Common Pitfalls in Single Page Apps with AngularJS & Spring Boot
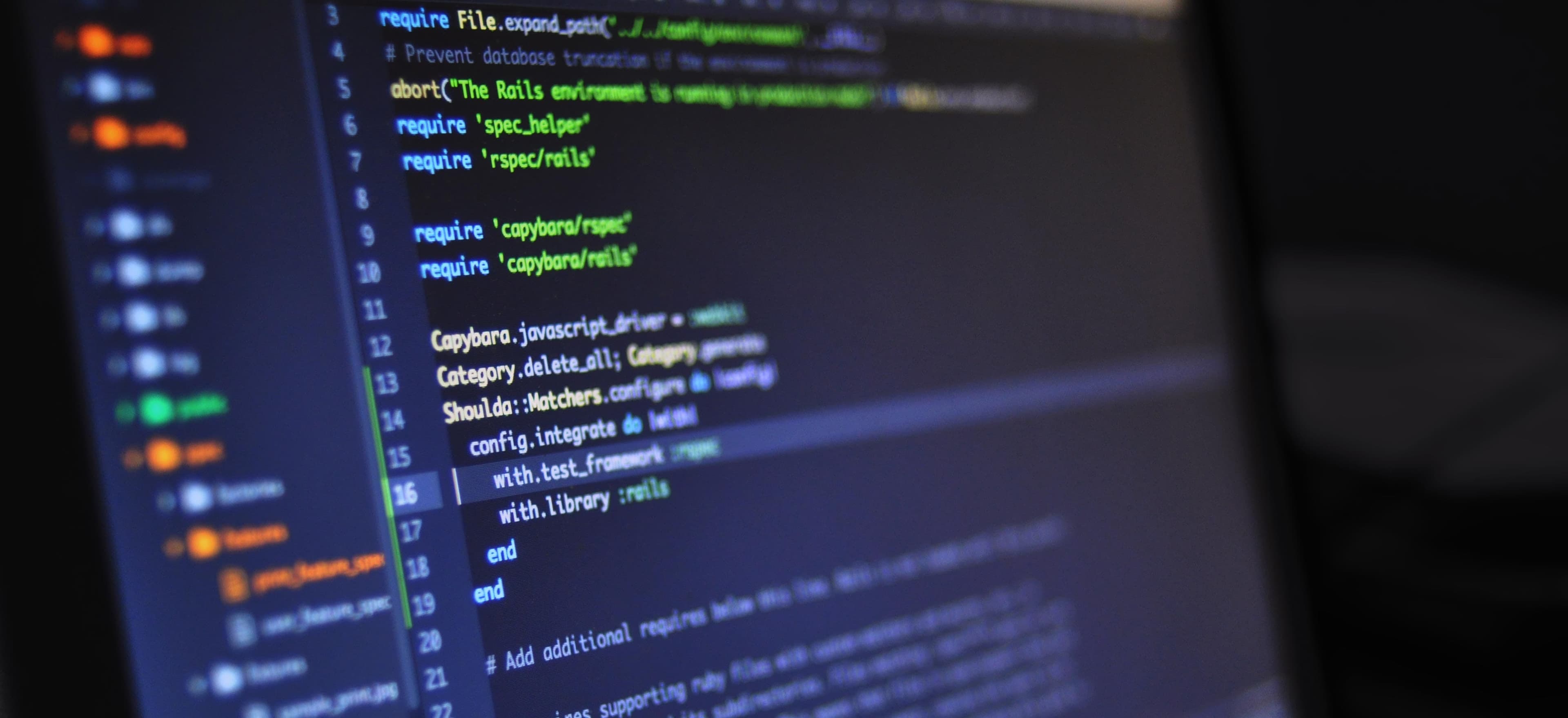
- Published on
Common Pitfalls in Single Page Apps with AngularJS & Spring Boot
Single Page Applications (SPAs) have gained immense popularity due to their ability to deliver smoother user experiences mimicking the behavior of desktop applications. AngularJS is a powerful framework for building SPAs, while Spring Boot serves as a robust backend solution. However, developers often face several common pitfalls when integrating these technologies. In this blog post, we will explore these pitfalls and provide solutions to effectively avoid them.
Table of Contents
- Understanding the Architecture
- Pitfall 1: Improper State Management
- Pitfall 2: Overusing $scope
- Pitfall 3: API Mismanagement
- Pitfall 4: Ignoring Security Best Practices
- Pitfall 5: Performance Issues
- Conclusion
Understanding the Architecture
Before diving into the pitfalls, it's essential to understand how AngularJS and Spring Boot communicate.
- AngularJS interacts with the user interface and makes HTTP calls to the backend.
- Spring Boot provides RESTful services to handle those API requests.
This architecture enables SPAs to load resources asynchronously, enhancing interactive experiences. However, it also introduces complexity that can lead to common development mistakes.
