Navigating Diamond Problems in Default Methods of Java
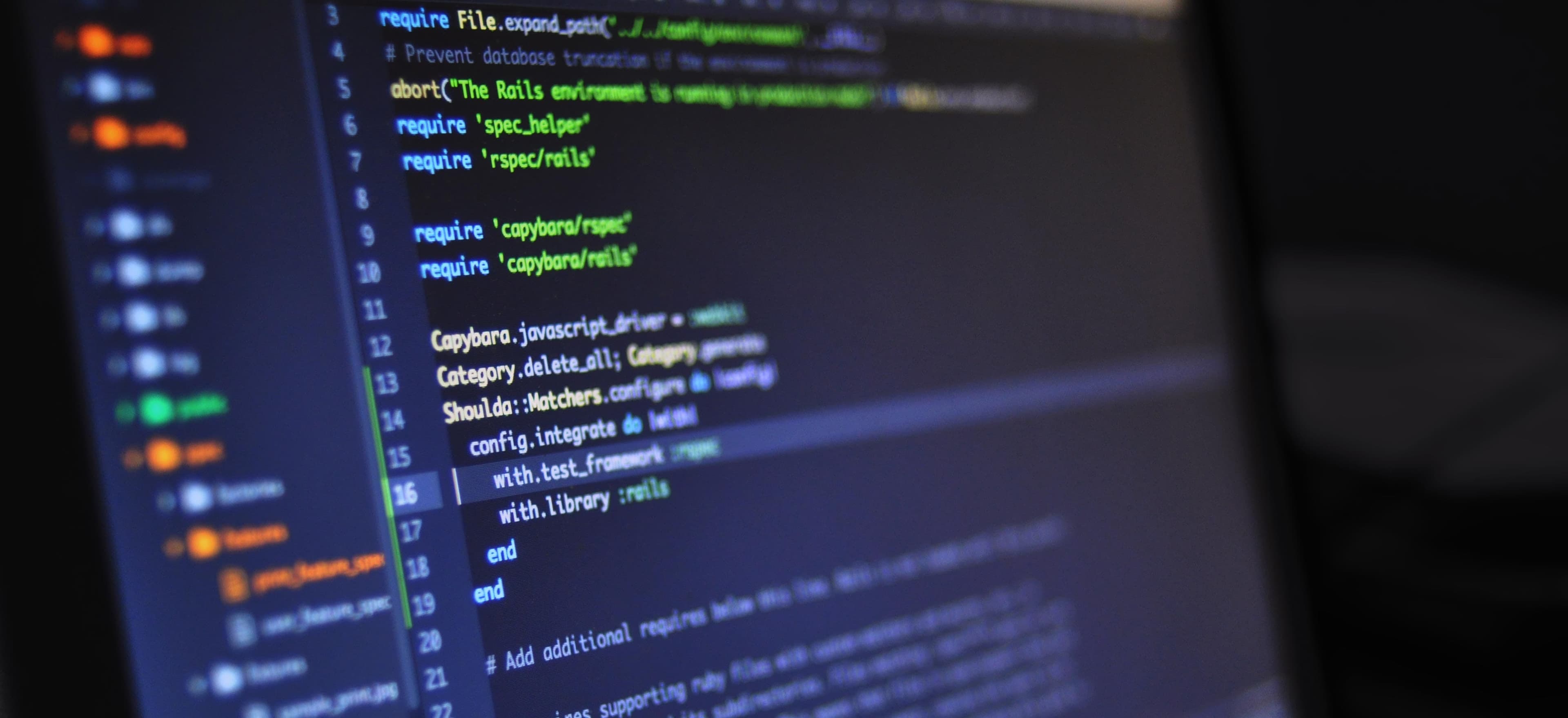
- Published on
Navigating Diamond Problems in Default Methods of Java
Java has evolved significantly since its inception, introducing features that enhance the functionality and flexibility of the language. One such feature is the introduction of default methods in interfaces with the arrival of Java 8. These methods allow developers to add new functionality to interfaces without breaking existing implementations. However, they also introduce complexities, notably the "diamond problem." This blog post will delve into the diamond problem in default methods of Java, explore how to navigate it, and present possibilities that enhance your coding experience.
