Why Redis's Single-Threading Can Be a Performance Limitation
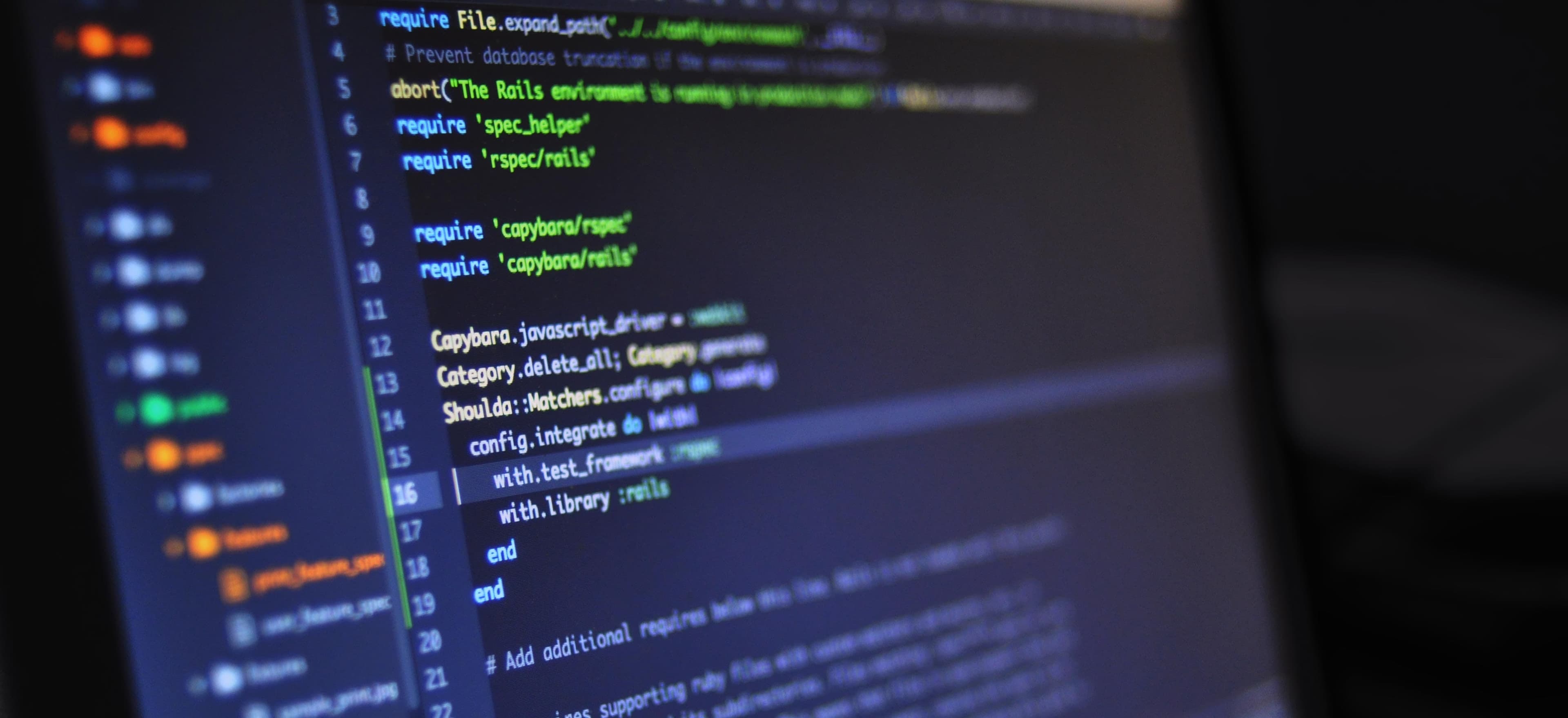
- Published on
Why Redis's Single-Threading Can Be a Performance Limitation
Redis is a powerful in-memory data structure store, widely used for caching, real-time analytics, and other high-performance applications. Its impressive speed is primarily due to its architecture, which allows for ultra-fast data retrieval. However, it’s important to understand the implications of Redis's design choices, particularly its reliance on a single-threaded event loop for handling requests. In this blog post, we will explore why Redis's single-threading can be a performance limitation, the scenarios where these constraints manifest, and potential strategies to mitigate these challenges.
Understanding Redis Architecture
Before delving into the specifics of single-threading, let’s take a brief look at Redis architecture.