Pitfall 1: Improper State Management
One prevalent challenge in AngularJS applications is the management of state. SPA applications often have multiple views and corresponding states, making it crucial to manage and persist state effectively.
Why It Matters
Without proper state management, applications become hard to debug, and it can lead to unexpected behaviors. Data loss during navigation or user interaction can frustrate users.
Solution: Use Services
Using AngularJS services to maintain the application state is a common and effective solution. Services are singleton objects, meaning their state persists throughout the application lifecycle.
angular.module('myApp').service('UserService', function() {
var user = {};
return {
setUser: function(data) {
user = data;
},
getUser: function() {
return user;
}
};
});
Commentary
Here, we define a service called UserService
that keeps user data. By using this service, we ensure that user information is consistent and accessible across different components without conflicting states. This approach will save you from the chaos of managing state directly in controllers.
Pitfall 2: Overusing $scope
In AngularJS, $scope
plays a critical role in the model-view-controller paradigm, acting as the bridge between the controller and the view.
Why It Matters
Many developers, especially beginners, often tend to overuse $scope
, which can lead to messy code and performance-related issues.
Solution: Use Controller As Syntax
Instead of relying heavily on $scope
, consider using the "controller as" syntax for more manageable and organized code.
angular.module('myApp').controller('MyController', function() {
var vm = this;
vm.greeting = "Hello, World!";
});
Commentary
By using controller as
, you create a more readable and maintainable codebase. vm
here stands for "view model," allowing us to clearly define the data we want to expose in the view while keeping it organized. This also aids in testing, as it's clearer what data is being referenced.
Pitfall 3: API Mismanagement
SPAs rely heavily on APIs for data fetching and manipulation. Mismanagement of these APIs can lead to performance issues, slow user experience, and other problems.
Why It Matters
APIs should be efficient. They need to handle multiple requests concurrently and respond quickly. A single inefficient API can bottleneck the entire application.
Solution: Use Proper HTTP Methods and Caching
Choose the correct HTTP methods (GET, POST, PUT, DELETE) and ensure you leverage caching wherever applicable. For example:
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping
public List<User> getAllUsers() {
// Logic to retrieve users from database
}
@PostMapping
public User createUser(@RequestBody User user) {
// Logic to save user in database
}
}
Commentary
Here, we see how the UserController
in Spring Boot is properly structured. We utilize REST principles, enhancing the predictability and scalability of our APIs. Furthermore, consider using HTTP caching headers to optimize repeated requests.
Pitfall 4: Ignoring Security Best Practices
Security is often an overlooked aspect of SPA development, especially when using AngularJS with Spring Boot.
Why It Matters
Failing to implement proper security practices can expose your application to various vulnerabilities, including XSS (Cross-Site Scripting) and CSRF (Cross-Site Request Forgery).
Solution: Implement Token-Based Authentication
To enhance security, implement token-based authentication using JSON Web Tokens (JWT). This helps in validating user sessions without the need for traditional cookie-based approaches.
@RestController
public class AuthController {
@PostMapping("/auth/login")
public ResponseEntity<?> login(@RequestBody AuthRequest authRequest) {
// Authenticate user and generate token
}
}
Commentary
By using token-based authentication, you can better protect your RESTful endpoints. Each time a request is made, the server checks the validity of the token, ensuring secure access.
Pitfall 5: Performance Issues
As SPAs grow in complexity, performance issues may arise, leading to a poor user experience.
Why It Matters
Long loading times, unresponsive interfaces, and high resource consumption can easily drive users away from your application.
Solution: Optimize Loading & Avoid Memory Leaks
- Lazy Loading: Load only the necessary modules when required to reduce the initial load time.
- Optimize Digest Cycle: Reduce the number of watched variables to prevent excessive digest cycles.
angular.module('myApp')
.controller('LazyLoadController', ['$scope', '$timeout', function($scope, $timeout) {
$scope.loadData = function() {
// Logic to load data
};
$timeout(function() {
$scope.loadData();
}, 1000); // Loads data after a second
}]);
Commentary
In this example, using $timeout
helps delay the loading of data, improving the initial page load and optimizing user experience. Always keep an eye on performance profiling to identify any memory leaks or performance bottlenecks.
My Closing Thoughts on the Matter
Navigating the complex landscape of Single Page Applications using AngularJS and Spring Boot necessitates a thorough understanding of both the technologies and the common pitfalls developers may encounter. By adhering to the best practices outlined in this post, you can enhance your application's stability, maintainability, and security.
If you're looking to dive deeper into AngularJS and Spring Boot, check out the official AngularJS documentation here and the Spring Boot documentation here.
Final Thoughts
As you continue building your SPA, remember that careful planning and implementation can save you time and resources in the long run. Stay updated on best practices and trends, and don't hesitate to revisit your architecture and solutions as your application evolves. Happy coding!
Checkout our other articles