Why Unit Testing Often Misses the Mark on Value
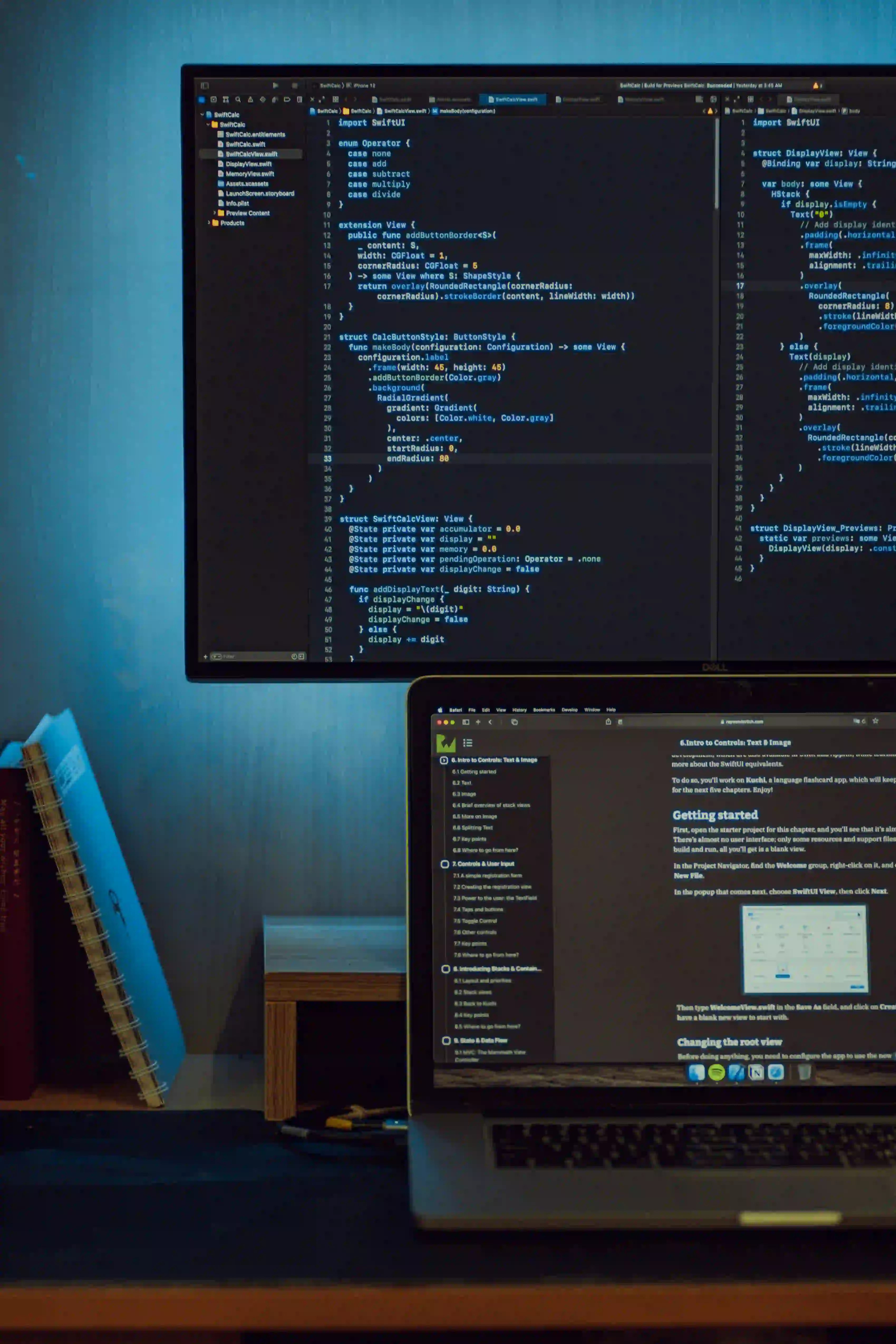
Why Unit Testing Often Misses the Mark on Value
In the journey of software development, unit testing holds a pivotal role. Unit tests are designed to validate individual components of your code, ensuring that each unit behaves as expected. They provide a safety net for developers as they write new functionalities. However, many developers and teams discover that unit testing often falls short of delivering the expected value. This blog explores several reasons why unit testing may not deliver its promised benefits, along with best practices to bridge that gap.
The Importance of Unit Testing
Before diving into the downsides, let’s briefly discuss why unit testing is important. Effective unit testing can lead to several benefits:
- Immediate Feedback: Developers receive rapid feedback on their code.
- Improved Code Quality: Tests help identify bugs and edge cases early in development.
- Documentation: Tests serve as living documentation of code behavior.
- Facilitates Refactoring: Developers can confidently refactor code knowing there are tests that will catch errors.
Despite these advantages, many teams experience dissatisfaction with their unit testing practices.
