Debugging Byte Buddy Proxy Creation: Common Hurdles & Fixes
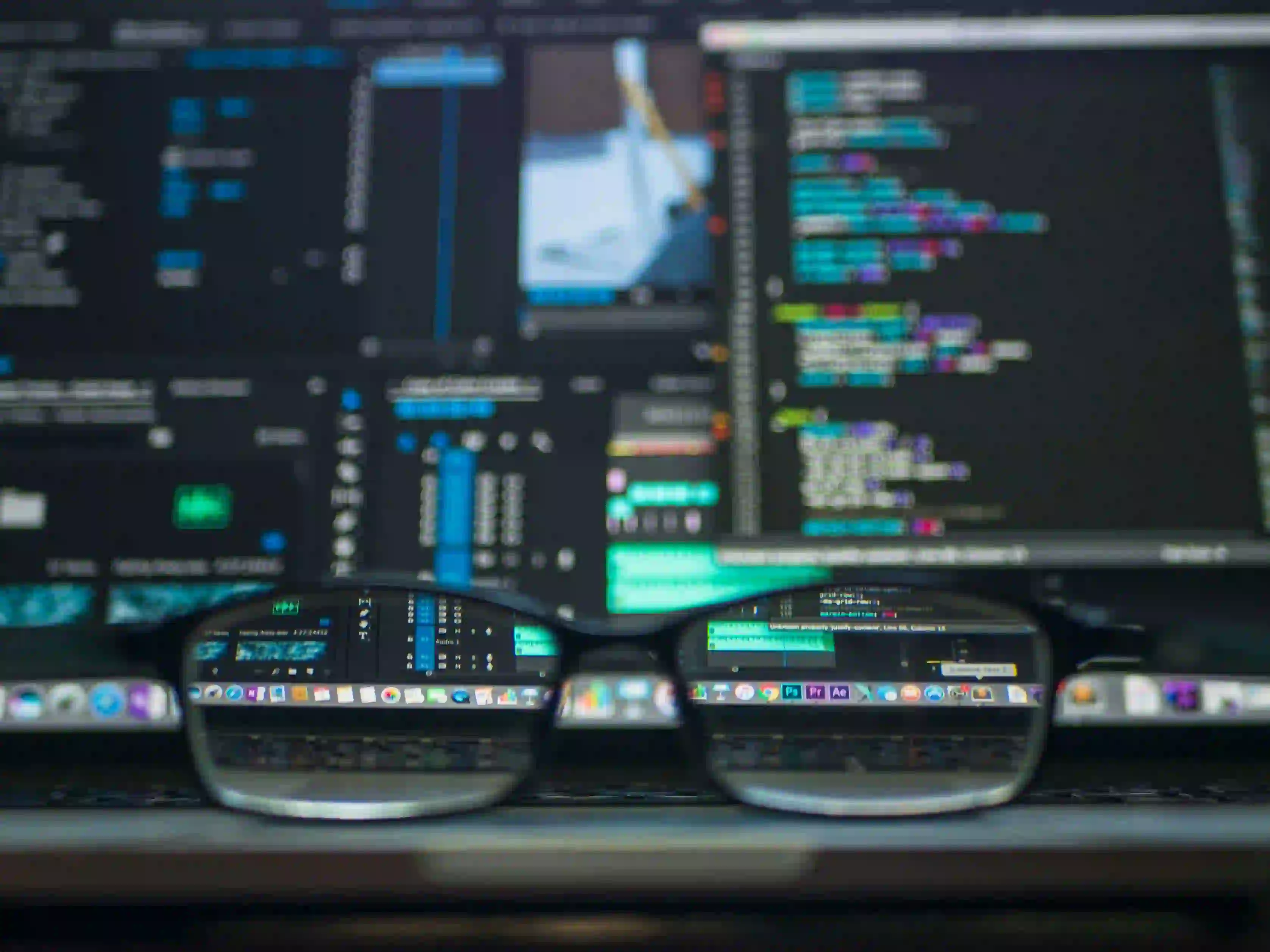
Debugging Byte Buddy Proxy Creation: Common Hurdles & Fixes
When developing Java applications, dynamic proxies can be a lifesaver when it comes to separating concerns, enhancing functionality, and implementing cross-cutting concerns. One of the most powerful libraries for creating dynamic proxies in Java is Byte Buddy. However, working with Byte Buddy can sometimes lead to frustrations, especially during the proxy creation phase. In this blog post, we will explore common hurdles developers face while using Byte Buddy to create proxies and provide effective solutions to these challenges.
