Unlocking Java: Finding the First Duplicate Index in Arrays
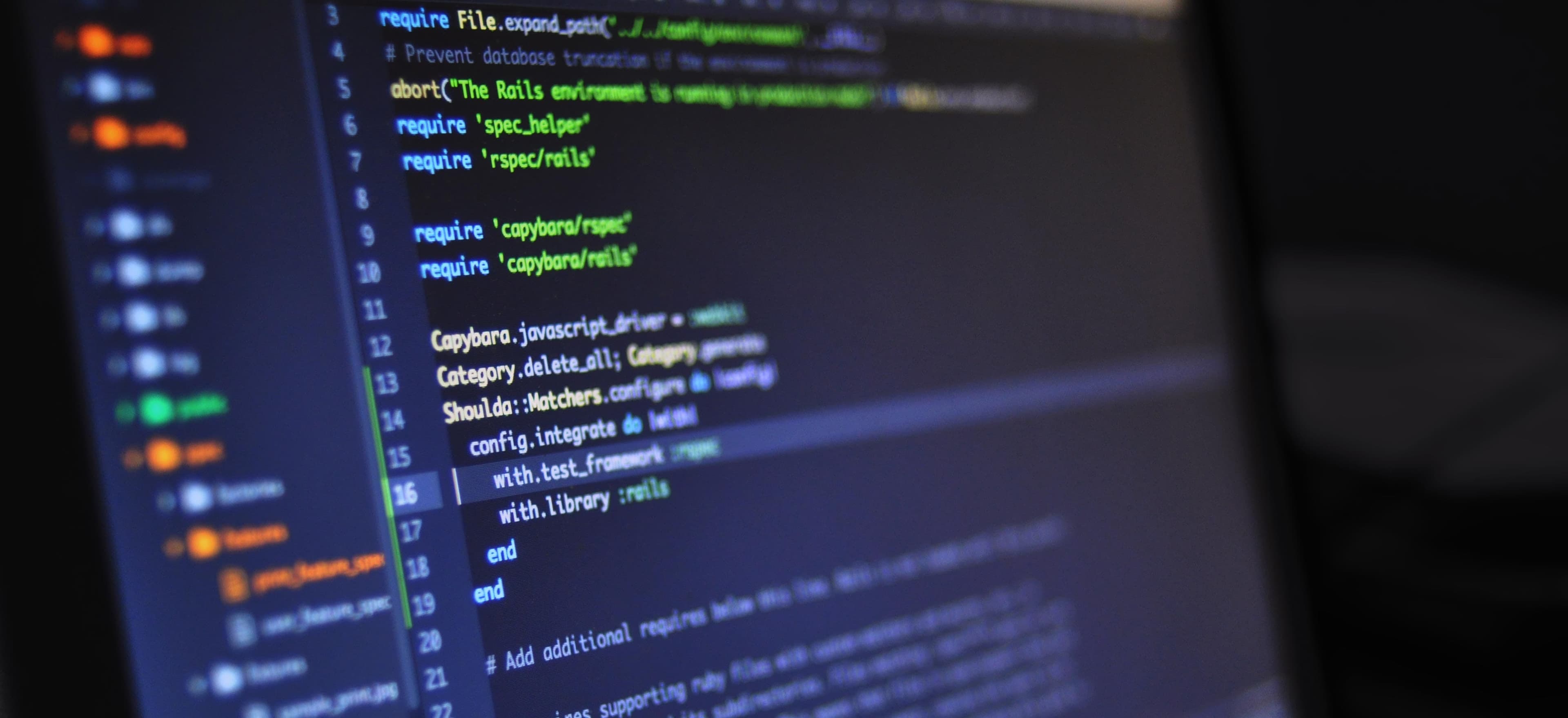
- Published on
Unlocking Java: Finding the First Duplicate Index in Arrays
Java is a robust and versatile programming language cherished by developers worldwide. One of the common challenges in software development is efficiently finding duplicate values within collections, such as arrays. In this blog post, we will not only tackle how to find the first duplicate index in an array but also delve into the reasoning behind our approach, optimizing for speed and clarity.
