Applying AspectJ Pointcuts to All Package Methods Made Easy
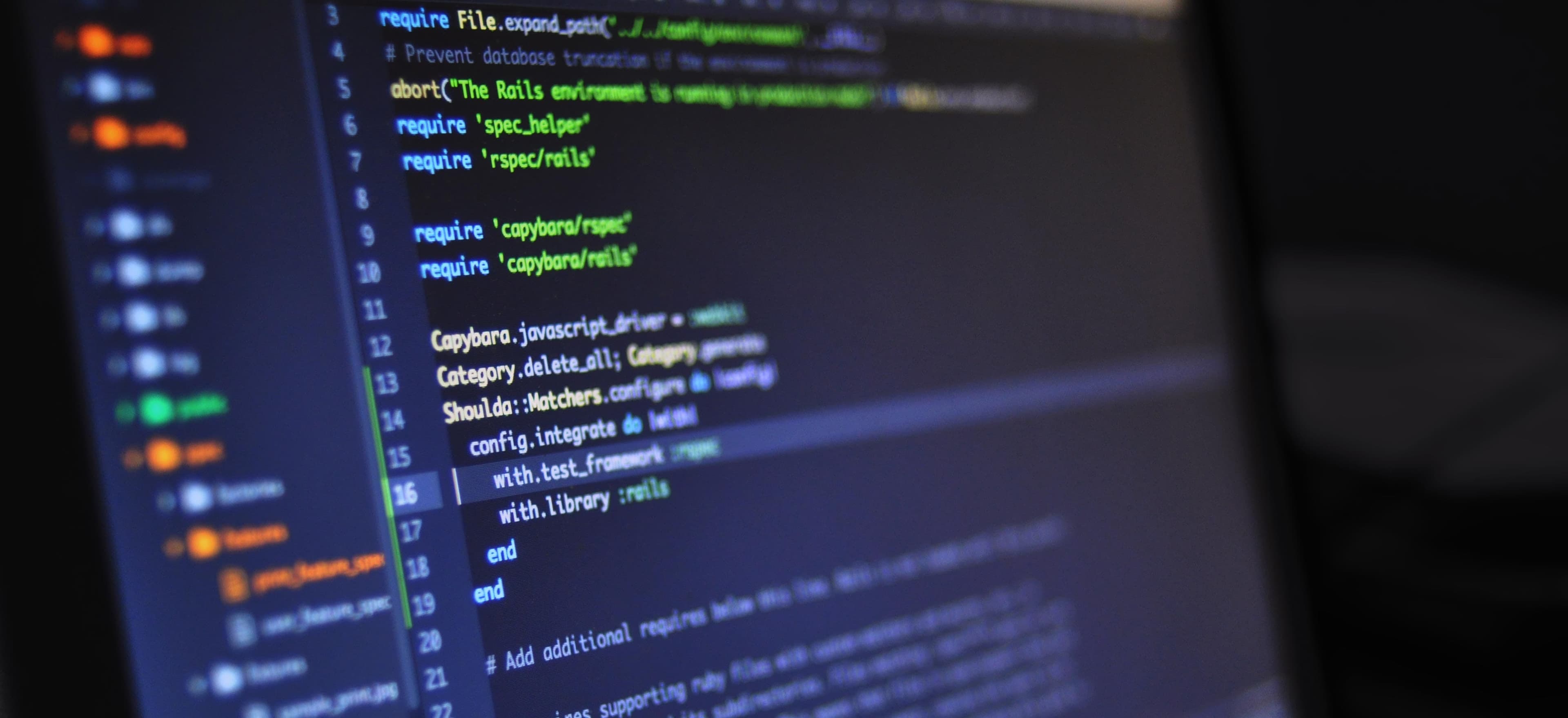
- Published on
Applying AspectJ Pointcuts to All Package Methods Made Easy
AspectJ is a powerful extension of Java that allows developers to implement aspect-oriented programming (AOP). This approach makes it simple to separate cross-cutting concerns—such as logging, security, and transaction management—from the business logic of your application. In this blog post, we will explore how to apply AspectJ pointcuts to all methods within a particular package effortlessly.
What Are AspectJ Pointcuts?
