Common Pitfalls in Defining EJB Services and JPA Entities
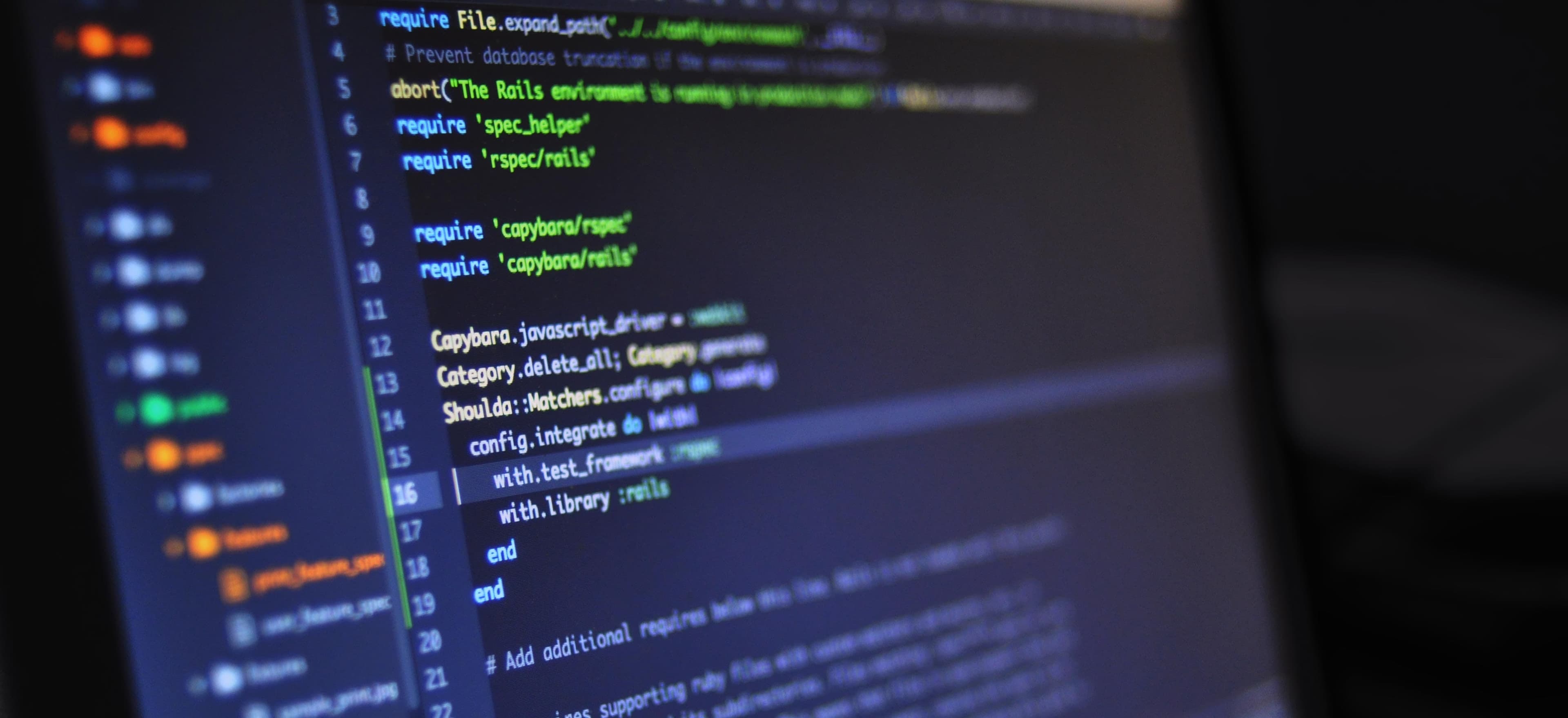
- Published on
Common Pitfalls in Defining EJB Services and JPA Entities
Enterprise JavaBeans (EJB) and Java Persistence API (JPA) are foundational components of Java EE that facilitate the development of scalable and robust enterprise applications. Yet, despite their advantages, developers often encounter pitfalls when defining EJB services and JPA entities. This blog post explores these pitfalls, their implications, and how to avoid them effectively.
Understanding EJB and JPA
Before diving into the pitfalls, let's briefly outline what EJB and JPA are:
- Enterprise JavaBeans (EJB): A server-side component architecture that simplifies the development of large-scale enterprise applications by providing a robust model for building distributed applications.
