Java 8 Project Jigsaw: Unpacking Module System Challenges
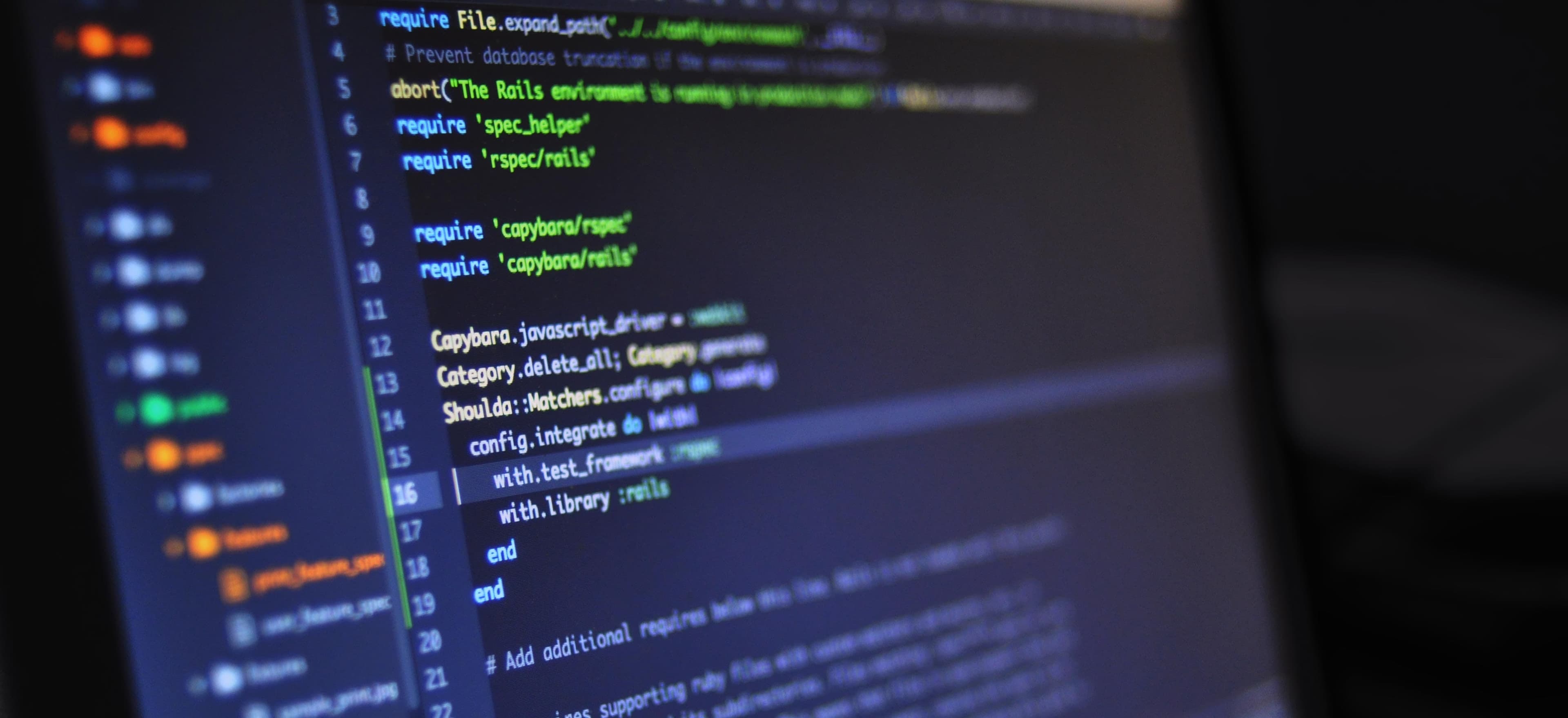
- Published on
Java 8 Project Jigsaw: Unpacking Module System Challenges
Java has evolved significantly since its inception. Among the myriad of enhancements, Java 8 brought forth Project Jigsaw, paving the way for a robust module system. The transition from a monolithic structure to a modular architecture has far-reaching implications for developers, maintainers, and the Java ecosystem as a whole. In this post, we will delve deep into the challenges and solutions tied to the Java Module System introduced in Project Jigsaw.
