design patterns that help avoid issues like deadlocks.
By integrating these practices, you'll be one step closer to mastering Java concurrency and creating responsive applications that can manage multiple threads effectively.