Mastering Docker: Sending Multiple Commands in One Run
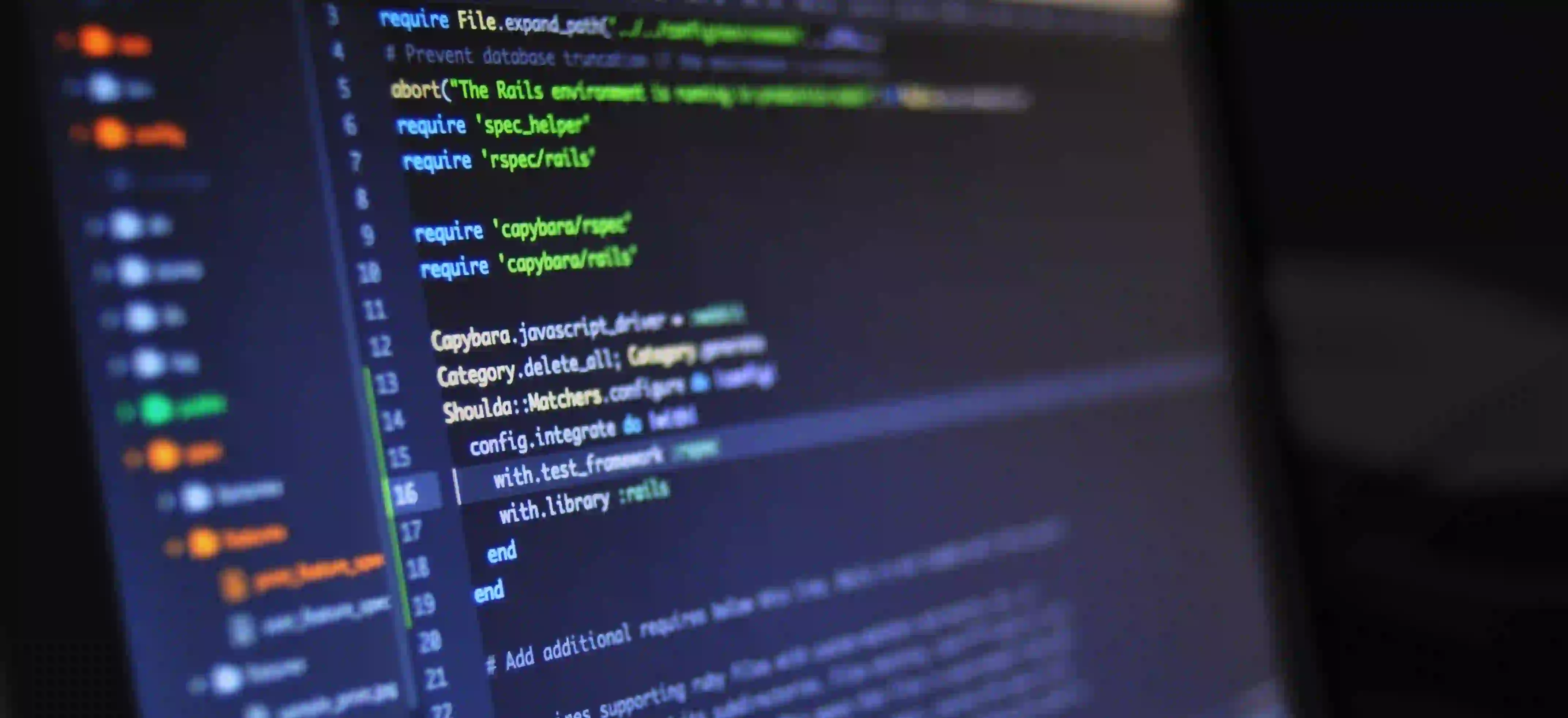
Mastering Docker: Sending Multiple Commands in One Run
In the ever-evolving landscape of software development, Docker has emerged as a crucial tool for efficiently managing application deployment. It simplifies environments, allows for easy scalability, and ensures consistency across different stages of development. A common requirement when working with Docker is the ability to execute multiple commands in a single run. This post delves into how to achieve that while maintaining clarity and efficiency.
Why Use Docker?
Before we dive deep into running multiple commands, let's understand why Docker is popular:
- Environment Consistency: Docker containers ensure your application runs the same regardless of the environment.
