Mastering Avro to JSON Conversion in Java: A Step-by-Step Guide
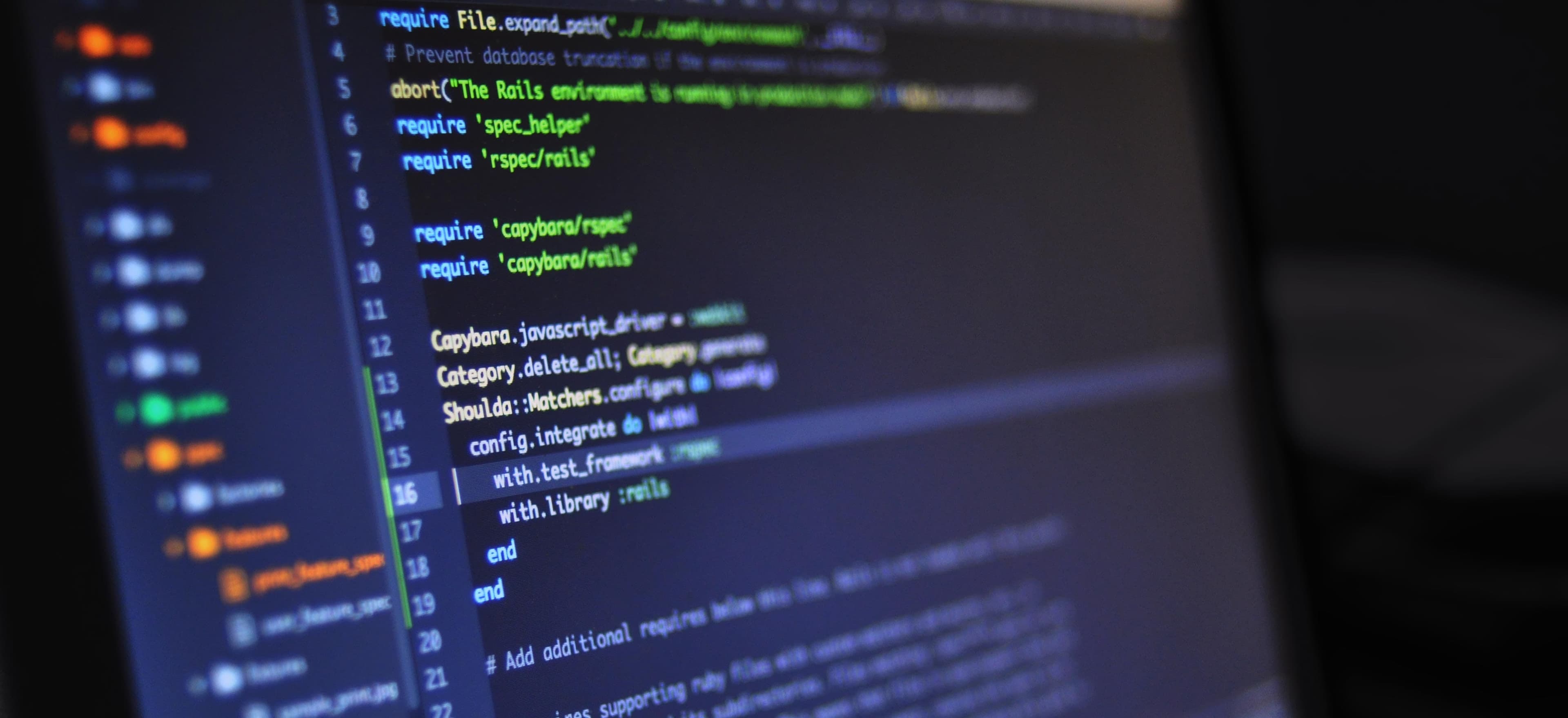
- Published on
Mastering Avro to JSON Conversion in Java: A Step-by-Step Guide
In the realm of data serialization, Avro stands out for its efficiency and schema evolution capabilities. It's widely used in big data frameworks such as Apache Hadoop and Apache Kafka. On the other hand, JSON's readability makes it a popular choice for data interchange. This blog post will guide you through the process of converting Avro data into JSON format using Java, providing insights and code snippets along the way.
Table of Contents
- Introduction to Avro and JSON
- Setting Up Your Java Environment
- Understanding Avro Schemas
- The Conversion Process
- Example Code Walkthrough
- Handling Errors and Validations
- Conclusion: Key Takeaways
Getting Started to Avro and JSON
Why Avro?
