Mastering Android Multi-Touch: Common Gesture Pitfalls
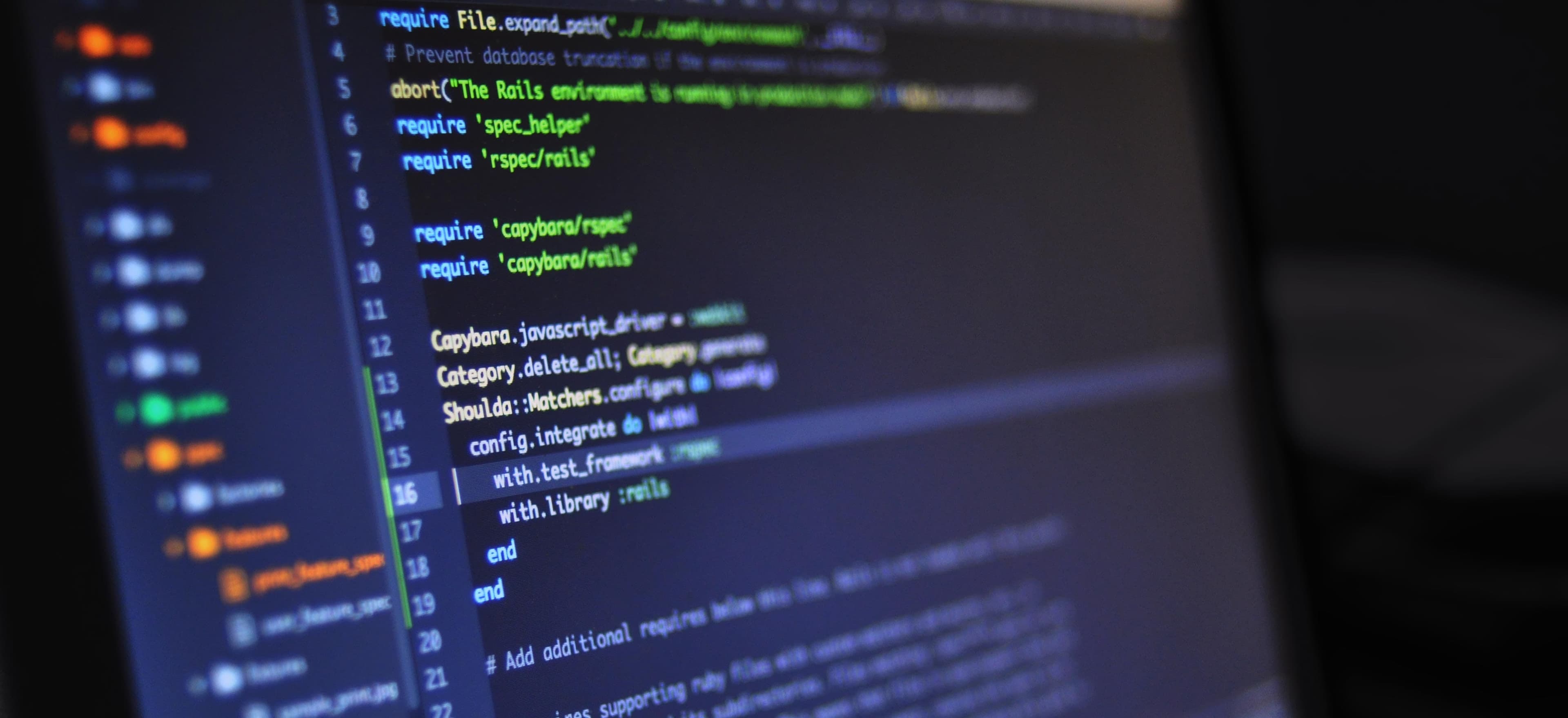
- Published on
Mastering Android Multi-Touch: Common Gesture Pitfalls
As mobile devices become increasingly sophisticated, multi-touch functionality has emerged as a cornerstone of user interaction. Android, as a dominant mobile operating system, offers robust support for multi-touch gestures. Yet, dealing with gestures can be tricky, and it's easy to encounter pitfalls that can lead to a less-than-optimal user experience. This blog post explores common missteps in Android multi-touch implementation and provides strategies for achieving a seamless interaction that keeps users engaged.
Understanding Multi-Touch Gestures
Before diving into common pitfalls, we must first understand what multi-touch gestures entail. Multi-touch refers to the ability of a device screen to recognize multiple touch points simultaneously. For instance, pinch-to-zoom is a classic example, where two fingers can move apart to zoom in or come together to zoom out.