A pointcut is a crucial concept in AspectJ that helps identify where an aspect (a module that encapsulates a cross-cutting concern) should be applied within the application. Pointcuts provide a way to specify certain join points, which are specific points in the program execution, where the advice (code that runs at a join point) should be executed.
For instance, you can use pointcuts to apply logging across different packages or methods within your Java application. This functionality is particularly useful when you need consistent behavior, like logging or security checks, throughout your application.
Why Use AOP with AspectJ?
Using AspectJ provides several advantages:
- Separation of Concerns: AOP allows you to separate business logic from cross-cutting concerns.
- Code Reusability: Aspects can be reused across different parts of your application.
- Reduced Code Duplication: By centralizing cross-cutting logic, you minimize code duplication.
- Improved Maintainability: Changes to cross-cutting concerns can be made in one place, reducing the risk of bugs.
Let's delve into an example where we will create an Aspect that logs method execution for all methods within a specific package.
Setting Up AspectJ in Your Project
To use AspectJ in your Java project, you'll need to set it up with Maven. Here’s how you can do it:
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.9.7</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.7</version>
</dependency>
Creating the Aspect Class
Once you've set up AspectJ, the next step is to create your aspect class. Below, we will create a simple logging aspect that captures method execution within a specific package.
Sample Aspect Class
package com.example.logging;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
@Aspect
public class LoggingAspect {
// This pointcut matches all methods in the com.example.service package
@Pointcut("execution(* com.example.service..*(..))")
public void serviceLayerExecution() { }
// Advice that gets executed after the matched methods
@After("serviceLayerExecution()")
public void afterServiceLayer(JoinPoint joinPoint) {
System.out.println("Executed method: " + joinPoint.getSignature());
}
}
Explanation of the Code
-
@Aspect: This annotation defines the class as an Aspect.
-
Pointcut Definition: The
serviceLayerExecution
pointcut matches all methods within thecom.example.service
package and any of its sub-packages.- Execution Specification:
Here,execution(* com.example.service..*(..))
execution
signifies that we are capturing method execution. The*
in the wildcard represents any return type, and..
specifies that we want to include methods from sub-packages as well.
- Execution Specification:
-
Advice: The
afterServiceLayer
method is the advice that runs after any method matching the pointcut. We log the method's signature usingjoinPoint.getSignature()
.
Testing the Aspect
To see our aspect in action, you'll want to create some service classes within the specified package that simulate business logic.
Sample Service Class
package com.example.service;
public class UserService {
public void addUser(String username) {
// Simulate adding user logic
System.out.println("User " + username + " added.");
}
public void deleteUser(String username) {
// Simulate deleting user logic
System.out.println("User " + username + " deleted.");
}
}
Using the Service
Here's how you can test your aspect in your main application:
package com.example;
import com.example.service.UserService;
public class Main {
public static void main(String[] args) {
UserService userService = new UserService();
userService.addUser("JohnDoe");
userService.deleteUser("JohnDoe");
}
}
Expected Output
When you run the above Main
method, you should observe output similar to the following, demonstrating that the logging aspect is applied:
User JohnDoe added.
Executed method: public void com.example.service.UserService.addUser(java.lang.String)
User JohnDoe deleted.
Executed method: public void com.example.service.UserService.deleteUser(java.lang.String)
Advanced Pointcut Expressions
AspectJ provides a variety of powerful pointcut designators to refine which methods should be intercepted based on access modifiers, annotations, or the type of the method.
Example with Annotations
Suppose you want to apply logging only to methods annotated with a specific annotation. You could modify your pointcut like so:
@Pointcut("execution(* com.example.service..*(..)) && @annotation(com.example.annotations.Loggable)")
public void serviceLayerExecutionWithLoggable() { }
This pointcut will now only trigger if the method has the @Loggable
annotation.
Final Thoughts
AspectJ and AOP offer a robust approach to managing cross-cutting concerns within Java applications. By utilizing pointcuts, you can easily inject behaviors, such as logging, into methods across an entire package, keeping your code cleaner and more maintainable.
For further reading on AspectJ and AOP concepts, consider checking out the AspectJ Documentation or Spring AOP.
Employ AspectJ in your projects and redefine how you manage cross-cutting concerns today! Happy coding!