Boost Your Android Game's Performance: Measuring FPS Made Easy
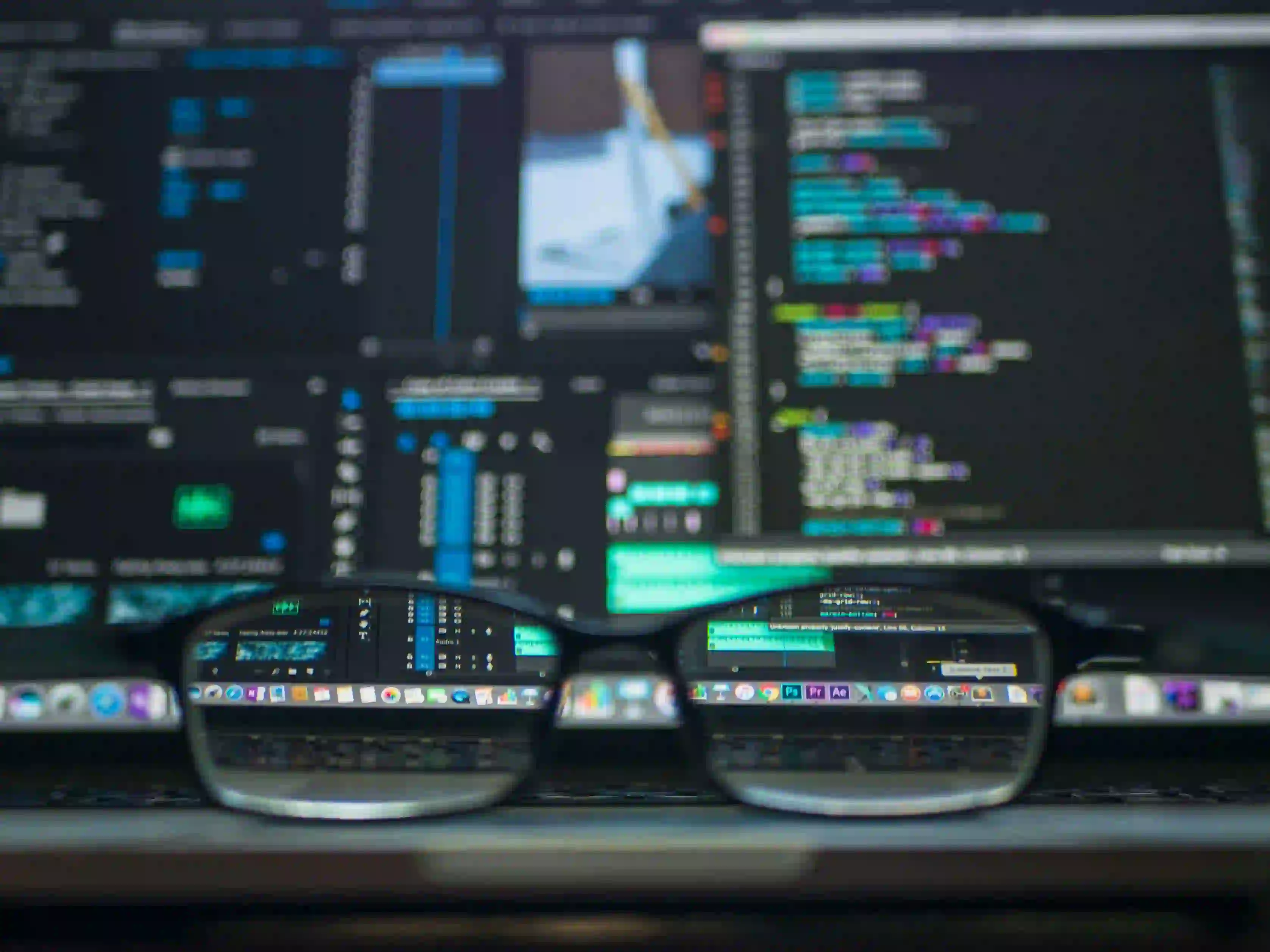
Boost Your Android Game's Performance: Measuring FPS Made Easy
In the world of mobile gaming, performance is key. Gamers expect smooth, immersive experiences, and when your game struggles with frame rates, it can lead to frustration and ultimately, uninstalls. In this blog post, we will explore how to measure Frames Per Second (FPS) in your Android game, understand why it’s crucial for performance optimization, and look at strategies to enhance your game's performance.