Understanding how gestures work allows developers to properly build applications that make full use of these capabilities. The MotionEvent
class in Android provides the necessary tools to detect and respond to gestures. Here's a simple overview of how to handle touches:
Example of Basic Touch Handling
@Override
public boolean onTouchEvent(MotionEvent event) {
int action = event.getAction();
switch (action & MotionEvent.ACTION_MASK) {
case MotionEvent.ACTION_DOWN:
// Capture the initial touch position
handleActionDown(event);
break;
case MotionEvent.ACTION_MOVE:
// Handle movement
handleActionMove(event);
break;
case MotionEvent.ACTION_UP:
// Handle touch release
handleActionUp(event);
break;
}
return true;
}
In the code above, we use the onTouchEvent
method to differentiate between various touch actions. Each MotionEvent
provides information about the action and the position of the touch.
Common Gesture Pitfalls
While multi-touch can enhance your app, pitfalls are common among developers who are still learning the ropes. Here are some typical challenges and how to resolve them.
1. Neglecting Gesture Detection
One common pitfall is not implementing proper gesture detection. Developers often rely solely on onTouchEvent
for gesture analysis. However, certain gestures, such as double-tap or swipe, require dedicated handlers.
Solution: Use the GestureDetector
class.
GestureDetector gestureDetector = new GestureDetector(context, new GestureDetector.SimpleOnGestureListener() {
@Override
public boolean onDoubleTap(MotionEvent e) {
// Handle double tap
return true;
}
@Override
public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) {
// Handle scrolling
return true;
}
});
Here, GestureDetector.SimpleOnGestureListener
provides override methods to capture specific gestures cleanly. This makes gesture detection manageable and performance-oriented.
2. Inadequate Handling of Touch States
Developers often overlook the importance of recognizing touch states effectively. Implementing proper state management can prevent problems such as repeated actions or incomplete gestures.
Solution: Maintain a state variable within your gesture handler.
private boolean isGestureCompleted = false;
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
// Start gesture
isGestureCompleted = false;
break;
case MotionEvent.ACTION_MOVE:
// Update gesture
if (isGestureConditionMet(event)) {
// Perform action
isGestureCompleted = true;
}
break;
case MotionEvent.ACTION_UP:
if (!isGestureCompleted) {
// Reset state
handleIncompleteGesture();
}
break;
}
return true;
}
By maintaining isGestureCompleted
, we ensure that we accurately capture whether a gesture was finished, allowing for smarter responses.
3. Ignoring Performance Implications
Complex gesture handling can lead to performance bottlenecks that degrade the user experience. Excessive calculations or poorly optimized drawing routines can stutter the UI.
Solution: Optimize your rendering code and calculations.
@Override
public boolean onTouchEvent(MotionEvent event) {
if (event.getPointerCount() > MAX_FINGERS) {
return false; // Limit the number of processed fingers
}
// Perform complex calculations or drawing only when necessary
if (shouldRedraw(event)) {
invalidate(); // Trigger a redraw
}
return true;
}
By setting a threshold (MAX_FINGERS
), you mitigate the performance hit that comes with processing too many touch points. Always ensure rendering is done at optimal times to keep the UI responsive.
4. Lack of Feedback
User feedback is crucial whenever a gesture is recognized. Skipping tactile or visual prompts can create confusion and lead users to wonder if their gesture had any effect.
Solution: Implement haptic feedback and UI animations.
@Override
public boolean onDoubleTap(MotionEvent e) {
Vibrator vibrator = (Vibrator) getSystemService(Context.VIBRATOR_SERVICE);
if (vibrator != null) {
vibrator.vibrate(50); // Provide haptic feedback
}
animateDoubleTapEffect(); // Custom animation
return true;
}
Providing haptic feedback and visual confirmation boosts user satisfaction. It demonstrates that the app is responsive and engaged with the user's actions.
Testing and Iteration
After implementing changes, thorough testing is vital. Use tools like Android's UI Automator to automate gesture tests, ensuring all pathways are covered. Run your application on multiple devices, accounting for varying touch sensitivity and screen sizes.
Closing the Chapter
Mastering Android multi-touch involves recognizing common pitfalls and actively working to prevent them. By dedicating attention to gesture detection, state management, performance optimization, and user feedback, developers can create applications that are not only functional, but also enjoyable to use.
With the code snippets and solutions provided, you're well on your way to crafting a solid multi-touch experience for your users. As technology continues to evolve, being diligent about refining gesture handling will keep you ahead of the curve.
To further refine your understanding, consider exploring Android's official documentation on multi-touch events for additional insights and deeper dives into the intricacies of touch event management. Happy coding!