Redis uses an event-driven, single-threaded model to handle client requests. This means that only one command is processed at a time, allowing Redis to avoid the complexities associated with multithreading, such as context switching and race conditions.
Benefits of Single-Threading
-
Simplicity: The single-threaded model simplifies the codebase considerably, making it easier to maintain and debug.
-
Consistent Performance: With only one thread, Redis avoids the pitfalls of concurrency issues, resulting in predictable performance, especially under concurrent access.
-
Efficient Memory Management: Memory management is unambiguous in a single-threaded context, helping to maximize performance.
These benefits come at a cost, however, as we will explore in the next section.
The Limitations of Single-Threading
1. CPU Bottlenecks
Since Redis runs on a single thread, it can only utilize one CPU core at a time, which becomes a bottleneck on multi-core systems. This means that as client requests increase, the performance won’t improve much because the single thread cannot take advantage of additional CPU resources.
Example Scenario:
Imagine an application with heavy read and write operations:
import redis
# Connect to Redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
# Simulate workload
for i in range(10000):
client.set(f'key_{i}', f'value_{i}')
While this example is simplistic, scaling up the number of concurrent clients can lead to increased latency and potentially cause Redis to lag.
2. Blocking Commands
Certain commands in Redis can block the server. When a blocking command (like BRPOP
) is executed, it can lead to latency for all subsequent requests. In a multi-threaded environment, these could be handled in parallel, thus improving overall responsiveness.
3. Limited Throughput
As the demand on Redis increases, the single-threaded model restricts throughput. This becomes especially relevant with write-heavy applications where the queue grows, and commands are queued, waiting for execution.
4. Impact on Performance Metrics
For monitoring tools or APIs that depend on metrics, the single-threaded nature can skew performance benchmarks, particularly under stress-testing scenarios. For instance, a typical testing setup that sees a single-thread performance measurement will not accurately reflect the potential of a multi-core system.
Mitigating Performance Limitations
Despite these limitations, there are several strategies that can help you get around the inherent constraints of Redis's single-threaded architecture.
1. Redis Clustering
Redis supports clustering, allowing you to partition databases across multiple nodes. This way, you can horizontally scale your application to utilize more CPU resources.
Example Command for Setting Up Clusters:
redis-cli --cluster create <node1>:<port1> <node2>:<port2> ... --cluster-replicas <num>
Through clustering, each node handles part of the load, effectively increasing throughput and ensuring better resource utilization.
2. Use Multiple Redis Instances
Running multiple Redis instances on the same machine can also help. Each instance can operate on its own dataset and effectively use one core.
3. Optimize Your Workload
Optimize your Redis commands by avoiding blocking calls when possible and ensuring that your data access patterns are efficient. Grouping commands and leveraging pipelines can minimize round trips.
Example of Pipelining:
pipeline = client.pipeline()
for i in range(10000):
pipeline.set(f'key_{i}', f'value_{i}')
pipeline.execute()
Pipelining reduces the number of round trips to Redis, allowing multiple commands to be sent in one request, thus minimizing latency.
4. Choosing the Right Data Structures
Redis offers various data structures (strings, sets, hashes, etc.) that can be more efficient for certain workloads. For example, using hashes instead of strings when dealing with objects can reduce memory usage and improve performance.
5. Asynchronous Clients
Using asynchronous Redis clients can improve throughput in scenarios where blocking commands are necessary. An async-based architecture can mitigate latency by allowing multiple commands to be sent while waiting for responses.
Lessons Learned
While the single-threaded nature of Redis has its advantages, it can also be a limiting factor under specific circumstances, especially as application complexity and performance demands increase. Understanding this trade-off is critical for architects and developers designing systems that incorporate Redis.
By leveraging clustering, optimizing workloads, running multiple instances, and using suitable data structures, you can mitigate the limitations posed by its single-threaded design, enabling you to harness the full potential of Redis.
For further learning, consider exploring Redis's official documentation for commands, architecture, and best practices, or check out Learn Redis with Redis University, which offers comprehensive courses on leveraging Redis effectively.
By following these best practices and being cognizant of Redis's inherent limitations, you can build resilient applications capable of handling various workloads while maintaining speed and efficiency.
In conclusion, Redis remains an outstanding tool for in-memory data structure needs. However, understanding its architecture ensures that you can effectively utilize its strengths while minimizing its weaknesses. Happy coding!