Common Spring Social Challenges and How to Overcome Them
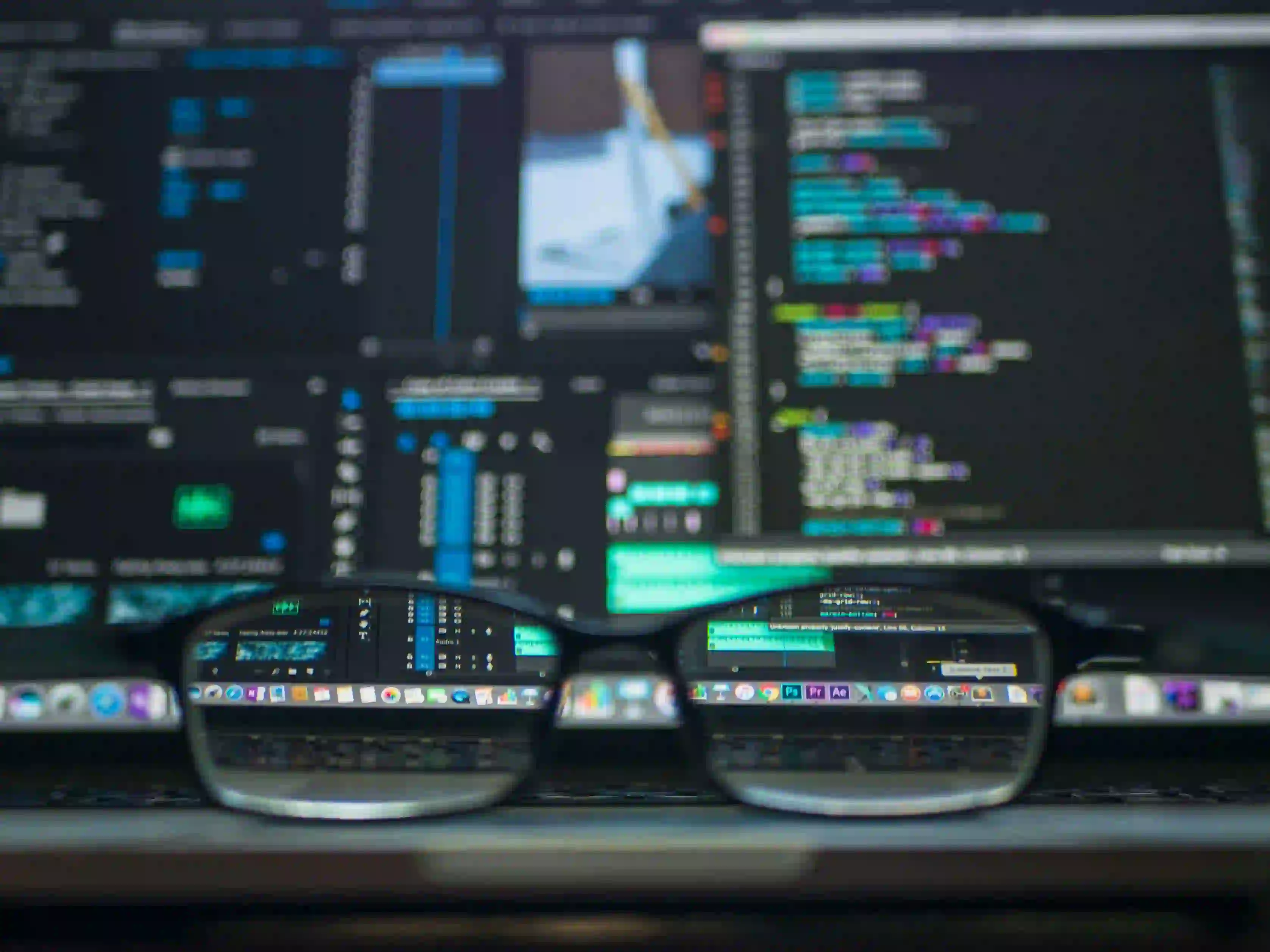
Common Spring Social Challenges and How to Overcome Them
Spring Social is an exciting project aimed at simplifying the integration of social media platforms into Java applications. Nevertheless, it comes with its own collection of challenges. This blog post will dissect common Spring Social challenges and provide practical solutions to overcome them.
Understanding Spring Social
Before we dive into the challenges, it’s crucial to grasp what Spring Social is. Made for developers who wish to connect their applications to various social media networks like Facebook, Twitter, and LinkedIn, Spring Social provides a consistent programming model for integrating with these platforms.
