Overcoming Common Pitfalls in Apache POI Workbook Evaluation
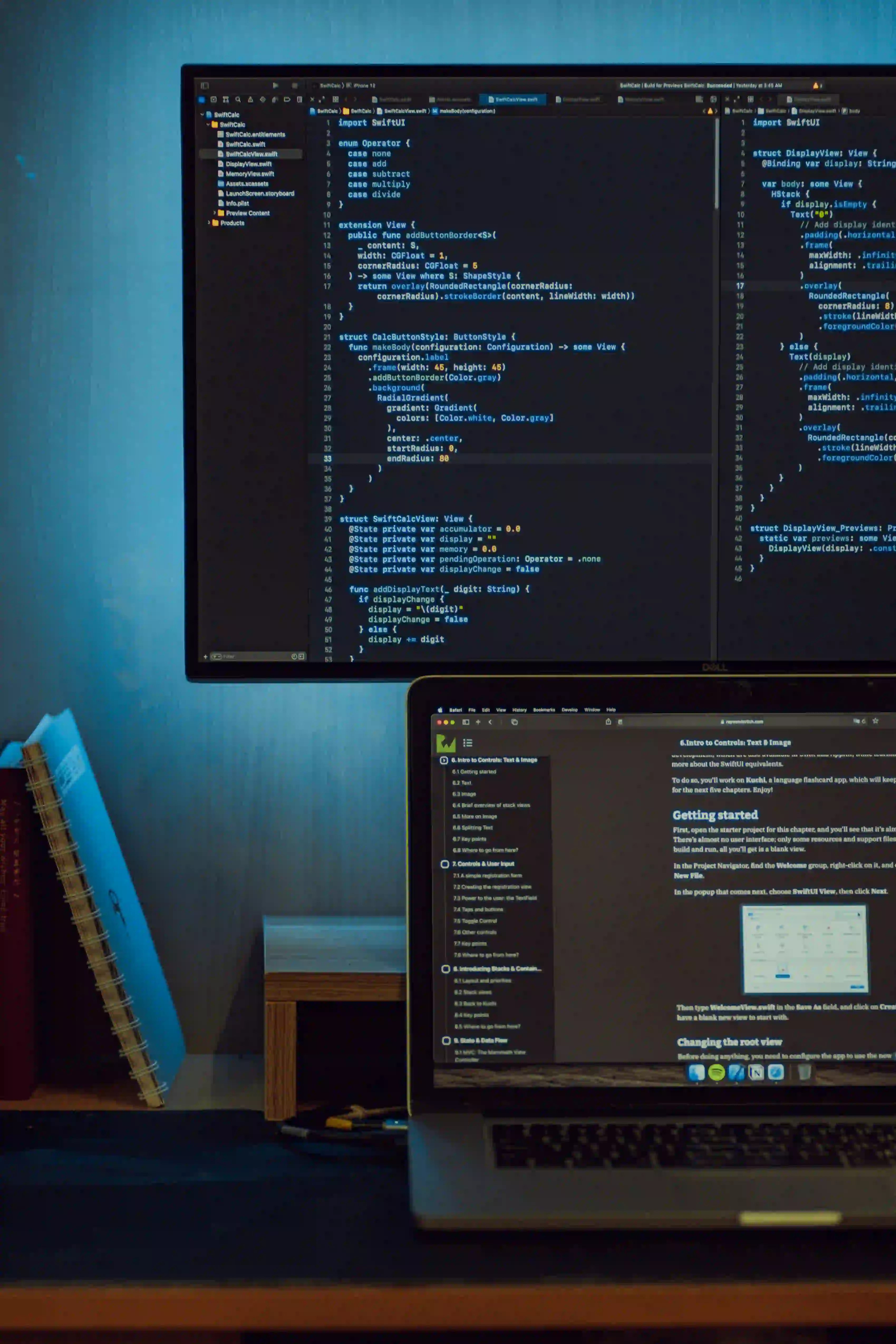
Overcoming Common Pitfalls in Apache POI Workbook Evaluation
Excel is one of the most widely used spreadsheet applications. For Java developers, the Apache POI library provides the ability to read, write, and manipulate Excel spreadsheets seamlessly. However, working with Apache POI can sometimes be challenging due to various pitfalls that inexperienced developers might encounter. In this blog post, we will dive into some of these common issues related to workbook evaluation and discuss effective strategies and methods to overcome them.
What is Apache POI?
Apache POI is an open-source Java library used for reading and writing Microsoft Office files, including Excel. It allows you to work with both .xls and .xlsx formats. For developers dealing with financial data, statistics, and report generation, Apache POI can serve as a powerful tool.
Why Use Apache POI?
- Flexibility: Apache POI supports both older and newer Excel file formats.
- Comprehensive Features: The library offers extensive APIs to manipulate worksheets, rows, and cells.
