Optimizing JMH Benchmark Setup for Improved Performance
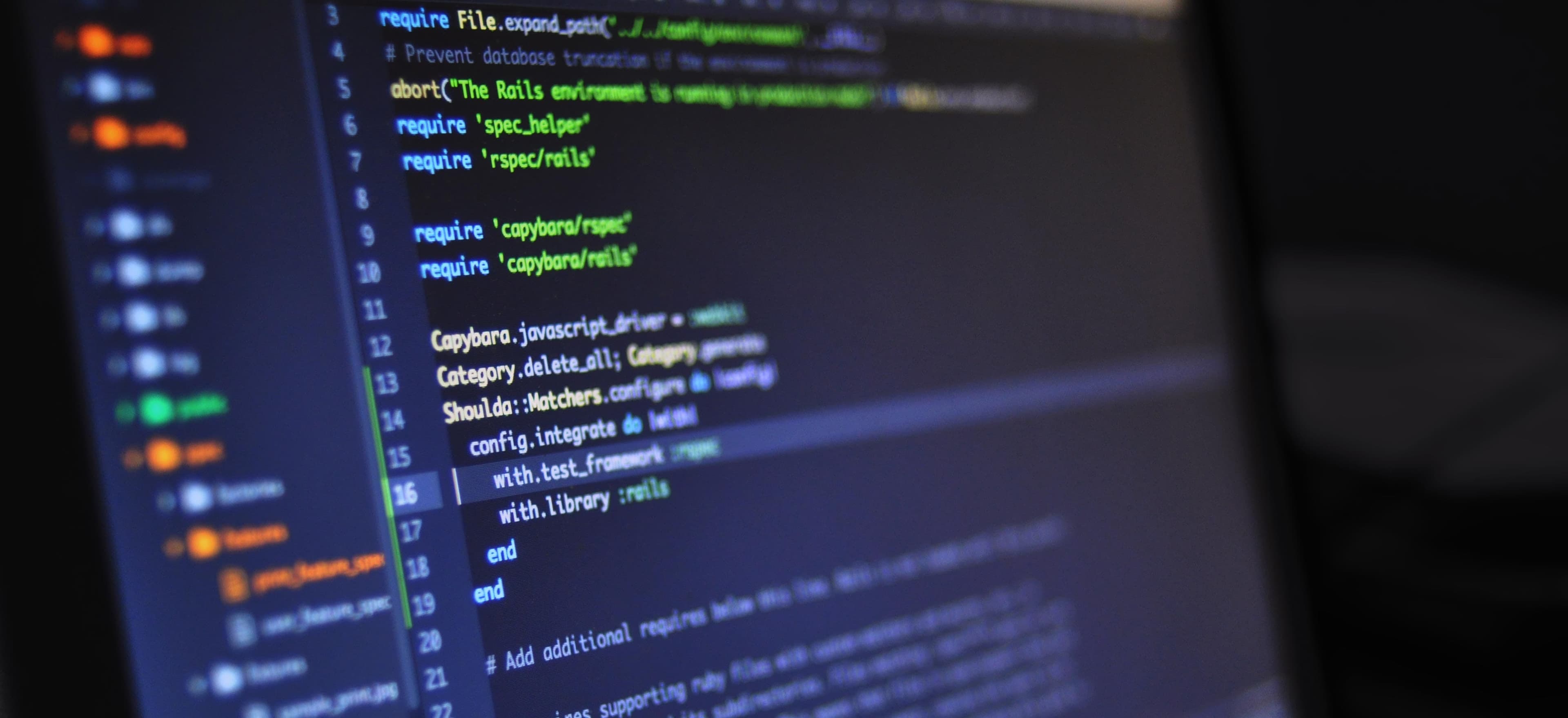
- Published on
Optimizing JMH Benchmark Setup for Improved Performance
In the evolving realm of Java performance analysis, the Java Microbenchmark Harness (JMH) stands out as a powerful tool. It provides a robust framework specifically designed to measure the performance of Java code—pushing microbenchmarking to a whole new level. However, to truly extract the best insights and achieve precise performance benchmarks, it's essential to properly configure and optimize your JMH setups.
What is JMH?
JMH, developed by the folks at OpenJDK, addresses the challenges of benchmarking Java code accurately. Java's Just-In-Time (JIT) compiler and various optimizations by the JVM can distort typical benchmarks. JMH circumvents these pitfalls by spinning up a controlled environment that accounts for these variances.
