Mastering Jackson's PropertyNamingStrategy: Common Pitfalls
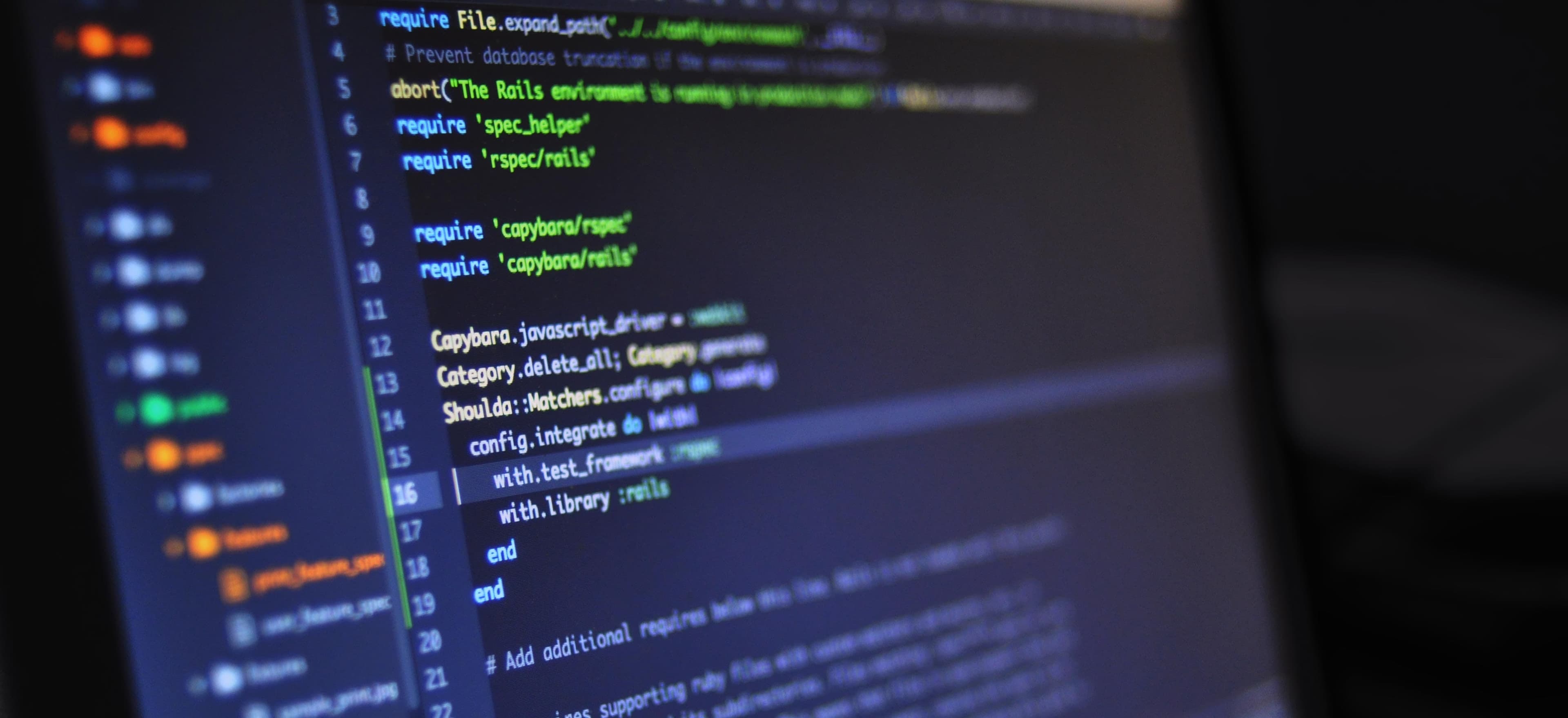
- Published on
Mastering Jackson's PropertyNamingStrategy: Common Pitfalls
Jackson is a powerful library for JSON serialization and deserialization in Java. One of its features, the PropertyNamingStrategy
, allows developers to customize how property names are converted to JSON field names. However, navigating its options can be tricky, leading to common pitfalls. This article will explore these pitfalls, elaborate on how to avoid them, provide code examples, and offer insights on best practices.
What is PropertyNamingStrategy?
Jackson's PropertyNamingStrategy
provides a way to define the naming convention used for properties in serialized JSON. By default, Jackson uses the Java bean naming convention, which can lead to inconsistencies when dealing with external APIs or legacy systems. For instance, a common convention in JSON is snake_case, whereas Java uses camelCase.
Why Use PropertyNamingStrategy?
- Consistency: Standardize naming across your application.
- Interoperability: Ensure that your JSON structure matches that of external systems.