- No Dependencies: POI does not require a specific version of Excel installed on the client machine, making it ideal for server-side applications.
Common Pitfalls in Workbook Evaluation
Despite its robust functionality, several common pitfalls can complicate using Apache POI when evaluating workbooks. Below, we outline these problems and how to tackle them for smoother implementation.
1. Not Understanding Workbook Formats
Problem: Apache POI supports multiple Excel formats, including .xls
(HSSF) and .xlsx
(XSSF). The improper selection of Workbook type can lead to compatibility issues.
Solution: Always check the file extension before processing the Workbook. For instance:
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class WorkbookFactory {
public Workbook createWorkbook(String filePath) throws IOException {
FileInputStream file = new FileInputStream(filePath);
if (filePath.endsWith(".xlsx")) {
return new XSSFWorkbook(file);
} else if (filePath.endsWith(".xls")) {
return new HSSFWorkbook(file);
} else {
throw new IllegalArgumentException("The specified file is not an Excel file.");
}
}
}
Why: This code snippet dynamically selects the correct workbook type based on the file extension. This minimizes file read errors caused by mismatched formats.
2. Ignoring Cell Types
Problem: Cells in Excel can contain different data types (e.g., numeric, string, date). Failing to ascertain a cell's data type can cause runtime errors.
Solution: Always check the cell type before attempting to read its value:
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
public void readCells(Workbook workbook) {
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
for (Cell cell : row) {
switch (cell.getCellType()) {
case STRING:
System.out.println("String Value: " + cell.getStringCellValue());
break;
case NUMERIC:
System.out.println("Numeric Value: " + cell.getNumericCellValue());
break;
case BOOLEAN:
System.out.println("Boolean Value: " + cell.getBooleanCellValue());
break;
default:
System.out.println("Unknown Cell Type");
}
}
}
}
Why: This approach ensures that you handle each cell appropriately, reducing the chances of exceptions and enhancing data integrity during evaluation.
3. Memory Management
Problem: Large Excel files can consume a significant amount of memory during processing, leading to OutOfMemoryError
.
Solution: Use the SXSSF
(Streaming Usermodel API) for large files. It writes data to disk instead of keeping everything in memory:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class LargeExcelWriter {
public void writeLargeExcel(String filePath) throws IOException {
Workbook workbook = new SXSSFWorkbook(); // Use SXSSFWorkbook for large datasets
Sheet sheet = workbook.createSheet("Large Data");
for (int i = 0; i < 100000; i++) {
Row row = sheet.createRow(i);
Cell cell = row.createCell(0);
cell.setCellValue("Row " + i);
}
try (FileOutputStream out = new FileOutputStream(filePath)) {
workbook.write(out);
}
((SXSSFWorkbook) workbook).dispose(); // Dispose of temporary files backing the workbook
}
}
Why: Leveraging SXSSF
helps manage memory usage efficiently, which is essential for applications dealing with large volumes of data.
4. Poor Error Handling
Problem: Neglecting to implement comprehensive error handling can make debugging issues in your application difficult.
Solution: Implement detailed error catching and exit strategies. Here is a basic example:
import org.apache.poi.ss.usermodel.Workbook;
public class WorkbookReader {
public void readWorkbook(String filePath) {
try {
Workbook workbook = createWorkbook(filePath);
readCells(workbook);
workbook.close();
} catch (IOException e) {
System.err.println("Error reading the Excel file: " + e.getMessage());
} catch (IllegalArgumentException e) {
System.err.println("Invalid file format: " + e.getMessage());
}
}
}
Why: Proper error handling not only prevents crashes but gives you meaningful feedback on what went wrong during the processing.
5. Not Optimizing Performance
Problem: Inefficient algorithms for processing cells, rows, or sheets can lead to long execution times, especially in large datasets.
Solution: Use optimizations like batch processing or parallel processing when evaluating large workbooks. For example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelCellReader {
public void readLargeWorkbook(Workbook workbook) {
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < workbook.getNumberOfSheets(); i++) {
final int sheetIndex = i;
executor.submit(() -> readCells(workbook.getSheetAt(sheetIndex)));
}
executor.shutdown();
}
}
Why: By distributing workloads among multiple threads, you can cut down on processing time, making your application more efficient.
A Final Look
Navigating the intricacies of Apache POI for workbook evaluation is crucial for building robust applications that manipulate Excel data. Awareness of the common pitfalls discussed above will empower you to write cleaner, safer, and more efficient code.
Whether you're new to Apache POI or have some experience, understanding these principles will enhance your productivity and help you avoid unnecessary headaches down the line.
For more advanced topics on Apache POI, consider exploring Apache POI Documentation for extensive resources, or check Java Excel API for practical insights into Excel file manipulation.
Now that you’re equipped with strategies to overcome common pitfalls make the most of this powerful library, and continue to refine your skills. Happy coding!