Overcoming Common SSHJ Connection Issues
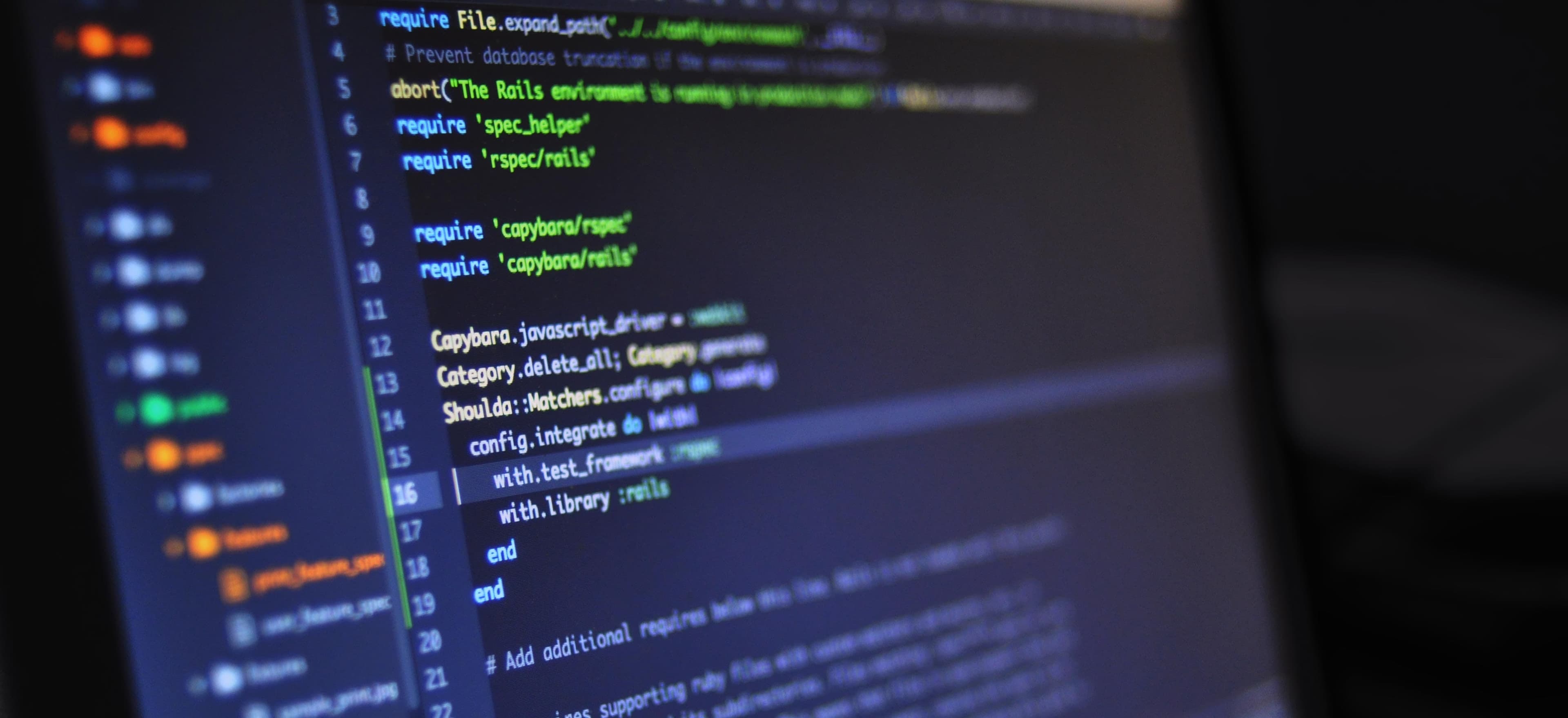
- Published on
Overcoming Common SSHJ Connection Issues
Secure Shell (SSH) is a widely used protocol that allows secure remote access to servers. In Java, SSHJ is a popular library for handling SSH connections. However, developers often encounter connection issues when using SSHJ. This post will explore common SSHJ connection problems, their potential causes, and how to resolve them.
Table of Contents
- Understanding SSHJ
- Common SSHJ Connection Issues
- Authentication Errors
- Timeout Issues
- Host Key Verification Issues
- Debugging Connection Issues
- Example Code Snippets
- Conclusion
Understanding SSHJ
Before diving into the common issues, let's briefly understand what SSHJ is. SSHJ is an open-source Java library designed for SSH and SFTP protocols. It supports many advanced features, including asynchronous operations, public key authentication, and more. This library is not just reliable; it’s also highly configurable and easy to use.
