Debugging Common Spring Boot Microservices Issues in Docker
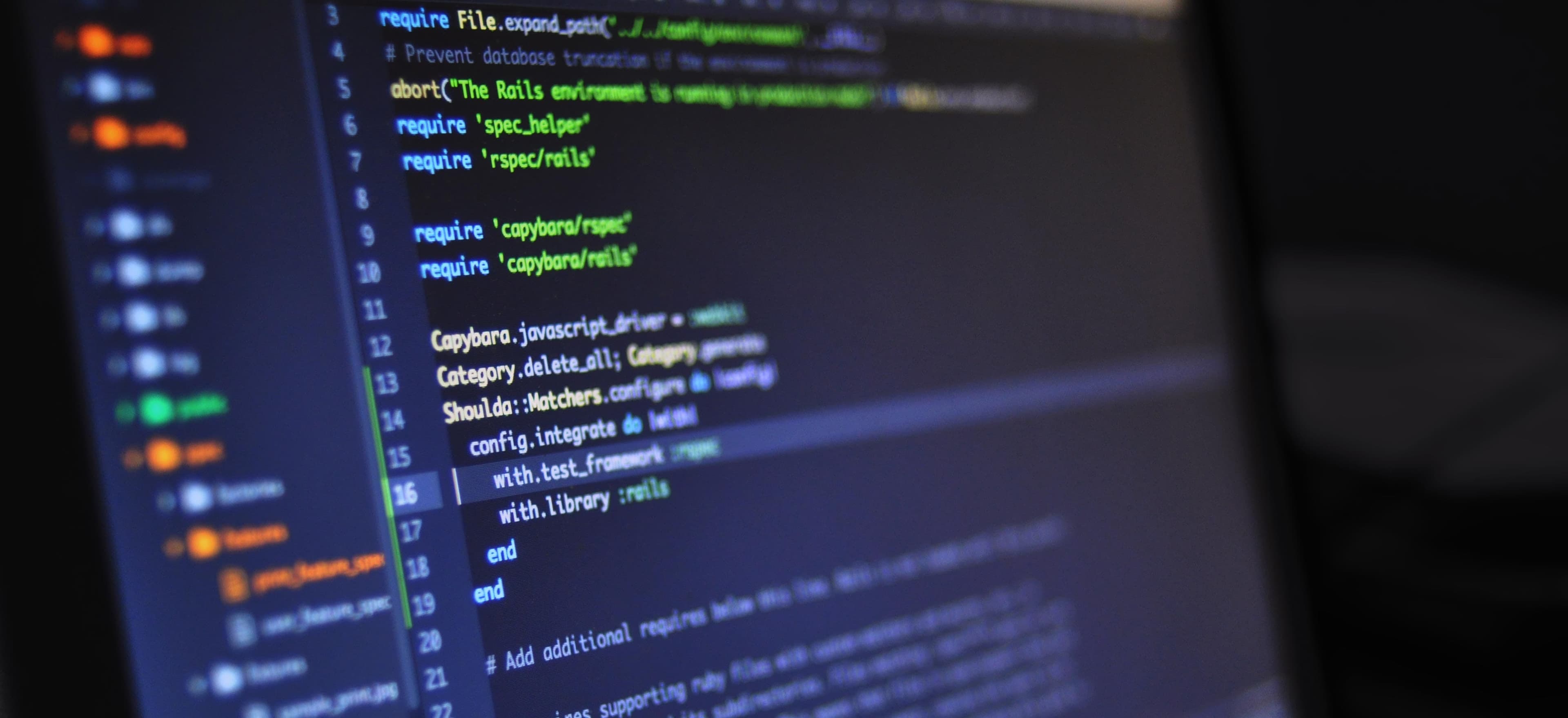
- Published on
Debugging Common Spring Boot Microservices Issues in Docker
Microservices architecture is revolutionizing how applications are built and deployed. Spring Boot, with its ease of use and capabilities, is one of the most popular frameworks for building Java-based microservices. When combined with Docker, scalability and productivity soar. However, this combination is not without its challenges. Common issues arise during development, deployment, and runtime.
In this blog post, we will discuss common issues encountered when debugging Spring Boot microservices in Docker, effective strategies to troubleshoot them, and some best practices to prevent these problems in the first place.
Table of Contents
- Understanding Spring Boot Microservices
- Common Issues While Using Docker
- Debugging Techniques
- Best Practices
- Conclusion
Understanding Spring Boot Microservices
Spring Boot is a part of the larger Spring Framework dedicated to simplifying Java application development. It provides out-of-the-box functionalities for setting up a microservice environment with minimal configuration.
Microservices architecture allows the division of application functionality into smaller, independent services that can be developed, deployed, and scaled independently. When packaged with Docker, these services can run in isolated environments, encapsulating all dependencies.
