Mastering PDF Headers and Footers with iText in Java
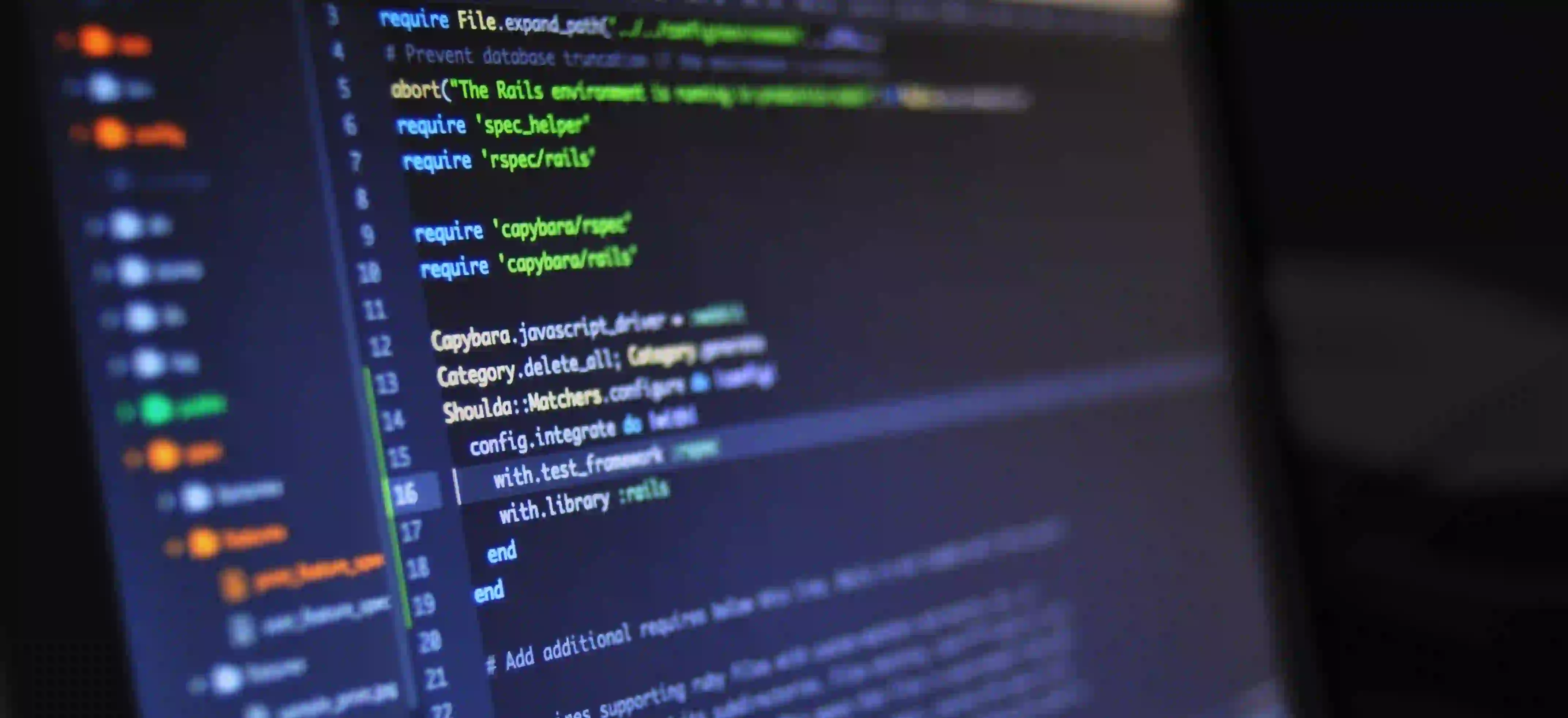
Mastering PDF Headers and Footers with iText in Java
Generating and manipulating PDFs is an essential skill for Java developers, especially when it comes to delivering well-structured documents. Among the critical aspects when working with PDFs are headers and footers. These elements provide context, enhance presentation, and guide the reader through the content of your document. In this blog post, we will dive deep into how to effectively implement headers and footers using the iText library.
Why Use iText?
iText is a robust, high-quality library for creating and manipulating PDF files in Java. Its versatility allows developers to generate complex documents while offering the ability to manipulate existing PDFs. Given that headers and footers are essential in professional documentation, iText makes the task straightforward with its various utilities.
Prerequisites
Before we get started, ensure you have the following:
- Basic understanding of Java and how to set up a Java project.
- iText library (version 7.x or higher). Add the following Maven dependency to your
pom.xml
:
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itext7-core</artifactId>
<version>7.1.15</version>
</dependency>
Additionally, you can download iText directly if you are not using Maven.