Understanding Project Jigsaw
Project Jigsaw was a major effort aimed at modularizing the Java platform itself. This initiative facilitates the creation of scalable Java applications by allowing developers to encapsulate packages within modules. The main objectives include:
- Improved security: By hiding internal APIs, the risk related to unintended dependency usage is minimized.
- Better performance: Modularization reduces the footprint of applications and can lead to faster class loading times.
- Easier maintenance: The clear separation of concerns leads to simpler version management and clearer module dependencies.
For a deeper understanding, you can explore the Project Jigsaw official documentation .
The Challenges of Adopting a Module System
While the benefits are clear, challenges abound. Let's discuss some of these hurdles:
1. Complex Transition from Legacy Code
Transitioning from a traditional JAR-based structure to a module-based framework can be daunting. Legacy code often doesn't follow the modular design principles required by Jigsaw.
Example:
module org.example.myapp {
exports org.example.myapp.main;
requires org.example.utils;
}
The above snippet shows the declaration of a module. Unfortunately, attempting to modularize existing projects may lead to missing requirements or conflicts due to non-modular practices.
Why this matters:
Understanding the existing structure is crucial. A comprehensive audit of your codebase can result in a smooth transition, enabling you to define clear entry points and dependencies.
2. Dependency Management
Jigsaw changes the way dependencies are handled. With a module system in place, all dependencies must be declared properly. This may result in discovering untracked dependencies in legacy systems.
To exemplify this:
module org.example.myapp {
requires java.sql; // Explicitly requires the SQL module
}
Neglecting to declare required modules can lead to runtime errors, complicating the build and deployment processes.
3. Access Restrictions
Modules strictly define the scope of accessibility. This leads to complications when attempting to access internal APIs.
Example of access control:
module org.example.utils {
exports org.example.utils.api;
// Other packages are not exported
}
Why this is crucial:
Access restrictions can complicate refactoring and integration efforts, particularly if the system relies heavily on internal APIs.
4. Tooling and Build Systems
Not all tools and build systems fully support the module system introduced by Jigsaw. This may require updates to build scripts and configurations.
For instance, if one were using Maven, transitioning to modules requires changes to the pom.xml
file:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example.myapp</groupId>
<artifactId>myapp-module</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.example.utils</groupId>
<artifactId>utils-module</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
5. Learning Curve
Understanding the Module Layer and its implications can require time and trial-and-error. Developers may need training to reorient their mindsets towards modular application design.
Best Practices for Implementing the Java Module System
To journey through these challenges, consider the following best practices:
1. Audit Legacy Code
Before diving into the migration, perform a thorough analysis of your existing codebase. Identify which sections can be modularized and which require significant rewriting.
2. Define Clear Module Boundaries
When creating a module, define clear responsibilities. Each module should encapsulate functionality that can be reused across various projects.
3. Utilize Automated Tools for Transformation
Consider using tools like JDeps (Java Dependency Analyzer) that can assist in identifying dependencies and managing module paths effectively.
4. Gradual Transition
It’s often more practical to convert existing applications in phases. Start with smaller modules and incrementally refactor bigger pieces of your application.
5. Keep Documentation Updated
Maintain adequate documentation throughout the transition. It should reflect the newly adopted modular architecture, which will serve as a guide for future developers.
My Closing Thoughts on the Matter
Project Jigsaw, by introducing a modular system, redefined the paradigm of Java application development. Yet, the path isn’t without challenges. From understanding legacy systems to managing dependencies and ensuring access control, developers must embrace a proactive, thoughtful approach.
By adhering to the best practices outlined above and relying on community resources, developers can navigate these hurdles successfully. The shift toward a modular architecture opens up a serene future for Java, facilitating the development of cleaner, more efficient, and maintainable applications.
To stay updated on Jigsaw and Java development best practices, explore communities like Stack Overflow and the Java subreddit.
As you embark on this journey, remember: every challenge presents an opportunity for growth. Embrace the modular revolution!