- Customization: Alter the way fields are represented without modifying the underlying Java model.
Common Pitfalls
Let's dive into some typical mistakes developers make when using PropertyNamingStrategy
.
Pitfall 1: Misunderstanding Naming Conventions
Jackson provides several built-in naming strategies, such as:
LOWER_CAMEL_CASE
UPPER_CAMEL_CASE
SNAKE_CASE
KEBAB_CASE
Many developers assume that using a naming strategy is as simple as declaring it. However, mistakes arise when they do not fully understand how each strategy modifies property names.
Example:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
public class User {
private String firstName;
private String lastName;
// getters and setters
}
// Example of using Default naming (no PropertyNamingStrategy)
ObjectMapper mapper = new ObjectMapper();
User user = new User();
user.setFirstName("John");
user.setLastName("Doe");
// Output as {"firstName":"John","lastName":"Doe"}
String jsonResult = mapper.writeValueAsString(user);
In this case, if you need the output to be in snake_case, simply setting a different PropertyNamingStrategy
will yield the desired format.
ObjectMapper mapper = new ObjectMapper();
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
String snakeCaseJson = mapper.writeValueAsString(user);
// Output will be {"first_name":"John","last_name":"Doe"}
Pitfall 2: Forgetting Annotations
Jackson allows annotations to specify custom property names. When used alongside PropertyNamingStrategy
, conflicts can arise. For example, if you specify @JsonProperty
at the field level, it may not adhere to the naming strategy defined at the ObjectMapper
level.
Example:
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("first_name")
private String firstName;
private String lastName;
// getters and setters
}
// Using SNAKE_CASE
ObjectMapper mapper = new ObjectMapper();
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
String outputJson = mapper.writeValueAsString(user);
// Output will have first_name as defined by @JsonProperty
In this scenario, the firstName
field explicitly uses the annotation, making it immune to the SNAKE_CASE
strategy, which could lead to confusion. Always check your output to ensure it aligns with your expectations.
Pitfall 3: Mixing Naming Strategies
Another common error is mixing naming strategies between different models. This can lead to unpredictable results, especially in larger applications where various developers might follow different conventions.
Example:
ObjectMapper mapper = new ObjectMapper();
// SNAKE_CASE for User
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
String userJson = mapper.writeValueAsString(new User());
// Output: {"first_name":"Jane","last_name":"Doe"}
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.LOWER_CAMEL_CASE);
String otherJson = mapper.writeValueAsString(new AnotherClass());
// Output might be inconsistent if AnotherClass uses different conventions.
To avoid this issue, maintain a consistent coding guideline throughout your application regarding naming strategies.
Pitfall 4: Limitations of Custom Naming Strategies
Creating custom PropertyNamingStrategy
subclasses can be beneficial, but it introduces complexity that can lead to unexpected behaviors if not managed carefully.
Example:
public class CustomNamingStrategy extends PropertyNamingStrategy {
@Override
public String translate(String propertyName) {
// Custom logic here
return propertyName.toUpperCase(); // this will convert all to uppercase
}
}
// Using custom naming strategy
ObjectMapper mapper = new ObjectMapper();
mapper.setPropertyNamingStrategy(new CustomNamingStrategy());
String customJson = mapper.writeValueAsString(user);
// Output: {"FIRSTNAME":"John","LASTNAME":"Doe"}
Using such a strategy broadly may lead to conflicts with other serialization libraries or create maintenance challenges, especially if properties are expected to follow specific naming conventions.
Best Practices
1. Consistent Naming Conventions
Adopt a uniform naming strategy across your application. This reduces confusion and ensures that JSON output remains predictable.
2. Minimal Use of Annotations
While annotations like @JsonProperty
are powerful, using them extensively can lead to clashes with PropertyNamingStrategy
. Prefer using one standard to maintain clarity.
3. Test Your Outputs
Always write tests to verify that your JSON serialization works as expected when using different naming strategies. Integration tests with external systems can also be beneficial.
4. Documentation
Ensure to document your approach to JSON serialization, including any custom naming strategies. This helps onboard new team members and mitigates future confusion.
Closing Remarks
Navigating Jackson's PropertyNamingStrategy
can be fraught with challenges, as discussed in this article. Misunderstanding conventions, misusing annotations, mixing strategies, and unnecessary complexity from custom strategies are common pitfalls that developers often encounter. By adhering to best practices and keeping things simple, you can maximize the effectiveness of JSON handling in your Java applications.
For further reading on Jackson, you might want to check out the official Jackson documentation or Jackson's PropertyNamingStrategy.
By mastering these concepts, you will not only improve the quality of your JSON outputs but also enhance your overall Java development skills. Happy coding!
Checkout our other articles