Extracting Schema from Avro Files: A Step-by-Step Guide
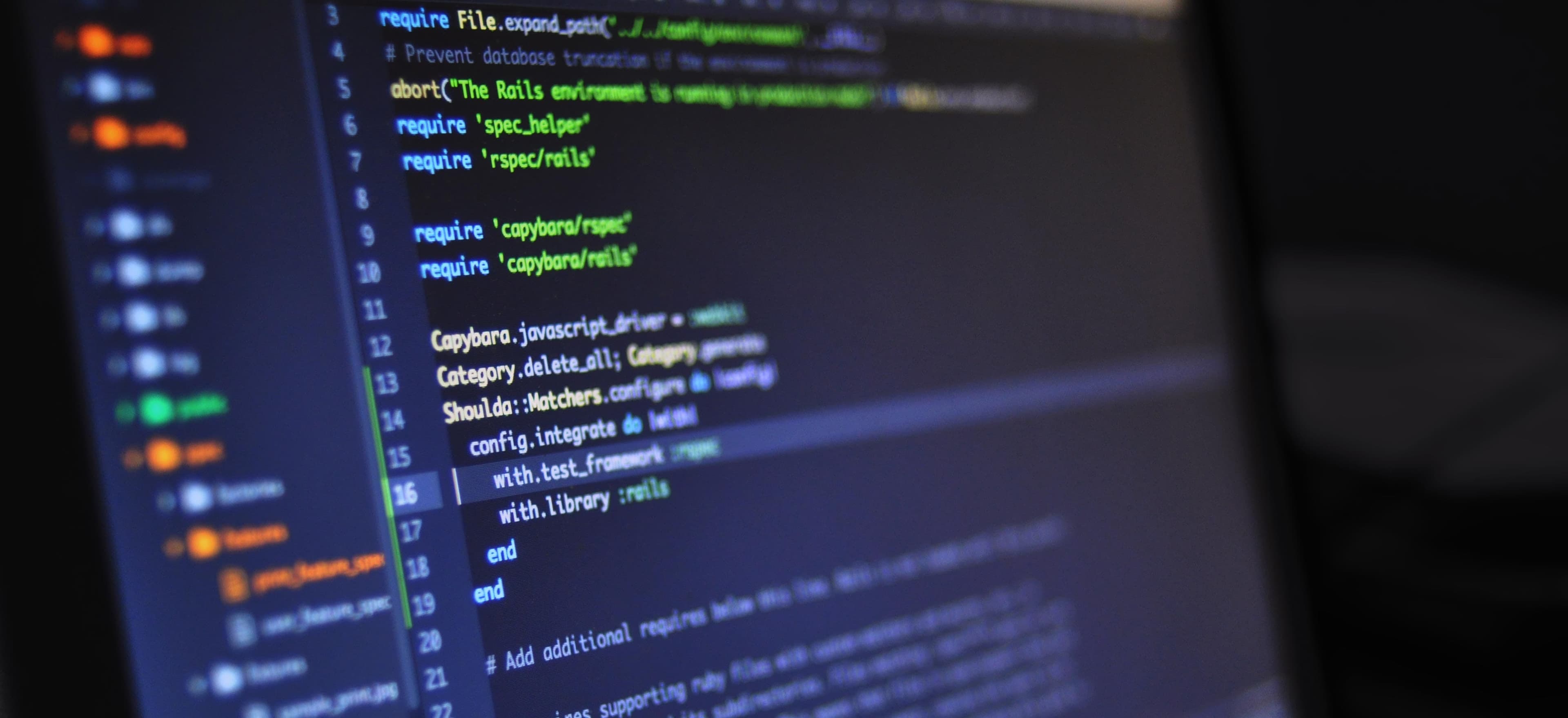
- Published on
Extracting Schema from Avro Files: A Step-by-Step Guide
Apache Avro is a popular data serialization framework that offers a rich set of features like schema evolution, dynamic typing, and efficient binary serialization. One of the key strengths of Avro lies in its schema declaration, which defines the structure of the data in the Avro file. In this post, we will explore how to extract the schema from Avro files effectively.
