Common Pitfalls When Building WebSocket Servers with Undertow
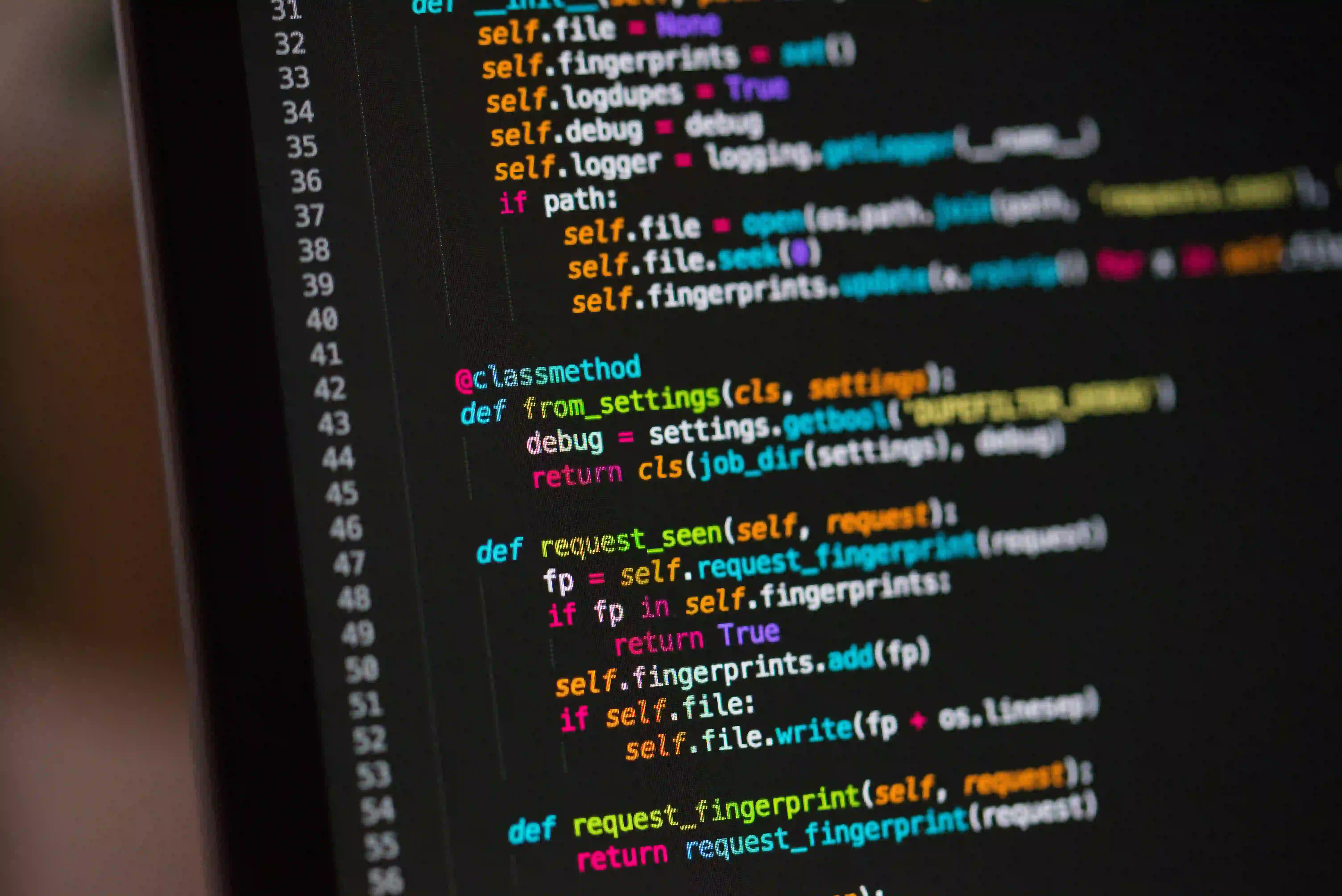
Common Pitfalls When Building WebSocket Servers with Undertow
WebSockets have revolutionized real-time communication on the web. They allow servers and clients to communicate directly without the need for constant polling, significantly reducing latency and resource usage. One of the popular frameworks to implement WebSocket servers in Java is Undertow.
Though Undertow is a robust and lightweight tool, building WebSocket servers can lead to common pitfalls. This article discusses these challenges and how to avoid them while providing useful code snippets. We will cover topics including error handling, security, scaling, and maintaining a clean architecture.
What is Undertow?
Undertow is a flexible and performant web server that implements the Java Servlet API, which makes it excellent for creating WebSocket servers. Understanding how to use Undertow effectively can lead to efficient real-time communication systems.