Reasons Unit Testing Often Misses the Mark
1. Poorly Designed Tests
One of the chief pitfalls of unit testing is poorly designed tests. Tests should be simple, focusing on a specific functionality. However, many developers write extensive tests that cover multiple concerns simultaneously.
Example of Poor Test Design
@Test
public void testCalculateTotalAmount() {
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("Apple", 1.0), 2);
cart.addItem(new Item("Banana", 0.5), 3);
double total = cart.calculateTotalAmount();
assertEquals(3.5, total, 0.01);
}
Why It’s Poorly Designed: This test checks multiple functionalities - adding items and calculating totals. If it fails, you won’t know which aspect is the problem (adding or calculating).
2. Lack of Isolation from Other Units
Unit tests must isolate the unit under test. If tests are dependent on external systems or incomplete states (like databases or APIs), reliability is compromised.
Example of Interdependent Tests
@Test
public void testProcessOrder() {
OrderProcessor processor = new OrderProcessor();
processor.processOrder(orderId);
// Assumes external service updates state.
assertTrue(database.isOrderProcessed(orderId));
}
The Problem: This test depends on an external database state. A failure could be due to an issue with the database rather than the order processing logic itself.
3. Overemphasis on Coverage
Many development teams place undue emphasis on code coverage metrics. While high coverage is generally a sign of thorough testing, it often leads to a false sense of security. A 100% coverage rate doesn’t guarantee that all code paths are tested effectively.
Example of Coverage Not Equaling Quality
public void processPayment(Payment payment) {
if (payment.isValid()) {
// process payment
}
}
Testing the above code simply for coverage might result in the following shallow test:
@Test
public void testProcessPayment() {
Payment payment = new Payment();
payment.setValid(true);
processor.processPayment(payment); // What about invalid payments?
}
Why It Fails: This test might give you a coverage score, but it doesn’t validate the important paths where payment is invalid.
4. Tests that Lack Context
Unit tests need context about the business requirements. Tests should reflect the user stories or business cases that dictate how the code should behave.
Example Lacking Context
@Test
public void testUserLogin() {
User user = new User();
user.setUsername("testUser");
user.setPassword("12345");
assertTrue(authenticationService.login(user));
}
The Shortcoming: This test checks the login process but lacks context about allowed usernames, password complexity, or failed login attempts.
5. Ignoring Edge Cases
A test suite that neglects edge cases is setting itself up for failure. Real-world use cases often present edge conditions. Well-designed tests should cover these scenarios.
Example Missing Edge Cases
@Test
public void testGetDiscount() {
Cart cart = new Cart();
cart.addItem(new Item("Book", 20.0));
double discount = cart.getDiscount();
assertEquals(0, discount, 0.01); // No discount for a single item.
}
The Flaw: There’s no test for scenarios like discounts on bulk purchases or giving discounts on specific items, which are crucial in a real-world scenario.
6. Neglecting Maintenance
Unit tests require maintenance, just like the code they test. As code evolves, tests also need to be updated. A common oversight is neglecting to refactor tests or removing outdated tests.
Impact of Neglected Maintenance
When tests grow stale and no longer reflect the functionality, they can become brittle. When stale tests break, it often leads to confusion about functionality rather than actual bugs in the code.
7. Focusing Solely on Unit Tests
Unit tests are a key aspect of a comprehensive testing strategy. However, they are not sufficient on their own. Integration testing, system testing, and other levels of testing are critical to achieve complete coverage.
Best Practices to Maximize Unit Testing Value
To mitigate the issues highlighted above and maximize the value derived from unit testing, consider the following best practices:
- Single Responsibility Tests: Each unit test should focus on a single functionality.
- Isolate Units: Ensure that tests do not rely on external systems or states.
- Prioritize Coverage Quality: Focus on covering the most critical paths and edge cases rather than a blanket approach to coverage.
- Keep Business Context in Mind: Write tests reflecting actual user stories and requirements.
- Cover Edge Cases: Don’t overlook tests for boundary conditions.
- Regularly Maintain Tests: Treat tests as first-class citizens and maintain them just like main code.
- Adopt a Comprehensive Strategy: Incorporate unit tests as part of a larger testing strategy that includes integration and system tests.
Wrapping Up
While unit testing remains a cornerstone of modern software development, it is essential to recognize its limitations. By understanding the common pitfalls and adhering to best practices, teams can elevate the effectiveness of their unit tests. Ultimately, well-crafted unit tests bolster code quality, improve maintainability, and significantly enhance the overall value derived from your software projects.
For further reading, check out the following resources:
- The Importance of Unit Testing
- Effective Unit Testing: A Guide
- Unit Testing Best Practices
By fostering a culture that appreciates the role and importance of unit testing, you can ensure more robust software solutions and a more agile development process.