Overcoming Common Pitfalls in Java Platform Module System
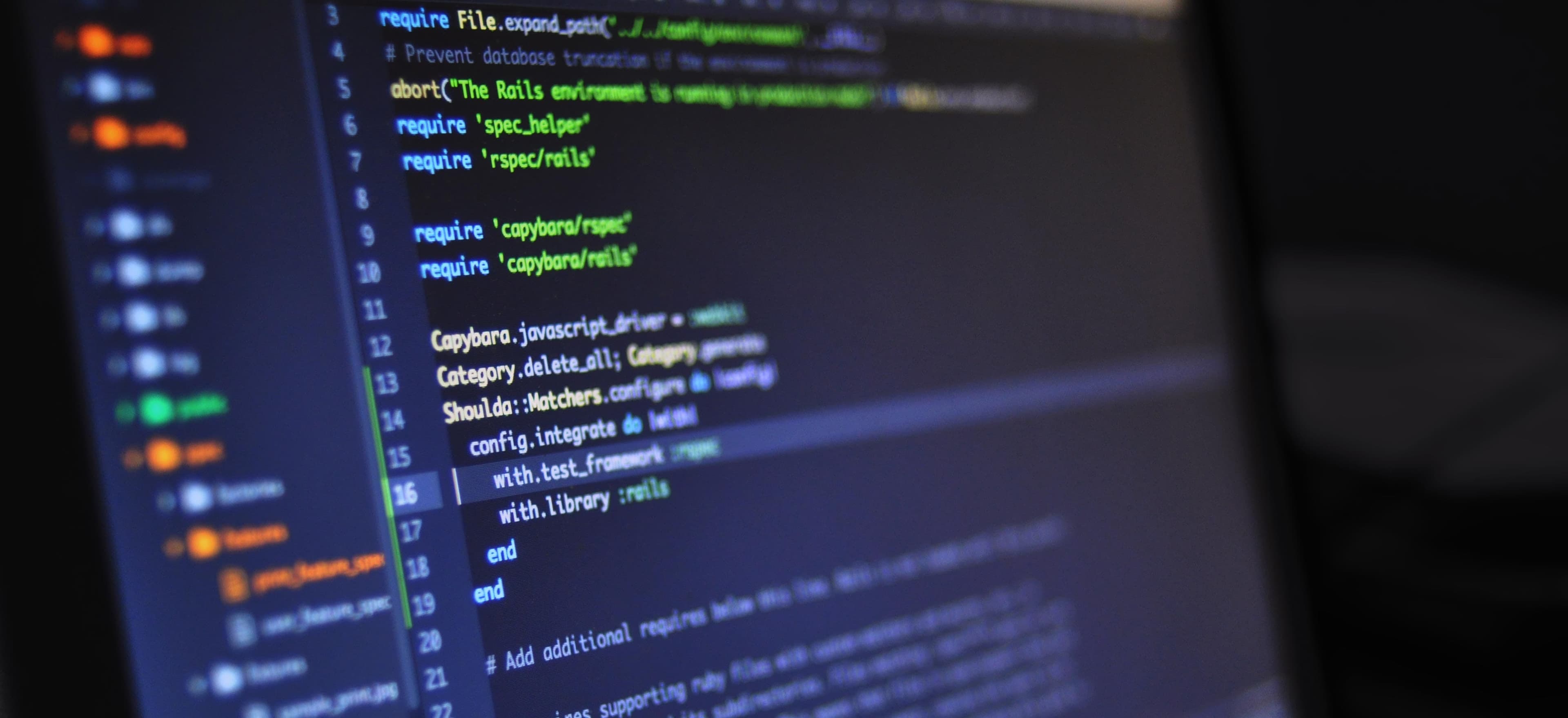
- Published on
Overcoming Common Pitfalls in Java Platform Module System
The Java Platform Module System (JPMS), introduced with Java 9, marked a significant change in how Java developers structure and manage dependencies within their applications. While the advantages are numerous, developers often encounter common pitfalls that can hinder the effective use of this modular system. This blog post aims to address these challenges and provide solutions, alongside practical examples.
Understanding JPMS
Before diving into the common pitfalls, let's briefly revisit what JPMS is. It allows developers to structure a Java program as a collection of modules, each with its own dependencies and access controls. The primary goals of JPMS are to improve application performance, enhance security, and provide clearer code organization.
