Mastering Date & Time Handling in JAX-RS 2.0 Applications
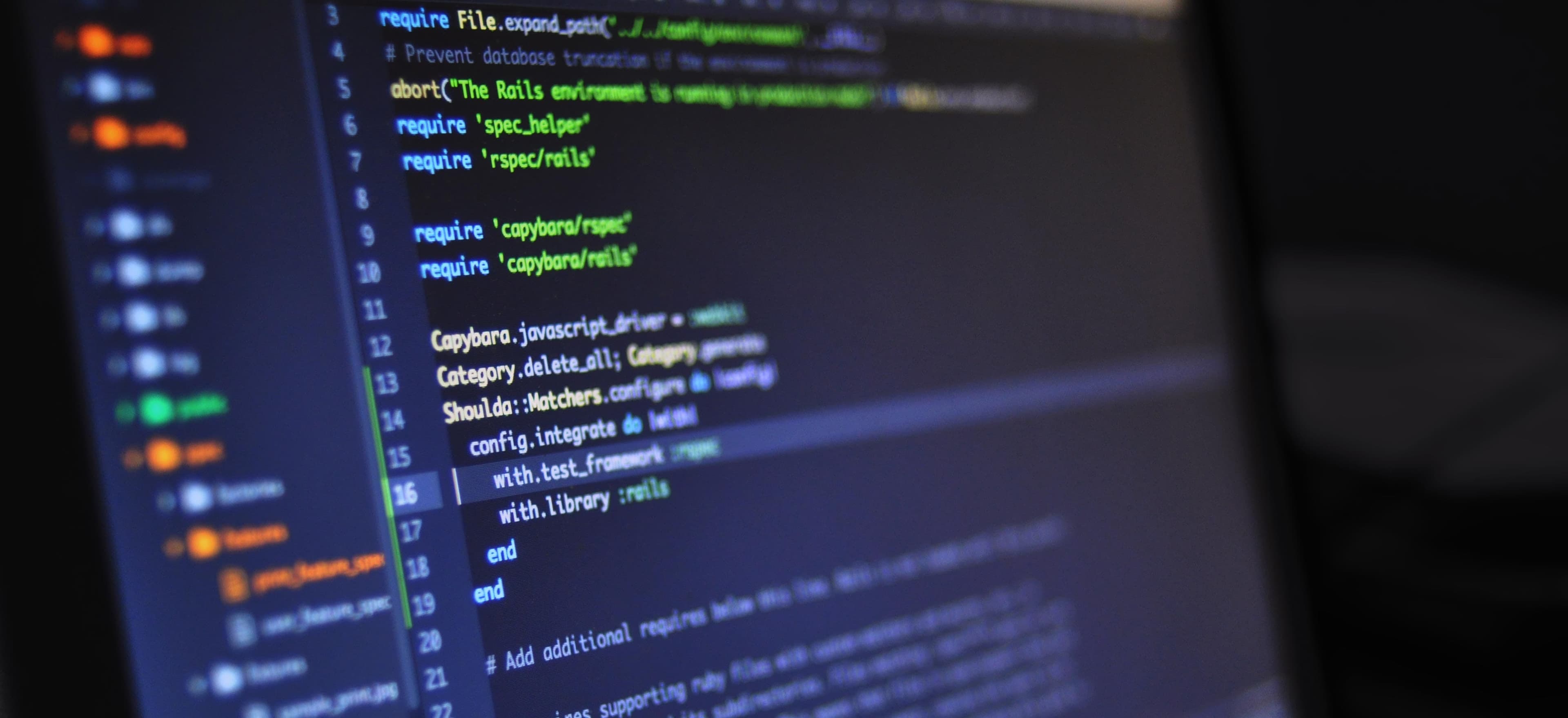
- Published on
Mastering Date & Time Handling in JAX-RS 2.0 Applications
In the rapidly evolving world of Java and web services, managing date and time effectively is crucial. With the rise of JAX-RS (Java API for RESTful Web Services), developers need an efficient way to handle date and time in their applications. This blog post aims to guide you through the nuances of date and time handling in JAX-RS 2.0 applications, ensuring your web services can seamlessly interact with temporal information.
