How to Avoid Charset Issues in Java Applications
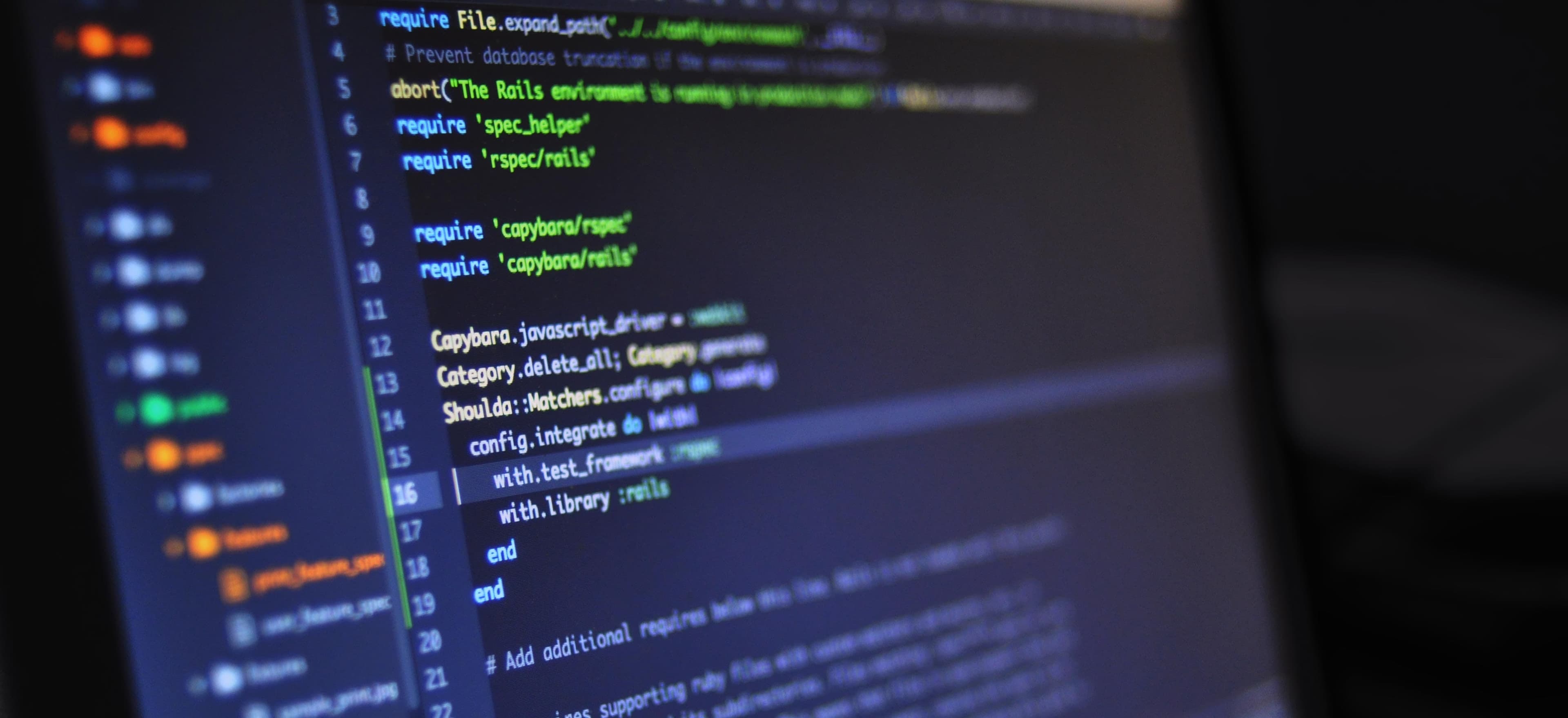
- Published on
How to Avoid Charset Issues in Java Applications
Character encoding is a fundamental concept in programming, especially in multi-language support and data interchange. Unfortunately, charset issues can lead to bugs that are difficult to trace, especially in Java applications. This blog post delves into understanding charset issues, and how to avoid them in your Java projects.
Understanding Character Encoding
Character encoding is a system that pairs each character in a given repertoire with a value (such as a number) that can be represented in a computer. The most common character encodings are UTF-8, UTF-16, and ISO-8859-1. Each encoding has its advantages and may support different sets of characters.