JPMS uses the module
keyword to define modules and the requires
and exports
statements to manage dependencies and visibility.
module com.example.myapp {
requires com.example.mylibrary; // Declaring a dependency on another module
exports com.example.myapp.api; // Making this package available to other modules
}
Now that we have a foundation, let's explore some common pitfalls and how to overcome them.
Pitfall 1: Poor Module Design
Problem
Many developers rush into creating modules without a solid understanding of what should be encapsulated. A poorly designed module can lead to both internal and external inconsistencies in code, making it difficult to maintain down the road.
Solution
When designing modules, consider the Single Responsibility Principle (SRP). Each module should encapsulate a specific piece of functionality. Additionally, think about the visibility of each component. Only expose packages that other modules absolutely need to access.
Example
// Good Module Design: Only export necessary packages
module com.example.calculator {
exports com.example.calculator.api; // Exposing just the API
}
By limiting exports, you effectively reduce the coupling between modules and enhance their reusability.
Pitfall 2: Misleading Dependencies
Problem
Modules can easily lead to a misunderstanding of dependency management. Newer developers may assume that all dependencies are inherited, which is not the case. If a module requires another, it must declare this dependency explicitly.
Solution
Always use the requires
directive for all dependencies. This declaration serves as documentation as well as a contract that maintains clear lines of dependencies.
Example
module com.example.service {
requires com.example.repository; // Explicitly stating the dependency
}
This way, anyone reading the module descriptor can quickly understand what dependencies exist.
Pitfall 3: Ignoring Readability and Documentation
Problem
Complex module structures can lead to confusion, especially for teams collaborating on the same project. If documentation is sparse or unclear, it can result in improper usage of modules.
Solution
Utilize Javadoc comments and provide clear module descriptors. Encourage your team to document any changes and decisions made regarding the module structure.
Example
/**
* This module is responsible for user authentication and authorization.
* It requires the data module for accessing user data.
*/
module com.example.auth {
requires com.example.data;
exports com.example.auth.api;
}
Clear documentation helps onboard new developers and facilitates understanding for all team members.
Pitfall 4: Ignoring the Module Path
Problem
When transitioning from a traditional JAR-based approach, many developers overlook the importance of the module path. Modules don't work like JAR files; instead, they have specific paths that need to be set up.
Solution
Make sure to understand the difference between the classpath and module path. For JPMS, you must run your applications with the --module-path
option, specifying where your modules are located.
Example
To execute a modular Java application, use the following command:
java --module-path mods -m com.example.myapp/com.example.myapp.Main
This command states where to find the modules and specifies which module to run.
Pitfall 5: Transitive Dependencies
Problem
Mismanaging transitive dependencies can lead to runtime errors. Developers may expect a module to be available through a direct dependency, but this isn’t guaranteed unless specified.
Solution
Use the requires transitive
directive to manage transitive dependencies effectively. This makes your module available to any module that requires it.
Example
module com.example.library {
exports com.example.library.api; // Exposing the API
}
module com.example.service {
requires transitive com.example.library; // Making library available to consumers
}
With this setup, any module that requires com.example.service
will automatically have access to com.example.library
.
Pitfall 6: Mismanagement of the 'open' Directive
Problem
The open
directive is often misunderstood. It is important for reflection-based frameworks, such as Spring, but can lead to unnecessary exposure of internal APIs if mismanaged.
Solution
Only use opens
for modules that truly need reflection. Instead, focus on exporting APIs that are meant for public use.
Example
module com.example.internal {
opens com.example.internal.data; // Use only when reflection is necessary
}
By carefully managing internal access, you maintain better encapsulation and minimize vulnerabilities.
Additional Resources
Here are some useful links for further reading:
- Java Platform Module System Guide - This official guide provides a comprehensive overview of JPMS.
- Understanding Modules in Java - A resourceful article discussing various aspects of modular programming in Java.
In Conclusion, Here is What Matters
JPMS presents significant opportunities for enhancing the modularity, security, and performance of Java applications. However, it's crucial to navigate common pitfalls effectively. By focusing on good design principles, understanding dependencies, maintaining clear documentation, and managing your module paths, you can leverage the full potential of Java modules.
Remember, the world of modules may seem daunting at first, but with practice and understanding, it will soon become second nature. Embrace the challenges, learn from them, and you'll be well on your way to becoming a proficient Java module developer.
Checkout our other articles