Why Spring Social?
- Ease of Use: It simplifies the process of authenticating and interacting with social media APIs.
- Customizable: You can easily tailor social connections to suit your application's needs.
- Integration: Allows seamless connectivity with Spring applications, enhancing overall functionality.
Common Challenges
1. Authentication Issues
Overview
Authentication is foundational for accessing user data from social media platforms. Each platform (like Facebook, Google, etc.) has its own authentication mechanism, and configuring them can sometimes be daunting.
Solution
To resolve authentication issues, you must keep the following practices in mind:
-
Use Correct Credentials: Ensure you have the correct keys and tokens from the social media platform.
-
Handle Redirects Properly: Make sure your redirect URIs are correctly set in the social platform's developer console and match what you configure in your Spring application.
@Configuration
@EnableSocial
public class SocialConfig extends WebMvcConfigurerAdapter {
// Define connectionFactoryConfigurer bean
@Bean
public ConnectionFactoryConfigurer connectionFactoryConfigurer() {
ConnectionFactoryRegistry registry = new ConnectionFactoryRegistry();
registry.addConnectionFactory(new FacebookConnectionFactory("appId", "appSecret"));
return registry;
}
}
In this example, we initialize a Facebook connection factory with application credentials. Always store sensitive data like application secrets in environment variables or external property files for security.
2. API Rate Limits
Overview
Most social media APIs impose rate limits to prevent abuse. If your application surpasses these limits, it may face temporary access locks, which can significantly disrupt user experience.
Solution
Monitor your application's API usage closely. A strategy to mitigate this is to implement caching for the data you retrieve. Use Spring's caching abstraction to store API responses temporarily.
@Service
public class UserService {
@Cacheable("users")
public User getUserProfile(String userId) {
// Call social media API to fetch user profile
return fetchUserProfileFromAPI(userId);
}
}
The @Cacheable
annotation caches the returned User
object, improving response time and mitigating rate limit concerns.
3. Dependency Management
Overview
Spring Social is built on top of the broader Spring framework, which can lead to complex dependency issues, especially when using different Spring versions or when third-party libraries are involved.
Solution
Use a dependency management tool like Maven or Gradle to manage versions and resolve conflicts effectively. Always ensure that you are using compatible versions of the Spring libraries.
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-facebook</artifactId>
<version>2.0.1.RELEASE</version>
</dependency>
Keep your dependencies updated, and regularly check for breaking changes in newer library releases.
4. Handling API Changes
Overview
Social media platforms continuously evolve their APIs, which can introduce breaking changes that affect your application.
Solution
Regularly review the API documentation of the platforms you are working with. Tools such as Swagger can help document and update your code automatically.
In addition to that, using an abstraction layer can shield your application from immediate disruptions. You could redefine your API calls within your service layer and manage changes centrally.
public class FacebookService {
public User getUser(String userId) {
try {
// Call the latest Facebook API
return apiClient.fetchUser(userId);
} catch (APIVersionException e) {
// Handle exception - possibly log and notify to update API
throw new CustomAPIException("API version is outdated, please update.");
}
}
}
With this design, if the Facebook API changes, only the FacebookService
needs to be updated, minimizing the potential impact on other parts of your application.
5. User Fields Management
Overview
When making API requests to retrieve user information, it's common for platforms to return a large number of fields, of which only a few may be necessary for your application.
Solution
Use fields
parameter in social APIs to minimize data retrieval and optimize performance.
public User retrieveUserData() {
// Only retrieving essential fields
String[] fields = { "id", "name", "email" };
return facebookTemplate.fetchObject("me", User.class, fields);
}
By fetching only necessary fields, you can reduce the payload size and potentially avoid hitting rate limits sooner.
Closing the Chapter
Spring Social is a powerful tool for integrating social media into Java applications, but it certainly presents its own set of challenges. Addressing authentication issues, managing rate limits, handling dependency conflicts, adapting to API changes, and optimizing user data retrieval are critical skills to master.
By implementing the solutions outlined above, you should be well-equipped to tackle these challenges while enhancing the efficiency and reliability of your Spring Social application.
Additional Resources
- Spring Social Documentation
- Facebook API Documentation
- Rate Limiting Best Practices
Remember, maintaining a proactive approach is the key to overcoming challenges in software development—you'll thank yourself later for the effort! Happy coding!