Java's Charset
class provides methods to handle different character encodings. The StandardCharsets
class contains some common charsets like UTF-8, UTF-16, etc.
Why Charset Issues Occur
Charset issues generally arise when your application processes data in multiple formats. For instance, reading from a file encoded in UTF-8 while using an ISO-8859-1 reader can lead to incorrect character representation. Errors often manifest as question marks (�) or other garbled outputs.
Common Scenarios Leading to Charset Issues
Here are some scenarios that may lead to charset issues in Java applications:
- File I/O: When files are read or written without specifying the correct charset.
- Network Protocols: Data sent over networks must explicitly use the correct charset.
- APIs and Web Services: Receiving or sending data without specifying the charset can lead to issues.
Best Practices to Avoid Charset Issues
1. Always Specify the Charset
When working with file I/O or network communications, explicitly specify the charset rather than relying on the default.
Example: Reading a file in UTF-8
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.charset.StandardCharsets;
public class CharsetExample {
public static void main(String[] args) {
try {
String content = new String(Files.readAllBytes(Paths.get("example.txt")), StandardCharsets.UTF_8);
System.out.println(content);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why: By specifying StandardCharsets.UTF_8
, you ensure that the text is read correctly regardless of system defaults.
2. Use the Built-in Charset Support
Java’s Charset
class allows you to convert between different charsets seamlessly.
Example: Converting a string from UTF-8 to ISO-8859-1
import java.nio.charset.Charset;
public class CharsetConversion {
public static void main(String[] args) {
String original = "Example String";
byte[] utf8Bytes = original.getBytes(Charset.forName("UTF-8"));
String converted = new String(utf8Bytes, Charset.forName("ISO-8859-1"));
System.out.println(converted);
}
}
Why: Using Java's built-in capacities for conversion helps prevent manual errors in charset management.
3. Set the Default Charset Wisely
Changing the default charset of your Java application can help if most files share a common encoding.
import java.nio.charset.Charset;
public class SetDefaultCharset {
public static void main(String[] args) {
System.setProperty("file.encoding", "UTF-8");
System.out.println("Default Charset: " + Charset.defaultCharset());
}
}
Why: By specifying a sensible default for the entire application, you can minimize charset mismatches across various components.
4. Use URI Encoding for URLs
When transmitting data in URL queries, use UTF-8 encoding to avoid issues with special characters.
Example: Encoding a parameter for a URL
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class URLEncodeExample {
public static void main(String[] args) {
try {
String original = "Hello World! こんにちは";
String encoded = URLEncoder.encode(original, "UTF-8");
System.out.println(encoded);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
Why: This ensures that special characters are properly transmitted, avoiding issues related to incorrect character parsing.
5. Handle Charset at API Boundaries
Ensure that APIs you develop or consume specify the charset in HTTP headers.
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpHeaderExample {
public static void main(String[] args) throws Exception {
HttpURLConnection connection = (HttpURLConnection) new URL("http://example.com").openConnection();
connection.setRequestProperty("Accept-Charset", "UTF-8");
connection.connect();
// Handle response
String response = new String(connection.getInputStream().readAllBytes(), StandardCharsets.UTF_8);
System.out.println(response);
}
}
Why: By setting the Accept-Charset in requests, you ensure that the server responds with the appropriate character encoding.
6. Testing for Charset Issues
Testing against various encodings can help you catch charset issues before deployment.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CharsetTest {
@Test
public void testCharset() throws UnsupportedEncodingException {
String testString = "Hello, こんにちは";
byte[] bytes = testString.getBytes("UTF-8");
String newString = new String(bytes, "UTF-8");
assertEquals(testString, newString);
}
}
Why: Testing ensures that your applications validate and maintain integrity across character sets.
Final Considerations
Dealing with character encoding can be challenging, but understanding the potential pitfalls and adhering to best practices can significantly mitigate charset issues in your Java applications. Always specify the charset explicitly, handle conversions correctly, and ensure testing is thorough.
For more advanced reading on character encodings, check out Java's official documentation on StandardCharsets and Character Encoding in Java.
By following these best practices, you can develop robust Java applications that handle character encoding seamlessly across different platforms and locales.