Understanding FPS: What It Is and Why It Matters
Frames Per Second (FPS) is a critical metric in gaming. It represents how many individual frames (or images) are rendered per second in a game. Higher FPS generally signifies smoother performance. For instance:
- 30 FPS: Acceptable for many games, though motion can appear choppy.
- 60 FPS: Ideal for most mobile games, providing fluidity and responsiveness.
- 120 FPS: Offers the highest performance, prevalent in high-end devices.
Monitoring FPS helps you pinpoint performance issues, enabling you to address lags and dips that could harm the gameplay experience. Poor FPS can distract players, leading them to abandon your game for better alternatives. To better understand FPS in your Android games, let’s learn how to measure it.
Measuring FPS in Your Android Game
To measure FPS in your Android game, one common approach is to utilize a dedicated Debug View. The process typically involves creating a custom view that overlays your game graphics. Here’s a step-by-step guide:
Step 1: Create a Custom FPS Counter View
First, we need to create a custom view that will monitor and display the FPS. Below is a simple implementation of an FPS counter class.
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.util.AttributeSet;
import android.view.View;
public class FPSCounterView extends View {
private Paint paint;
private long lastTime;
private int frameCount;
private float fps;
public FPSCounterView(Context context, AttributeSet attrs) {
super(context, attrs);
paint = new Paint();
paint.setTextSize(50);
lastTime = System.nanoTime();
frameCount = 0;
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
long currentTime = System.nanoTime();
frameCount++;
// Calculate FPS
if (currentTime - lastTime >= 1_000_000_000) { // every second
fps = frameCount;
frameCount = 0;
lastTime = currentTime;
}
// Draw FPS on screen
canvas.drawText("FPS: " + (int) fps, 50, 50, paint);
invalidate(); // Trigger the next draw
}
}
Explanation of the Code
- Paint Object: Used to draw text on the screen. The text size is set to 50 for good visibility.
- lastTime and frameCount: These help in calculating FPS.
lastTime
stores the previous timestamp, whileframeCount
counts frames. - onDraw Method: Called by the Android framework repeatedly. Inside it, we:
- Calculate the current time.
- Increment the frame count.
- If a second has passed, update the FPS and reset the counter.
Step 2: Integrate the FPS Counter in Your Game Layout
To display the FPS counter in your game, you need to add it to your layout. Modify your activity_main.xml
like this:
<com.example.yourapp.FPSCounterView
android:id="@+id/fpsCounter"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
Step 3: Add FPS Counter to Your Game Loop
Ensure your game loop calls the invalidate()
method frequently (video frames, logical updates, etc.), which will keep the FPS counter updated. You can integrate it easily with your existing game engine code.
public void gameLoop() {
while (gameRunning) {
updateGameLogic();
renderFrames();
fpsCounterView.invalidate(); // This triggers the FPS counter to update
}
}
Best Practices for Improving Game Performance
After measuring FPS, it is imperative to understand how to optimize it. Here are some strategies:
Optimize Graphics
- Texture Compression: Utilize tools like ETC1, PVRTC, or ASTC to compress textures. Compressed textures save memory and reduce the load on the GPU.
- Resolution Scaling: Consider rendering at a lower resolution and upscale. This approach can dramatically improve FPS while maintaining acceptable visual quality.
Manage Game Objects
- Object Pooling: Reusing objects instead of constantly allocating and deallocating can reduce lag and garbage collection overhead.
- Limit Draw Calls: Combine meshes and limit the number of materials to be drawn per frame to reduce GPU workload.
Tune Physics Calculations
Physics calculations can be resource-intensive. To optimize:
- Simplify Collisions: Use simpler shapes for collision detection rather than more complex meshes.
- Fixed Timestep: Reduce the frequency of updates. Run physics calculations at a fixed timestep that balances performance and gameplay needs.
To Wrap Things Up
Measuring FPS is an essential step in optimizing your Android game’s performance. With the FPS counter implemented, you can gain valuable insights into where improvements are needed. Combine that with best practices for graphics, object management, and physics, and you will not only boost FPS but also enhance the overall player experience.
Additional Resources
- Unity Documentation on Performance Optimization
- Graphics Performance Optimization Techniques
By regularly measuring and optimizing performance metrics like FPS, you create an enjoyable experience that keeps players engaged and coming back for more. Happy coding!