Creating a Basic PDF
To understand how to manipulate headers and footers, we’ll create a simple PDF document. Here’s how you can do that:
import com.itextpdf.kernel.pdf.*;
import com.itextpdf.kernel.pdf.canvas.PdfCanvas;
import com.itextpdf.layout.Document;
import com.itextpdf.layout.element.Paragraph;
import java.io.File;
public class SimplePDF {
public static void main(String[] args) {
String dest = "simple.pdf";
// Step 1: Create a PDF document
PdfWriter writer = new PdfWriter(dest);
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
// Step 2: Add content
document.add(new Paragraph("Hello, World! This is our first PDF."));
// Step 3: Close document
document.close();
System.out.println("PDF created successfully.");
}
}
Explanation of the Code
- PdfWriter and PdfDocument: These classes are responsible for writing content to a PDF file and creating a new PDF document, respectively.
- Document: This class helps manage the content and layout.
Once you run this program, you'll find a PDF file named simple.pdf
in your project directory.
Adding Headers and Footers
Now that we have a basic PDF, let's dive into adding headers and footers. The key to adding these elements is creating an event handler that triggers on each page's generation.
Creating a Custom Page Event Handler
We'll create a new class that implements IEventHandler
. This class will define how headers and footers should appear on each page.
import com.itextpdf.kernel.events.IEventHandler;
import com.itextpdf.kernel.pdf.PdfDocument;
import com.itextpdf.kernel.pdf.canvas.PdfCanvas;
import com.itextpdf.kernel.pdf.canvas.parser.listener.IEventData;
import com.itextpdf.kernel.pdf.canvas.parser.PdfCanvasProcessor;
import com.itextpdf.kernel.pdf.canvas.parser.listener.IEventData;
public class HeaderFooterEventHandler implements IEventHandler {
private String header;
private String footer;
public HeaderFooterEventHandler(String header, String footer) {
this.header = header;
this.footer = footer;
}
@Override
public void handleEvent(IEventData data) {
PdfDocument pdfDoc = (PdfDocument) data.getDocument();
PdfCanvas canvas = new PdfCanvas(pdfDoc.getPage(data.getEventType().getPageNumber()));
// Draw header
canvas.beginText()
.setFontAndSize(PdfFontFactory.createFont(FontConstants.HELVETICA), 12)
.newLineAt(36, pdfDoc.getPage(data.getEventType().getPageNumber()).getPageSize().getHeight() - 36)
.showText(header)
.endText();
// Draw footer
canvas.beginText()
.setFontAndSize(PdfFontFactory.createFont(FontConstants.HELVETICA), 12)
.newLineAt(36, 36)
.showText(footer)
.endText();
}
}
Explanation of the Event Handler
- Header and Footer Text: The header and footer text are defined and used to display relevant information on every page.
- PdfCanvas: This acts as the canvas for drawing text on the PDF. The
beginText
andendText
methods denote where the text drawing begins and ends. - setFontAndSize: This method allows you to specify which font and size you want for your text.
Integrating the Event Handler with PDF Generation
Now that we've created our event handler, we need to integrate it into our PDF creation process. Let’s update our previous SimplePDF
class.
String dest = "simple_with_header_footer.pdf";
PdfWriter writer = new PdfWriter(dest);
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
// Creating header and footer text
String headerText = "My PDF Header";
String footerText = "Page 1";
// Step 1: Adding event handler for headers and footers
pdf.addEventHandler(PdfDocumentEvent.END_PAGE, new HeaderFooterEventHandler(headerText, footerText));
// Adding content
for (int i = 1; i <= 5; i++) {
document.add(new Paragraph("This is page " + i));
// Create a new page
document.add(new AreaBreak());
}
// Closing document
document.close();
Explanation of the Enhanced PDF Creation
- Event Handler Registration: The line
pdf.addEventHandler()
associates our custom event handler with theEND_PAGE
event. Therefore, our headers and footers will appear automatically whenever a new page is created. - AreaBreak: This is used to create a new page programmatically in a loop.
Result
After running the updated program, you will have a new PDF file named simple_with_header_footer.pdf
. Each page will feature the predefined headers and footers that aid in identifying the content's context.
Additional Resources
To delve deeper into exploring iText functionalities, you may want to consider the following resources:
- iText 7 Documentation - A complete guide to mastering iText.
- PDF Association - Network and resources relating to PDF technology and innovations.
Key Takeaways
By leveraging the iText library, you can easily manage the complexities of document generation in Java, including headers and footers. Understanding how to implement and customize these elements allows developers to produce professional-quality PDFs that communicate effectively with readers.
In this blog post, we covered the essentials of working with PDFs in Java using iText. From setting up a basic document to incorporating headers and footers, developers now have the tools necessary for enhancing their PDF generation capabilities. Keep experimenting and see how you can further customize your PDFs to meet your project requirements. Happy coding!