What is Byte Buddy?
Byte Buddy is a Java library designed to dynamically create and modify Java classes at runtime. It allows developers to implement proxies, interceptors, and more with minimal effort while maintaining high performance.
Creating proxies using Byte Buddy typically involves defining a class and implementing an interface. However, various issues can arise during this process. If you encounter problems when creating proxies, don’t worry! This guide will help you troubleshoot common hurdles.
Common Hurdles and Fixes
1. Class Loading Issues
Problem: One of the most frequent problems developers face is related to class loading. If Byte Buddy cannot find the class to proxy, it will throw a ClassNotFoundException
.
Solution: Always ensure that the class you are trying to proxy is available in the classpath. Check your project structure and confirm that the necessary dependencies are included. If necessary, add additional logging to track the class loading process.
Here is an example of how to create a proxy in Byte Buddy:
import net.bytebuddy.ByteBuddy;
import net.bytebuddy.dynamic.DynamicType;
import net.bytebuddy.implementation.MethodDelegation;
import net.bytebuddy.implementation.StubMethod;
public class ProxyExample {
public static void main(String[] args) {
try {
DynamicType.Unloaded<SampleInterface> dynamicType = new ByteBuddy()
.subclass(SampleInterface.class)
.method(named("sayHello"))
.intercept(MethodDelegation.to(SampleInterceptor.class))
.make();
Class<? extends SampleInterface> loaded = dynamicType
.load(ProxyExample.class.getClassLoader())
.getLoaded();
SampleInterface proxy = loaded.getDeclaredConstructor().newInstance();
proxy.sayHello();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, we are creating a proxy class that implements the SampleInterface
and intercepts the sayHello
method. If the target class isn't found in the classpath, you'll notice the exception right away.
2. Interceptor Not Invoked
Problem: Another common problem occurs when the interceptor does not seem to be called. This is often caused by misconfigured method interception.
Solution: To fix this, ensure that you are correctly specifying the method you want to intercept. Use method names or annotations for more specific method matching.
Here's an example using method annotation interception:
import net.bytebuddy.implementation.MethodDelegation;
import net.bytebuddy.matcher.ElementMatchers;
public class ProxyExample {
public static void main(String[] args) {
DynamicType.Unloaded<SampleInterface> dynamicType = new ByteBuddy()
.subclass(SampleInterface.class)
.method(ElementMatchers.named("sayHello"))
.intercept(MethodDelegation.to(SampleInterceptor.class))
.make();
// Load and test proxy
}
}
3. Reflection Issues
Problem: If you're using reflection to call methods on the proxy, Java's access control may block the calls if methods are not public.
Solution: Ensure that the methods you want to invoke reflectively are declared as public in your interface and your implementing classes. Additionally, don't forget to handle IllegalAccessException
.
public interface SampleInterface {
void sayHello();
}
class SampleInterceptor {
public static void intercept() {
System.out.println("Intercepted!");
}
}
When invoking the method through reflection, always check accessibility:
Method method = proxyClass.getMethod("sayHello");
method.invoke(proxy);
4. Versioning Conflicts
Problem: Versioning conflicts between Byte Buddy and other dependencies can lead to unexpected behavior.
Solution: Always ensure you are using compatible versions of Byte Buddy along with other libraries in your build configuration. Ensure you are using the latest release or the version that is specifically known to work with your other libraries.
Check your pom.xml
for Maven or build.gradle
for Gradle to avoid version conflicts.
5. Debugging Issues with Logging
Problem: Debugging proxy creation issues can become tedious without proper logging.
Solution: Make sure to implement adequate logging throughout your code. Use the logging capabilities available in Java or integrate a logging framework like SLF4J or Log4J.
For example, you can add logging inside your interceptor:
public class SampleInterceptor {
public static void intercept() {
System.out.println("Intercepted!");
}
}
Using logging packages allows you to change log levels without modifying the code.
Closing Remarks
Debugging Byte Buddy proxy creation can be frustrating, but understanding the common pitfalls and their solutions can make your journey smoother. Always pay attention to class loading, method interception specifics, and access levels. Additionally, being mindful of version conflicts and having a robust logging mechanism can help troubleshoot your issues effectively.
For more information on Byte Buddy’s capabilities, check out Byte Buddy Documentation, which offers a myriad of examples and further reading on proxy creation and bytecode manipulation.
With these insights, you should be well-equipped to debug any issues you encounter when creating proxies with Byte Buddy. Stay persistent, and happy coding!