For a deeper dive into the JMH framework, refer to the official JMH documentation.
Initial Setup for JMH
To start using JMH, include its dependencies in your project. For Maven projects, add the following dependencies in your pom.xml
:
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-core</artifactId>
<version>1.34</version>
</dependency>
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-generator-annprocess</artifactId>
<version>1.34</version>
</dependency>
With Gradle, your build.gradle
would look like:
dependencies {
implementation 'org.openjdk.jmh:jmh-core:1.34'
annotationProcessor 'org.openjdk.jmh:jmh-generator-annprocess:1.34'
}
Creating Your First Benchmark
Here's a simple benchmark method that adds numbers. Let’s create a class named SimpleBenchmark
.
import org.openjdk.jmh.annotations.Benchmark;
public class SimpleBenchmark {
@Benchmark
public int sumNumbers() {
int sum = 0;
for (int i = 0; i < 100; i++) {
sum += i;
}
return sum;
}
}
Running the Benchmark
Compile the benchmark class and run it using the command line:
java -jar target/yourproject.jar SimpleBenchmark
This basic setup allows you to start measuring your Java code's performance. But how do we optimize this for better accuracy and precision?
Optimizing JMH Setup
The potential performance gains of JMH benchmarks can be significantly enhanced by tuning several parameters. Here are critical aspects you need to focus on:
1. Warm-Up Iterations
JMH provides warm-up iterations to let the JVM optimize the code before acquiring measurements. By default, there is one warm-up iteration. You can increase this to ensure the JIT compiler has fully optimized the method under test.
@Warmup(iterations = 5)
@Measurement(iterations = 10)
public void someBenchmarkMethod() {
// Benchmark logic here
}
In the above code, the benchmark will run five warm-up iterations before measuring ten performance iterations.
2. Forking
By forking a new JVM process for the benchmark, we can eliminate the impact of additional loaded classes or application state from other parts of the system:
@Fork(value = 2)
public void anotherBenchmarkMethod() {
// Your benchmarking code
}
This will create two separate JVMs for each measurement, ensuring consistency across runs.
3. Threads and Concurrency
Utilizing different thread counts can illustrate how your code performs under various levels of concurrency. Combining the @Threads
annotation with your benchmarks can provide deeper insights:
@Threads(4)
public void concurrentBenchmarkMethod() {
// Concurrent processing logic
}
This example will run the benchmark using four threads, simulating potential concurrent access.
4. Benchmark Modes
Choosing the right benchmark mode can significantly affect your results. JMH offers various modes such as Throughput
, AverageTime
, SampleTime
, and SingleShotTime
. Each serves different purposes:
- Throughput: measures the number of operations per time unit.
- AverageTime: captures the average time taken for each operation.
- SampleTime: tracks the distribution of time spent across samples.
Example for AverageTime mode:
@BenchmarkMode(Mode.AverageTime)
public void timeCriticalOperation() {
// Time-critical logic
}
5. State Management
Use the @State
annotation to manage the state within your benchmark. This allows you to keep shared state across multiple invocations, which is ideal for scenarios requiring resource sharing:
@State(Scope.Thread)
public class BenchmarkState {
int[] data = new int[1000];
// Initialize data here
}
By placing the setup code here, JMH can optimize the use of allocated memory better than if it had to recreate it for each iteration.
6. Avoiding Dead Code Elimination
If the JIT compiler determines that a part of the code isn't used, it may remove it altogether. To counter this, it's recommended to use the Blackhole
class, which serves as a "consume" mechanism:
import org.openjdk.jmh.infra.Blackhole;
@Benchmark
public void useBlackhole(Blackhole blackhole) {
int result = someHeavyCalculation();
blackhole.consume(result);
}
In the provided method, the Blackhole
instance ensures the result of someHeavyCalculation()
is utilized, avoiding inadvertent optimizations that eliminate the computation.
Final Thoughts
Optimizing JMH benchmark setups requires a synergy between strategic configurations and a robust understanding of Java performance characteristics. Following these guidelines will not only refine the accuracy of your benchmarks but also offer you insights into your application's behavior under varying loads and conditions.
For more information, consider exploring additional resources:
Continually refine your benchmarks, experiment with different configurations, and harness the core power of JMH to elevate your Java performance analysis to exceptional heights. Happy benchmarking!
Checkout our other articles