Understanding Avro and Its Schema
Before we dive into the specific steps to extract schema, it’s crucial to understand what Avro schema is and why it matters. Avro schemas are defined using JSON and describe the data types and structure, including:
- Primitive types: Such as
int
,long
,float
,double
,string
, etc. - Complex types: Such as
record
,enum
,array
,map
, andfixed
.
An Avro schema not only helps in reading data accurately but also ensures compatibility across different versions of the data. For example, a schema can evolve by adding new fields without breaking existing implementations.
Prerequisites
Ensure you have the following set up:
- Java Development Kit (JDK)
- Apache Avro library
- An Avro file (
.avro
) to work with
You can get the latest Avro Java library from Apache Avro Releases.
Step 1: Setting Up Your Environment
To extract the schema from Avro files, first, create a Maven project or a simple Java application. Here’s a basic Maven setup you may use in your pom.xml
file.
<dependencies>
<dependency>
<groupId>org.apache.avro</groupId>
<artifactId>avro</artifactId>
<version>1.10.2</version>
</dependency>
</dependencies>
This dependency will help you use the necessary classes to interact with Avro files.
Step 2: Writing Java Code to Extract the Schema
We’ll write a Java program that reads an Avro file and extracts its schema. Below is an example of how you can do this.
import org.apache.avro.Schema;
import org.apache.avro.file.DataFileReader;
import org.apache.avro.generic.GenericDatumReader;
import java.io.File;
import java.io.IOException;
public class AvroSchemaExtractor {
public static void main(String[] args) {
String avroFile = "path/to/your/file.avro"; // Update this with the path to your Avro file
try {
// Create a GenericDatumReader
GenericDatumReader<Object> reader = new GenericDatumReader<>();
// Use DataFileReader to read the Avro file
DataFileReader<Object> dataFileReader = new DataFileReader<>(new File(avroFile), reader);
// Extract the schema
Schema schema = dataFileReader.getSchema();
// Print the schema in a pretty format
System.out.println("Avro Schema: \n" + schema.toString(true));
dataFileReader.close(); // Close the data file reader
} catch (IOException e) {
System.err.println("Error reading Avro file: " + e.getMessage());
}
}
}
Explanation of the Code
- Dependencies: Ensure you have the Avro library for the Java project.
- File Path: Replace
"path/to/your/file.avro"
with the actual path to your Avro file. - GenericDatumReader: This class is used to read the data records from the Avro file, leveraging the schema found within.
- DataFileReader: This is the main class for reading Avro files; it allows you to read the schema and the contents within the file.
- Printing the Schema:
schema.toString(true)
provides a formatted JSON representation of the schema, making it easy to read.
Running the Code
Compile and run the program in your Java IDE or on your terminal. The outcome should display the schema structure of the specified Avro file.
Step 3: Understanding Extracted Schema
Once you run the Java program, you will see an output similar to the following:
{
"type": "record",
"name": "User",
"namespace": "com.example",
"fields": [
{ "name": "name", "type": "string" },
{ "name": "age", "type": "int" },
{ "name": "emails", "type": { "type": "array", "items": "string" } }
]
}
Schema Breakdown
- type: Defines the main type (record in this case).
- name: The name of the record.
- namespace: A namespace to avoid naming conflicts.
- fields: A list of fields, where each field specifies a name and a data type.
Step 4: Additional Considerations
- Schema Evolution: Avro supports schema evolution, which allows new fields to be added and existing ones removed, as long as it adheres to certain compatibility rules. Understanding this is essential for maintaining robust data governance.
- Handling Enums and Complex Types: When working with complex types, you might often encounter enums and maps, which are also defined in the schema. Ensure you understand these structures for efficient data processing.
For more in-depth understanding about Avro schema evolution, you may refer to Avro Documentation on Schema Evolution.
To Wrap Things Up
Extracting schema from Avro files is straightforward with the right tools and libraries. Understanding how to manage Avro schemas can vastly improve your data processing capabilities, making it simpler to manage, evolve, and consume data. Whether you’re implementing a data pipeline or working on a specific application, mastering Avro will undoubtedly benefit you.
As you continue exploring Apache Avro, you might want to dive deeper into features such as data compression and custom serialization. For further detailed insights, don't hesitate to look at the official Apache Avro documentation.
By following this guide, you should feel confident in extracting and understanding Avro schemas to better handle your data needs. Happy coding!