What is the Diamond Problem?
The diamond problem emerges when a class inherits from two interfaces that contain methods with the same signature. This conflict occurs when both interfaces provide their own implementation of the method, leading to ambiguity regarding which method should be invoked.
Visualizing the Diamond Problem
To put it in perspective, consider the following scenario:
A
/ \
B C
\ /
D
In this diagram:
A
is an interface with a default method.B
andC
are interfaces that extendA
and provide their own implementations of the same method.D
is a class that implements bothB
andC
.
When D
tries to access the method in question, the Java compiler raises a conflict. It does not know whether to call the implementation defined in B
or that in C
.
Example to Illustrate the Problem
Let’s illustrate the diamond problem with a practical example.
interface A {
default void show() {
System.out.println("Show from A");
}
}
interface B extends A {
default void show() {
System.out.println("Show from B");
}
}
interface C extends A {
default void show() {
System.out.println("Show from C");
}
}
class D implements B, C {
@Override
public void show() {
System.out.println("Show from D");
}
}
In this code:
- Interfaces
B
andC
both extendA
and provide their ownshow()
implementations. - Class
D
implements both interfaces, leading to ambiguity.
Compilation Error
If we try to compile this code, we get an error indicating that class D
inherits several methods from the interfaces. This is the diamond problem.
Resolving the Diamond Problem
To resolve the diamond problem, Java requires you to override the method in the implementing class. In our previous example, class D
explicitly overrides the show()
method.
class D implements B, C {
@Override
public void show() {
B.super.show(); // You can choose which interface's method to invoke
}
}
Why It's Important to Override
Overriding the method gives you complete control. This allows you to specify which implementation you want to use or to create a completely new implementation tailored to your needs.
Moreover, if you do not provide an implementation in D
, the compiler will not know which version to call, and this ensures clarity and maintainability in code.
Understanding Access to Super Interfaces
When you override a method, you can choose to call the superclass implementation. This is done using the super
keyword with the interface name.
For example:
class D implements B, C {
@Override
public void show() {
B.super.show(); // Explicitly calling B's implementation
C.super.show(); // Explicitly calling C's implementation
System.out.println("Show from D");
}
}
Explanation of the Code
B.super.show();
: This line calls theshow()
method defined in interfaceB
.C.super.show();
: This line calls theshow()
method defined in interfaceC
.- Finally, it prints "Show from D".
By doing this, you can leverage the implementations from multiple interfaces, avoiding redundancy and promoting code reuse.
Best Practices to Avoid the Diamond Problem
1. Keep Interfaces Focused
Design interfaces to be narrowly focused on a specific function. This reduces the likelihood of method conflicts down the line. The single-responsibility principle should be embraced here.
2. Avoid Deep Inheritance Trees
Deep inheritance can lead to several interfaces inheriting conflicting default implementations. Try to keep your interface hierarchy shallow.
3. Document Your Interfaces Well
Make it clear in your interface documentation which classes or components are expected to implement these interfaces. Good documentation makes it easier to understand the interface design and its implications.
4. Explicit Overriding
Always favor explicit method overriding when implementing multiple interfaces with methods of the same signature. This will increase the readability of your code and make it clear which implementations are chosen.
Key Takeaways
The diamond problem in Java's default methods, while a potential source of confusion, can be effectively managed through careful planning and explicit implementation. By calling out the method implementations you wish to use and overriding where necessary, you maintain control and clarity in your inheritance model.
Understanding and addressing the diamond problem can lead to cleaner, more maintainable code while leveraging the power of default methods. As Java continues to evolve, it is essential to explore new features critically and integrate them into your coding practices efficiently.
Further Reading
For an in-depth understanding of Java interfaces, consider exploring the following resources:
By adhering to the principles outlined in this blog, you can navigate the complexities of Java interfaces and harness their full potential. Happy coding!
Checkout our other articles