- Java Persistence API (JPA): A specification for accessing, persisting, and managing data between Java objects and relational databases.
Both technologies are designed to work together, but mistakes in their implementation can lead to performance issues, data inconsistency, and a convoluted architecture.
Common Pitfalls When Defining EJB Services
1. Ignoring Transaction Management
Transaction management is at the core of enterprise applications. EJB provides built-in transaction support through annotation-based configuration or declarative transaction demarcation. Ignoring this can lead to inconsistent data states.
Why is this important?
If transactions are not managed properly, you risk having partially completed operations, which may lead to data integrity issues.
Example
@Stateless
public class UserService {
@PersistenceContext
private EntityManager entityManager;
@TransactionAttribute(TransactionAttributeType.REQUIRED)
public void createUser(User user) {
entityManager.persist(user);
}
}
Here, the @TransactionAttribute
annotation ensures that if createUser
fails, no changes will be committed to the database, maintaining data integrity.
2. Overusing Stateless Beans
Stateless session beans are efficient, but overusing them for every single service can quickly complicate your application logic. Sometimes, it’s more suitable to use stateful beans, or to simply employ a different architectural pattern.
Why is this important?
Using stateful beans only when necessary can improve performance by reducing overhead and managing state more effectively.
Example
@Stateful
public class ShoppingCart {
private List<Item> items = new ArrayList<>();
public void addItem(Item item) {
items.add(item);
}
}
In this case, a stateful bean makes sense because you need to track user-specific shopping cart data.
3. Not Utilizing Annotations Effectively
Annotations like @EJB
, @Schedule
, and @Asynchronous
can simplify development but may lead to misconceptions when misused or overused.
Why is this important?
Correct usage of annotations leads to clearer and more maintainable code.
Example
@Stateless
public class OrderService {
@EJB
private PaymentService paymentService;
public void processOrder(Order order) {
// Order processing logic
paymentService.processPayment(order.getPayment());
}
}
Here, @EJB
allows for easy access to another bean but make sure that PaymentService
is not excessively referenced, leading to tight coupling.
Common Pitfalls When Defining JPA Entities
1. Ignoring the Importance of Serializability
Entities should be serializable when they are used to send data over networks. Failing to implement Serializable
on your entity classes can lead to runtime exceptions.
Why is this important?
When EJBs are accessed remotely, their states must be preserved, requiring entities to be serializable.
Example
@Entity
public class User implements Serializable {
@Id
private Long id;
private String name;
// Constructor, Getters, Setters
}
By implementing Serializable, you ensure that the User
entity can be transmitted over the network.
2. Forgetting to Use Lazy Loading
By default, JPA loads relationships eagerly unless specified otherwise. Fetching unnecessary data can slow down your application.
Why is this important?
Using lazy fetching for large associated entities optimizes data retrieval.
Example
@Entity
public class User {
@Id
private Long id;
@OneToMany(fetch = FetchType.LAZY)
private List<Order> orders;
// Constructor, Getters, Setters
}
In this case, FetchType.LAZY
allows orders to be loaded on-demand, reducing initial load time.
3. Maintaining Transactions with JPA Entities
It's essential to maintain transaction boundaries when operating with JPA entities. Not doing so may lead to issues with entity state or persistence context.
Why is this important?
Aligning database operations with transactions ensures that your entity states remain consistent.
Example
@Stateless
public class UserService {
@PersistenceContext
private EntityManager entityManager;
@TransactionAttribute(TransactionAttributeType.REQUIRED)
public void updateUser(User user) {
entityManager.merge(user);
}
}
The transaction attribute ensures that the merge operation completes successfully before committing.
The Closing Argument
Knowing the common pitfalls when defining EJB services and JPA entities can go a long way toward creating efficient and maintainable enterprise applications. By implementing transaction management, using annotations correctly, ensuring your entities are serializable, and optimizing data fetch strategies, you can avoid critical errors that may compromise your application's integrity and performance.
Additional Resources
For further reading, consider visiting:
By understanding and addressing these pitfalls, you'll set a solid foundation for your Java enterprise application, ensuring better performance, reliability, and maintainability. Happy coding!
Checkout our other articles