Apache Avro is a binary serialization format that provides robust support for schema evolution and is designed for high performance. Avro is schema-based, which means that the data can be self-describing. It allows for both backward and forward compatibility, making it a sensible choice in dynamic data environments.
Why JSON?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write for humans and machines. It's language-agnostic and is widely accepted in web applications for data transmission.
Setting Up Your Java Environment
To get started with Avro and JSON conversion in Java, ensure you have the following:
- Java Development Kit (JDK) - Version 8 or later.
- Apache Avro Library - You can find it in the Maven repository. Add the following dependency to your
pom.xml
if you are using Maven:
<dependency>
<groupId>org.apache.avro</groupId>
<artifactId>avro</artifactId>
<version>1.10.2</version> <!-- Please check for the latest version -->
</dependency>
- JSON Library - You can use Jackson or Gson. Here, we will use Jackson, so add the following dependency as well:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version> <!-- Please check for the latest version -->
</dependency>
Understanding Avro Schemas
An Avro schema defines the structure of the data. It is written in JSON format and specifies fields, data types, and other characteristics.
Here's an example schema:
{
"type": "record",
"name": "User",
"fields": [
{"name": "name", "type": "string"},
{"name": "age", "type": "int"},
{"name": "email", "type": "string"}
]
}
- type: Identifies the type of data structure.
- name: The name of the record.
- fields: An array of field objects with names and types.
The Conversion Process
The conversion process involves reading Avro data and then mapping it into JSON format. The basic steps are:
- Read the Avro file using an Avro
DataFileReader
. - Convert each Avro record into a JSON object using the Jackson library.
- Write the JSON to an output file or print it to the console.
Example Code Walkthrough
Now that we understand the fundamentals, let’s dive into the code.
Step 1: Reading Avro Data
The following code snippet demonstrates how to read an Avro file:
import org.apache.avro.file.DataFileReader;
import org.apache.avro.generic.GenericDatumReader;
import org.apache.avro.generic.GenericRecord;
import org.apache.avro.io.DatumReader;
import java.io.File;
import java.io.IOException;
public class AvroReader {
public static Iterable<GenericRecord> readAvroFile(String filePath) throws IOException {
File file = new File(filePath);
DatumReader<GenericRecord> datumReader = new GenericDatumReader<>();
DataFileReader<GenericRecord> dataFileReader = new DataFileReader<>(file, datumReader);
return dataFileReader;
}
public static void main(String[] args) {
try {
Iterable<GenericRecord> records = readAvroFile("path/to/your/input.avro");
// Process records (convert to JSON)
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation
- DataFileReader: This class reads Avro data from a file. It is initialized with a
DatumReader
. - GenericRecord: Represents a record with fields defined in the schema.
Step 2: Converting Avro to JSON
Using the Jackson library, we can now convert each record to JSON:
import com.fasterxml.jackson.databind.ObjectMapper;
public class AvroToJsonConverter {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String convertRecordToJson(GenericRecord record) throws IOException {
return objectMapper.writeValueAsString(record);
}
}
Explanation
- ObjectMapper: A class from Jackson that provides functionality for converting between Java objects and JSON.
- writeValueAsString: Converts the provided Java object (in this case, the GenericRecord) to a JSON string.
Step 3: Putting It All Together
Combining the reading and conversion process:
public class Main {
public static void main(String[] args) {
try {
Iterable<GenericRecord> records = AvroReader.readAvroFile("path/to/your/input.avro");
for (GenericRecord record : records) {
String json = AvroToJsonConverter.convertRecordToJson(record);
System.out.println(json);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation
This main
method reads Avro records and converts each record to JSON, printing the results to the console.
Handling Errors and Validations
When working with file I/O and data serialization, robust error handling is essential.
- File Not Found: Ensure the provided file path is correct.
- Schema Mismatches: Validate that the Avro data aligns with the expected schema.
- JSON Serialization: Handle exceptions during the JSON conversion.
Consider wrapping conversion code in try-catch blocks to manage and log errors efficiently.
Bringing It All Together: Key Takeaways
In this guide, we explored the process of converting Avro data to JSON in Java. This conversion is vital for integrating systems that utilize different data formats. Key points to remember include:
- Use Avro for efficiency in data serialization.
- JSON simplifies data interchange across different environments.
- Proper error handling enhances the robustness of your applications.
For more information on Avro, check the official Apache Avro Documentation.
Happy coding!