Basic WebSocket Example
Before we dive into pitfalls and solutions, let's set up a simple WebSocket server using Undertow:
import io.undertow.Undertow;
import io.undertow.server.handlers.PathHandler;
import io.undertow.websockets.core.*;
import io.undertow.websockets.jsr.ServerContainer;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/websocket")
public class MyWebSocketEndpoint {
@OnOpen
public void onOpen(Session session) {
System.out.println("Session opened: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Received message: " + message);
sendMessage(session, "You sent: " + message);
}
@OnClose
public void onClose(Session session) {
System.out.println("Session closed: " + session.getId());
}
private void sendMessage(Session session, String message) {
try {
session.getBasicRemote().sendText(message);
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Undertow server = Undertow.builder()
.addHttpListener(8080, "0.0.0.0")
.setHandler(new PathHandler().addPath("/websocket", new MyWebSocketEndpoint()))
.build();
server.start();
}
}
Explanation
In this example, we define a WebSocket server endpoint that responds to connection events, incoming messages, and disconnection events. The sendMessage
method is central for communicating back to the client.
Now, let’s explore some common pitfalls.
1. Lack of Proper Error Handling
Problem
When an error occurs (e.g., a malformed message), you might simply print the stack trace or do nothing, which could lead to resource leaks or unresponsive clients.
Solution
Implement graceful error handling to ensure that clients receive feedback and that the server remains stable.
@OnMessage
public void onMessage(String message, Session session) {
try {
System.out.println("Received message: " + message);
// Processing...
sendMessage(session, "You sent: " + message);
} catch (Exception e) {
System.err.println("Error processing message: " + e.getMessage());
sendMessage(session, "Error processing your message");
}
}
Why This Matters
This ensures that even when errors occur, the server can handle them gracefully and provide users with understandable messages, contributing to a better user experience.
2. Not Considering Security Aspects
Problem
WebSocket servers can be vulnerable to attacks such as Cross-Site WebSocket Hijacking. If security is not taken seriously, sensitive data can be compromised.
Solution
You should authenticate users before establishing a WebSocket connection. Always use HTTPS to encrypt WebSocket connections.
@OnOpen
public void onOpen(Session session) {
String authToken = session.getRequestParameterMap().get("token").get(0);
if (!isValidToken(authToken)) {
throw new IllegalArgumentException("Invalid token");
}
System.out.println("Session opened: " + session.getId());
}
Why This Matters
Authentication ensures that only valid users can interact with your WebSocket server, preventing unauthorized access and protecting user data.
3. Ignoring Session Management
Problem
Neglecting to manage user sessions can lead to memory leaks and performance degradation. If sessions are not closed properly, resources will be consumed unnecessarily.
Solution
Properly manage WebSocket sessions with appropriate open and close procedures.
@OnClose
public void onClose(Session session) {
System.out.println("Session closed: " + session.getId());
// Perform any cleanup if necessary
}
Why This Matters
Managing sessions ensures that the server is not overwhelmed with inactive connections, which can lead to improved performance and resource management.
4. Not Handling Backpressure
Problem
A common issue in WebSocket applications is dealing with backpressure, where the server may send messages faster than the client can process them.
Solution
Implement throttling mechanisms or apply flow control. For instance, if you are sending bulk data, ensure the client can request data at a manageable pace.
public void sendMessage(Session session, String message) {
if (session.isOpen()) {
try {
session.getAsyncRemote().sendText(message);
} catch (IllegalStateException e) {
System.err.println("Failed to send message: " + e.getMessage());
}
}
}
Why This Matters
Handling backpressure ensures that the communication remains smooth and efficient without overwhelming the client.
5. Failing to Scale
Problem
Many developers overlook the need for scalability in their initial designs. As your user base grows, your server needs to handle more connections effectively.
Solution
Consider employing load balancers or clustering your WebSocket servers to manage multiple connections. Tools like Apache Kafka can help manage messaging across distributed services.
Why This Matters
Scaling means your application can grow with demand, ensuring a consistent user experience even under heavy load.
The Bottom Line
Building WebSocket servers with Undertow can be a straightforward process if you pay attention to common pitfalls. By implementing proper error handling, considering security measures, managing sessions, addressing backpressure, and planning for scalability, you can create robust WebSocket applications that thrive in real-world scenarios.
For more details on Undertow and WebSockets, explore the following resources:
- Undertow Documentation
- Java EE WebSocket Specification
Following best practices will not only enhance the performance of your application but also foster a more secure and user-friendly environment. Happy coding!