Advantages of Spring Boot Microservices
- Rapid Development: Reduced boilerplate code with Spring Boot.
- Independent Deployability: Each microservice can be deployed independently.
- Scalability: Easy to scale individual services based on their loads.
Common Issues While Using Docker
Despite the benefits, several common issues can plague Spring Boot microservices running in Docker. Let's explore some of these.
1. Configuration Issues
Microservices often come with multiple configurations depending on the environment (development, testing, production). This diversity can lead to configuration mismatches.
Solution: Use Spring Cloud Config to manage configuration across microservices seamlessly. It leverages a centralized configuration server from which services can pull their configuration properties.
2. Networking Problems
One common issue developers face is container networking. By default, Docker containers can’t communicate unless they are on the same network. This can lead to timeouts and connection errors.
Solution: Use Docker Compose to define networks in your docker-compose.yml
file. This ensures all services can communicate effectively.
version: '3'
services:
service-a:
image: service-a:latest
networks:
- my-network
service-b:
image: service-b:latest
networks:
- my-network
networks:
my-network:
In this configuration, both Service A and Service B are included in the same network, allowing them to communicate seamlessly.
3. Resource Limitation
Docker containers have resource limits. An inadequate allocation can lead to performance issues, crashes, or unresponsive services.
Solution: Specify resource limits in your docker-compose.yml
file to ensure each service has enough memory and CPU.
services:
service-a:
image: service-a:latest
deploy:
resources:
limits:
cpus: '0.1'
memory: 100M
Here, Service A is allocated a strict CPU limit of 0.1 and a memory constraint of 100 MB.
4. Logs and Monitoring
When debugging microservices, accessing logs can often be cumbersome. Unlike traditional applications, logs from different services get scattered across multiple places.
Solution: Use a centralized logging solution like ELK Stack (Elasticsearch, Logstash, Kibana) for better log management. This can consolidate logs from all microservices into a single database for easier access.
Debugging Techniques
Given the common issues outlined above, here are some effective debugging techniques to resolve problems within your Spring Boot microservices in Docker.
1. Logging Configuration
Add effective logging to your Spring Boot applications. The framework provides various logging options, including Logback and SLF4J, making it easier to tailor logs according to the environment.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
private static final Logger logger = LoggerFactory.getLogger(MyController.class);
@GetMapping("/hello")
public String hello() {
logger.info("Request received at /hello endpoint");
return "Hello, World!";
}
}
In the above code, every request to the /hello
endpoint is logged, which can help trace issues during runtime.
2. Docker Logs
Use the Docker command-line interface to access logs from a specific container. The command below retrieves logs from a running container.
docker logs <container-id>
Combining this with the -f
flag allows you to follow log updates in real time:
docker logs -f <container-id>
3. Remote Debugging
For severe issues, you may need to debug your Spring Boot application remotely. You can start your Docker container with JVM debugging enabled.
docker run -p 8080:8080 -p 5005:5005 -e JAVA_OPTS="-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=*:5005" service-a:latest
This configuration allows you to attach your IDE's debugger to the running application via port 5005. With an IDE like IntelliJ or Eclipse, you can set breakpoints to inspect application behavior closely.
4. Health Checks
Docker allows you to define health checks that automatically assess the state of a service. Utilize this feature to ensure the health of your microservices before routing traffic.
services:
service-a:
image: service-a:latest
healthcheck:
test: ["CMD", "curl", "-f", "http://localhost:8080/actuator/health"]
interval: 30s
timeout: 10s
retries: 5
In this example, Docker periodically checks if the health endpoint of Service A is responding correctly.
Best Practices
Navigating common issues can be cumbersome. Here are some best practices to help avoid problems altogether.
- Container Orchestration: Use Kubernetes or Docker Swarm to better manage container deployments, scaling, and networking complexities.
- Service Discovery: Implement a service discovery mechanism (like Eureka or Consul) for microservices to find each other dynamically.
- Centralized Configuration: Leverage Spring Cloud Config throughout your applications to maintain consistency across different environments.
- Continuous Integration/Continuous Deployment (CI/CD): Automate the deployment process to reduce human errors associated with manual deployments.
To delve into proper container orchestration with Kubernetes, check out Kubernetes Official Documentation.
In Conclusion, Here is What Matters
Debugging Spring Boot microservices in Docker may present numerous challenges, but by understanding the common issues and employing effective debugging strategies, you can streamline your development process. Using best practices not only aids in preventing problems but significantly enhances the overall performance and reliability of your applications.
Ultimately, as you navigate the landscape of microservices, remember that a combination of continuous learning and robust tooling will lead to your success. Happy coding!
Checkout our other articles