Understanding the Problem Statement
Given an array of integers, our objective is to identify the first duplicate element and its index. For example, consider the array:
int[] numbers = {3, 5, 1, 3, 4, 2};
In this case, the first duplicate number is 3
, which appears at index 0
and again at index 3
. Hence, the output should be an integer representing the index of the duplicate, which is 3
.
Approaches to Solve the Problem
There are various methods to tackle this problem:
- Brute Force Approach: Check each element against others.
- Hashing: Utilize a data structure like a HashSet to track seen elements.
- Sorting: Sort the array and check adjacent elements.
While the brute force method can be considered straightforward, it is inefficient for larger arrays, giving rise to O(n^2) complexity. Instead, we will focus on the hashing approach due to its average time complexity of O(n).
The Hashing Approach
Why Hashing?
Hashing allows us to keep track of the numbers we have seen while iterating through the array. By leveraging a HashSet, we can easily check whether a number is already present and thus find the first duplicate efficiently.
Implementation
Here’s a step-by-step breakdown of our approach:
- Initialize a HashSet to store unique elements as we iterate.
- Loop Through the Array: For each element, check if it exists in the HashSet.
- On Finding a Duplicate: Immediately return the current index of that duplicate.
Below is the Java implementation of this approach:
import java.util.HashSet;
public class FindFirstDuplicate {
public static Integer firstDuplicateIndex(int[] array) {
HashSet<Integer> seen = new HashSet<>();
for (int currentIndex = 0; currentIndex < array.length; currentIndex++) {
int currentElement = array[currentIndex];
// Check if the current element was addressed before
if (seen.contains(currentElement)) {
return currentIndex; // Return the index of the first duplicate found
} else {
seen.add(currentElement); // Add the current element to the HashSet
}
}
return null; // No duplicates found
}
public static void main(String[] args) {
int[] numbers = {3, 5, 1, 3, 4, 2};
Integer duplicateIndex = firstDuplicateIndex(numbers);
if (duplicateIndex != null) {
System.out.println("The first duplicate index is: " + duplicateIndex);
} else {
System.out.println("No duplicates found.");
}
}
}
Code Commentary
-
HashSet Initialization: We start by creating a HashSet called
seen
to store unique elements. This allows for O(1) average time complexity when checking if an element exists. -
The Loop: The
for
loop iterates over the array. For every element, we check if it has already been encountered usingseen.contains(currentElement)
. -
Found a Duplicate: If the current element is found in
seen
, this indicates the first duplicate. The function returns the current index. -
Adding Elements: If it’s not a duplicate, we add the element to the HashSet for future reference.
Efficiency
The algorithm runs in O(n) time due to the single pass through the array, making it efficient for large data sets. The space complexity is also O(n), as we store each unique element in the HashSet.
Testing the Implementation
Let’s consider more diverse cases to validate our solution:
int[] test1 = {4, 2, 3, 4, 5}; // Expected output: 3
int[] test2 = {1, 2, 3, 4}; // Expected output: null (no duplicates)
int[] test3 = {7, 8, 8, 9, 9}; // Expected output: 2
int[] test4 = {10, 11, 12, 13}; // Expected output: null (no duplicates)
System.out.println(firstDuplicateIndex(test1)); // 3
System.out.println(firstDuplicateIndex(test2)); // null
System.out.println(firstDuplicateIndex(test3)); // 2
System.out.println(firstDuplicateIndex(test4)); // null
Why Testing Matters
Testing with various input sizes and conditions (like arrays without duplicates) ensures our function behaves as expected under different scenarios. This is crucial in software development to maintain robustness and reliability.
Closing the Chapter
In this post, we explored how to find the first duplicate index in an array using a HashSet for efficient lookup. The O(n) time complexity makes the solution scalable for larger datasets.
For further reading on Java collections and data structures, check out the Java Collections Framework and Set Interface Documentation.
Embrace the power of efficient coding practices, and you'll elevate your software development skills to new heights! Happy coding!
Checkout our other articles