Understanding the JAX-RS Framework
JAX-RS is a set of APIs that allow developers to create RESTful web services in Java. It simplifies the development of REST by providing annotations and a simple structure, allowing for clean code.
Why Date and Time Matter
In web applications, managing date and time is vital for various reasons, including:
- User Experience: Displaying dates correctly enhances user understanding.
- Data Integrity: Accurate timestamps are crucial for logging events.
- Integration: Different systems may use different time zones; handling this smoothly is critical.
To better understand how to handle dates and times in JAX-RS, we will explore several methods, including the Java Date and Time API (java.time
), integrating it with JAX-RS, and managing different formats and time zones.
The Java Date and Time API
Since Java 8, the Date and Time API (java.time
) provides a modern and comprehensive framework for date and time manipulation. It includes classes such as LocalDate
, LocalDateTime
, and ZonedDateTime
, which allow for easy manipulation of dates and times.
Example of Using java.time
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class DateExample {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
String formattedDate = today.format(formatter);
System.out.println("Today's date in dd-MM-yyyy format: " + formattedDate);
}
}
Why Use java.time
?
- Immutable: Objects in
java.time
are immutable, which means they are thread-safe. - Type-Safe: Each class is designed for a specific date/time use case, minimizing errors.
Integrating Date Handling in JAX-RS
JAX-RS makes it straightforward to incorporate date and time handling in your RESTful services through parameter and response handling.
Creating a RESTful Service with Date Handling
Let’s look at an example of a simple JAX-RS application that exposes an endpoint to fetch the current date.
Step 1: Add Dependencies
In your Maven pom.xml
, include the JAX-RS and Jackson dependencies:
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.12.3</version>
</dependency>
Step 2: Create the Resource Class
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import java.time.LocalDate;
@Path("/date")
public class DateResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public LocalDate getCurrentDate() {
return LocalDate.now();
}
}
Key Points:
- @Path: Defines the URL path for the service.
- @GET: Specifies that this method should be invoked for GET requests.
- @Produces: Indicates the media type of the response, in this case, JSON.
Handling Date Input from Clients
When clients send date data, we must ensure it is correctly parsed. We can use @QueryParam
, @PathParam
, or @FormParam
annotations depending on the request type.
Parsing Input Date
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
@Path("/date")
public class DateResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
public String postDate(LocalDate inputDate) {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
return "Received date: " + inputDate.format(formatter);
}
}
Why Handle Dates Correctly?
Handling dates and times correctly is vital for ensuring consistent experiences across different clients and varying locales. When designing APIs, consider the expected date format and time zone.
Time Zones and Date Handling
When dealing with different regions, time zones become crucial. Using ZonedDateTime
allows you to represent a date and time with an associated time zone.
Example of Using ZonedDateTime
import java.time.ZonedDateTime;
public class ZonedDateExample {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now();
System.out.println("Current date and time with time zone: " + zonedDateTime);
}
}
Testing Date Handling in JAX-RS
Consider using tools like Postman or Swagger UI to test your API. You can send requests to your endpoints and verify the output for different date formats and time zones.
Closing Remarks
Mastering date and time handling in JAX-RS 2.0 applications is not just about storing timestamps but ensuring your application remains user-friendly, efficient, and error-proof. By utilizing the modern Java Date and Time API and integrating it smoothly with JAX-RS, you can build RESTful services that manage temporal data effectively.
Further Readings
For additional insights, you may find these resources helpful:
- JAX-RS 2.1 Documentation
- Java 8 Date and Time: An Overview
Leveraging the techniques discussed in this article will set you on the right path to mastering date and time handling in your JAX-RS applications, ultimately leading to better-built and more robust web services. Happy coding!