For more information, you can check out the SSHJ GitHub repository.
Common SSHJ Connection Issues
1. Authentication Errors
Authentication is a crucial part of establishing an SSH connection. If authentication fails, you will be unable to connect, despite having the correct SSH host information.
Common Causes:
- Incorrect username or password.
- Missing private key files or wrong passphrase.
- The SSH server may not allow certain authentication methods.
Solution: Double-check your credentials and ensure that the server allows the authentication type you are using. You can also specify multiple authentication methods to improve the chances of a successful connection.
2. Timeout Issues
Timeout occurs when an SSH connection attempt takes too long, often due to network issues or server unavailability.
Common Causes:
- Network connectivity issues.
- The SSH server is not responsive.
- Firewall configurations blocking the connection.
Solution: You might need to check your internet connection and the state of the remote server. Also, consider increasing the timeout setting in your SSHJ connection configuration.
3. Host Key Verification Issues
To prevent Man-in-the-Middle (MitM) attacks, SSH requires host key verification. Any mismatch in keys or if the key is not recognized can lead to connection refusal.
Common Causes:
- The host key has changed since the last connection.
- The host key is not in known hosts.
Solution: Update your known hosts file or adjust your key verification settings to ignore unknown hosts (not recommended for production use).
Debugging Connection Issues
When things go wrong, debugging is essential. SSHJ provides a way to enable verbose logging so you can identify where the connection is failing.
Here’s how you can enable logging in SSHJ:
import net.schmizz.sshj.common.LoggerFactory;
import net.schmizz.sshj.common.SSHJLogger;
Logger logger = LoggerFactory.getLogger(SSHJLogger.class);
logger.setLevel(java.util.logging.Level.ALL);
By increasing the logging level, you can see detailed output regarding the SSH connection attempts. These logs can be helpful for diagnosing issues.
Example Code Snippets
Let’s look at an example of how to establish an SSH connection using SSHJ. This example will also include connection options to handle some of the common issues we discussed.
Basic SSH Connection
import net.schmizz.sshj.SSHClient;
import net.schmizz.sshj.connection.channel.direct.Session;
import net.schmizz.sshj.userauth.password.PasswordAuth;
import net.schmizz.sshj.userauth.keyprovider.SimpleGeneratorHostKeyProvider;
import java.io.IOException;
public class SSHConnectionExample {
public static void main(String[] args) {
SSHClient ssh = new SSHClient();
// Set up logging
Logger logger = LoggerFactory.getLogger(SSHJLogger.class);
logger.setLevel(java.util.logging.Level.ALL);
try {
ssh.addHostKeyVerifier((h, p, k) -> true); // Insecure - only for testing
ssh.connect("your.ssh.server.com");
ssh.authPublickey("username", new FilePrivateKeyProvider());
// Or use Password Authentication
// ssh.authPassword("username", "password");
// Open a session
final Session session = ssh.startSession();
session.exec("your-command");
session.close();
} catch (IOException e) {
logger.severe("Connection failed: " + e.getMessage());
} finally {
ssh.disconnect();
}
}
}
Commentary
-
Host Key Verifier: In this example, we allow all host keys. While this helps during development, you should validate host keys in production to enhance security.
-
Authentication Methods: Choose an authentication method that fits your security needs. Password authentication is simpler but can be less secure than public key authentication.
-
Error Handling: Always implement error handling to manage exceptions better. Logging connection issues provides insights into failures.
My Closing Thoughts on the Matter
Overcoming common SSHJ connection issues requires a mix of debugging techniques, understanding potential pitfalls, and implementing best practices. Authentication errors, timeout issues, and host key verification complications can all disrupt your connection attempts, but with the right strategies, these challenges can be effectively managed.
Remember to leverage logging for debugging, validate host keys for security, and test various configurations where necessary. SSHJ provides a robust framework, so being informed about its capabilities can save you time and improve your development experience.
To dive deeper and enhance your SSHJ skills, explore the SSHJ GitHub repository and its documentation.
For further reading on SSH and secure remote connections, check out resources like DigitalOcean's tutorial on using SSH and SSHv2 Protocol Overview.
This knowledge will not only help you overcome current challenges but will also equip you with the best practices for secure communications in your Java applications. Happy coding!
Checkout our other articles