- Isolation: Each container runs in isolation, securing your applications and their dependencies.
- Scalability: Easily scale your application by adding or removing containers at runtime.
Now that we've established the importance of Docker, let's explore how to run multiple commands in one go.
The Basics of Docker Commands
Docker commands are primarily executed through the docker run
command, which launches a new container. You can pass commands to be executed inside this container. Let’s take a look at the standard syntax:
docker run <image-name> <command>
However, if you want to run multiple commands, things can get a bit tricky.
Running Multiple Commands with Docker
One common approach to executing multiple commands within a single container instance is to use a shell form. This approach executes the commands sequentially in a single shell environment.
Example of Running Commands Sequentially
docker run ubuntu bash -c "echo 'Updating package list' && apt-get update && echo 'Installing nginx' && apt-get install -y nginx"
Breakdown of the Command:
docker run ubuntu
: This tells Docker to run a new container using the Ubuntu image.bash -c
: This indicates that we want to run a command in the Bash shell."echo '...' && ..."
: These commands are chained using&&
, ensuring that each command is executed in order only if the preceding command succeeds.
Why the Use of &&
?
Using &&
between commands allows you to control the flow of execution. This means:
- If any command fails, the subsequent command in the chain will not execute.
- This is particularly useful for ensuring that critical setup steps do not proceed if previous steps weren’t successful.
Running Commands in Parallel
Sometimes you may want to run multiple commands in parallel. For that, you can use &
instead of &&
. This allows you to send multiple commands to run concurrently.
docker run ubuntu bash -c "echo 'Updating package list' & apt-get update & echo 'Installing nginx' & apt-get install -y nginx"
Why Use &
?
Using &
allows for asynchronous execution. However, be cautious when running commands this way, as it doesn't prevent later commands from executing if earlier ones fail.
Practical Example
Let's consider a scenario where you need to install multiple packages and set up configurations. Running these commands sequentially is essential to ensure proper installation.
docker run ubuntu bash -c "apt-get update && apt-get install -y nginx git curl && git clone https://github.com/some/repo.git"
In-depth Breakdown:
apt-get update
: Update the package list.apt-get install -y nginx git curl
: Install multiple packages at once.git clone https://github.com/some/repo.git
: Clone a repository after ensuring git is installed.
Using Dockerfile for Repeated Commands
For scenarios where you frequently need to run a set of commands, consider using a Dockerfile. This is particularly advantageous for creating an image that includes all dependencies.
Here’s a basic example of a Dockerfile:
# Use the official Ubuntu image
FROM ubuntu:latest
# Set environment variables
ENV DEBIAN_FRONTEND=noninteractive
# Update package list and install packages
RUN apt-get update && apt-get install -y nginx git curl
# Clone the repository
RUN git clone https://github.com/some/repo.git /app
# Expose default nginx port
EXPOSE 80
# Command to run when starting the container
CMD ["nginx", "-g", "daemon off;"]
Why Use a Dockerfile?
- Repeatability: A Dockerfile lets you recreate the exact same environment.
- Layering: Docker builds layers, so unchanged steps are cached; next builds are faster.
- Version Control: Keep your Dockerfile in version control for easier team collaboration and updates.
Reducing Image Size with Multi-Step Builds
When your Dockerfile contains various commands, it may lead to a larger image size. To overcome this, Docker supports multi-step builds which can vastly diminish the size of your output image.
# Step 1: Build
FROM node:14 AS build
WORKDIR /app
COPY . .
RUN npm install && npm run build
# Step 2: Serve
FROM nginx:alpine
COPY --from=build /app/build /usr/share/nginx/html
Explanation:
- Build Stage: The first stage (node:14) builds the application.
- Serve Stage: The second stage (nginx:alpine) takes only the necessary build artifacts, keeping the runtime image lightweight.
Key Takeaways
Mastering Docker involves understanding how to efficiently send commands and configure images. Running multiple commands can simplify your setup process, but using a structured approach like a Dockerfile will facilitate reproducibility and maintainability.
For more in-depth Docker command guides, check out Docker Documentation.
This powerful containerization technology is a big leap towards efficient development workflows. As you explore Docker further, remember that mastery comes with hands-on practice. Happy Dockering!